GitLab CI/CD is a tool that automates the software development lifecycle by allowing you to define pipelines for continuous integration and continuous deployment directly within your GitLab repository.
Here's a simple GitLab CI/CD configuration example in a `.gitlab-ci.yml` file:
stages:
- build
- test
- deploy
build_job:
stage: build
script:
- echo "Building the application..."
test_job:
stage: test
script:
- echo "Running tests..."
deploy_job:
stage: deploy
script:
- echo "Deploying the application..."
Understanding GitLab CI/CD
What is GitLab CI/CD?
GitLab CI/CD is an integrated part of GitLab that automates the software development process through Continuous Integration (CI) and Continuous Deployment (CD). It seamlessly integrates with the version control features of GitLab, allowing developers to build, test, and deploy their applications automatically whenever they push changes to their code repository. This automation leads to a quick feedback loop, enabling faster and more reliable software development cycles.
Benefits of Using GitLab CI/CD
There are several compelling reasons to adopt GitLab CI/CD in your development workflow:
-
Improved Code Quality: CI/CD allows for automated testing of code upon each commit, significantly reducing the risk of bugs and vulnerabilities slipping through to production. This ensures that only well-tested code is released.
-
Faster Deployment: With GitLab CI/CD, the entire process from code changes to deployment can be automated, resulting in quicker iterations and time-to-market for new features.
-
Enhanced Collaboration: CI/CD encourages team collaboration by ensuring that everyone is working with the same code base and processes. This creates a unified workflow that can help avoid 'integration hell.'
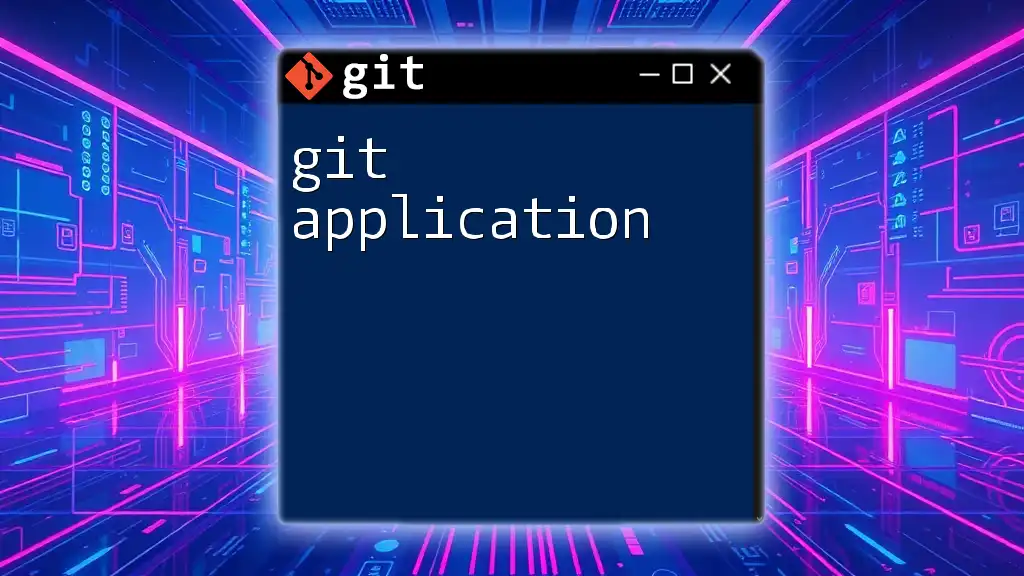
Setting Up GitLab CI/CD
Prerequisites for GitLab CI/CD
Before diving into the setup, ensure you meet the following prerequisites:
- A basic understanding of Git and GitLab is necessary to effectively utilize GitLab CI/CD.
- Access to a GitLab repository where you want to implement CI/CD.
- Proper permissions to configure CI/CD features in the repository settings.
Creating a .gitlab-ci.yml File
The backbone of your GitLab CI/CD setup is the `.gitlab-ci.yml` file. This file defines the pipeline and various jobs to be executed. The structure is simple yet powerful.
A basic example of a `.gitlab-ci.yml` file might look like this:
stages:
- build
- test
- deploy
build_job:
stage: build
script:
- echo "Building the application..."
test_job:
stage: test
script:
- echo "Running tests..."
deploy_job:
stage: deploy
script:
- echo "Deploying application..."
In this example, there are three stages: build, test, and deploy. Each job is assigned to a specific stage and executes its script when the pipeline runs.
