A Git roadmap outlines the essential commands and concepts needed to effectively manage version control in software development and collaborative projects.
Here’s a quick example of basic Git commands to get you started:
# Initialize a new Git repository
git init
# Clone an existing repository
git clone <repository-url>
# Check the status of your Git repository
git status
# Add changes to the staging area
git add .
# Commit changes to the repository
git commit -m "Your commit message here"
# Push changes to a remote repository
git push origin main
# Pull changes from a remote repository
git pull origin main
Getting Started with Git
Setting Up Git
Installing Git
Before you can start using Git, you need to install it on your system. Follow these step-by-step instructions:
-
Windows: Download the Git installer from the official Git website. Run the installer and follow the prompts, making sure to choose the default options unless you have specific needs.
-
macOS: The easiest way to install Git is via Homebrew. Open your terminal and run:
brew install git
-
Linux: Most distributions come with Git in their package manager. For example, on Ubuntu, you can run:
sudo apt-get install git
Configuring Git
Once Git is installed, you’ll want to configure it by setting your name and email. This information is associated with your commits, so it’s essential for collaboration:
git config --global user.name "Your Name"
git config --global user.email "you@example.com"
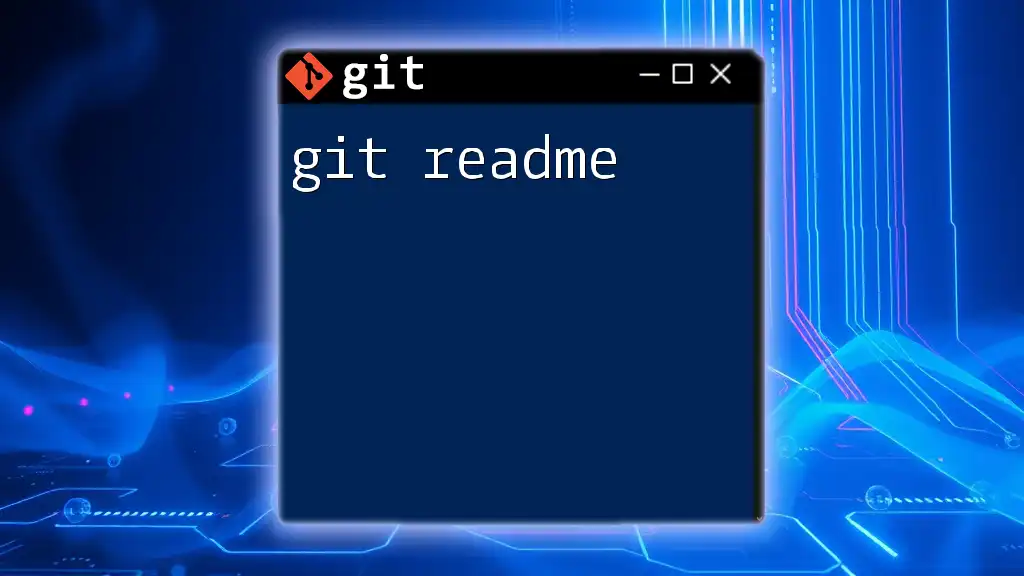
Basic Git Concepts
Repositories
Definition and Types
A Git repository (repo) is a directory where your project files and the version control history are stored. There are two types of repositories: local and remote. Local repositories exist on your computer, while remote repositories are hosted on services like GitHub or GitLab.
Creating a New Repository
To start a new project, you can create a repository using the following command:
git init my-repo
This command generates a new directory called `my-repo`, where you can begin adding files.
Commits
Understanding Snapshots in Git
Commits are snapshots of your project's changes at a given point in time. Each commit records the history of changes made in the repository.
Making Your First Commit
After creating your repository, you can add files and make your first commit. Start by staging all your files:
git add .
