To search for a specific string or pattern across all branches in a Git repository, you can use the following command:
git grep 'search_term' $(git rev-list --all)
Understanding Git Branches
What are Git Branches?
In Git, branches are essentially pointers to commits. Think of a branch as an isolated environment for development and experimentation. Each branch allows developers to work on new features or fix bugs without interfering with the main codebase, often referred to as the master or main branch. When you're ready to merge your changes, you can bring those new updates into the main branch seamlessly.
Types of Git Branching Strategies
Branches can be utilized in various strategies, such as:
- Feature Branching: Each new feature is developed in its own branch, which can later be merged into the main branch.
- Git Flow: This outlines a very structured workflow, involving multiple types of branches for features, releases, and hotfixes.
By understanding branching strategies, you’ll appreciate the importance of searching across all branches. With multiple branches, finding specific code changes becomes crucial, particularly when trying to understand project evolution or troubleshooting issues.
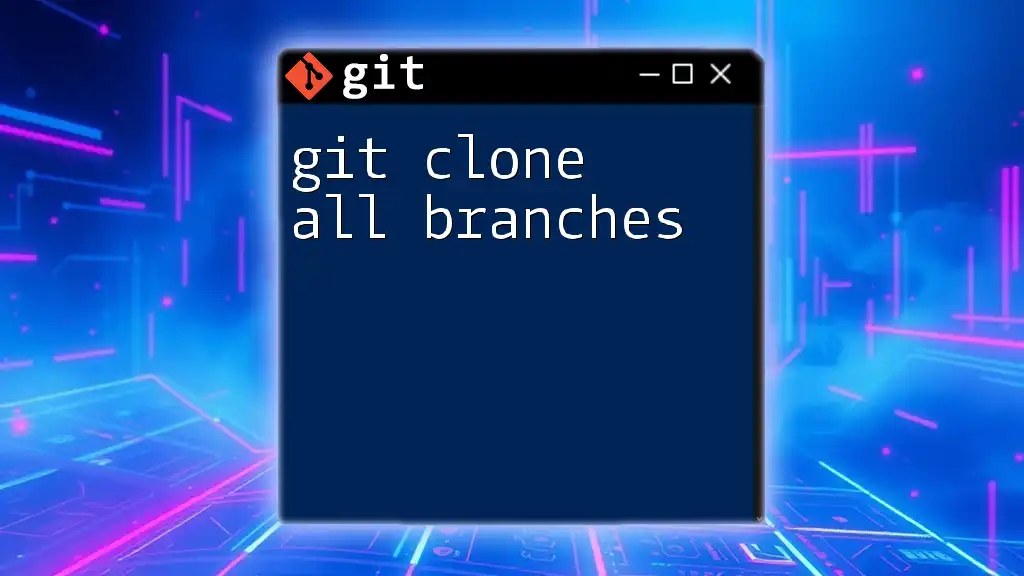
Why You Might Need to Search Across All Branches
Common Use Cases
Searching across branches in Git isn't just a nice-to-have; it's often essential for various reasons:
- Finding Commit History: If you need to locate a specific commit that fixes a bug, you may need to look across all branches.
- Tracking Bugs/Regressions: When a bug arises, the most efficient way to understand its origin is by examining related branches where changes were made.
- Reviewing Code Before Merging: Prior to merging a feature branch into the main branch, you'll want to examine modifications made across other branches.
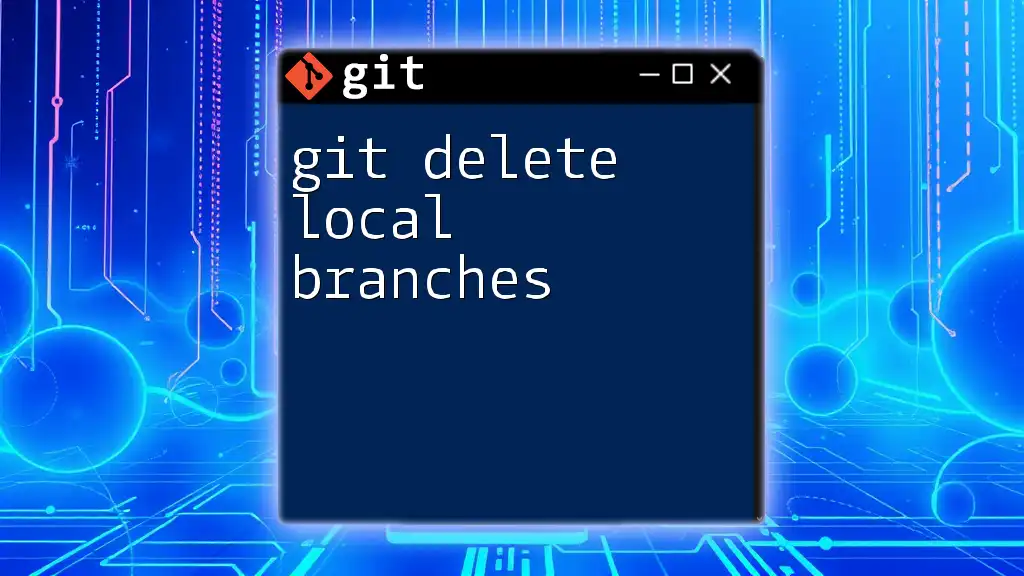
Basic Commands for Searching in Git
Searching for Commits
One of the first commands you'll want to familiarize yourself with is `git log`. This command allows you to view the commit history of your repository.
