When the terminal asks for a password to download a Git repository, it is typically due to the repository being hosted on a remote server that requires authentication to access, such as when using SSH or HTTPS protocols.
Here's an example of the command you might use to clone a private repository using HTTPS, which would prompt for your password:
git clone https://github.com/username/private-repo.git
Understanding Git Repositories
What is a Git Repository?
A Git repository is a collection of files that are tracked and managed by Git. This powerful version control system allows developers to collaborate on projects while maintaining a comprehensive history of changes. There are two main types of repositories: local repositories, which exist on your computer, and remote repositories, hosted on platforms like GitHub, GitLab, and Bitbucket.
Common Ways to Access a Git Repository
You can access Git repositories via two main protocols: HTTPS and SSH.
- HTTPS is straightforward and secure, often requiring a username and password when interacting with private repositories.
- SSH (Secure Shell) uses key-based authentication, allowing you to avoid password prompts once set up correctly, thereby streamlining your workflow.
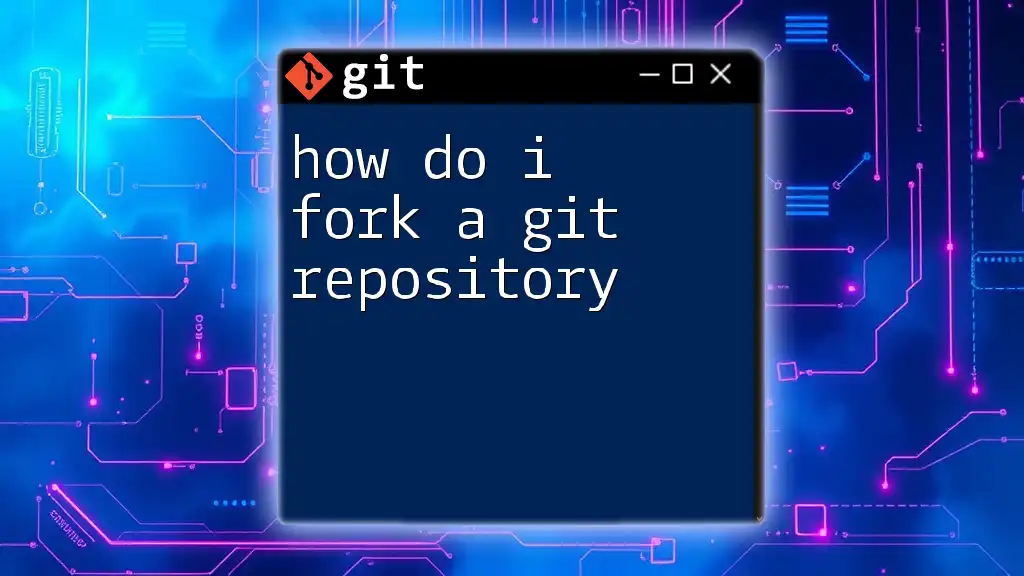
When Does the Terminal Ask for a Password?
Nature of Authentication
When you perform operations such as cloning, pushing, or pulling from a Git repository, the terminal checks your authentication status. If the repository requires verification, particularly for private repositories, the terminal will prompt you for your credentials.
Scenarios Where Password Prompt Occurs
- Cloning a public repository: Generally, public repositories on platforms like GitHub do not require a password as they are accessible to anyone.
- Cloning a private repository: For private repositories, the terminal will prompt for your username and password.
- Pushing changes: When pushing changes to a private repository, you will also encounter the password prompt for authentication.
