In Git, `HEAD` refers to the current commit your repository is "pointing" to, often representing the latest commit in your checked-out branch.
Here’s how you can view the current `HEAD` commit:
git rev-parse HEAD
What is Git HEAD?
In the Git context, HEAD refers to a special pointer that indicates the current working location in your project. It essentially represents the current branch and commit that you are working on. Understanding how HEAD operates is crucial for effective version control because it affects your commits, branches, and merges.
The HEAD Pointer
HEAD plays a vital role as a reference point within your repository. It tells Git which commit you are currently on, enabling you to track your positioning within the project's history. When you make a new commit, HEAD automatically moves to this new commit, affirming its role as a dynamic pointer.
Detached HEAD State
A detached HEAD means that you are not on a branch. Instead, HEAD is pointing directly to a specific commit. This state typically occurs in scenarios like checking out a specific commit or tagging a version of your codebase. For example, if you want to review a previous version of your project, you might run:
git checkout <commit-hash>
This command will put you in a detached HEAD state, isolating you from any branches. Understanding when you are in a detached HEAD state is important, as changes made here won’t belong to any branch unless you create one from this state.
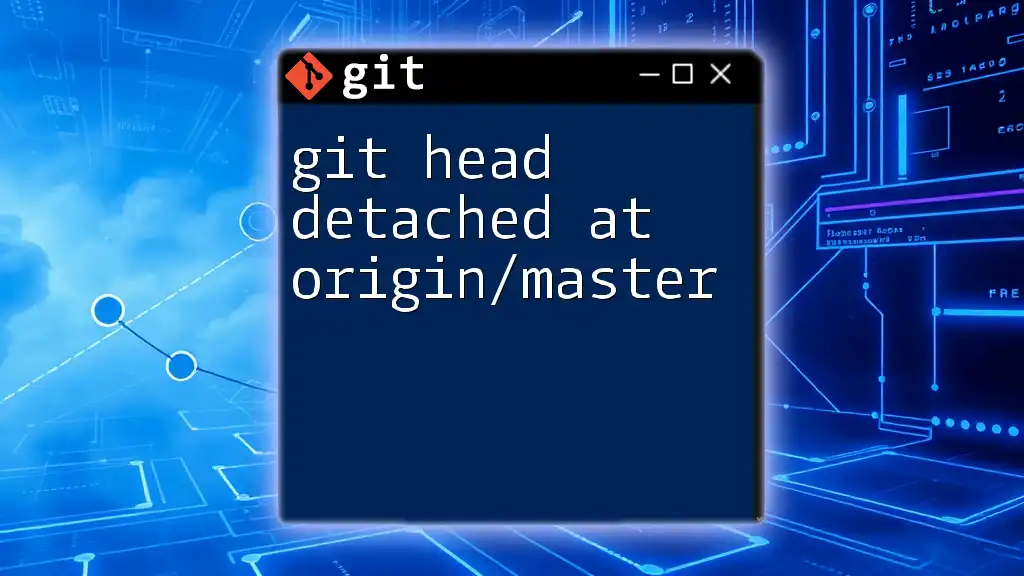
Navigating with HEAD in Git
Moving the HEAD Pointer
To move the HEAD pointer between branches, you can use the `git checkout` command. This command updates HEAD to point to the latest commit on the branch you are switching to.
For instance, if you want to switch to a branch named `feature-branch`, you simply run:
git checkout feature-branch
Upon executing this command, HEAD now points to the latest commit on `feature-branch`, while your working directory is updated to reflect the state of files in this branch.
Using HEAD in Tracking Changes
You can check the status of HEAD with the `git status` command. This command informs you of your current branch and whether your working directory is clean or contains changes that are staged or unstaged.
To view the latest commit that HEAD points to, use:
git log -1
This command displays the most recent commit and serves a dual purpose by letting you know your application's current state.