To search for specific commits across all branches, you can use:
git log --all --grep="search-term"
This command searches through commit messages in every branch for the specified search term. It’s an effective way to quickly locate relevant commits without having to manually check each branch.
Searching for File Changes
Sometimes, you might want to compare how a file has evolved across branches. Here, `git diff` is your go-to command.
To find changes in a file between two specific branches, run:
git diff branch1..branch2 -- path/to/file
This command shows you the differences in that specific file between the specified branches, helping you understand changes more clearly.
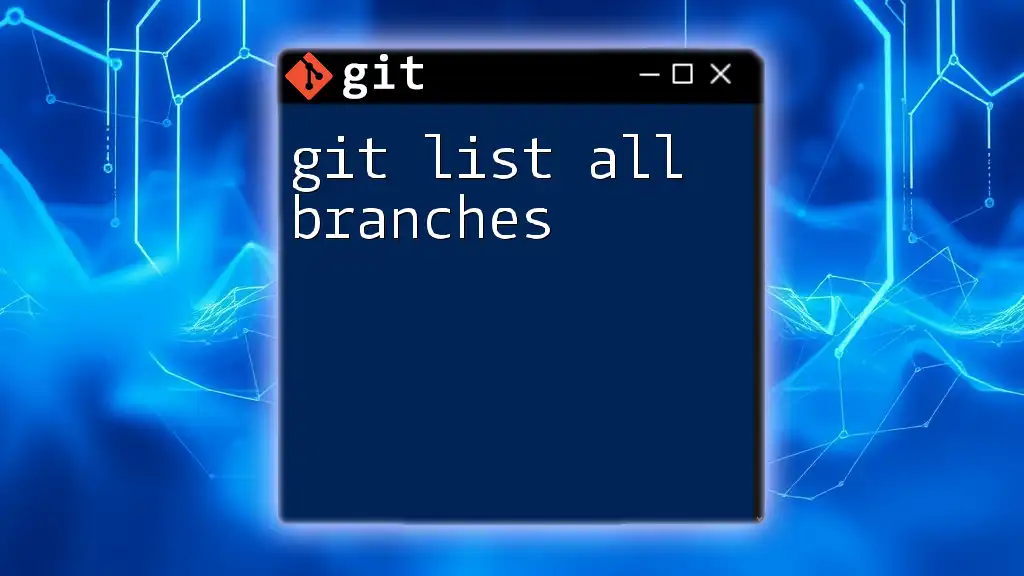
Advanced Git Search Techniques
Using `git grep`
For searching text patterns directly within your codebase, the `git grep` command proves invaluable. It allows you to search for a specific string across your entire history.
To find occurrences of "search-text" across all branches, you can use:
git grep "search-text" $(git rev-list --all)
This command uses `git rev-list --all` to target every commit, ensuring your search encompasses the entire repository's history. It's efficient and powerful for locating code snippets or comments that may have been inadvertently modified.
Searching for Specific Words Across All Branches
Another method for locating specific pieces of text in your commit history is by using a combination of `git log` and `grep`. For example, if you want to track down appearances of a particular word or phrase, you can do:
git log --all -p | grep "word-or-phrase"
This searches through all commits and their patches (indicated by `-p`) for the entered word or phrase, enabling you to trace the context in which it was used.