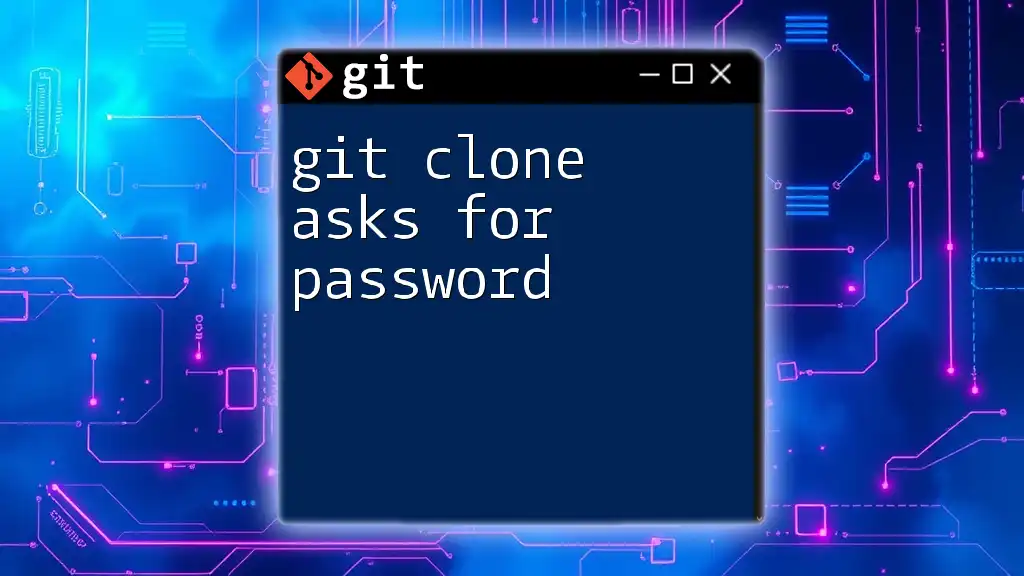
Reasons for Password Prompts
Using HTTPS vs. SSH
When using HTTPS to access a repository, you need to provide your credentials every time unless you use a credential helper to cache them temporarily. On the other hand, SSH allows you to set up a key pair that authenticates without needing a password every time, making it a preferred option among developers.
Authentication Credentials
During authentication, Git requires specific credentials—typically your username and password. For SSH, you utilize a private key that corresponds to a public key registered with the remote repository, eliminating the need for passwords in many cases.
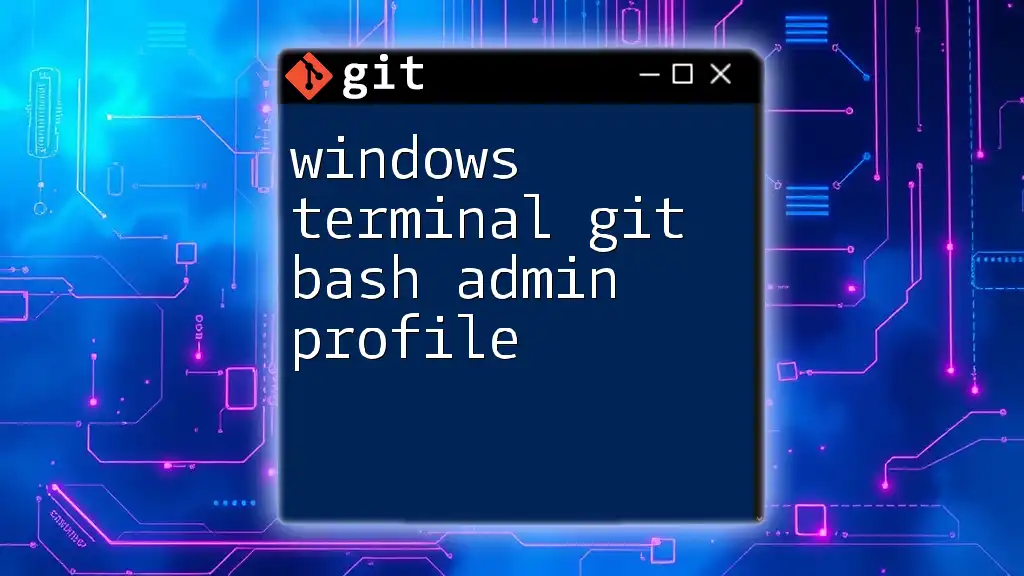
How to Manage Username and Password in Git
Caching Credentials Using Credential Helper
To simplify your interaction with Git over HTTPS, you can use the credential helper. This command instructs Git to temporarily cache your credentials:
git config --global credential.helper cache
Additionally, you can specify how long the system should cache your credentials in seconds (e.g., `credential.helper 'cache --timeout=3600'` for one hour).
