To reset a file to a previous commit in Git, use the following command, replacing `<commit-hash>` with the actual commit ID and `<file-path>` with the path of the file you want to revert:
git checkout <commit-hash> -- <file-path>
Understanding Git Commits
What is a Git Commit?
A Git commit is essentially a snapshot of your project at a specific point in time. Each commit records changes to your files, allowing you to track the evolution of your project over time. This commit history is critical as it serves as a timeline of changes, enabling you to refer back to previous versions of your files whenever necessary.
Why Use Previous Commits?
There are various scenarios where you might need to revert to a previous commit. For instance, when you realize that a bug has been introduced in the latest version or when you want to experiment with features that were present in earlier commits. Understanding the differences between methods to revert changes is crucial. While resetting a file brings it back to a previous state, reverting a commit adds a new commit that undoes the changes of the specified commit. Each approach has its place, but resetting a file provides a simpler, more straightforward way to retrieve earlier versions directly.

Prerequisites
Before diving into the practical commands, ensure that you have Git properly installed and set up. Familiarize yourself with basic Git commands and have a local repository created to practice these techniques. Having a grasp of fundamental concepts will enable you to handle more complex operations confidently.
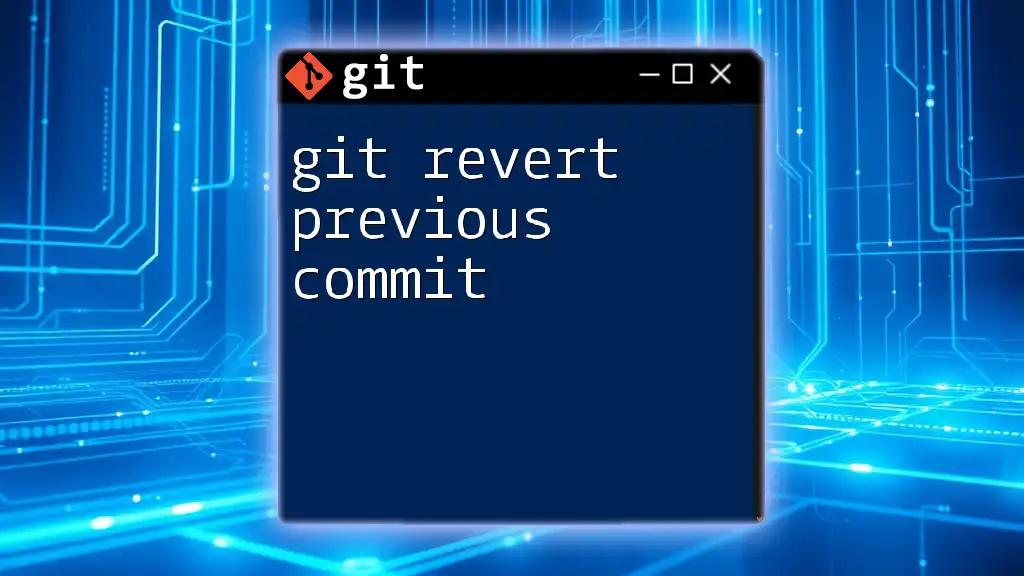
The Git Reset Command
Introduction to `git reset`
The `git reset` command plays a pivotal role in your Git workflow. It modifies the current branch’s history, allowing you to "reset" the state of your files and commits. There are three main types of resets in Git: soft, mixed, and hard.
- Soft reset: Moves the HEAD pointer without changing the working directory or index. Any changes from previous commits remain in the staging area.
- Mixed reset: The default option. This reset moves the HEAD pointer, clears the index, but leaves your working directory unchanged.
- Hard reset: This dangerous option moves the HEAD pointer and resets the index and working directory to match the specified commit, discarding all changes.
The Role of `git reset` in Resetting a File
Resetting a file allows you to revert that file to the state it was in at a specific commit, without affecting other files in your repository. You can identify the exact commit using a commit hash or move back to the last committed state.
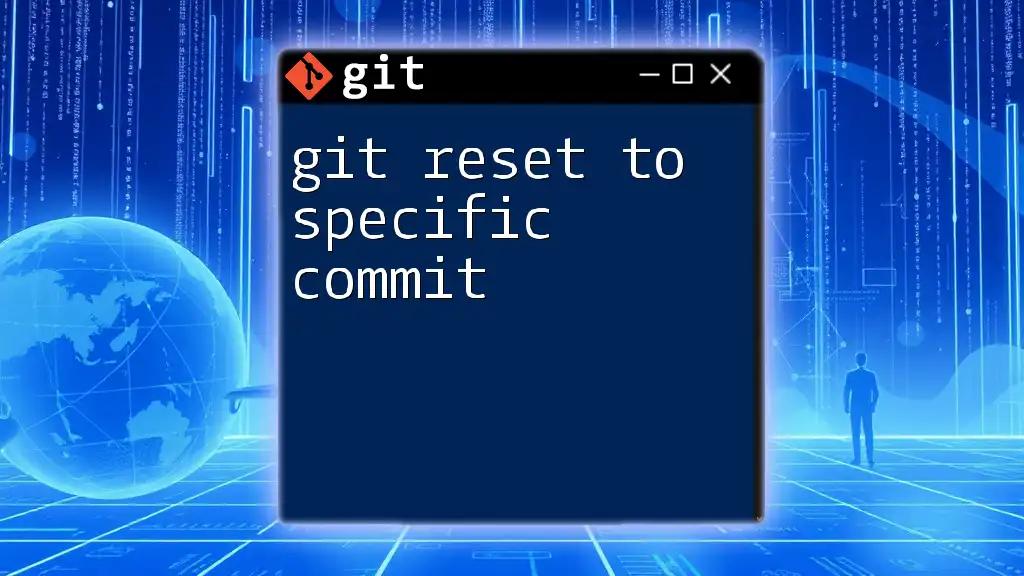
How to Reset a File to a Previous Commit
Identifying the Commit
To reset a file, the first step is to identify the commit you want to revert to. Use the following command to view your commit history:
git log --oneline
This command displays a concise list of commits with their hashes and messages. Find the commit hash associated with the version of the file you want to restore. Remember, commit hashes are unique identifiers, so take note of the hash for the specific commit you wish to revert to.
Resetting to a Previous Commit
Resetting a Single File
Once you have the commit hash, you can reset a specific file to its state at that commit. Use the following command:
git checkout <commit_hash> -- <file_path>
- `<commit_hash>` is the hash of the commit you want to reset to.
- `<file_path>` is the path to the specific file you wish to restore.
This command updates the file in your working directory to match the specified commit. It is a non-destructive operation that allows you to keep your other files intact.
Alternative Methods
In addition to `git checkout`, you can also use the `git restore` command, which is a newer addition to Git that simplifies the syntax. This method is particularly useful if you want a clear distinction between restoring files and checking out branches. Use it as follows:
git restore --source=<commit_hash> -- <file_path>
This command serves the same purpose as the checkout command, restoring the specified file to its state in the mentioned commit.
Verifying Changes
After executing the reset command, it is essential to verify that the changes have been made successfully. Use the following command:
git status
This command will display the current state of your working directory and staging area, highlighting any modifications. It’s a helpful way to confirm that the file has been reverted correctly.