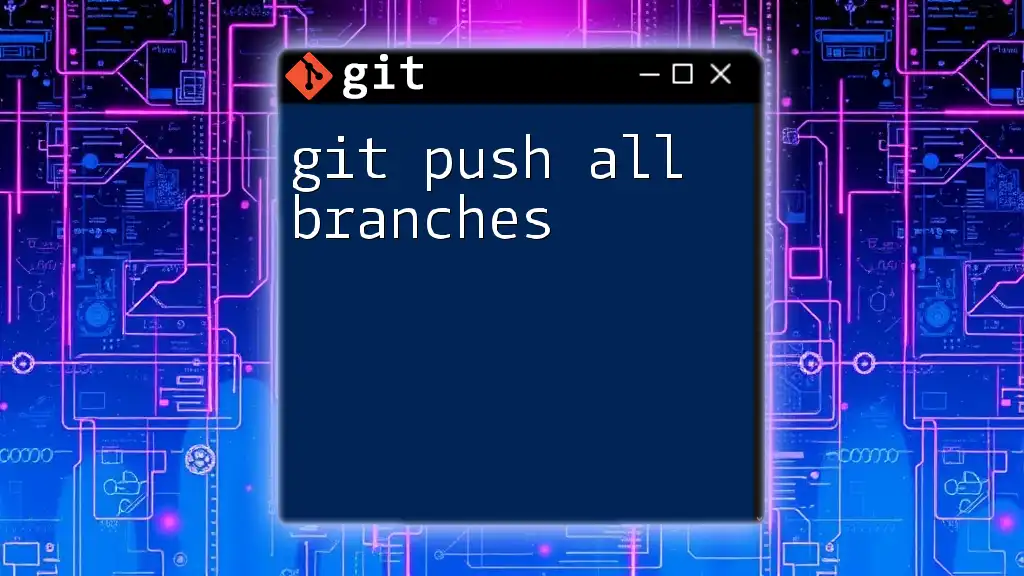
Automated Git Search Tools
Introduction to Git Tools
While command-line tools are powerful, GUI tools and extensions can streamline your search process, particularly for those who prefer a visual interface.
Popular Tools for Searching in Git
-
SourceTree:
- This Git GUI provides a user-friendly interface and includes search capabilities, making it easy to find information quickly without delving into terminal commands.
-
GitKraken:
- This tool stands out with its visual commit graphs and powerful search features, enabling users to navigate through branches and commits effectively.
-
VS Code Git Integration:
- If you're using Visual Studio Code, its built-in Git support can help by allowing you to search and navigate through your codebase and commits easily.
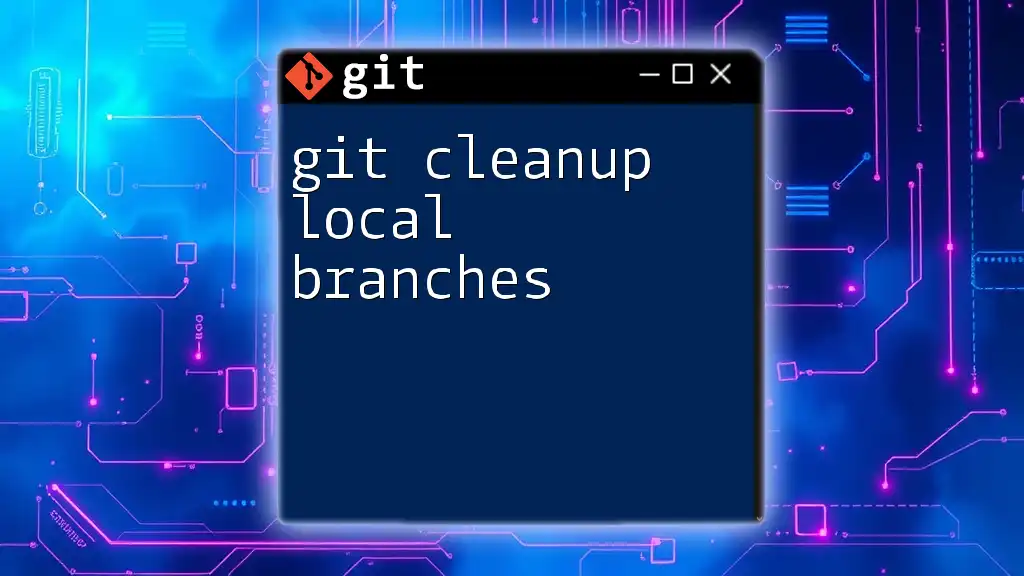
Best Practices for Efficient Searching
Keeping Your Repository Organized
Consistent commit messages are essential. When developers adhere to a standard format, finding specific commits becomes a far simpler task. It’s recommended to include the issue number or feature description in the commit messages for better tracking.
Regularly Cleaning Up Branches
Old branches can clutter your repository and make searches less efficient. Regular maintenance, such as deleting merged branches, contributes to a clean environment. To delete a branch that has been merged, use:
git branch -d branch-name
For other branches that haven't been merged yet, if you're sure you want to delete them, you can use the `-D` flag:
git branch -D branch-name
This keeps your branch list manageable and enhances the performance of commands that search across branches.