Next, commit your changes with a descriptive message:
git commit -m "Initial commit"
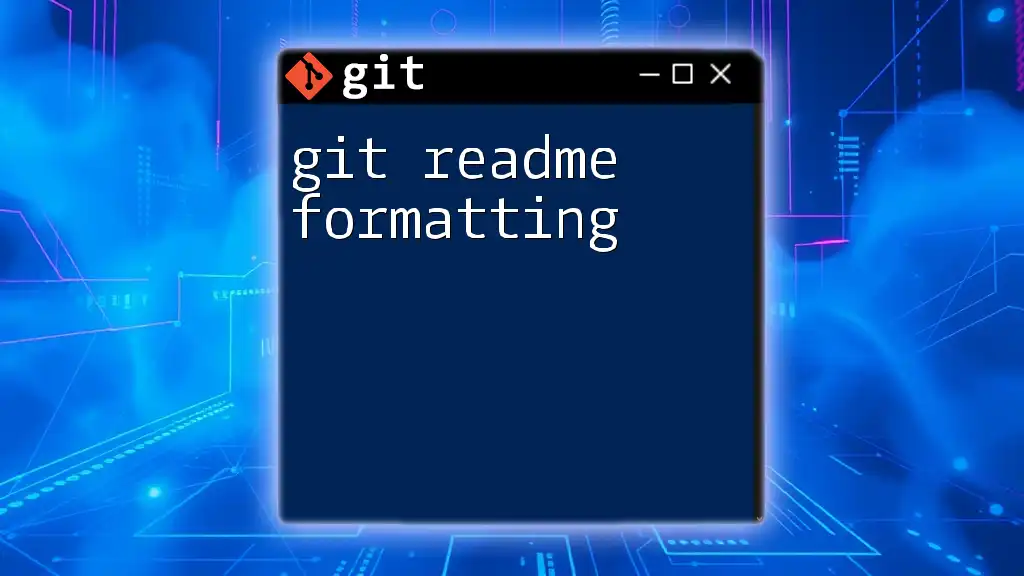
Core Git Commands
Working with Git
Staging Changes
The staging area allows you to prepare changes before committing them. Use the following commands to manage your staging:
-
To stage a specific file:
git add <file>
-
To stage all changes in the current directory:
git add .
Committing Changes
Be mindful of your commit messages; they should clearly describe the changes made. Aim for small, atomic commits that each serve a single purpose, making it easier to understand project history.
Branching and Merging
Understanding Branches
Branches allow you to work on new features or fixes without altering the main codebase. This is essential for separating different lines of development.
Creating and Switching Branches
You can create a new branch and switch to it using:
git branch new-feature
git checkout new-feature
Now you are on the `new-feature` branch, where you can safely make changes without affecting other branches.
Merging Branches
Once you've completed your work on a feature branch, you can merge it back into the main branch. First, switch back to the main branch:
git checkout main
Then, merge in the changes:
git merge new-feature
If there are any conflicts, Git will let you know, and you'll need to resolve them before completing the merge.