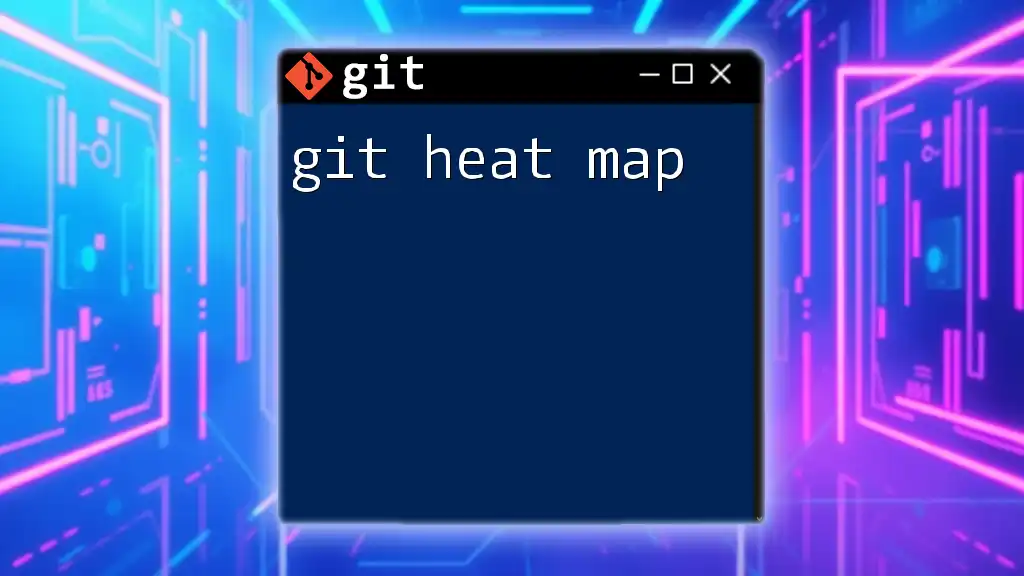
Using HEAD in Common Git Commands
Reverting Changes with HEAD
At times, you need to revert changes that have been staged. The command `git reset HEAD` allows you to unstage files by resetting the index to the last commit that HEAD pointed to. Here are the modes you can use with this command:
- Soft: Keeps changes staged.
- Mixed (default): Removes changes from staging while keeping them in your working directory.
- Hard: Deletes the changes completely.
For example, if you want to undo the last commit entirely, you would run:
git reset --soft HEAD~1
This command resets the staging area to the previous commit while leaving the working directory intact.
Undoing Changes with HEAD
If substantial changes have been made to one or more files, and you want to revert a specific file back to the version in the most recent commit, leverage the `git checkout` command. The syntax is as follows:
git checkout HEAD -- <file-name>
This command restores the specified file to its last committed state while leaving your other changes intact.
Committing with HEAD
When you are satisfied with your changes and want to commit them, you can do so by using the `git commit` command. This will record all staged changes along with a descriptive message:
git commit -m "Your commit message"
Once you execute this command, HEAD moves to point to the new commit, adding it to the repository history.
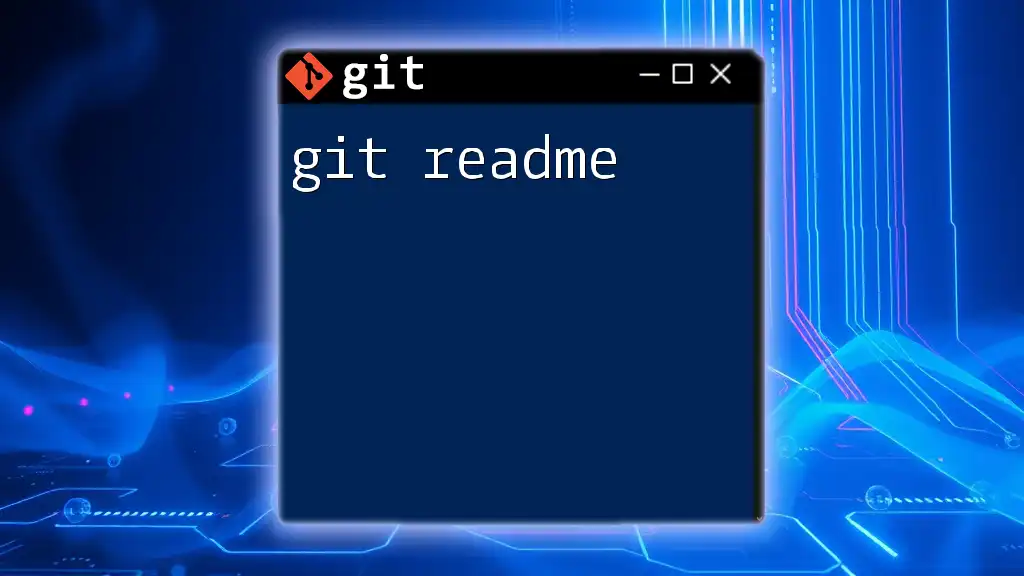
Advanced HEAD Manipulations
Moving HEAD with `git reflog`
Git reflog tracks where your HEAD has been. This tool is particularly useful when you need to retrieve lost branches or commits. You can view the reflog with:
git reflog
This command will display a list of where HEAD has pointed previously, allowing you to locate a lost commit or branch. To recover a lost state, you would check out the relevant commit hash.