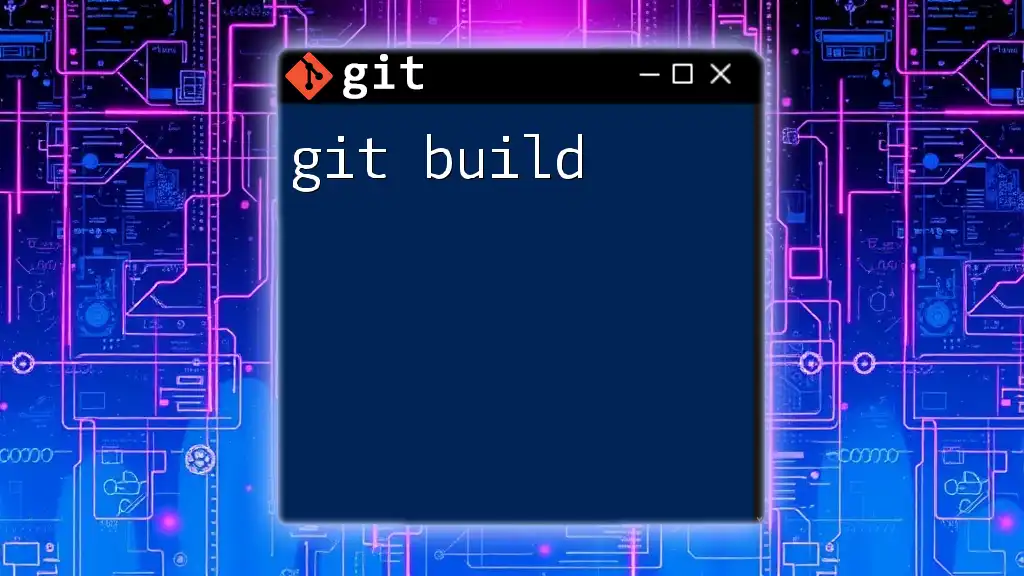
GitLab CI/CD Processes
CI/CD Pipeline Overview
A CI/CD pipeline is a series of automated processes that outline the workflow from coding to deployment. It consists of jobs that run sequentially through defined stages like build, test, and deploy. Each job can have specific command-line instructions, called scripts, and creates artifacts that can be used in later stages.
Breaking Down the .gitlab-ci.yml Structure
Understanding the components of your `.gitlab-ci.yml` file is crucial:
-
Stages: Defined under the `stages` keyword, these dictate the sequence of execution in the pipeline.
-
Jobs: Each job represents a specific task performed during a stage. Jobs can run scripts, perform tests, or deploy applications.
-
Scripts: These are the commands executed by the jobs.
-
Artifacts: These are files created by jobs that can be passed to subsequent jobs.
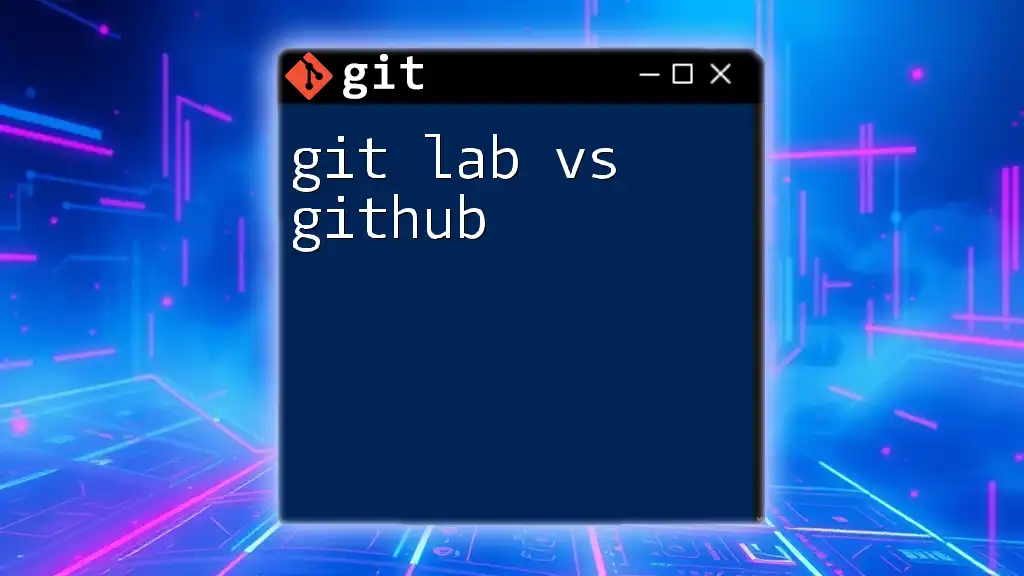
Implementing CI/CD Workflows
Creating a Simple CI/CD Workflow
To see GitLab CI/CD in action, let’s create a simple CI/CD workflow for a sample project. Below is an example `.gitlab-ci.yml` incorporating caching, which improves build speed:
cache:
paths:
- node_modules/
stages:
- install
- test
install_job:
stage: install
script:
- npm install
test_job:
stage: test
script:
- npm test
In this configuration, the `install_job` runs first, ensuring that all dependencies are installed. The second part, `test_job`, runs the tests to validate that the application is functioning as expected.
