To delete a branch in Git, use the `git branch -d` command followed by the branch name for local branches, or `git push --delete` followed by the remote branch name for remote branches.
Here are the code snippets for both cases:
# Delete a local branch
git branch -d branch_name
# Delete a remote branch
git push --delete remote_name branch_name
Understanding Branches in Git
What is a Git Branch?
A Git branch is essentially a pointer to a snapshot of your changes. It allows you to diverge from the main line of development and continue to work independently without affecting other branches. This feature is crucial in version control as it enables multiple developers to work on different features, fix bugs, or explore new ideas simultaneously.
Types of Branches
In Git, you generally deal with two types of branches:
-
Local Branches: These are the branches you create and manage locally in your Git repository. They are specific to your local environment and can be freely modified without impacting the central repository until you decide to push your changes.
-
Remote Branches: These branches exist on remote repositories, such as those on GitHub or GitLab. They are used to track branches from the remote repository and can be interacted with through clone, fetch, pull, or push operations.
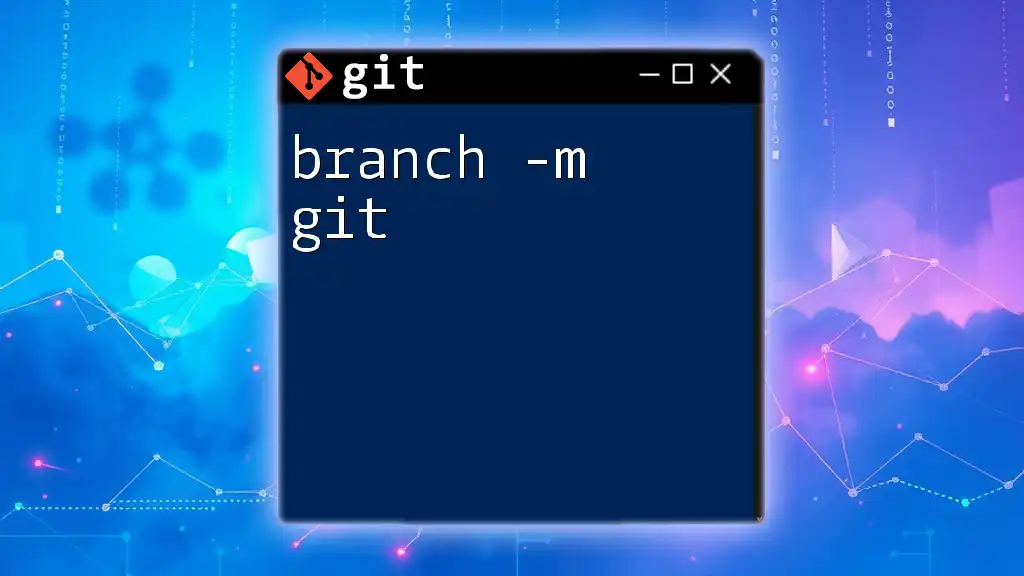
Why Should You Delete Branches?
Clean Up Your Repository
Maintaining an organized repository is crucial for productivity. As new branches are created for features or bug fixes, old and unused branches may pile up. Deleting obsolete branches helps reduce clutter, streamlining your workflow and making it easier to navigate your project.
Preventing Confusion
An excess of branches can lead to chaos—not only for you but also for your team members. By deleting branches that are no longer needed, you can prevent merge conflicts and simplify the understanding of the current development landscape. This clarity is especially beneficial for onboarding new team members who may find it difficult to navigate a cluttered branch structure.
