A Git heat map visually represents the frequency of commits over time, helping you identify patterns of activity in your repository.
Here's how you can generate a simple heat map using Git commands:
git config --global alias.heatmap '!git log --pretty=format: --date=format:"%Y-%m-%d" --no-merges | awk "{print \$1}" | uniq -c | sort -n'
What is a Git Heat Map?
A Git heat map is a visual representation of contributions made to a Git repository over a specified timeframe. It provides insights into the frequency and volume of commits, allowing developers and project managers to quickly identify patterns of activity within a project. By employing a color-coded system, heat maps enable viewers to grasp information at a glance, showcasing active periods and times of stagnation.
Unlike other visualization tools, such as commit graphs or individual contributor statistics, heat maps aggregate a substantial amount of information into a compact format. This feature is essential for teams who want a concise view of overall activity and contributions without sifting through countless logs.
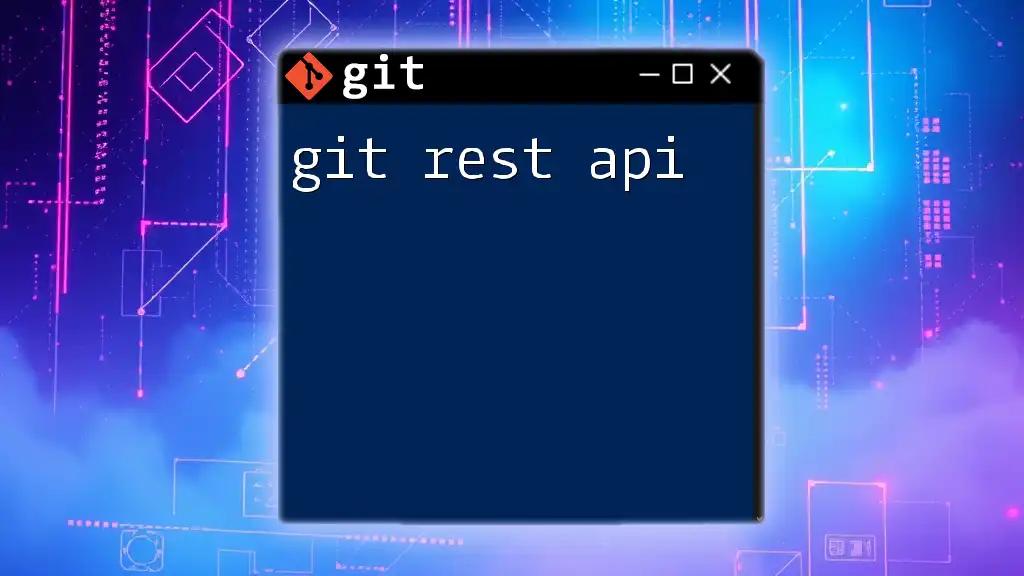
Understanding the Components of a Git Heat Map
Commits
Commits are the cornerstone of Git's version control system. They document changes made to the codebase over time, acting as snapshots that capture the state of a project at a particular point. The significance of commits cannot be overstated, as they provide a history that is essential for collaboration among team members.
To view the commit history in a concise format, you can use the following command:
git log --oneline
This command will give you a straightforward list of recent commits, showing their respective hashes and messages. The number of commits within a specified timeframe directly influences the richness of the heat map; more commits typically indicate a more vibrant and engaged development process.
Contributors
Contributors are key players in any project, directly impacting the health and dynamism of the codebase. A Git repository can have multiple contributors, each with varying degrees of involvement. Understanding who is contributing and how frequently allows teams to appreciate contributions and assess areas for improvement.
To view a list of contributors along with their total contributions, you can execute:
git shortlog -sn
This command generates a sorted list of contributors and their contributions. In a Git heat map, the activity of contributors can be visually represented, showing who has been the most active during specific intervals and clarifying team dynamics.
Timeframe
Establishing a timeframe for your analysis is critical. It allows you to zero in on specific periods of activity, whether for a sprint, a release cycle, or a project's lifespan. With clearly defined timeframes, you can interpret changes in contribution patterns and provide feedback based on observable data.
To filter commits by date, you can use the following command:
git log --since="1 month ago"
This command narrows the results to show only commits made within the last month, which can be useful for generating a heat map for that specific period.