Managing Environments
Managing different deployment environments—such as development, staging, and production—is an integral part of GitLab CI/CD. By defining environments in your `.gitlab-ci.yml`, you can ensure that different configurations or scripts are executed based on the deployment target.
Implementing Branch-Specific CI/CD
GitLab CI/CD allows for granular control over when jobs are executed using the `only` and `except` keywords, creating branch-specific pipelines. For instance, if you want your tests to run only on the `main` branch, you can configure your `.gitlab-ci.yml` like this:
test_job:
stage: test
script:
- echo "Running tests on main branch"
only:
- main
This configuration ensures that the job will not run for branches other than `main`, effectively controlling the CI/CD process based on your branching strategy.
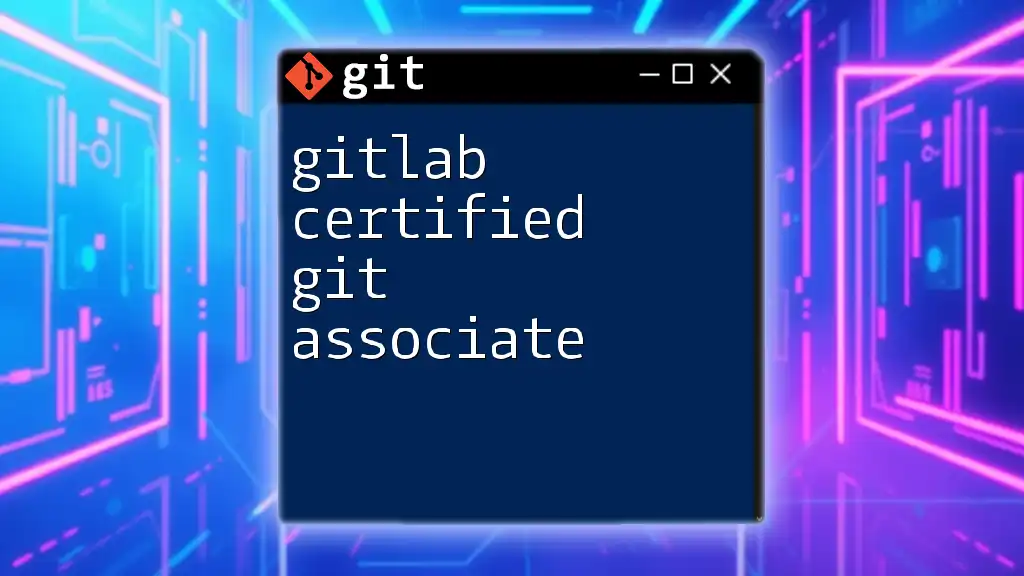
Monitoring and Debugging CI/CD Pipelines
Viewing Pipeline Status
Once your CI/CD pipeline is set up, monitoring its status is crucial. GitLab provides an intuitive interface to view all pipelines and detailed logs for each job, making it easy to track progress and identify issues.
Debugging Failed Jobs
Job failures can occur for various reasons, such as incorrect configurations, missing dependencies, or failed tests. Understanding how to debug failed jobs is essential. Common steps include:
- Reviewing the detailed logs available in the GitLab UI for clues on what went wrong.
- Checking configuration settings in your `.gitlab-ci.yml` for correctness.
- Running the highlighted scripts locally to verify the expected behavior outside of the CI/CD environment.