Storing Credentials Securely
You also have the option to store your credentials securely using a `.netrc` file or integrating with system keychains, which encrypt and manage your credentials, making them easily retrievable during Git operations.
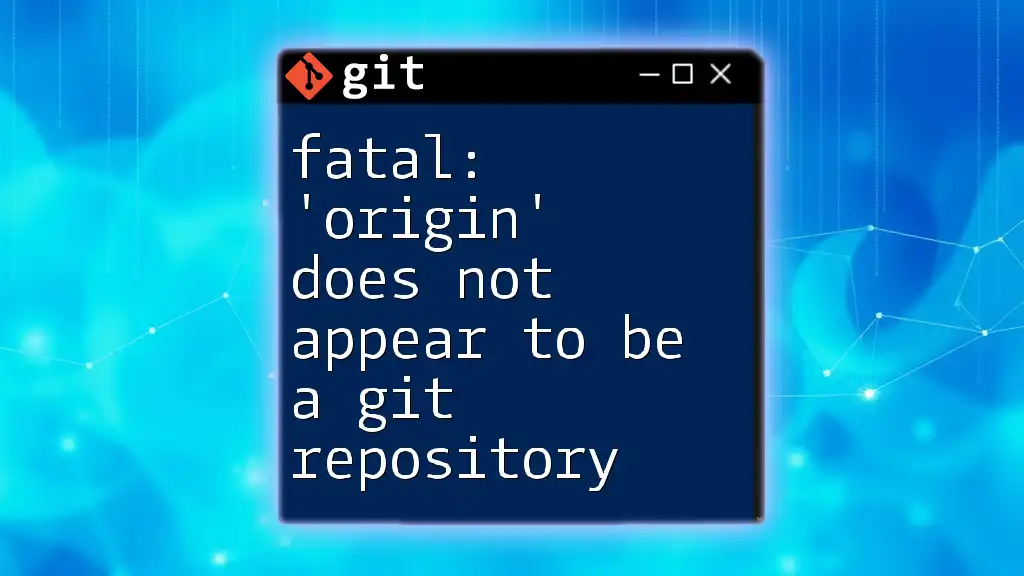
Using SSH to Avoid Password Prompts
Setting Up SSH Keys
To set up SSH, you first need to generate SSH keys if you haven't done so already. This is how you can create a new SSH key:
ssh-keygen -t rsa -b 4096 -C "your_email@example.com"
This command will generate a private-public key pair. The public key should then be added to your Git hosting service (e.g., GitHub, GitLab).
Configuring Git to Use SSH
After generating and adding your SSH key, you should switch your remote URL to utilize SSH instead of HTTPS. For instance, alter your remote URL with:
git remote set-url origin git@github.com:username/repo.git
Once configured, you should no longer encounter password prompts for pushes or pulls.
