"Happy Git with R" refers to the integration of Git version control with R programming, enabling users to efficiently manage their R projects through Git commands.
Here's a code snippet demonstrating how to initialize a new Git repository in an R project:
git init
Getting Started with Git in R
Installing Git
To begin using Git with R, the first step is installing Git on your machine. You can download Git from the [official Git website](https://git-scm.com/downloads). Follow the installation prompts specific to your operating system. After installation, verify that Git is installed by opening the command line and typing:
git --version
This command will display the installed Git version, indicating a successful installation.
Configuring Git for R
Once Git is installed, it’s time to configure it to work effectively with R. Start by setting your global username and email, which Git will use to identify commits. Execute the following commands in the command line:
git config --global user.name "Your Name"
git config --global user.email "you@example.com"
To incorporate Git into your RStudio workflow, ensure that you enable version control for your projects. When creating a new project, select the option to create it as a Git repository. RStudio integrates Git functionalities, allowing seamless version control right from the interface.
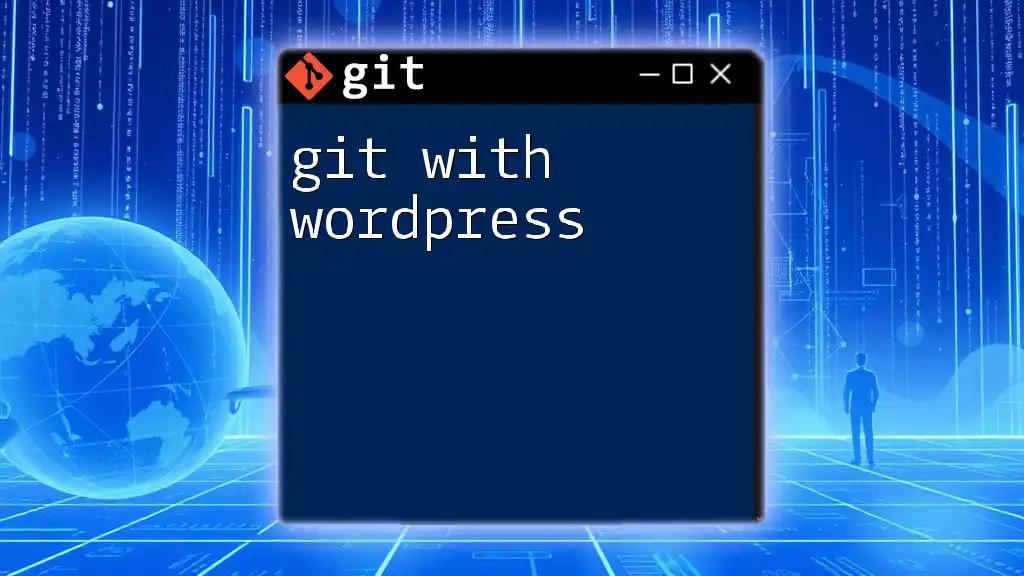
Core Git Concepts for R Users
Repositories
A repository (often referred to as a repo) is a storage space for your project, where all your files, history, and version control are managed. To create a new Git repository, navigate to your project directory in the command line and run:
git init my_project
This command initializes a new repository in the specified folder.
Committing Changes
Every time you finish a feature or fix a bug, you’ll want to save your changes with a commit. A commit is essentially a snapshot of your changes, allowing you to revisit previous versions later. To make your first commit, add files to the staging area and commit the changes:
git add .
git commit -m "Initial commit"
Using the `git add .` command stages all your modified files, and `git commit -m` saves that state to the repository with a descriptive message.
Branching and Merging
Branches in Git are vital for parallel development. They allow you to work on features or experiments without affecting the main codebase. To create and switch to a new branch, use the following commands:
git branch new_feature
git checkout new_feature
This creates a branch named `new_feature`, and the next command switches you to that branch. Once you finish the new feature, merge your changes back to the main branch (often called `main` or `master`) with:
git checkout main
git merge new_feature
Merging incorporates the changes from `new_feature` into `main`, keeping your project up to date while preserving the integrity of the code.