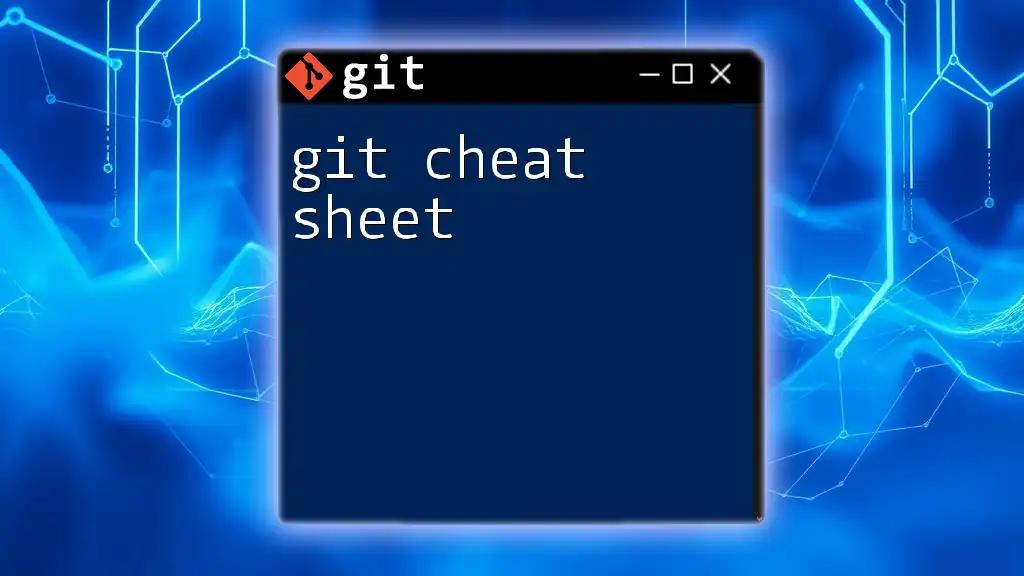
Generating a Git Heat Map
Using GitHub
GitHub offers a native feature that allows users to access a heat map of contributions effortlessly. To find this feature, follow these steps:
- Navigate to the GitHub repository you are interested in.
- Click on the “Insights” tab, located at the top of the repository page.
- From the options available, select the “Contributors” graph.
This interface will provide a graphical representation of contributions over time using colored blocks, with colors indicating the volume of commits made.
Third-party Tools
If you are looking for more advanced visualizations, several third-party tools can help generate content-rich Git heat maps.
-
GitStats: A powerful and versatile tool that can generate various statistics about your repository, including heat maps. To generate a heat map, you can run:
gitstats /path/to/repo /path/to/output
-
GitHub Weekly: This tool focuses on weekly contributions and provides easy-to-use interfaces for tracking development activity. Users can view summaries of activity over weeks, helping them to stay informed about project progress.
Custom Scripts for Generating Heat Maps
For those inclined to customize their visualizations, creating a Git heat map using scripting presents an excellent opportunity. Below is an example of a basic Python script that utilizes `matplotlib` and `seaborn` for generating a heat map:
import matplotlib.pyplot as plt
import seaborn as sns
import pandas as pd
# Code logic to process git commit data and prepare for heatmap generation will go here.
# Sample data representation using pandas
data = { 'date': [...], 'commits': [...] }
df = pd.DataFrame(data)
# Generate heatmap
pivot_df = df.pivot("date", "commits") # Modify as needed for actual data
sns.heatmap(pivot_df, cmap="YlGnBu")
plt.title("Git Heat Map of Commits")
plt.show()
This script serves as a foundational template. You can enhance it by incorporating commit information from your repository and configuring the visual output to match your desired aesthetic.