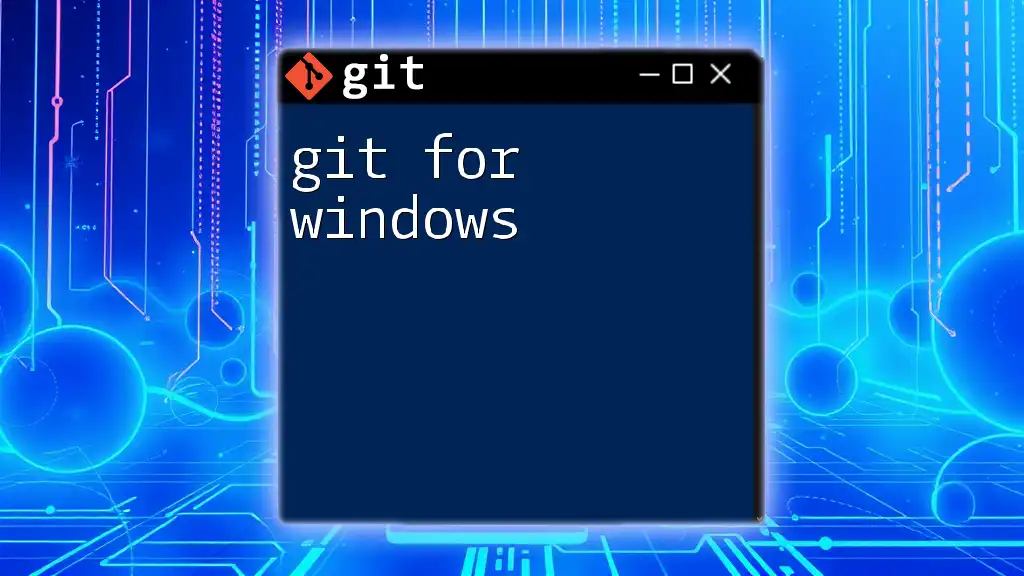
Advanced GitLab CI/CD Features
Using Variables in GitLab CI/CD
GitLab CI/CD supports both predefined and custom variables, which can be used to store configuration settings, secrets, and more. For example, you can define a variable for your testing environment:
variables:
NODE_ENV: "test"
test_job:
script:
- echo "Running in $NODE_ENV environment"
This example demonstrates how to use variables to tailor job behaviors based on different environment settings.
Integrating External Services
GitLab CI/CD can easily integrate with various external services and tools. For instance, if you're using Docker, you can specify Docker images directly within your `.gitlab-ci.yml` to run jobs in a containerized environment:
build_job:
image: node:14
script:
- npm run build
This capability adds flexibility to your CI/CD processes, further enhancing automation.
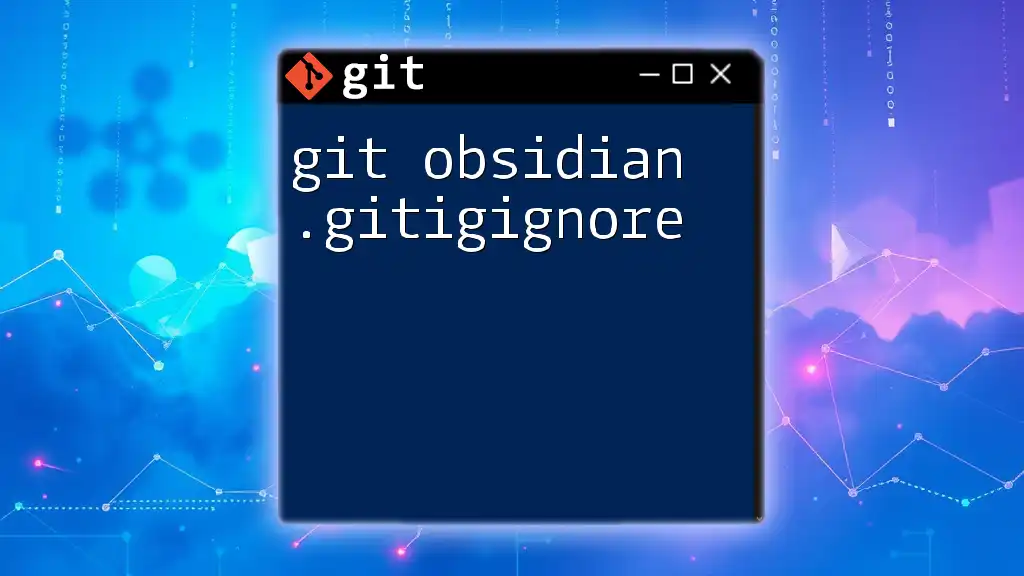
Best Practices for GitLab CI/CD
To maximize the effectiveness of GitLab CI/CD, consider the following best practices:
-
Keep CI/CD Scripts Simple: Aim for modular scripts that are easy to read, maintain, and debug.
-
Proper Resource Allocation: Ensure that jobs are allocated sufficient resources without exceeding limits, preventing slow build times.
-
Regular Review: Continuously review and update your CI/CD configurations based on project needs and team feedback, ensuring the setup remains relevant and efficient.
