A "git playground" is an interactive environment that allows users to experiment with Git commands safely without affecting their actual projects.
Here’s an example of initializing a new Git repository in a playground:
git init my-playground
What is a Git Playground?
Definition of Git Playground
A Git Playground is a controlled environment that allows users to experiment with Git commands without the fear of disrupting important code or data. It serves the essential purpose of helping both beginners and advanced users practice Git concepts in an exploratory manner, providing a safe space to learn and make mistakes.
Purpose of Using a Git Playground
The Git Playground’s primary purpose is to facilitate an accelerated learning process. In this environment, users can experiment with various Git features and commands without repercussions or the anxiety of affecting a live project. It empowers users to build their confidence by providing them with the freedom to explore different workflows and scenarios.
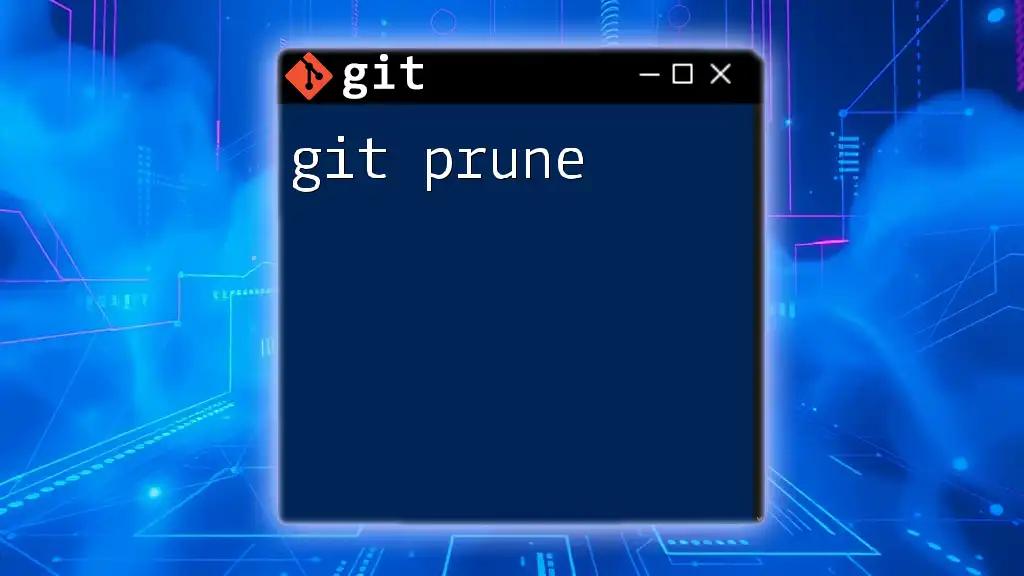
Setting Up Your Git Playground
Prerequisites
Before diving into a Git Playground, it’s crucial to have a basic understanding of Version Control Systems (VCS). This foundational knowledge will help you grasp the concepts and commands more effectively. Additionally, ensure that you have Git installed on your local machine, as you will need it to create and manage your Git Playground.
Creating Your Git Playground
Setting up your Git Playground involves a straightforward process. Here’s how to create a new Git repository:
- Open your terminal or command line interface.
- Create a directory for your Git Playground:
mkdir git-playground cd git-playground
- Initialize a new Git repository:
git init
Congratulations! You now have a basic Git Playground to work within.
Recommended Tools and Platforms
Choosing the right environment for your Git Playground can greatly enhance your learning experience. You can opt for local tools such as command line interfaces or integrated development environments (IDEs) like Visual Studio Code. Alternatively, consider using cloud-based platforms such as GitHub, GitLab, or Bitbucket, which provide additional features like collaborative workspaces and visual representation of repositories.
Choosing the Right Environment
When determining whether to work locally or online, consider the following pros and cons:
- Local Playgrounds:
- Pros: Immediate feedback, complete control, no internet dependency.
- Cons: Limited collaboration capabilities.
- Cloud Platforms:
- Pros: Easy sharing and collaboration, integrated issue tracking, real-time collaboration.
- Cons: Requires internet access and may have learning curves associated with the interface.
