You can find the largest files in your Git history by using the following command, which lists files sorted by size and displays the top entries.
git rev-list --objects --all | git cat-file --batch-check='%(objecttype) %(rest)' | grep '^blob ' | awk '{print $2}' | git cat-file --batch-check='%(objectsize:disk) %(rest)' | sort -n -r | head -n 10
Understanding Git’s File Storage
How Git Stores Files
In Git, every file is treated as an object, and these objects are stored in a unique way that optimally tracks changes over time. Git primarily uses three types of objects: blobs, trees, and commits.
- Blobs are the binary file contents. Each file is stored as a blob, which is uniquely identified by a hash.
- Trees represent directories and link to blobs and other trees, maintaining the structure of your project.
- Commits are snapshots that link to a tree object, along with metadata like the author, date, and message.
What Makes a File "Large"?
Determining what constitutes a "large" file in your Git repository often comes down to context and usage. Generally, files larger than 100 KB can start to affect performance, and sizes over 1 MB are often considered problematic. Common file types that lead to large sizes include images (like `.png` or `.jpg`), compiled binaries, and media files. Understanding this helps set reasonable expectations for file management.
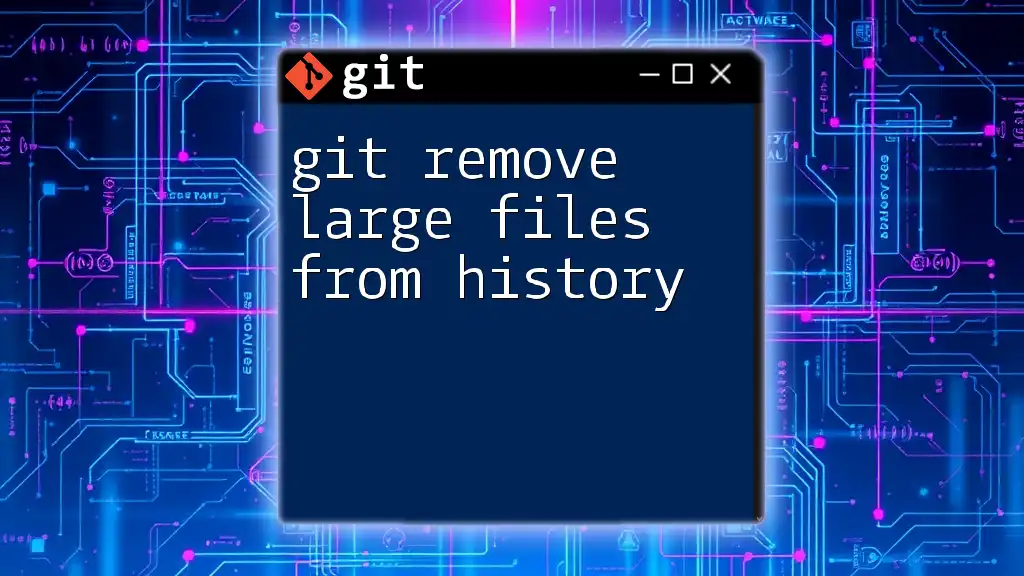
Prerequisites
Required Tools
Before you start searching for large files, make sure you have Git installed on your machine. This typically includes having access to the terminal or command line interface where you can run the necessary commands.
Setting Up Your Repository
If you're working on an existing project, you should begin with a basic Git setup. Clone the repository you want to analyze using:
git clone <repository-url>
cd <repository-name>
This way, you’ll have the repository on your local machine to execute the commands.
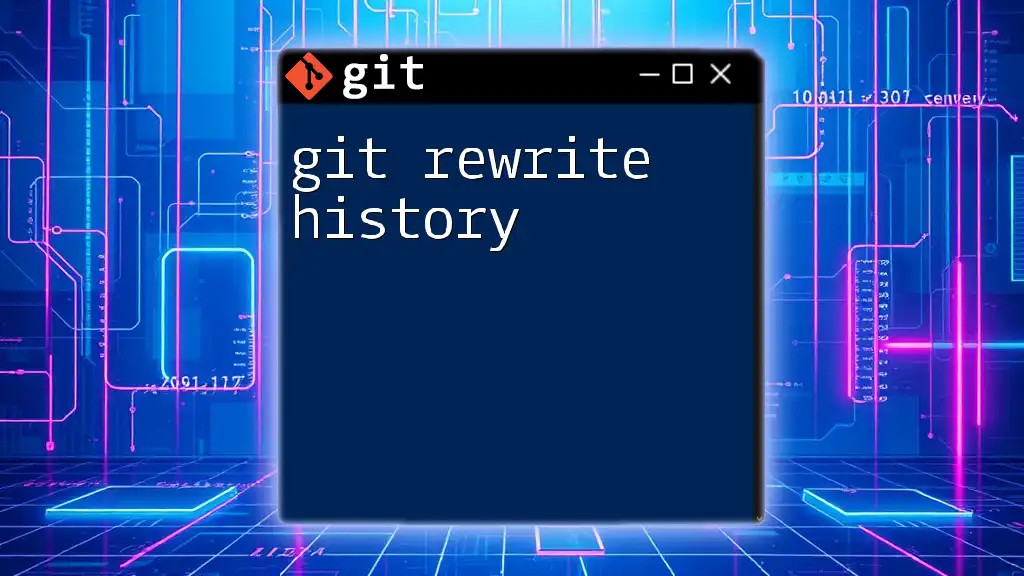
Finding the Largest Files in Your Git History
Using Git Commands
The `git rev-list` Command
One of the primary methods to find the largest files in your Git history is to utilize the `git rev-list` command. This command allows you to list all the objects in the repository. The following command does that while filtering only the blob objects:
git rev-list --objects --all | git cat-file --batch-check='%(objecttype) %(objectname) %(rest)' | grep '^blob' | sort -k 2 -n
In this command:
- `git rev-list --objects --all` fetches all objects in the repository.