Interactive Rebasing with HEAD
Interactive rebasing allows you to edit, delete, or reorder commits leading up to HEAD for a cleaner commit history. To initiate an interactive rebase of the last three commits, execute:
git rebase -i HEAD~3
This command opens an editor where you can choose actions (like editing or squashing commits) to personalize your commit history.
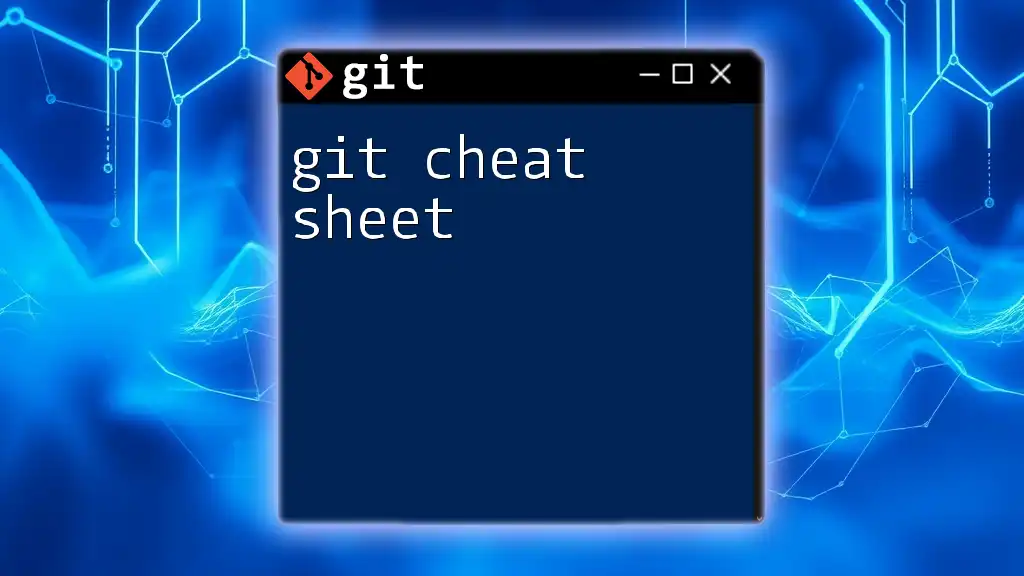
Best Practices for Using HEAD
To safely manipulate HEAD, keep these tips in mind:
- Always ensure you work on a clean working directory to avoid potential loss of unsaved changes.
- When in a detached HEAD state, remember that any commits you make will not belong to any branch unless you explicitly create one.
- Tagging important commits using `git tag <tag-name>` can serve as a backup, making it easier to access significant points in your project’s history.
