The `git checkout` command is used to switch between branches or restore working tree files in Git.
git checkout <branch-name>
What is `git checkout`?
Definition and Purpose
The `git checkout` command is one of the fundamental tools in Git. It allows you to navigate between different branches or commits, bringing the content of your working directory in line with the version of the project stored in those branches or commits. This is essential for collaborative work and version control, providing a way to isolate tasks and manage features efficiently.
Real-World Scenarios
Imagine you’re working on a project that requires adding a new feature while still maintaining release stability. Using `git checkout`, you can create a separate branch for this new feature without affecting the main (often referred to as `main` or `master`) branch. You can also check back to previous code versions when troubleshooting, making it an invaluable tool for developers.
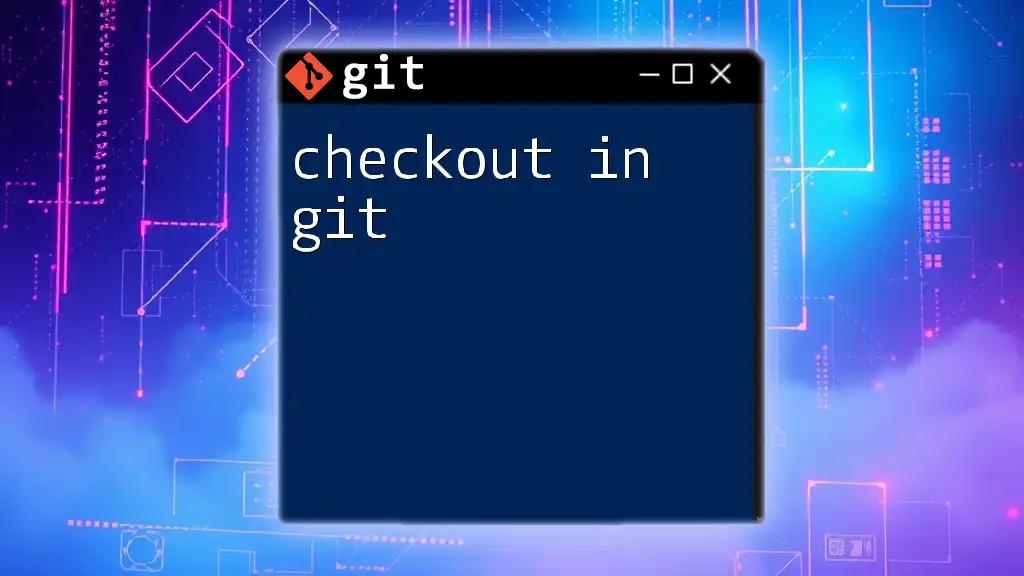
Basic Syntax of `git checkout`
General Structure
The basic syntax for `git checkout` can be structured as follows:
git checkout [branch-name | commit-hash | file-path]
- branch-name: The name of the branch you want to switch to.
- commit-hash: A unique identifier for a commit that you may want to check out.
- file-path: The specific file(s) you wish to restore to their last committed state.
Understanding this syntax lays the groundwork for mastering the command.
Using `git checkout` with Branches
Switching Branches
To switch from your current branch to another, such as moving from your `feature` branch to the `develop` branch, you would use:
git checkout develop
This command updates your working directory, as well as the staging area, to reflect the state of the `develop` branch. It's important to be aware of any uncommitted changes, as they might interfere with the switch.
Creating and Switching to a New Branch
If you decide to create a new branch dedicated to a feature, you can do so with a single command:
git checkout -b new-feature
The `-b` flag creates a new branch and checks it out in one seamless action. This practice is not only efficient but also encourages a clear branching strategy, making it easier to manage multiple developments simultaneously.

Advanced Usage of `git checkout`
Checking Out Previous Commits
Moving to a Specific Commit
To navigate to a previous commit, you can use:
git checkout abc1234
In this instance, `abc1234` represents the unique hash of the commit you wish to inspect. Upon executing this command, your repository enters a detached HEAD state, indicating that you are not on any branch. While in this state, your changes won't belong to a branch until you either create a new branch or return to an existing one.