- `git cat-file --batch-check` checks the contents of these objects.
- `grep '^blob'` filters out only the blob objects.
- `sort -k 2 -n` sorts the blobs numerically based on their size.
Combining with `git verify-pack`
To enhance performance, especially in larger repositories, you might want to combine the above command with `git verify-pack`. This command helps you analyze packed objects more efficiently. Use the following command:
git verify-pack -v $(git rev-parse --git-dir)/objects/pack/pack-*.idx | sort -k 3 -n
The output will show you the largest files in your packed Git history, ordered by size.
Analyzing Output
Once you run these commands, you'll receive a list displaying file sizes along with their object IDs. Understanding this output is crucial for managing your files—large files will be highlighted, allowing you to identify potential candidates for removal or optimization.
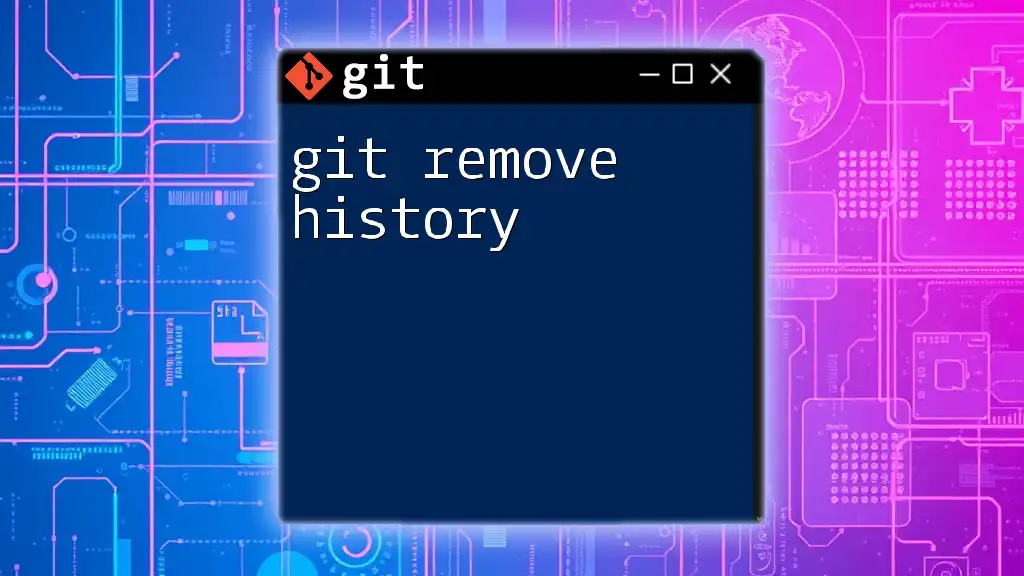
Visualizing Large Files Using Additional Tools
Using `git-sizer`
For a more user-friendly visualization, consider using the `git-sizer` tool. This utility gives you a detailed breakdown of your repository's size and its components.
Installation Instructions
To get started, you’ll first need to install `git-sizer`. If you are on macOS, you might install it using Homebrew:
brew install git-sizer
If you use other operating systems, follow the appropriate installation methods.
Running `git-sizer`
Once installed, simply run the tool using:
git-sizer
You will receive a report detailing the sizes of files, blobs, and other objects in your Git repository. This report allows you to visualize which files take up the most space.