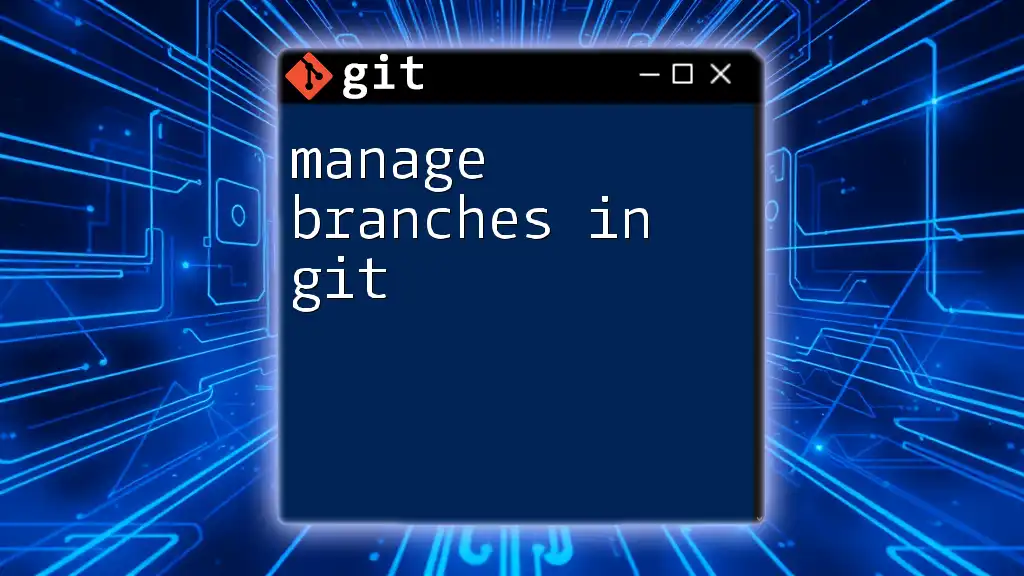
How to Delete Branches in Git
Deleting a Local Branch
- Using the Git Command Line
Deleting a local branch is straightforward. You can use the following command:
git branch -d <branch-name>
This command deletes the specified branch but only if it has already been fully merged into the main branch (often `main` or `master`). If the branch has changes that are not merged, Git will prevent this deletion to protect your work.
For example:
git branch -d feature/my-new-feature
If the branch has not been merged and you still wish to delete it, you can use a force delete.
- Force Deleting a Branch
To force delete a branch irrespective of its merge status, use:
git branch -D <branch-name>
This command should be used with caution since it will remove the branch and its unmerged changes permanently.
Example of a force delete:
git branch -D feature/my-old-feature
Deleting a Remote Branch
- Using the Git Command Line
To delete a branch from a remote repository, the command syntax is:
git push <remote-name> --delete <branch-name>
Replace `<remote-name>` with the name of your remote repository, typically `origin`, and `<branch-name>` with the branch you want to delete.
For example:
git push origin --delete feature/my-old-feature
This command will remove the branch from the specified remote location.
- Using GitHub Interface (or GitLab, etc.)
If you prefer a graphical interface, you can delete branches directly from platforms like GitHub or GitLab. Navigate to the branches section of your repository, find the branch you wish to delete, and look for the corresponding delete button. This method offers a user-friendly alternative that doesn't require command-line proficiency.
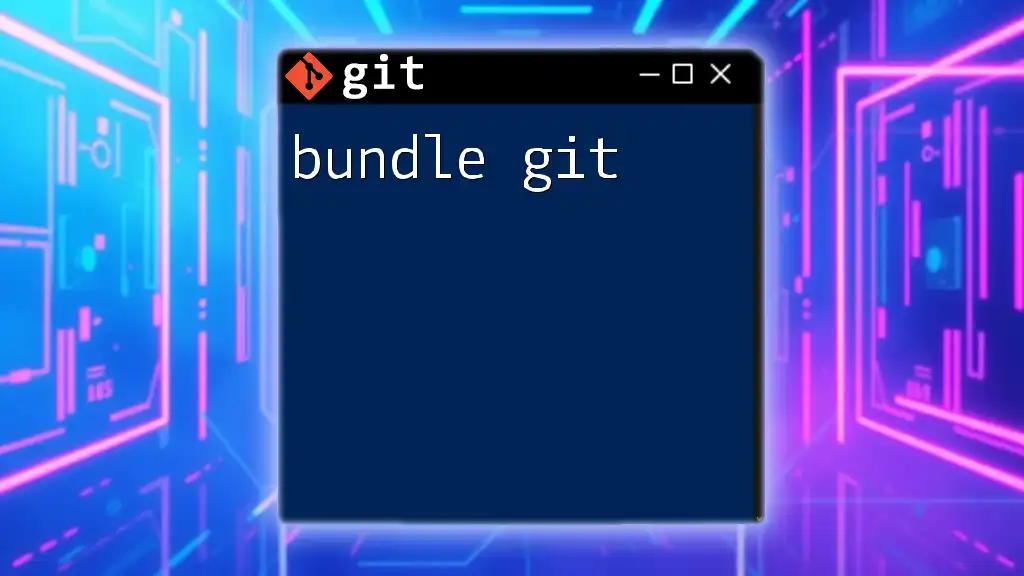
Confirming Branch Deletion
Listing Local Branches
To verify that a local branch has been deleted, you can list all local branches using:
git branch
The output will display only those branches that are still present in your local repository.