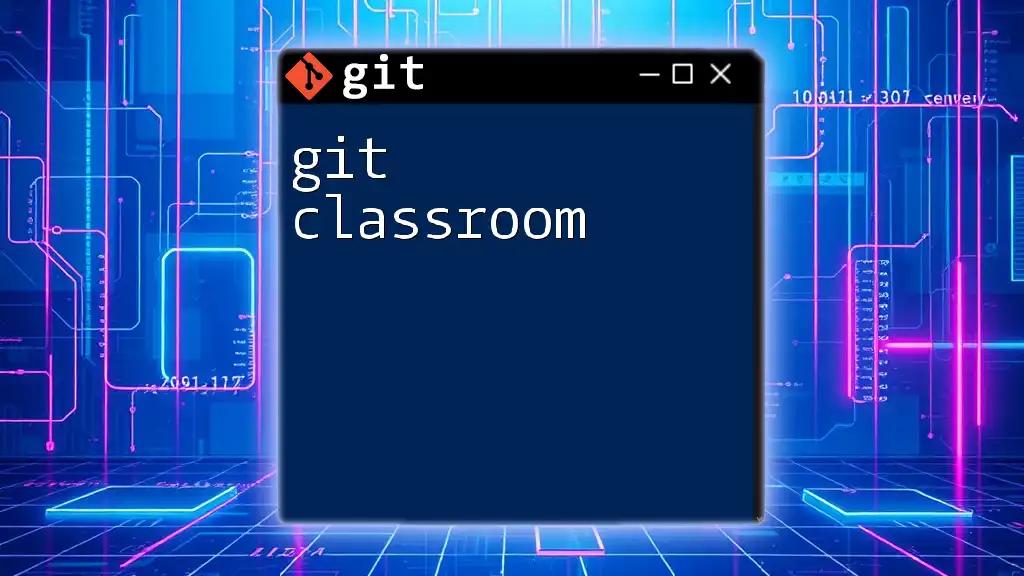
Navigating Your Git Playground
Git Basic Commands
Familiarizing yourself with essential Git commands is critical to utilizing your Git Playground effectively. Here are some of the commands you’ll want to master:
-
Check the status of your repository:
git status
-
Add files to the staging area:
git add <file>
-
Commit your changes:
git commit -m "Your commit message"
These basic commands lay the groundwork for more advanced operations within your Git Playground.
Understanding Branches
Branches are fundamental to using Git efficiently. They allow users to create separate lines of development, making it easy to work on new features without disturbing the main codebase.
To create and switch to a new branch, use the following commands:
git branch new-feature
git checkout new-feature
This approach enables you to isolate changes, test new ideas, and ultimately merge them back into the main branch confidently.
Experimenting with Merging
Once you’ve created different branches, merging them becomes essential. Merging integrates changes from one branch into another. To merge a feature branch back into the main branch, execute:
git checkout main
git merge new-feature
This command effectively combines your changes, ensuring new features and fixes become part of the main codebase.
Handling Conflicts
Conflicts may arise during merging when two branches make changes to the same part of a file. Understanding how to resolve these conflicts is crucial. When a conflict occurs, Git will highlight the problematic areas in the affected files.
To resolve a conflict, you will need to:
- Manually edit the file to determine which changes to keep.
- After resolving the conflict, stage the file:
git add <file>
- Finally, commit your merged changes:
git commit -m "Resolved merge conflict"
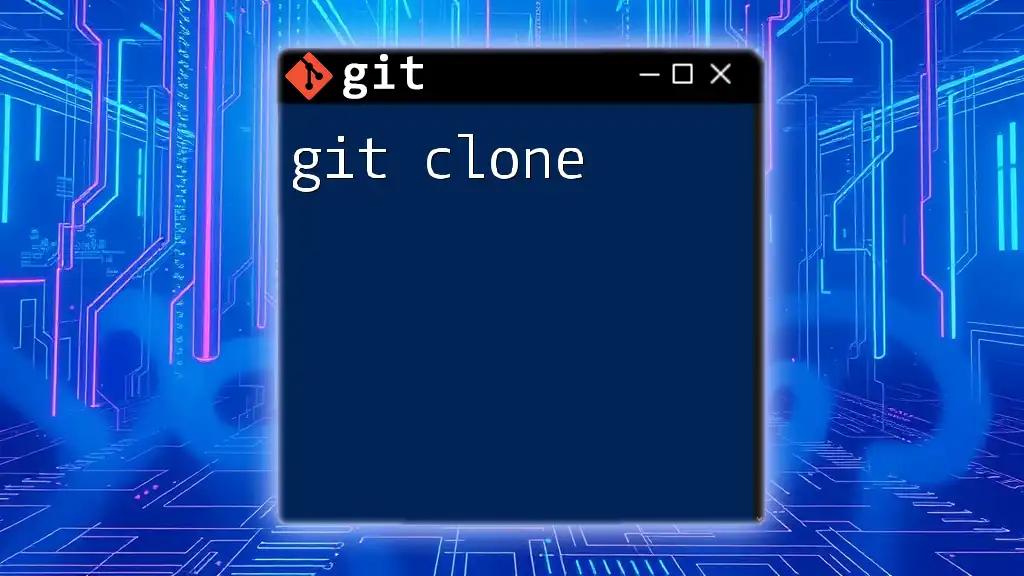
Advanced Techniques in Your Git Playground
Using Tags
Tags are markers that allow you to mark specific points in the Git history, typically used for releases. They provide a way to tag important commits. You can create a tag with a message using the following command:
git tag -a v1.0 -m "Initial release"
git push origin v1.0
This process helps you create a clear timeline of releases, enhancing the management of your project.