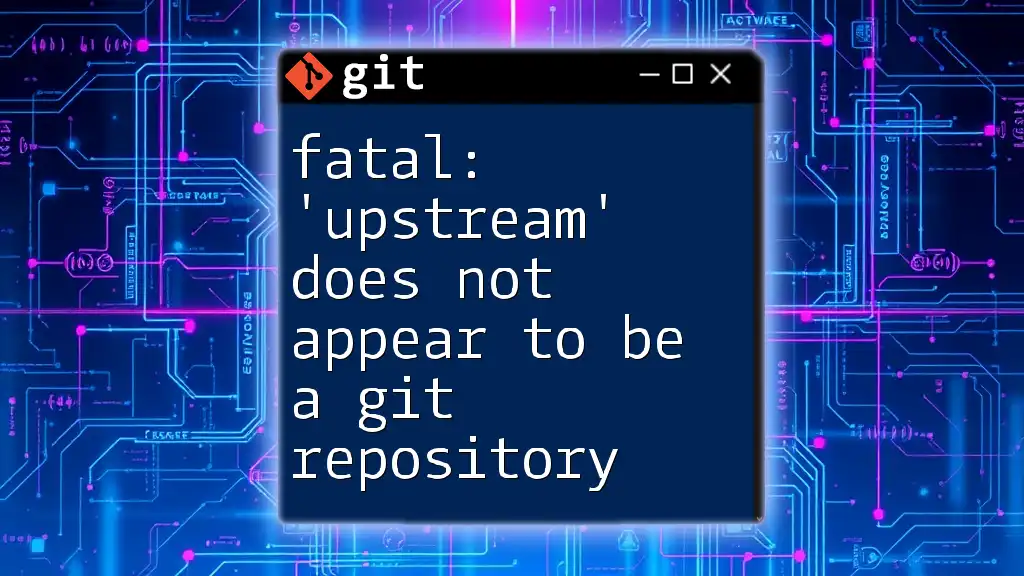
Troubleshooting Password Prompts
Common Issues and Solutions
If you continue to receive password prompts despite having followed the steps above, several common issues might be at play:
- Incorrect credentials: Double-check your username and password, particularly for HTTPS.
- SSH key not added to the ssh-agent: Ensure your SSH key is loaded into the ssh-agent.
- Misconfigured remote repository URL: Verify that the remote URL is accurately set up as SSH.
Changing Passwords and Updating Credentials
If you need to change your passwords for HTTP access, you can do so through the respective platform's settings. For SSH, if you've regenerated your key, update the public key in your Git hosting service to reflect the new key.
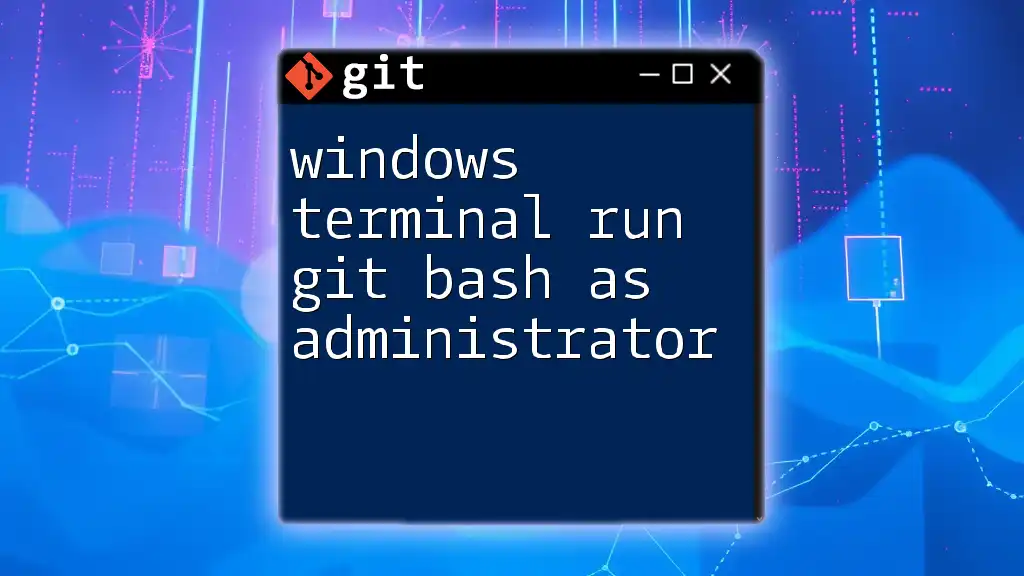
Conclusion
Understanding why the terminal asks for a password to download a Git repository is crucial for every developer. By managing your authentication methods effectively—you can streamline your workflow and minimize disruptions. Transitioning to SSH not only enhances security but also simplifies the process, allowing for a smoother coding experience.
For those looking to become more proficient with Git commands, practice is key. Dive into tutorials and engage with community resources to build confidence in using Git in your projects.