Listing Remote Branches
To check if a remote branch has been successfully deleted, list all remote branches with:
git branch -r
This command will confirm whether the specified remote branch still exists or has been removed.
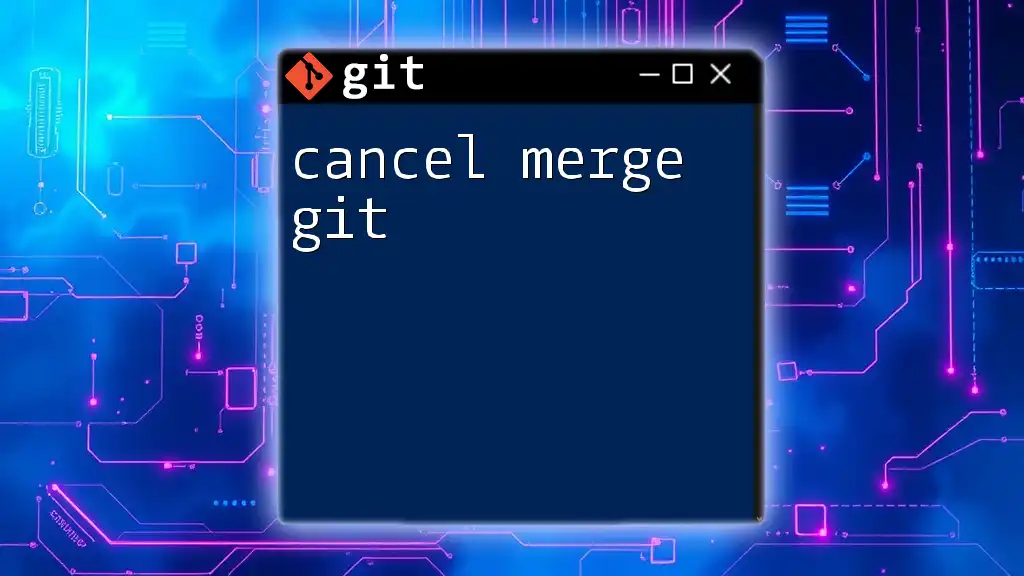
Common Issues and Troubleshooting
Error Messages Upon Deletion
When attempting to delete a branch, you may encounter error messages. For instance, if you receive an error stating that you cannot delete the branch, this usually indicates that the branch contains unmerged changes. In such cases, consider reviewing the branch or using force deletion if appropriate.
Tips for a Smooth Deletion Process
-
Always check if changes are merged. Before deleting a branch, ensure that any essential changes are integrated into the main branch to prevent loss of work.
-
Maintain a consistent naming convention. This practice can help clarify which branches are temporary and which are intended for ongoing development, making it easier to identify branches that may need deletion.