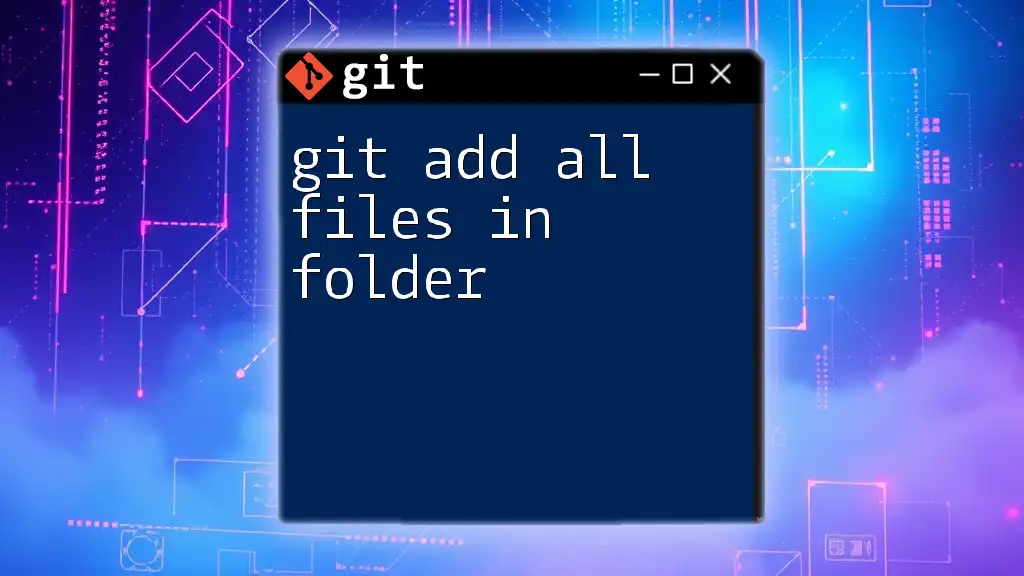
Managing and Reducing Large Files
Strategies for Handling Large Files
Having identified large files, the next step is managing them effectively. One common strategy is to use Git LFS (Large File Storage). Git LFS allows you to handle large files more efficiently by storing them outside your main Git repository.
Using Git LFS
To work with Git LFS, begin by installing it:
git lfs install
You can then specify file types to be tracked with Git LFS, as shown below:
git lfs track "*.psd" # Example for Photoshop files
This setup helps keep your repository lightweight, as large files are stored separately and only pointers to their locations are stored in your repository.
Removing Large Files from History
If you need to remove large files from the Git history, the `git filter-repo` tool is an effective solution. This tool enables you to rewrite Git history to exclude large files.
The `git filter-repo` Tool
To leverage `git filter-repo`, first ensure it is installed. Then, execute a command to remove a specific file from the history:
git filter-repo --invert-paths --path largefile.zip # Example command to remove largefile.zip from history
This command rewrites the repository's history, effectively removing the specified large file from all historical commits.
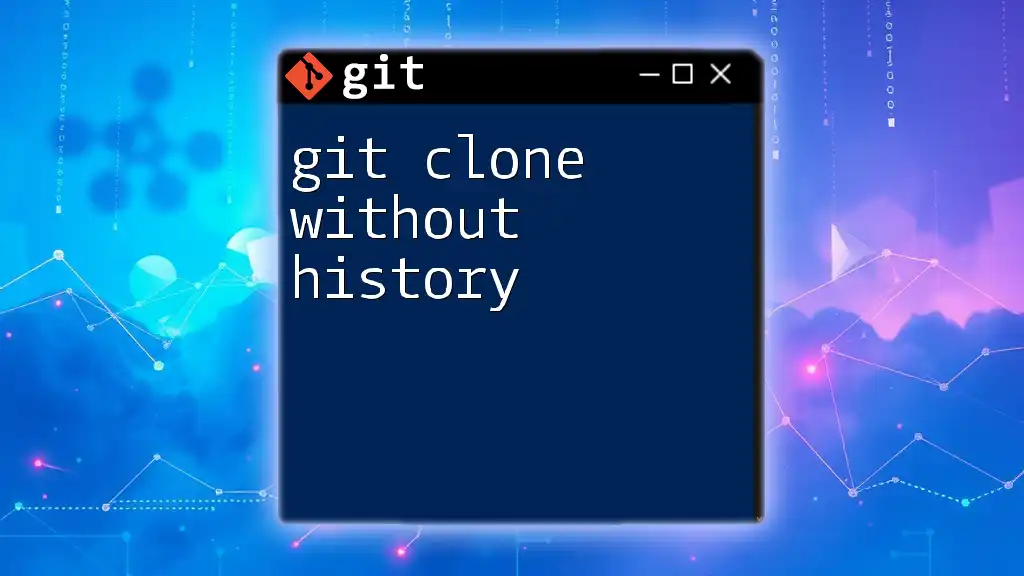
Best Practices for Managing File Size in Git
Regular Audits of Repository Size
To maintain a healthy repository, conduct regular audits. This practice involves checking for unusually large files and assessing how they impact your project's performance. Utilize the previously mentioned commands routinely to stay informed.