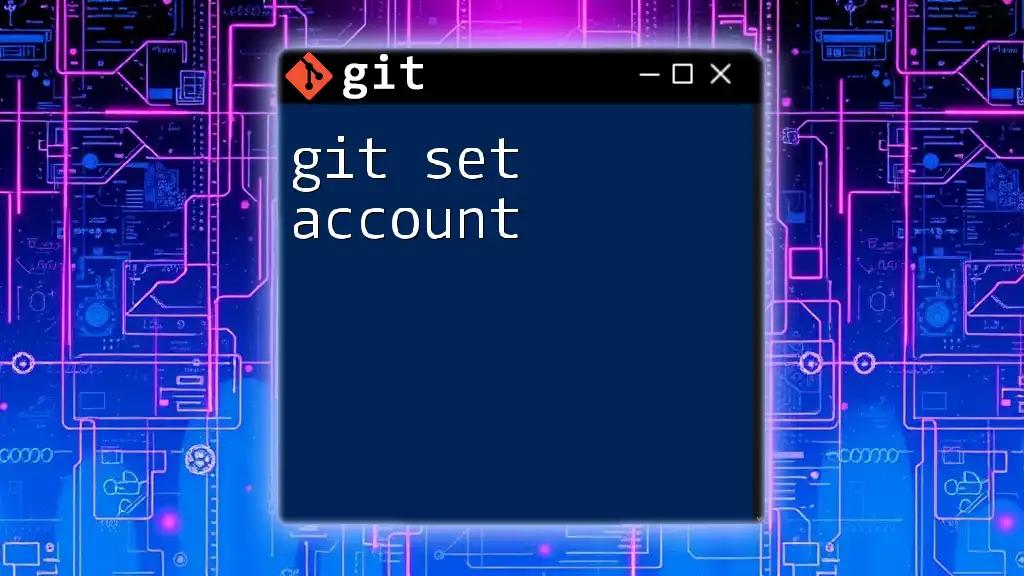
Interpreting Git Heat Maps
Identifying Patterns and Trends
Reading a Git heat map visually can provide profound insights at a glance. The color gradients typically represent the volume of commits—darker or more intense colors often indicate higher activity. By analyzing these patterns, teams can accurately identify periods of high engagement or witness the drop-off in activity, which may prompt discussions about workflow challenges or motivations.
Analyzing Contributor Performance
A Git heat map can also serve as a performance analysis tool for contributors. By scrutinizing who is contributing and when, leaders can make data-driven decisions regarding resource allocation, mentorship, or restructuring teams. If particular contributors display consistent output, it may be worth acknowledging their contributions and exploring how their approach can benefit the entire team.
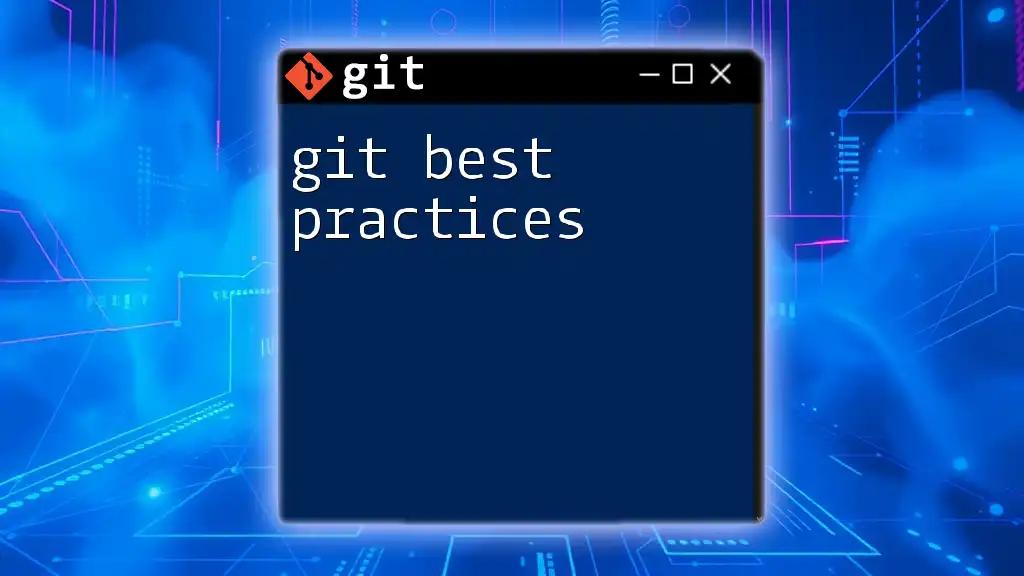
Best Practices for Maintaining a Healthy Git Repository
To ensure that your Git repository remains efficient and effective, consider implementing the following best practices:
-
Maintain meaningful commit messages: Each commit should have a clear and descriptive message, effectively communicating the purpose of the changes.
-
Commit regularly: Frequent commits make it easier to track changes, enabling a more accurate representation of activity in your heat map.
-
Foster team collaboration: Encourage practices that promote knowledge sharing and collective problem-solving, leading to a healthier codebase and enriched team dynamics.