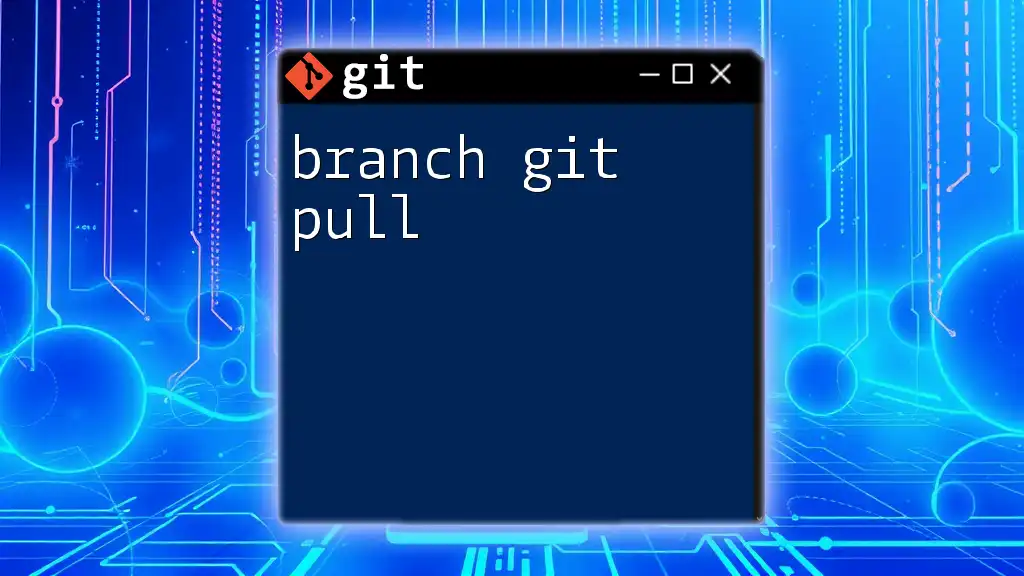
Best Practices for Managing Branches
Regular Cleanup Sessions
Make branch cleanup a regular task in your workflow. Scheduling periodic reviews of your branches will help to keep your repository neat and manageable.
Delegating Responsibilities
Assign team members the task of managing branches effectively. This division of labor ensures that branch management is not overlooked and encourages accountability within the team.
Keeping a Branching Strategy
Develop a clear branching strategy that outlines guidelines for creating, using, and deleting branches. A formal strategy can improve collaboration and streamline the development process for all team members.
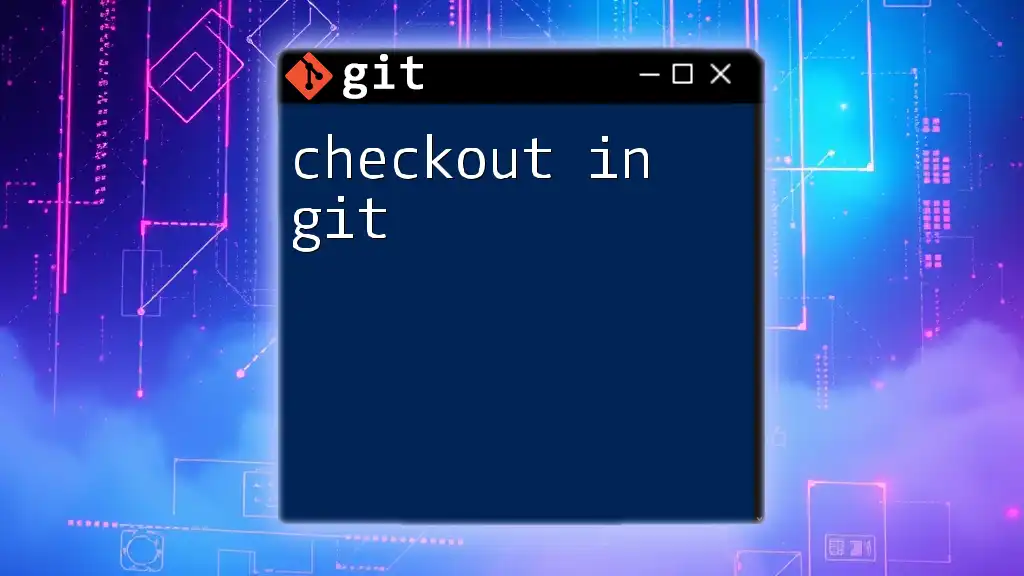
Conclusion
Effective branch management is essential for utilizing Git to its fullest potential. By understanding how to properly delete branches—both local and remote—you can maintain a clean, organized repository that promotes better collaboration and productivity. Embracing these practices will not only enrich your own workflow but also enhance the experience for your entire team.