Important Considerations
Impacts of Resetting a File
Resetting a file can impact collaborative workflows. If you work on a team, communicate with team members before making changes that affect shared files, as reverting to a previous file version can create inconsistencies in the project. Additionally, be cautious, as some commands can lead to loss of uncommitted changes, which may not be recoverable.
Best Practices
Foremost, consider making a backup or creating a new branch before resetting files. This allows you to experiment with changes safely and return to the original state if necessary. Understanding when to use a reset versus creating a new commit or branching off is crucial. If you are unsure about a change, using a new branch can be a safer way to experiment without risking the integrity of the main project.

Common Issues and Troubleshooting
Common Errors
While utilizing `git reset`, you may encounter errors like trying to reset a file that hasn’t been committed yet. Remember, if a file was modified but not staged before the reset command was issued, you won’t be able to restore it via this method. Always ensure your changes are committed before resetting anything.
Using `git reflog` for Recovery
If you find that you’ve inadvertently lost important changes, `git reflog` can be a lifesaver. This command records changes to the HEAD, which can help you recover lost commits. Use it as follows:
git reflog
This command provides a log of where your HEAD has been; you can identify the commit to which you want to return. To reset to that lost commit, use `git reset --hard <commit_hash>`, bearing caution as this command will erase any uncommitted changes.
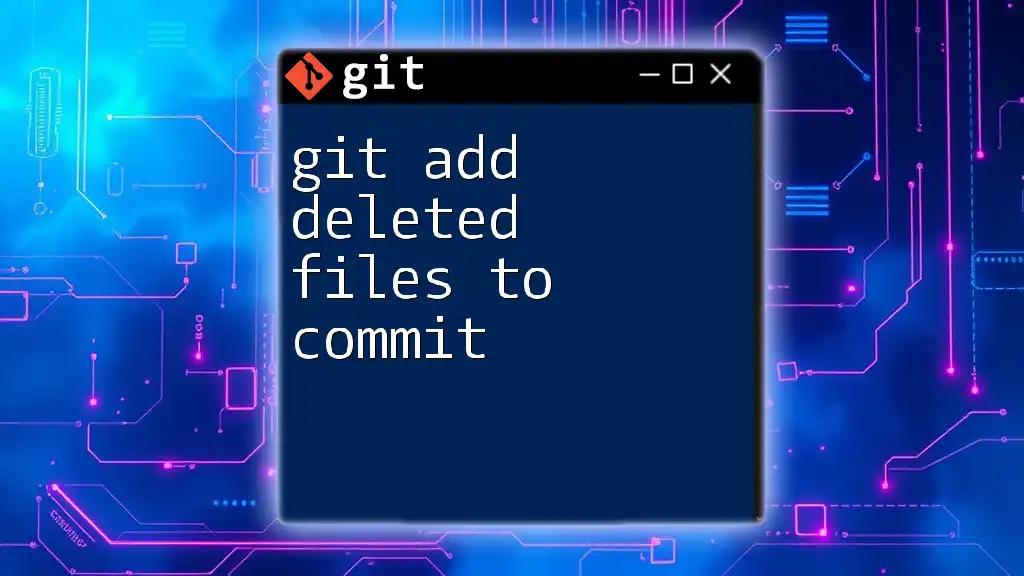
Conclusion
Understanding how to reset a file to a previous commit in Git is a vital skill for maintaining control over your project's history. As you practice and become more familiar with these commands, you’ll enhance your development workflows and mitigate risks associated with changes. Embrace the learning process, and don’t hesitate to explore further resources to master Git commands and best practices.