In Git, before you can merge changes, you must either move or remove any conflicting files in your working directory that are preventing the merge.
git stash # Stash your changes
git merge <branch_name> # Attempt the merge
git stash pop # Apply the stashed changes back
Understanding the Merge Process in Git
What is Merging in Git?
Merging in Git is a fundamental process that allows developers to combine changes from different branches into a single branch. It plays a crucial role when multiple users collaborate on the same project. Merging enables seamless integration of features, bug fixes, and enhancements into the main codebase, preserving the history of all changes made along the way.
How the Merge Process Works
The merging process typically involves several steps depending on whether a fast-forward merge or a three-way merge occurs.
In a fast-forward merge, Git simply moves the pointer of the branch up to the latest commit of the merged branch without creating a new commit. This is possible only when there are no new commits on the branch to which you are merging.
A three-way merge occurs when both branches have diverged. Here, Git will create a new merge commit that references both branches, incorporating changes from both.
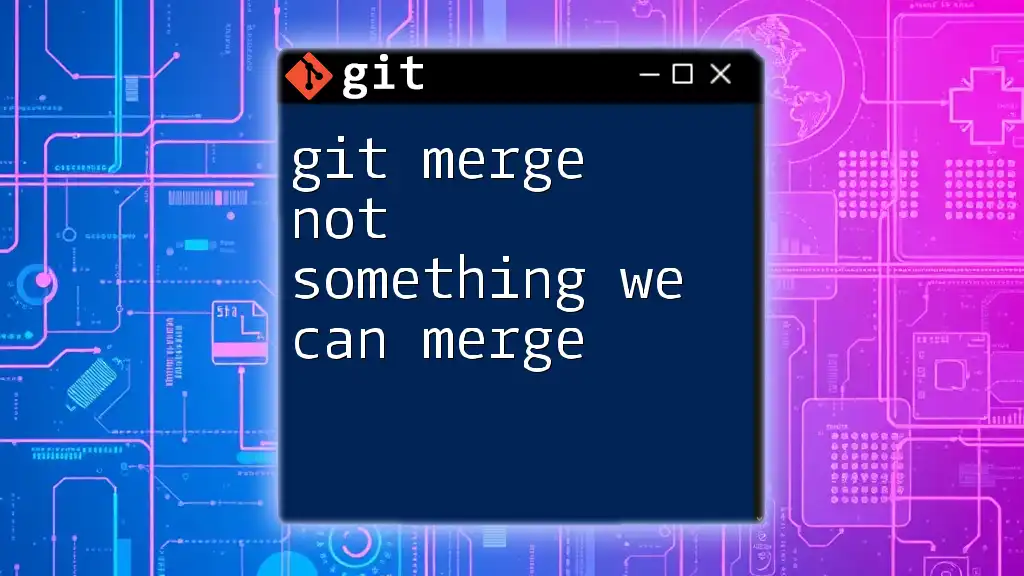
The Error: "Please Move or Remove Them Before You Can Merge"
What Causes the Error?
The error message "please move or remove them before you can merge" typically arises in a situation where your working directory contains untracked files that would be overwritten by the merge operation. Essentially, Git prevents you from proceeding with the merge to safeguard your untracked changes. Common scenarios leading to this error include:
- Adding files to your current branch that are not tracked.
- Attempting to merge a branch that would affect existing untracked files.
The Impact of Ignoring the Error
Ignoring this merge error is not advisable; it can lead to inconsistencies in your codebase and potentially overwrite untracked files unintentionally. Maintaining a clean repository is essential to ensure that your commit history remains clear and that all changes are documented.
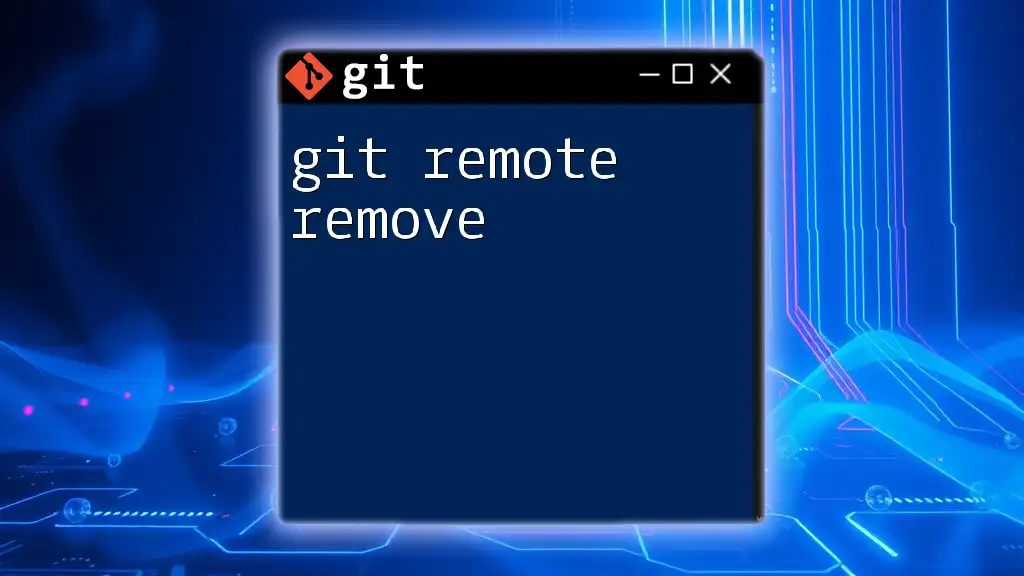
Diagnosing the Issue
Checking for Untracked Files
To identify untracked files, you can use the command:
git status
This command will show you a summary of the current status of your repository, highlighting untracked files and any changes made to tracked files. Understanding this output is critical for diagnosing merge issues.