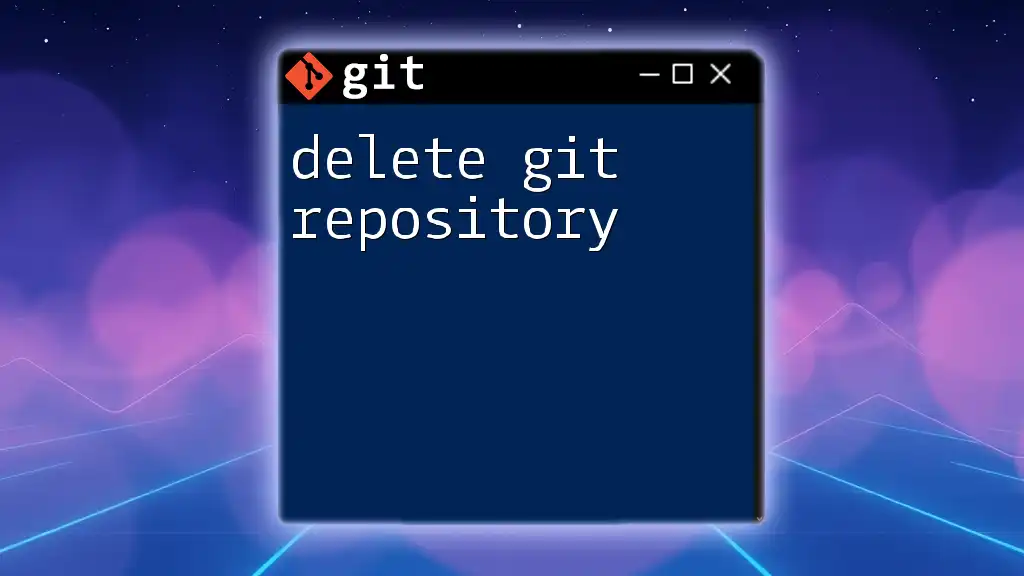
Additional Resources
For further reading, consider exploring the official Git documentation on branch management for more detailed guidelines. Additionally, various Git GUI tools can simplify the process and provide visual insights into your branches.
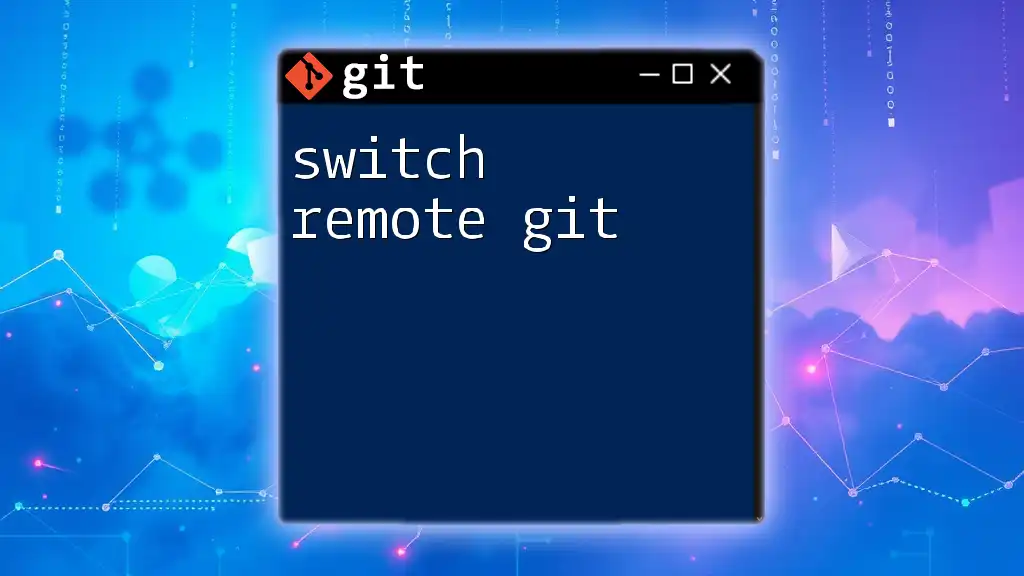
Call to Action
If you found this guide helpful, consider subscribing for more concise tutorials on essential Git commands. Additionally, we welcome your feedback on other Git topics you would like to learn about!