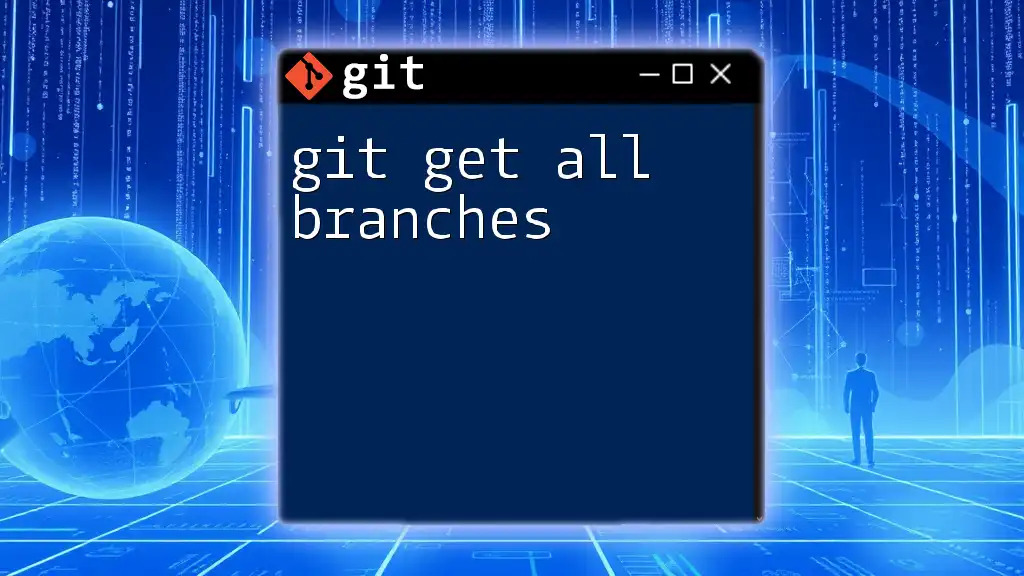
Conclusion
Searching across all branches in Git is an invaluable skill that can significantly enhance your development workflow. With a clear understanding of Git commands and available tools, navigating and retrieving information becomes more straightforward. By incorporating best practices surrounding organization and branch management, you’ll position yourself for success in any coding project, ensuring that vital code changes are always just a search away.
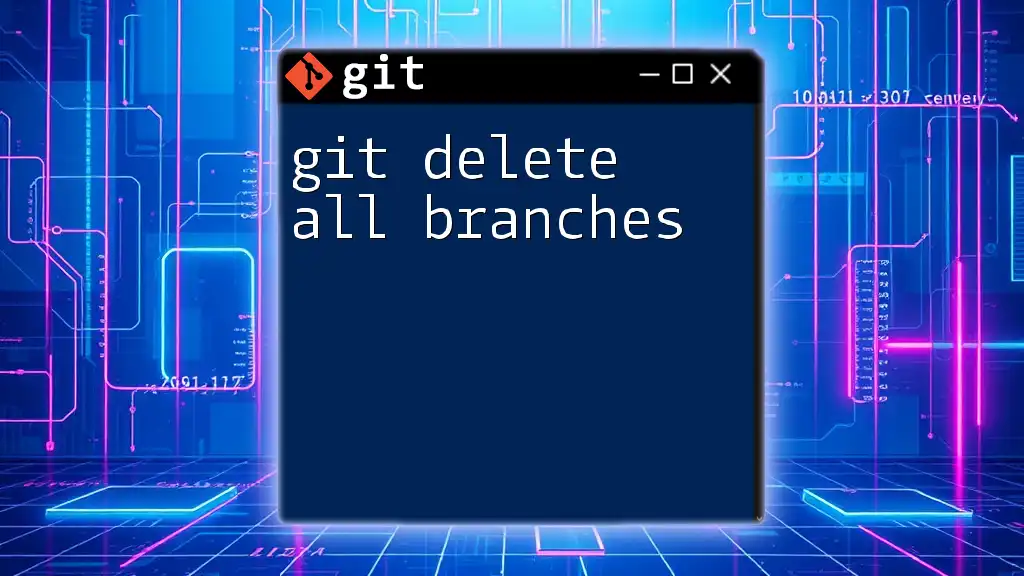
Call to Action
For those eager to deepen their Git knowledge, explore our range of resources and courses designed specifically for mastering Git commands. Stay connected with us through our newsletter and social media for the latest updates and insights!