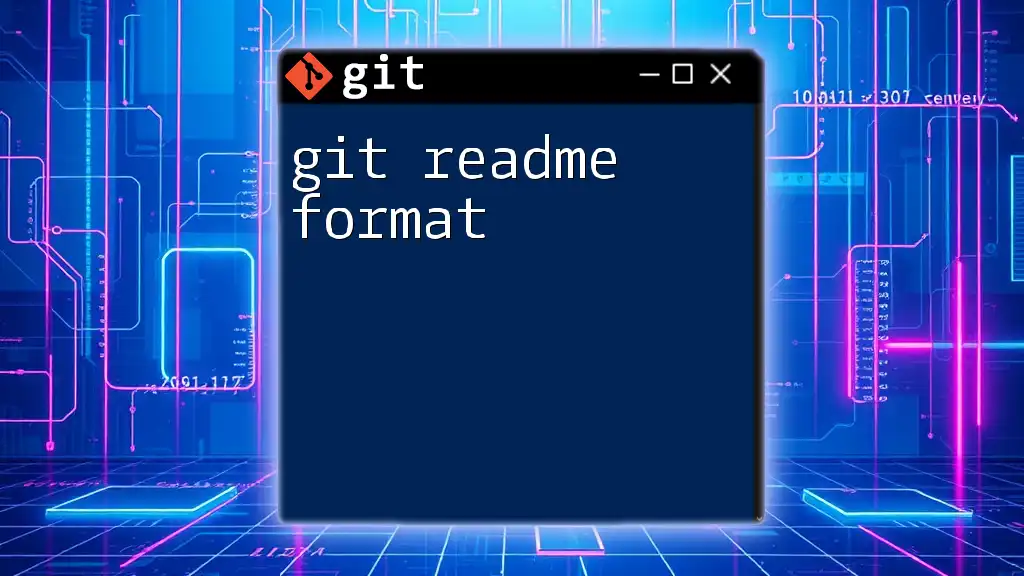
Advanced Git Concepts
Working with Remote Repositories
Adding a Remote Repository
Remote repositories allow you to collaborate with others. To add a remote repository, use:
git remote add origin https://github.com/user/repo.git
This connects your local repository to a remote named "origin".
Pushing and Pulling Changes
To share your local changes with others, push them to the remote repository:
git push origin main
Similarly, when you want to incorporate changes made by others, you can pull the latest updates:
git pull origin main
Resolving Conflicts
What are Merge Conflicts?
Merge conflicts arise when changes from different branches affect the same part of a file. This can happen during a merge or pull process.
Resolving Conflicts
If you encounter a conflict, Git will indicate which files need attention. You'll need to open these files, manually resolve the conflicts, and then add and commit the resolved files:
git add <resolved-file>
git commit -m "Resolved merge conflict"
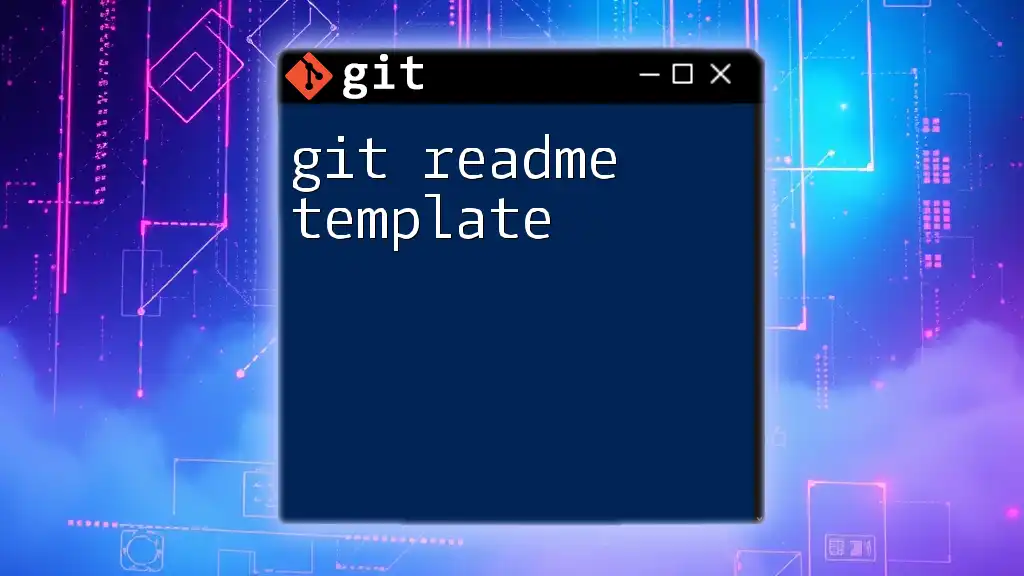
Best Practices in Git
Effective Commit Strategies
Writing Meaningful Commit Messages
Good commit messages provide context and facilitate project maintenance. Follow this simple structure: Use the imperative mood, explain what is being changed, and keep your message concise yet descriptive.