Setting Up File Size Limits
Implementing repository policies regarding acceptable file sizes is another crucial step. Define thresholds for file sizes that can be included, ensuring team members are aware of these changes. This standardization aids in preventing future issues.
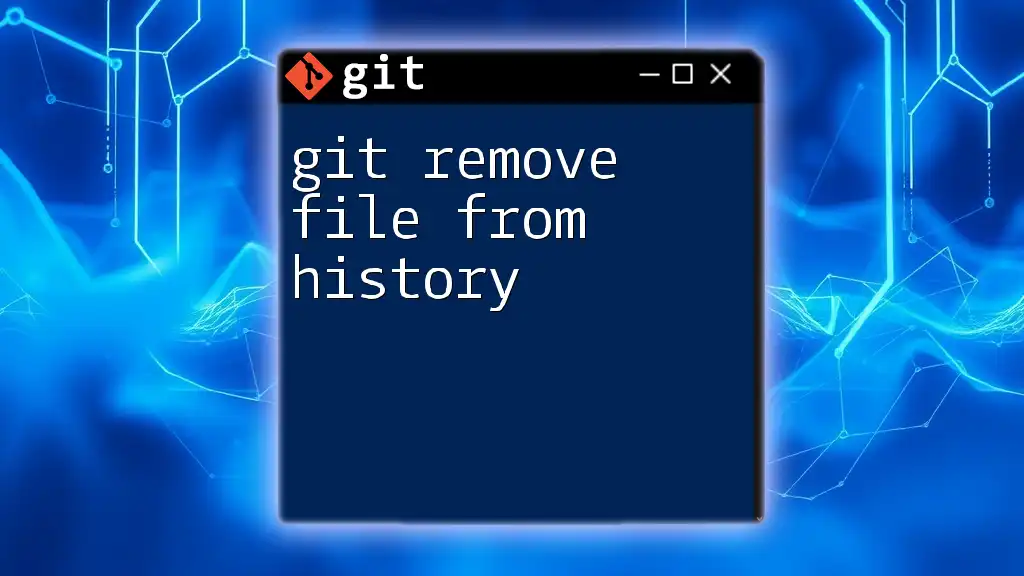
Conclusion
Managing large files in Git history is crucial for maintaining both performance and repository efficiency. Monitoring file sizes, using tools like Git LFS, and regularly auditing your repository will help keep your projects optimized. Remember to continually evaluate your repository practices as your project evolves to ensure a smooth development process.
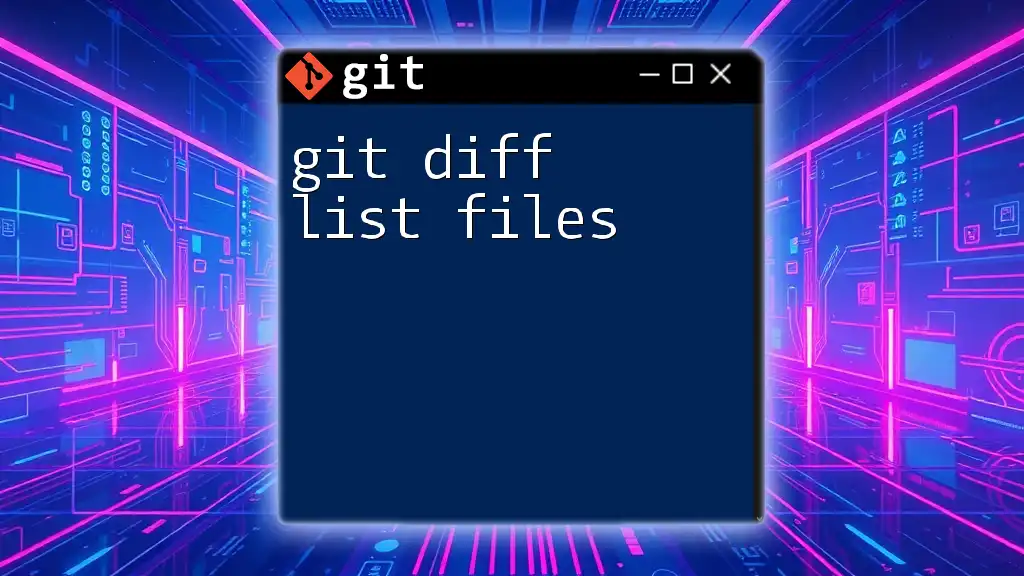
Additional Resources
For further reading and tools, consult the [Git documentation](https://git-scm.com/doc) and explore other applications specialized in file management within Git. These resources will aid in enhancing your skills and knowledge regarding file management in Git repositories.