Restoring Files to a Previous State
Checking Out Specific Files
Sometimes, you might want to revert certain files to their last committed versions without affecting the entire branch. You can do this using:
git checkout -- file.txt
The `--` indicates that what follows is a file path rather than a branch name. This command retrieves `file.txt` from the last commit and replaces it with the current version in your working directory. It’s an efficient way to undo uncommitted changes for specific files.
Undoing Changes in the Working Directory
Unstaging Changes
If you've added changes to the staging area but wish to remove them, `git checkout` can help:
git checkout -- <file>
This command will revert the file back to its last committed state, effectively unstaging any modifications. It's crucial to understand this capability, as it can often prevent unnecessary commits and keep your repository clean.
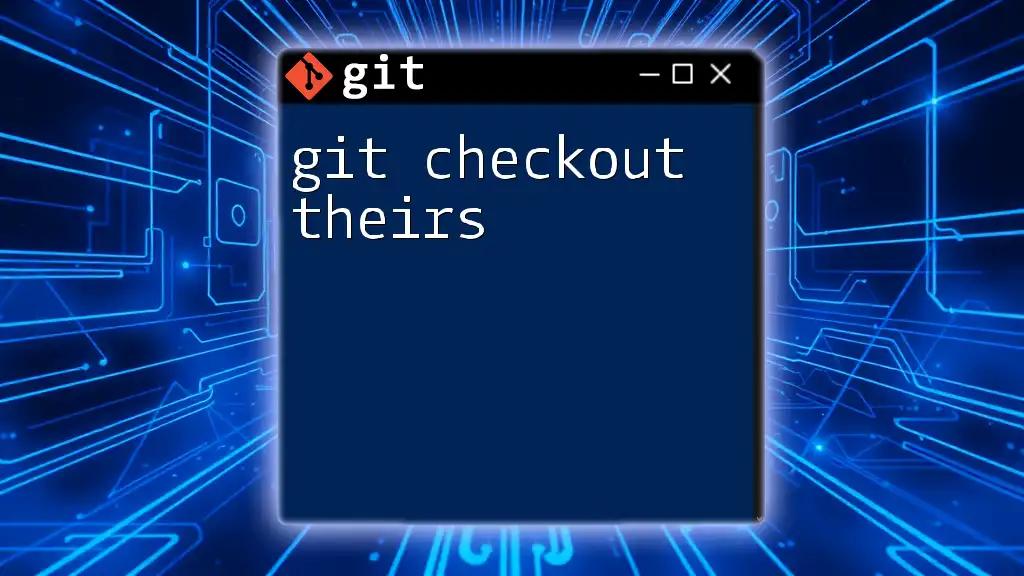
Common Mistakes and Troubleshooting
The "Detached HEAD" State
When you check out an old commit, you find yourself in a detached HEAD state. This means that while you can view and interact with the files at that commit, any new commits you create won’t belong to any branch. If you wish to retain your changes, it's vital to create a new branch before making any new commits:
git checkout -b new-branch-name
This action will safeguard your work and keep your commit history intact.
Conflicts During Checkout
Conflicts may occur if there are changes in the working directory that haven’t been committed while attempting a branch switch. Git won’t allow the checkout until you resolve those conflicts. You have a few options:
- Stash your changes: Save your local modifications temporarily using `git stash`.
- Commit your changes: Finish your current work by committing it.
- Discard your changes: Use `git checkout -- <file>` for specific changes or `git reset --hard` to get back to a clean state. Be cautious with this option as it cannot be undone.