Using Branches Effectively
When naming branches, be clear and consistent. Descriptive names help teammates understand the purpose of the branch at a glance, e.g., `feature/login-page` or `bugfix/cart-issue`.
Collaborating on Git
Pull Requests and Code Reviews
Pull requests are a way to propose changes to a project. They enable discussion about the changes before they are merged into the main codebase. Always review pull requests to ensure code quality and maintainability.
Collaborative Workflows
Explore popular workflows like the Feature Branch Workflow, where each feature is developed in a dedicated branch, or Git Flow, which involves multiple branches for development and releases. Choosing a workflow that suits your team’s needs can enhance productivity.
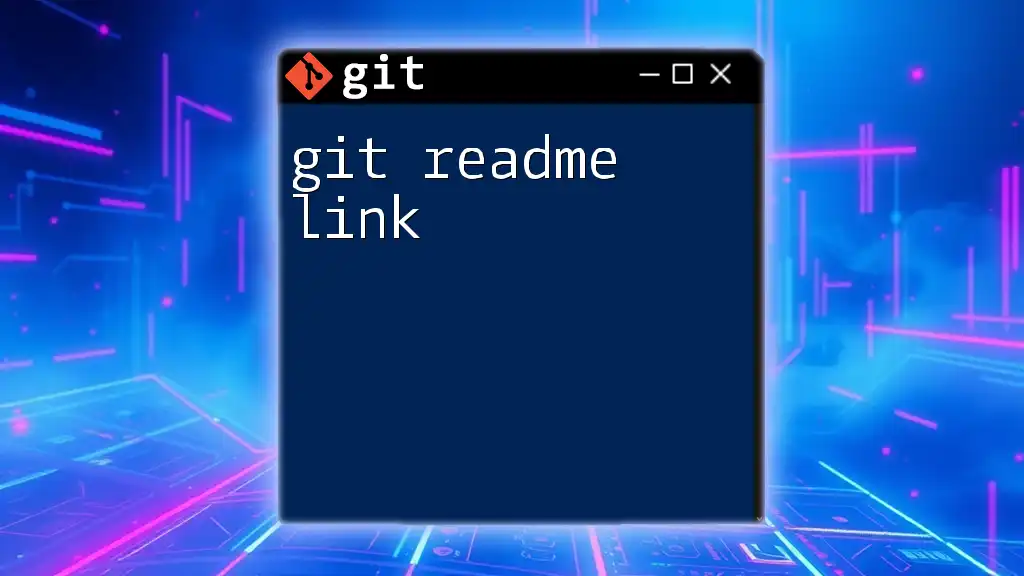
Tooling and Resources
Recommended Git GUIs
Git Desktop Clients
For those who prefer graphical interfaces, several Git GUIs are available. SourceTree, GitKraken, and GitHub Desktop are popular choices that can simplify the Git experience, offering intuitive interfaces for common tasks.
Learning and Reference Materials
Online Resources
To further your understanding of Git, explore resources like the official Git documentation, online courses on platforms like Coursera or Udacity, and books like Pro Git. Continuous learning is vital for mastering Git.