Cherry Picking Commits
Cherry picking allows you to apply a specific commit from one branch to another without merging entire branches. It’s particularly useful when you only need to bring over specific changes. To cherry-pick a commit, use:
git cherry-pick <commit-hash>
This command will create a new commit in your current branch based on the changes from the selected commit.
Rebasing
Rebasing is a powerful technique that allows you to move or combine a sequence of commits to a new base commit. It’s an alternative to merging that can provide a cleaner project history. To perform a rebase, use the following command:
git rebase main
Rebasing incorporates changes from another branch and is particularly useful when you want to maintain a linear project history.
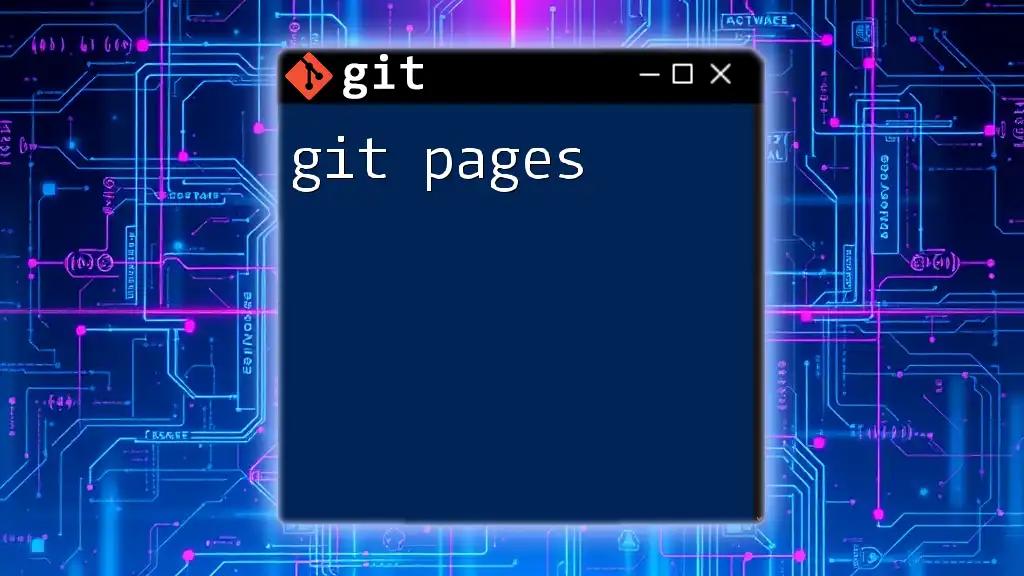
Best Practices in Using Git Playground
Keeping Your Repository Clean
Maintaining a clean repository is essential for collaboration and historical accuracy. Always aim for clear, descriptive commit messages that encapsulate the changes made. Organizing your branches effectively is another way to keep your Git Playground tidy.
Regularly Syncing with Remote Repositories
Keeping your local playground in sync with remote repositories is critical for effective collaboration. Use the commands `git fetch` and `git pull` to regularly update your local copy with changes made by others. This action helps prevent unnecessary conflicts and keeps your environment current.
Utilizing .gitignore
A .gitignore file helps you manage which files should not be tracked by Git. It’s crucial for excluding temporary files, logs, or any sensitive information from your repository. Creating a .gitignore file is straightforward:
# .gitignore example
*.log
node_modules/
By managing what goes into your repository, you ensure a cleaner, more focused project.