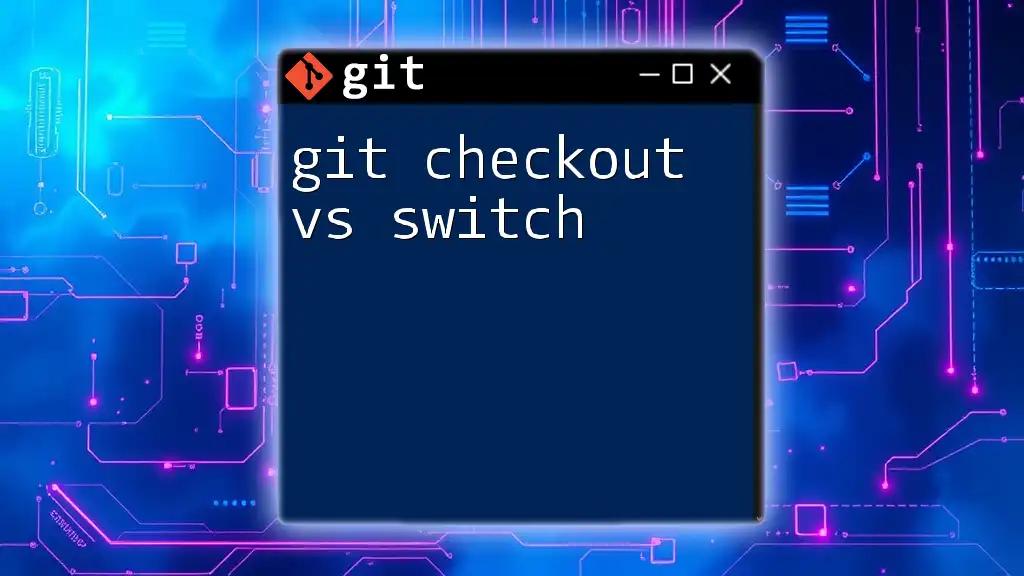
Alternatives to `git checkout`
Using `git switch` for Branch Operations
Introduced in more recent versions of Git, `git switch` serves as a clearer alternative for switching branches:
git switch develop
This command enhances readability, distinguishing branch switching from file restoration purposes, which can sometimes create confusion with `git checkout`.
Using `git restore` for File Operations
Similarly, `git restore` provides a dedicated command for restoring files to their last committed state:
git restore file.txt
This differentiation is advantageous for both learning and practical usage, helping prevent mistakes that often occur when using only `git checkout`.
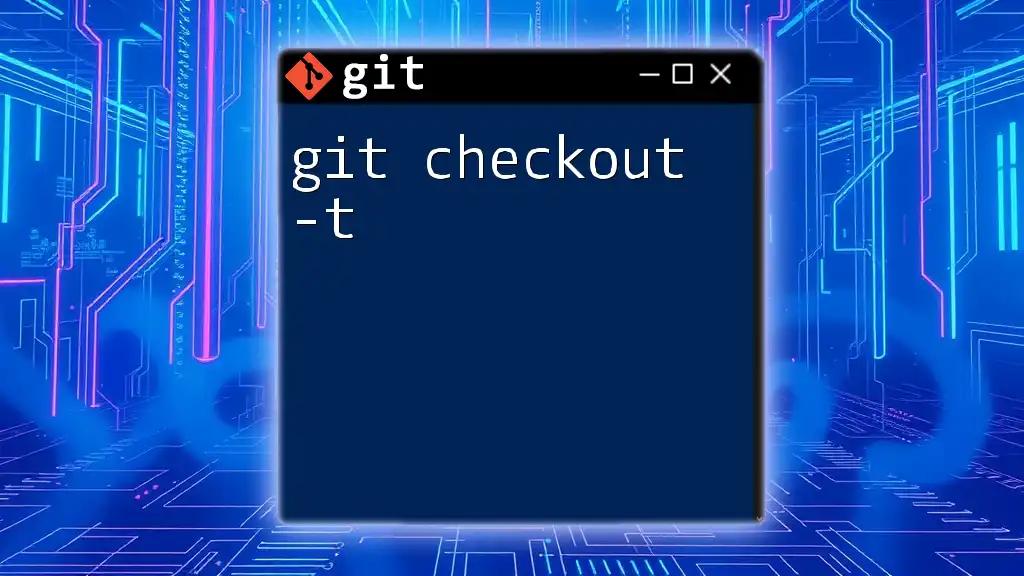
Conclusion
In mastering the `git checkout` command, you gain powerful control over your development workflow. From switching branches to managing file states and navigating your commit history, this command is foundational in using Git effectively. Practice utilizing these commands in your projects to reinforce your understanding and enhance your productivity.