Identifying the Conflicting Files
Within the output of `git status`, look for files labeled as "untracked files." These files are the culprits causing the merge error. Being aware of the types of files that may lead to this issue is essential.
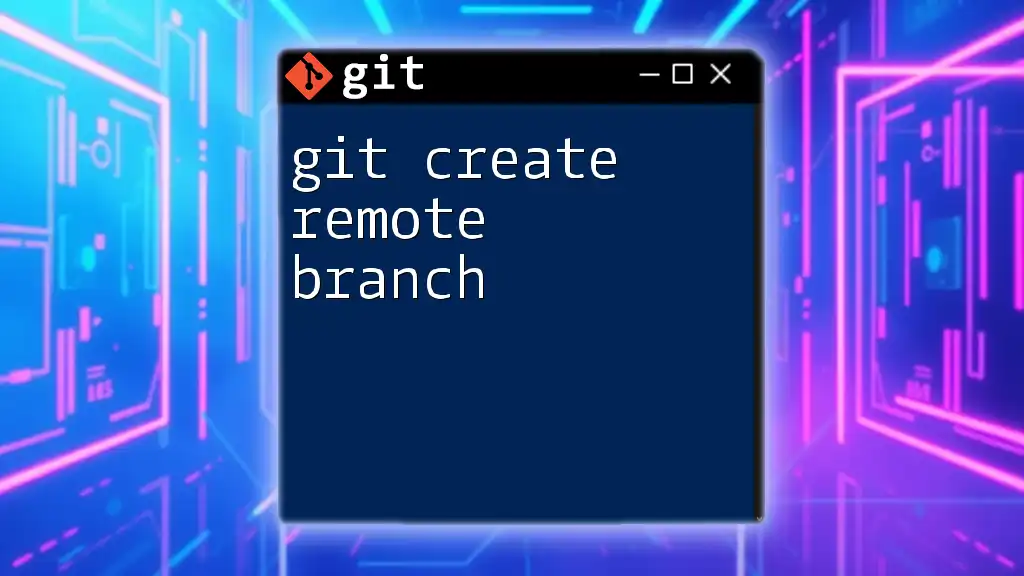
Resolving the Error
Option 1: Moving Untracked Files
When to Move Files
Moving untracked files is appropriate when you want to preserve them but don’t want them to be part of the merge process. This is often the best course of action if you are still working on these files.
How to Move Files
You can move untracked files using the `git mv` command. Here is the basic syntax:
git mv <source> <destination>
For example, if you want to move an untracked file named `untracked_file.txt` to a new directory called `new_directory`, the command would look like this:
git mv untracked_file.txt new_directory/
This allows you to relocate your files without losing any work.
Option 2: Removing Untracked Files
When to Remove Files
Removing untracked files is appropriate when you no longer need them and are confident they are not part of the ongoing work.
How to Remove Files
To remove untracked files, you can use the `git rm` command as follows:
git rm <file>
Warning: Be cautious when using this command, as it permanently deletes the file from your storage.
Using `git clean` to Remove Untracked Files
Another approach to remove untracked files is using Git’s cleaning command:
git clean -f
This command removes all untracked files from your working directory. If you want to also remove untracked directories, you can use the `-d` option:
git clean -fd
It is advisable to preview the changes before executing this command by using the `-n` flag for a dry-run:
git clean -n
This will show you which files would be removed without actually deleting anything.