Troubleshooting Common Git Issues
Common Errors and Solutions
As you use Git, you may encounter errors such as detached HEAD or failed merges. Understanding these issues and how to address them will enhance your Git skills. For example, to get back to the main branch, you can use:
git checkout main
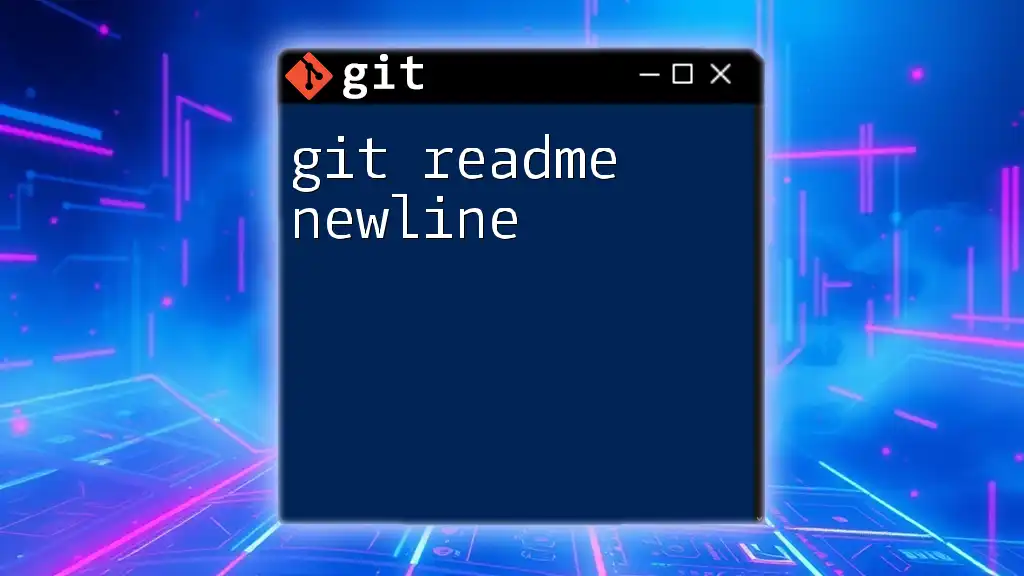
Conclusion
In summary, following a git roadmap equips you with the knowledge and skills to effectively manage your code, collaborate with others, and handle complex project workflows. Continuous practice and exploration will solidify your proficiency in Git, making it an invaluable tool in your development toolkit.
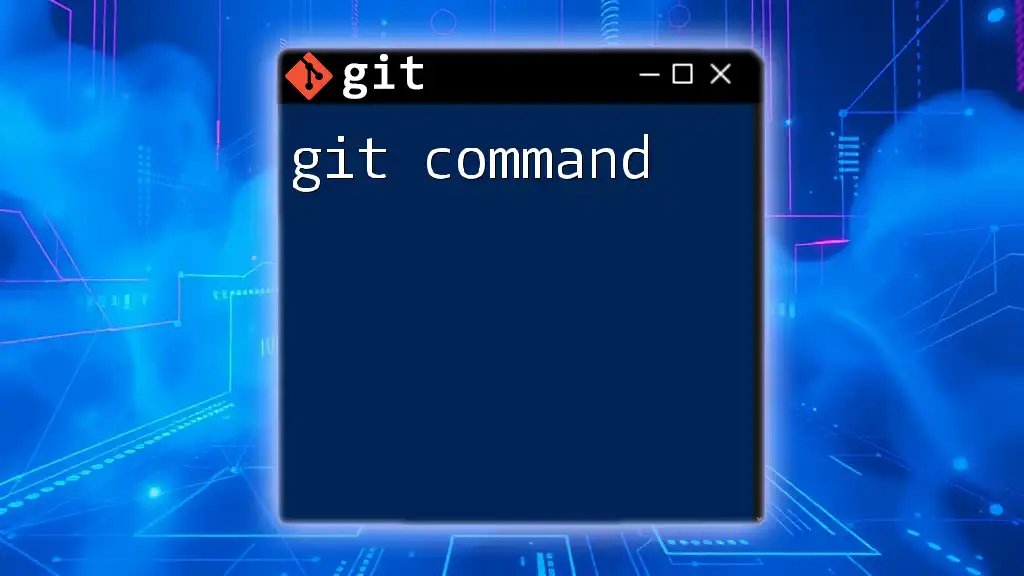
Call to Action
Join our community to engage with others on this learning journey, share your experiences, and continue growing your Git skills. Whether you're just starting or seeking advanced techniques, there's always more to discover in the world of version control!