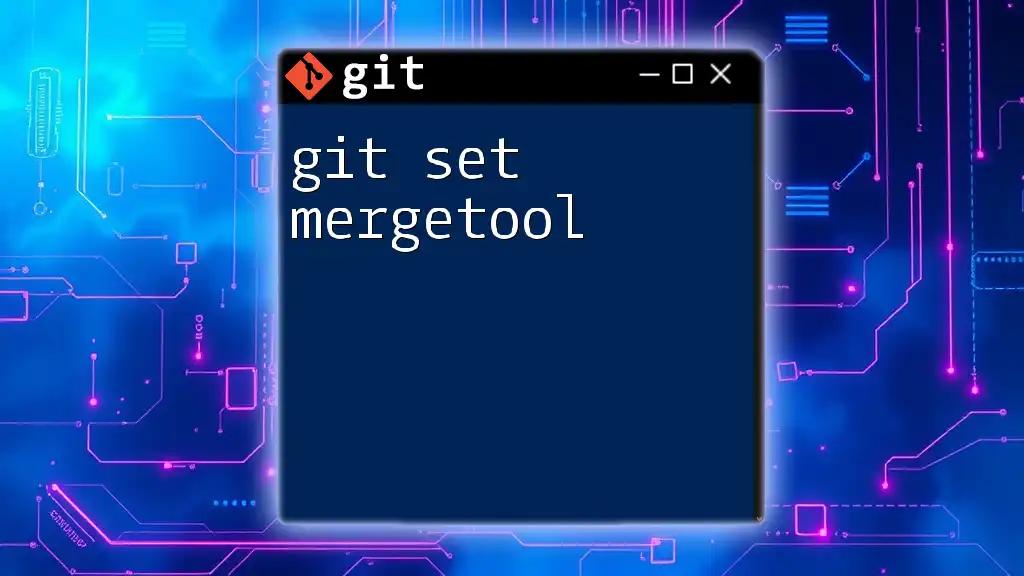
Conclusion
Git heat maps are invaluable tools that provide a clear visual representation of contributions over time. By leveraging this data, teams can make informed decisions about their workflows, Contributor performance, and project planning. Utilizing known practices can amplify these benefits, leading to a more productive team environment. As you explore Git and its powerful features, integrating a heat map into your analysis toolset will undoubtedly enrich your understanding and management of developments within your projects.
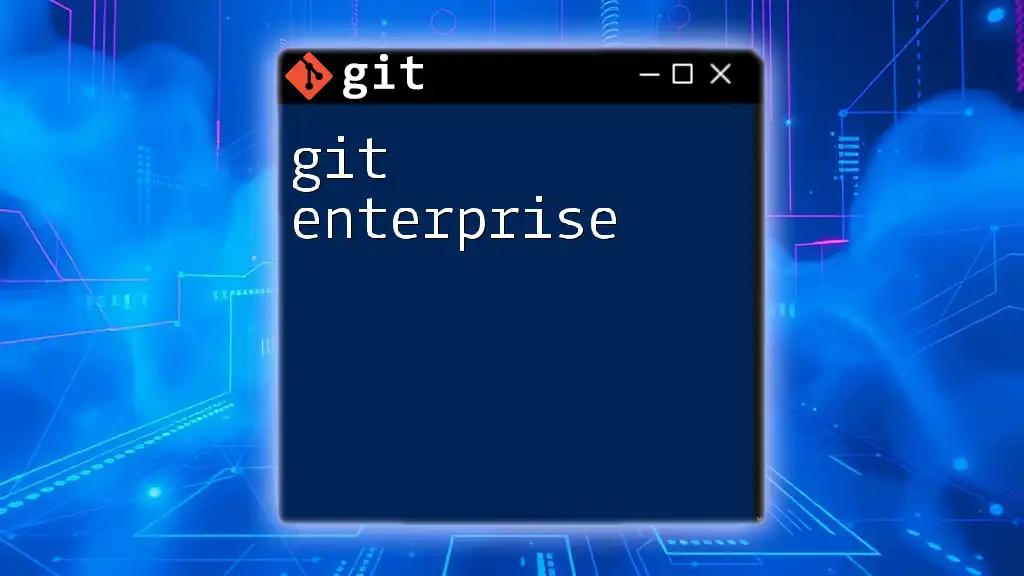
Additional Resources
To further enhance your knowledge of Git and Git heat maps, consider browsing through official Git and GitHub documentation, complementing readings, and tutorials that delve deeper into version control practices. By continually educating yourself and your team, you’ll unlock the full potential that Git offers for collaborative development.