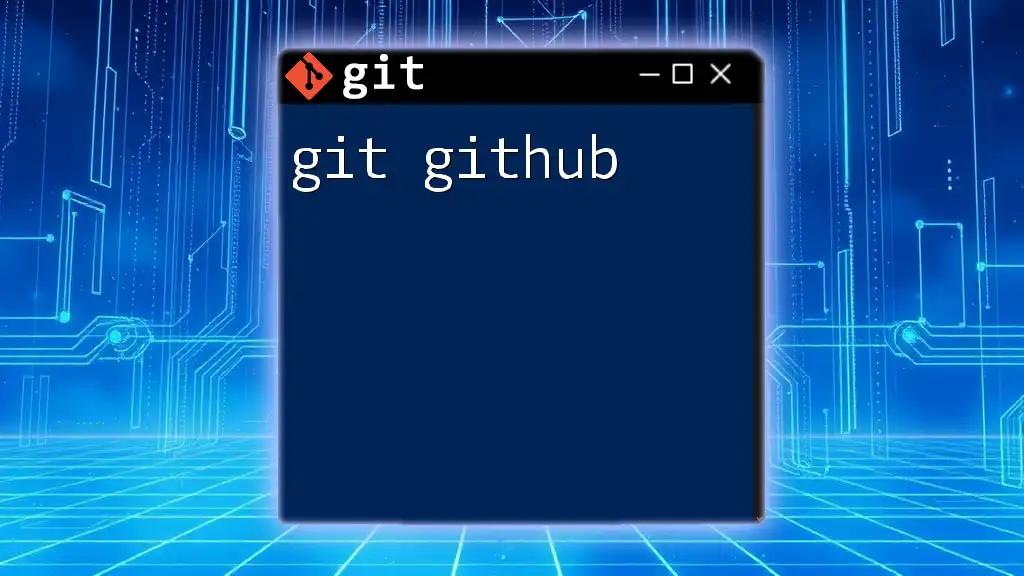
Advanced Git Commands for R
Stashing Changes
During development, you may find the need to temporarily set aside changes that you are not ready to commit. This is where stashing comes in handy. Use the following commands to stash your changes:
git stash
This command saves your local modifications to a stack and reverts your working directory to the last commit. To reapply these stashed changes later, use:
git stash apply
This retrieves the last stashed change, allowing you to continue right where you left off.
Working with Remote Repositories
Remote repositories like GitHub or GitLab allow for collaboration and backup. To add a remote repository, use the command:
git remote add origin https://github.com/username/repo.git
After adding a remote, you can push your local changes to it with:
git push origin main
To fetch recent changes from the remote repository, use:
git pull origin main
This command synchronizes your local codebase with any changes made by collaborators.
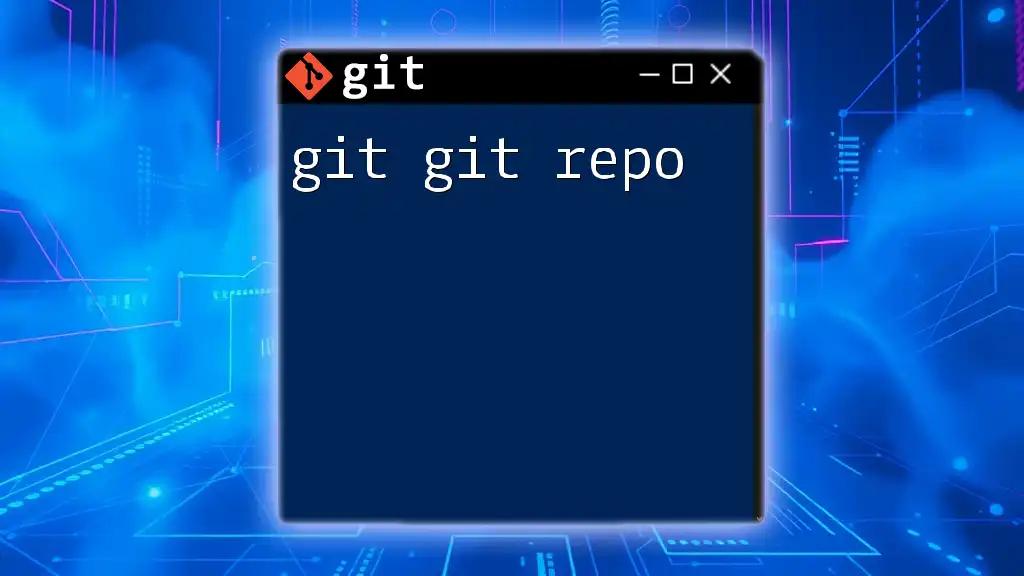
Using Git with R Projects: Best Practices
Structuring Your R Projects with Git
Employing a well-organized directory structure in your R projects can greatly enhance your productivity. A typical structure might include folders for data, scripts, and outputs. Create a `.gitignore` file in your project’s root directory to specify files that Git should ignore, such as large datasets or temporary files. This keeps your repository clean and focused on relevant code and data.
Commit Message Guidelines
Writing effective commit messages is crucial for maintaining a readable project history. A good commit message should briefly summarize the changes being made, following a format like "Added [feature] to [module]". This practice makes it easier for you and your collaborators to understand the project’s evolution.