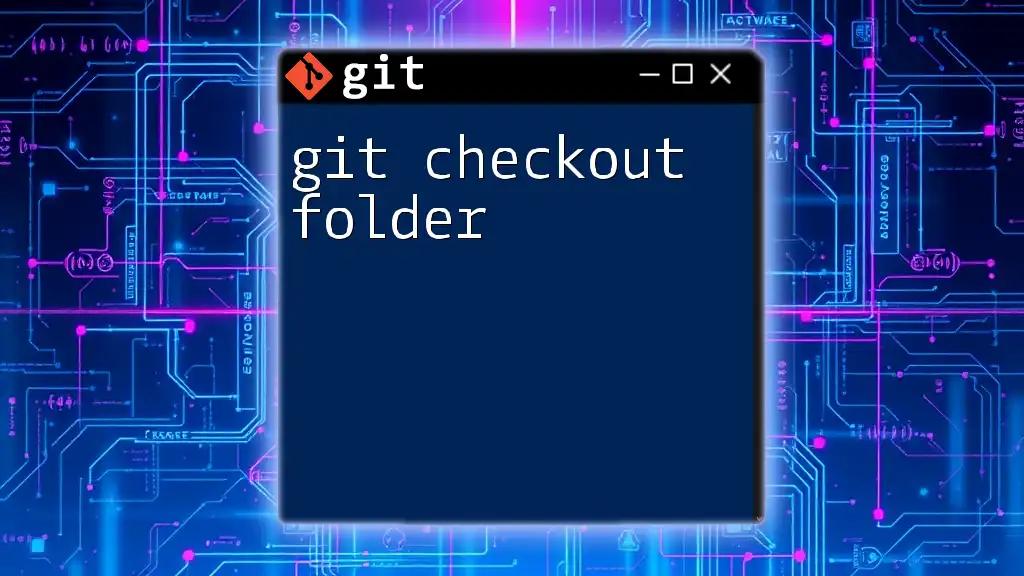
Additional Resources
For a deeper understanding of Git, explore the official Git documentation and various online tutorials. Community forums can also be invaluable for gaining insights and tips from fellow developers.
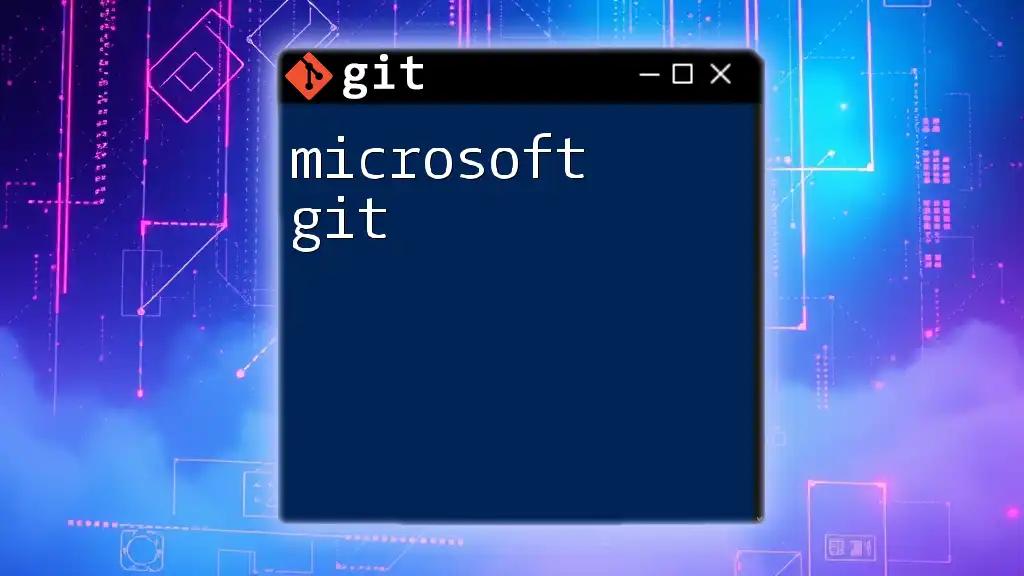
Call to Action
We encourage you to share your experiences and any tips you've discovered while using `git checkout`. Stay tuned for more articles that will expand your Git skills and enhance your command over version control.