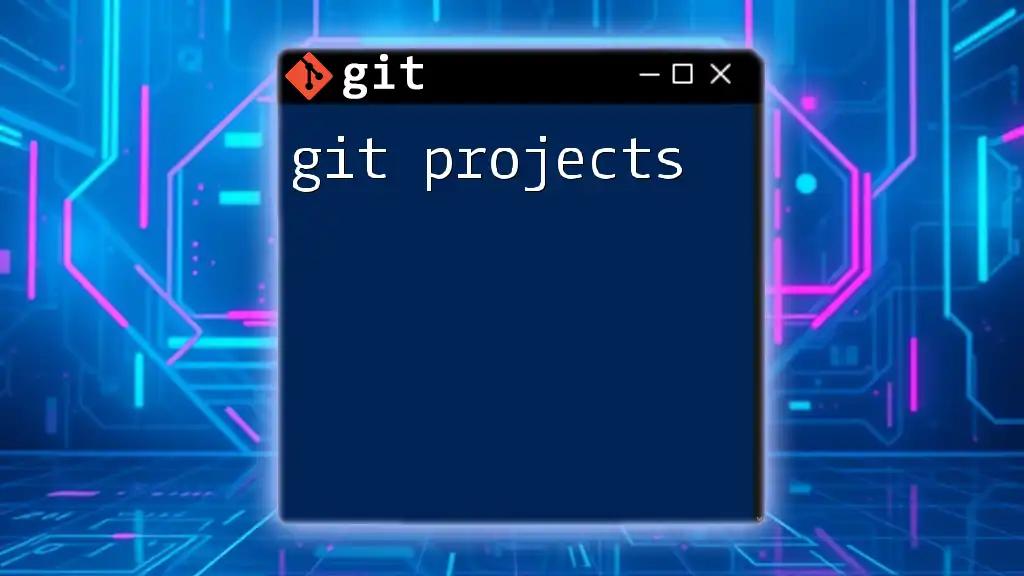
Learning Resources and Continuing Education
Recommended Books and Online Courses
To further your Git knowledge, consider diving into reputable books and online courses. Resources such as "Pro Git" by Scott Chacon or platforms like Coursera and Udacity offer valuable insights into Git and version control best practices.
Joining Git Communities
Engaging with the Git community can significantly enhance your learning experience. Participate in forums, attend local meetups, or join social media groups focused on Git. These platforms provide opportunities for collaboration, advice, and support from other users.
Practice Challenges
To solidify your Git skills, consider completing practical challenges in your Git Playground. For example:
- Create a new feature branch and later merge it.
- Simulate a merge conflict and practice resolving it.
- Tag a commit and push it to a remote repository.
By practicing these scenarios, you will enhance your understanding of Git operations and build confidence in your abilities.
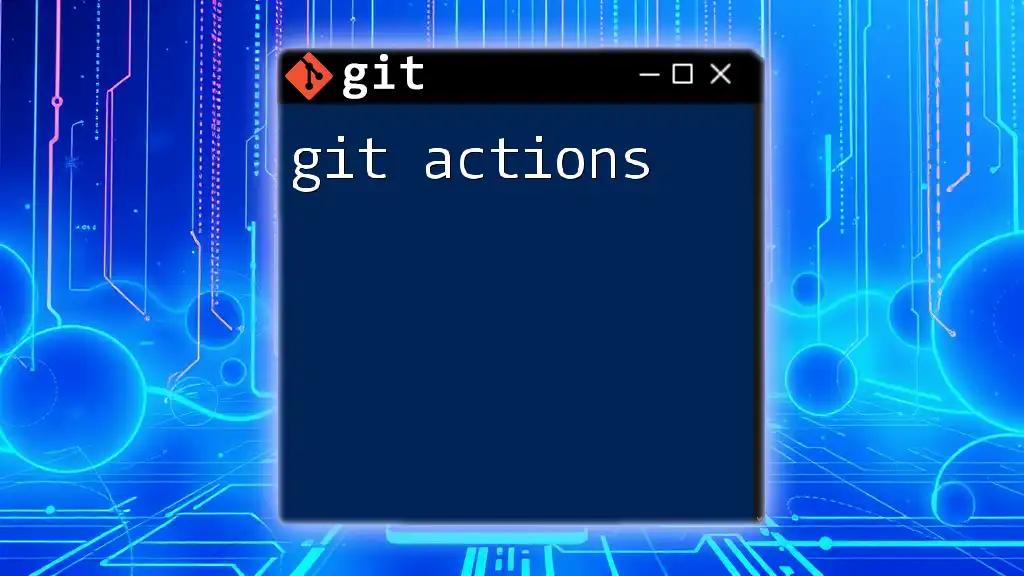
Conclusion
In conclusion, utilizing a Git Playground accelerates your learning and mastery of Git commands. By setting up a dedicated environment, practicing with various commands, and incorporating advanced features, you will gain the knowledge and experience necessary to manage projects effectively. Embrace the freedom to experiment and make mistakes in your Git Playground, and remember that every failure is a step toward mastery in version control.

Further Reading
For those interested in expanding their Git knowledge, consult the following resources:
- Git documentation on [git-scm.com](https://git-scm.com/doc).
- Online tutorials from reputable coding platforms.
- Interactive Git courses on sites like Codecademy or Udacity.
By exploring these resources, you can continue to grow and deepen your understanding of version control and Git.