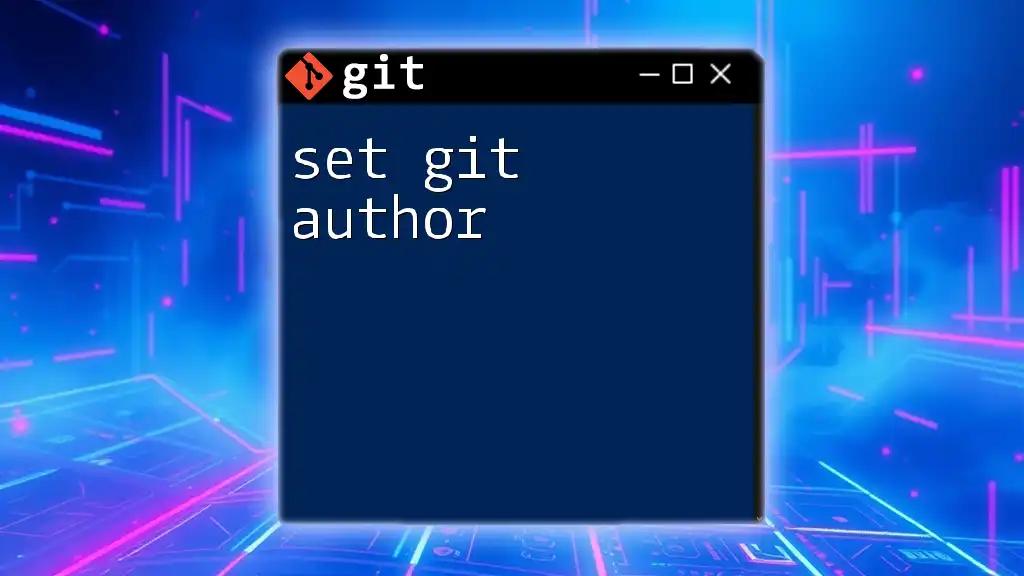
Troubleshooting Common Git Issues in R
Common Errors and Their Solutions
When using Git, you may encounter errors such as merge conflicts. These happen when two branches have changes in the same part of a file. To resolve merge conflicts effectively, you’ll need to manually edit the affected files and then stage the resolved files for committing.
If mistakes are made, Git provides powerful tools to rectify them. Commands like `git revert` and `git reset` can be used to undo changes safely, ensuring your project's integrity remains intact.
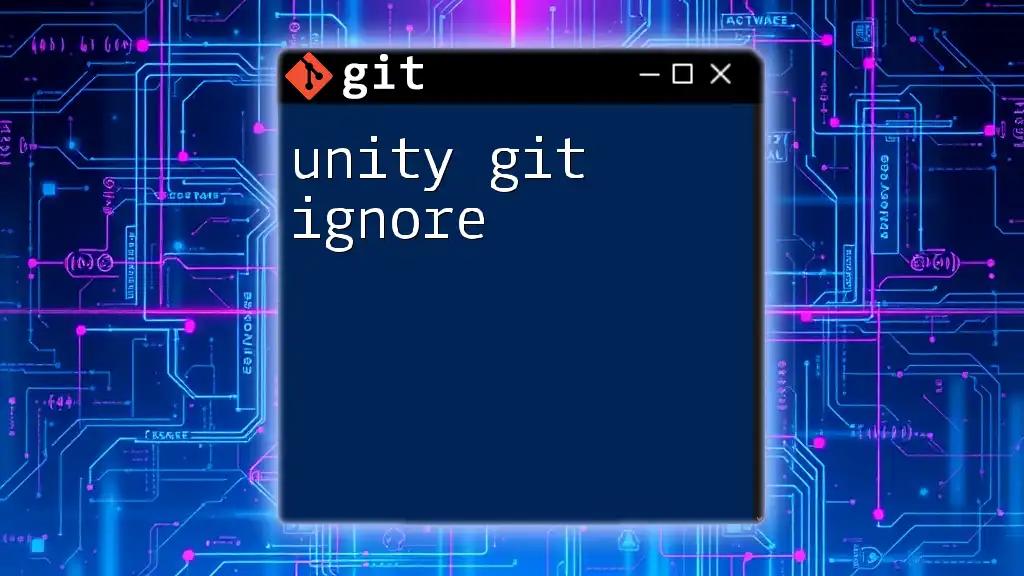
Integration of Git with R Markdown
Utilizing Git with R Markdown
R Markdown documents are popular for generating reports and dynamic analysis. When using Git in R Markdown projects, be sure to commit your Rmd files regularly to keep track of changes. Proper version control in R Markdown not only facilitates collaboration but also aids in creating reproducible research that can be easily shared.