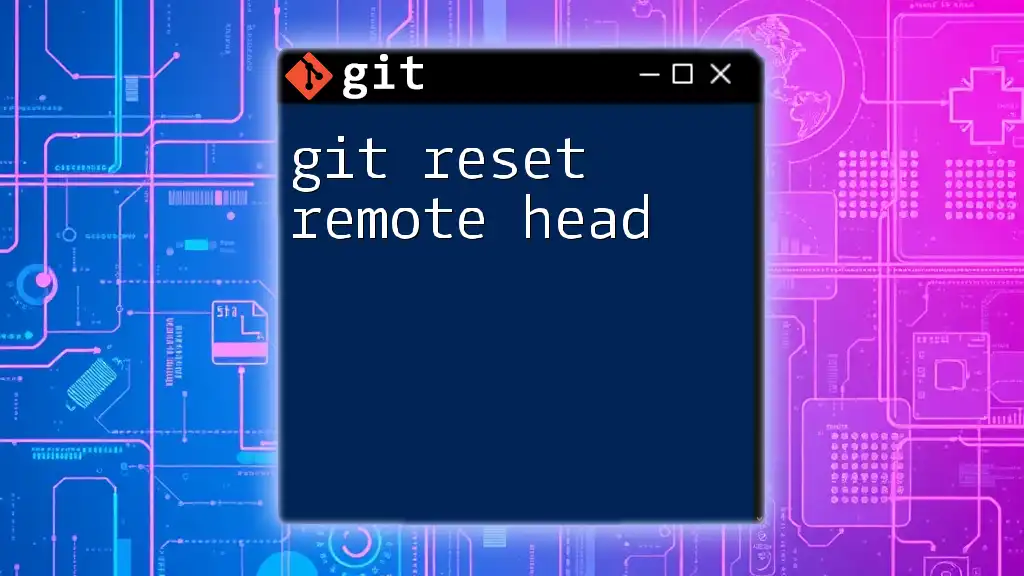
Best Practices to Avoid the Merge Error
Maintain a Clean Working Directory
To avoid running into the merge error in the future, regularly check the status of your Git repository with `git status` before performing merges. Keeping a clean working directory can save you from a lot of headaches.
Using `.gitignore` Effectively
Implementing a `.gitignore` file can significantly help prevent untracked files from conflicting during merges. By specifying patterns of files that should be ignored, you can ensure that unnecessary files do not clutter your Git operations. A simple example of a `.gitignore` file could look like this:
# Ignore all log files
*.log
# Ignore all temp files
temp/
By properly configuring this file, you can sidestep many merge-related issues.
Regularly Commit Changes
Frequent commits can not only help to minimize potential conflicts but also maintain a clear history of your changes. When you commit often, you can isolate changes and make merging much smoother.
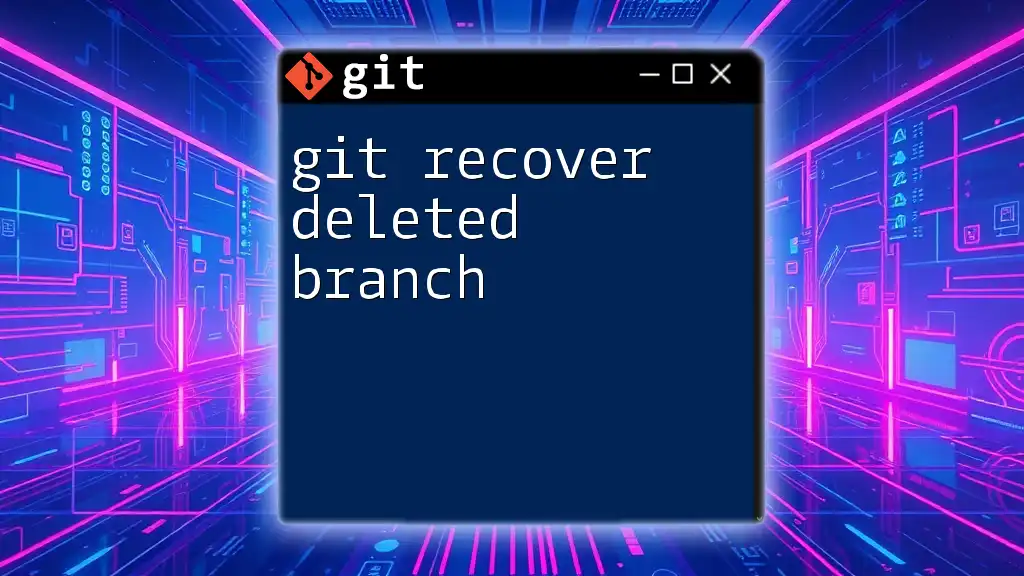
Conclusion
In summary, understanding and effectively managing the merge process in Git is crucial for developers. Resolving the "please move or remove them before you can merge" error ensures a clean workflow, preserves your work, and maintains a coherent history. By mastering the commands and techniques outlined in this article, you can improve your Git proficiency and avoid common pitfalls associated with merging. Remember, practice makes perfect. Start integrating these practices into your workflow to become more adept at handling Git merges.