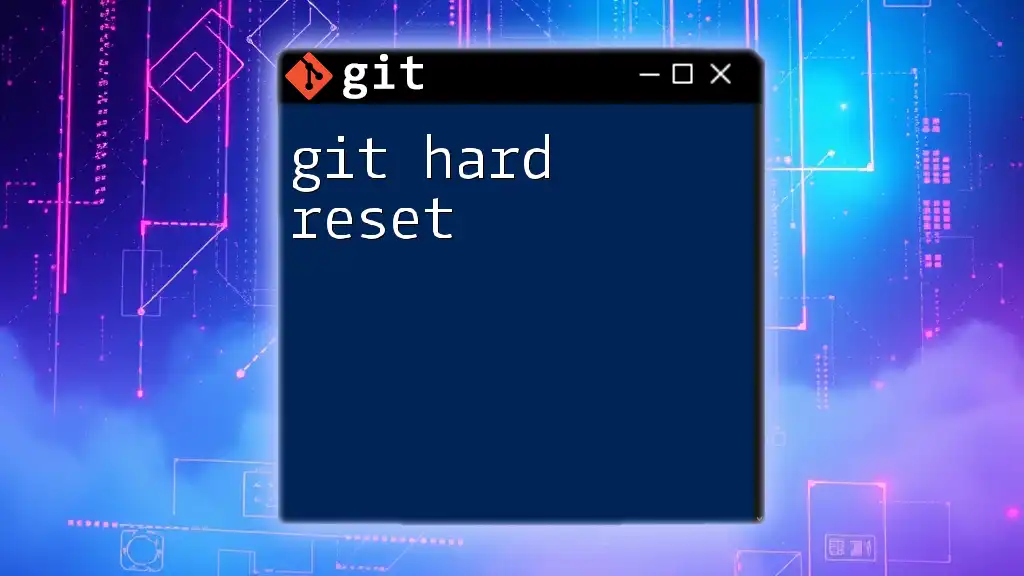
Conclusion
Understanding git head 1 is essential for any version control strategy. It serves as a fundamental concept in navigating branches and commits, tracking changes in your project. By mastering HEAD, you can greatly enhance your efficiency and confidence when using Git. Continue to explore Git features and practice these commands for a more robust experience in managing your codebase!
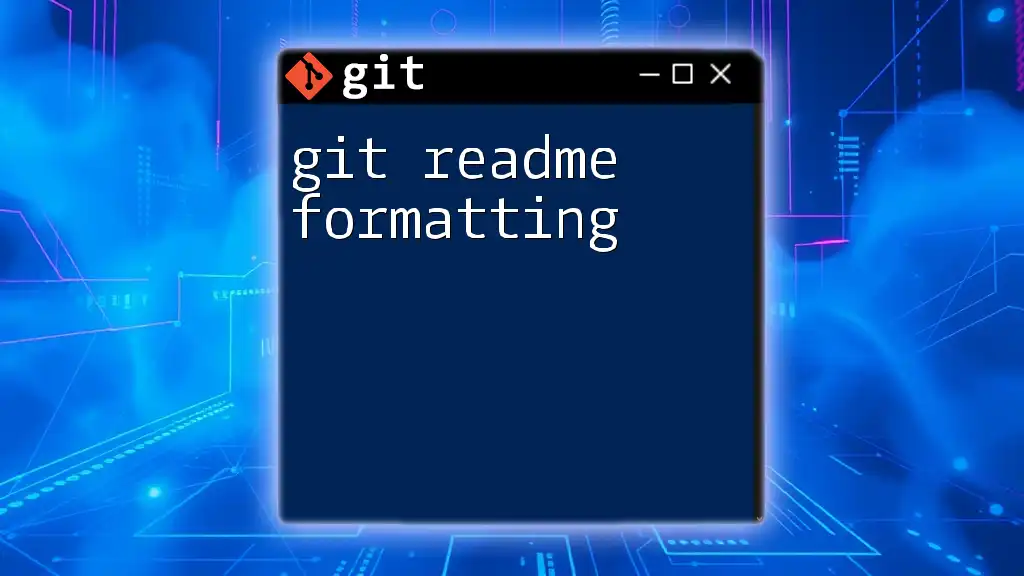
Additional Resources
For further learning, you may explore the official Git documentation and check out recommended tutorials or books to deepen your understanding of Git's powerful capabilities.