Best Practices for Reproducible Research
Using Git helps ensure that your research is reproducible. By version controlling your analysis, collaborators can go back to any point in the project, see what data and code were used, and replicate results effectively.
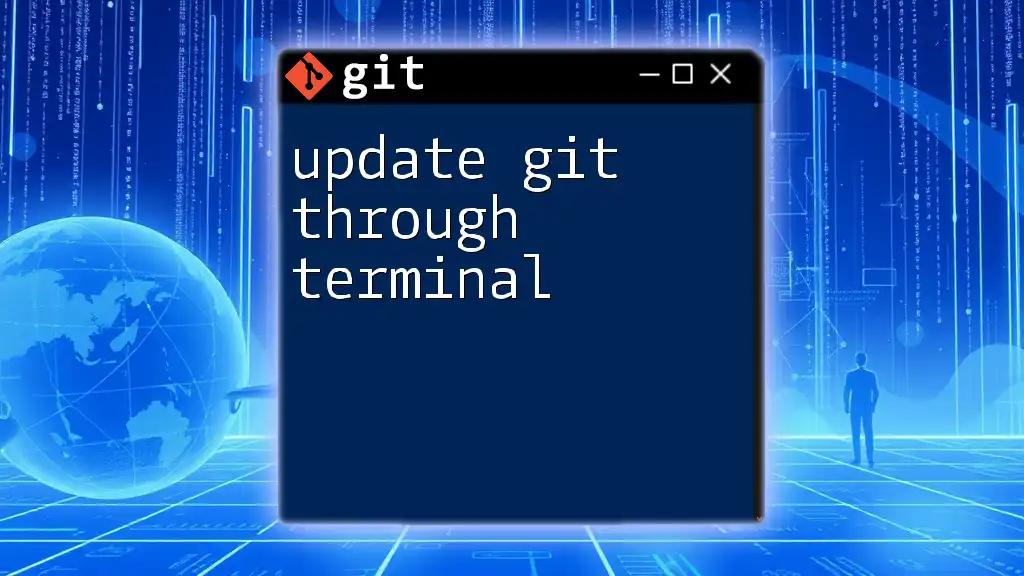
Conclusion
In summary, establishing a happy git with R workflow significantly enhances your programming efficiency and collaboration skills. By mastering essential Git commands and employing best practices, you create a robust environment for managing your R projects. Remember, consistent practice and usage of Git will lead you to become proficient in version control, ultimately benefiting your development and analysis processes.
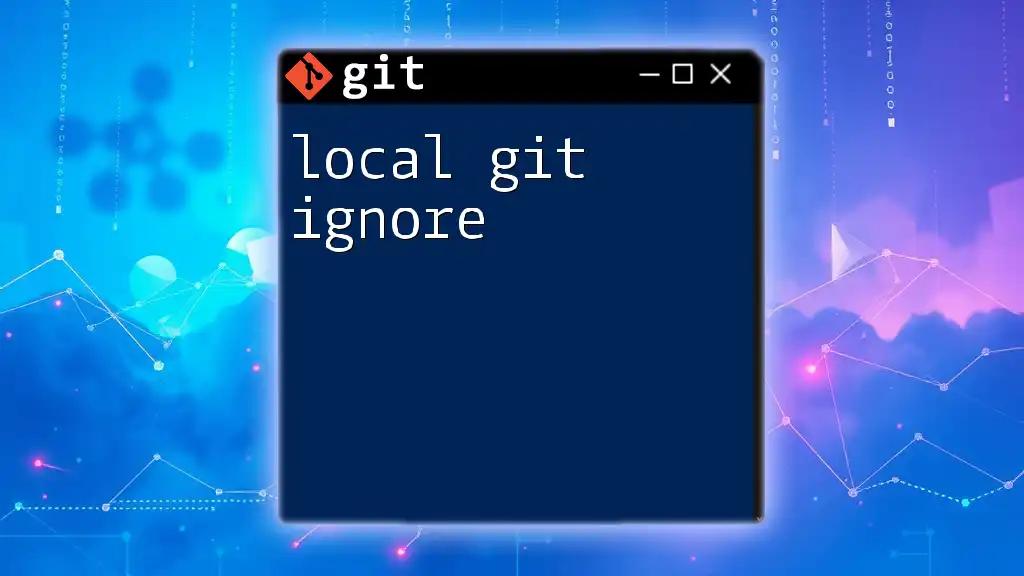
Additional Resources
For further enriching your understanding of Git and R, consider exploring additional tutorials and documentation on both Git and R programming. Resources such as [Pro Git](https://git-scm.com/book/en/v2) and the [RStudio documentation](https://rstudio.com/resources/training/what-is-git/) can provide valuable insights and advanced techniques.