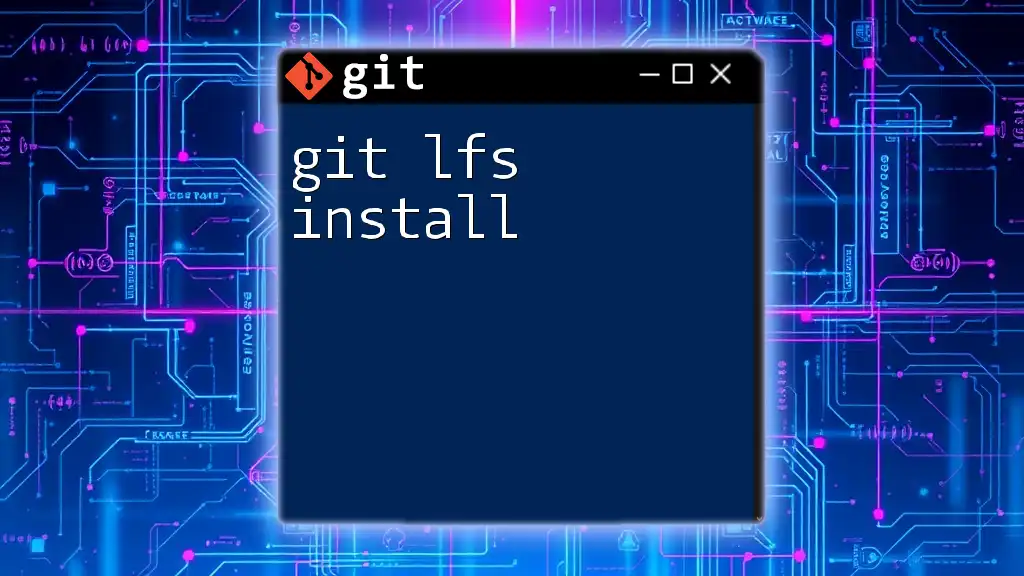
Conclusion
Implementing GitLab CI/CD can significantly enhance your development process by automating the build, test, and deployment cycle. The benefits reaped through improved code quality and faster deployment times cannot be overstated. As you explore GitLab CI/CD further, you will find that it is not just a tool but a methodology that fosters a culture of continuous improvement among development teams. Embrace the power of automation and take your development process to the next level with GitLab CI/CD.
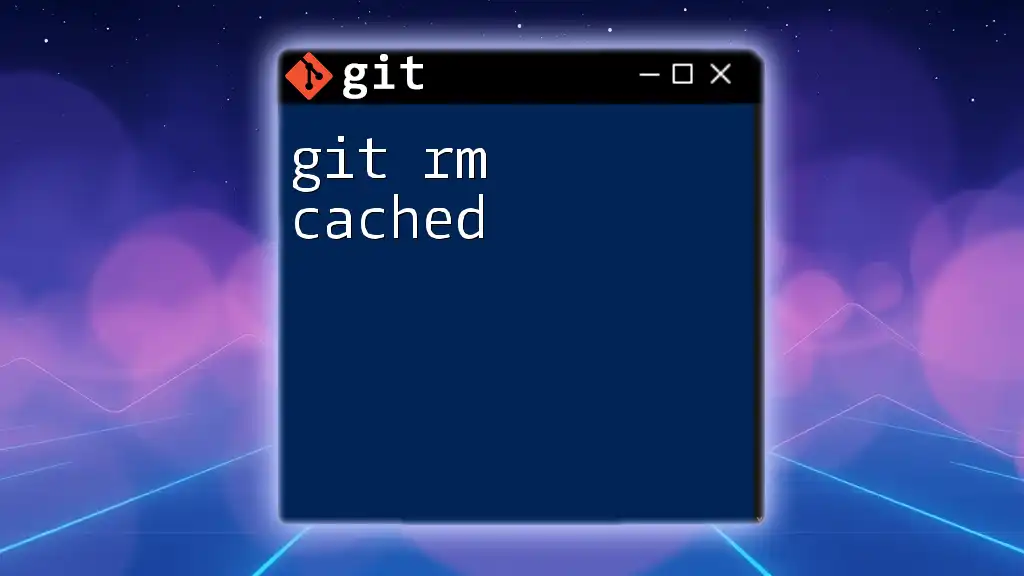
Additional Resources
For further exploration:
- Visit the [official GitLab CI/CD documentation](https://docs.gitlab.com/ee/ci/).
- Look for recommended tutorials and courses to deepen your understanding.
- Join community forums and support channels for GitLab users to connect and learn from others.