To automatically pull the latest changes from a Git repository at scheduled intervals, you can set up a cron job in Linux using the following command in your crontab file.
* * * * * cd /path/to/your/repo && git pull origin main
This cron job will execute every minute, navigating to your repository directory and pulling the latest updates from the `main` branch.
Understanding Git Repositories
What is a Git Repository?
A Git repository is a storage space where your project files and their revision history are kept. It allows you to track changes, collaborate with others, and manage different versions of your projects efficiently. Understanding the structure of a Git repository—which includes the working directory, staging area, and the .git directory—is crucial for effective version control. Keeping your repository updated ensures that you are working with the latest version of the code, which is vital in a collaborative environment.
The Role of Git Commands
Git commands are the tools you’ll use to interact with your repository. Among these commands, `git pull` is particularly significant. This command fetches changes from a remote repository and merges them into your current branch, ensuring that your local copy reflects the most up-to-date state of the project. For anyone looking to automate their workflows, understanding how to use this command effectively is essential.

Setting Up the Environment
Prerequisites
Before diving into the setup, ensure that you have the necessary software installed on your Linux machine. Primarily, you will need Git and Cron. To install Git, you can use the package manager for your distribution. For example, on Debian-based systems, you can run:
sudo apt-get update
sudo apt-get install git
After installation, confirm that Git has been installed correctly by running:
git --version
Creating a Git Repository
To demonstrate how to set up your cron job for pulling the latest Git repo, you’ll first need a Git repository. You can create a new one by following these steps:
- Create a new directory for your repository:
mkdir my-repo
cd my-repo
- Initialize the Git repository:
git init
- Add a remote origin, which is the URL of your remote repository:
git remote add origin <repository-url>
Replace `<repository-url>` with the actual URL of your remote Git repository. Now you have a basic setup to work with.

Introduction to Cron Jobs
What are Cron Jobs?
Cron jobs are scheduled tasks in Linux that allow users to automate repetitive actions. By defining cron jobs, you can ensure that certain commands or scripts run at specified intervals, enhancing productivity. The cron syntax has a specific format, which includes five fields representing minute, hour, day of the month, month, and day of the week, followed by the command you wish to run.
How to Edit the Crontab
To create or edit a cron job, you access the crontab file using:
crontab -e
This command opens the crontab in the default editor, allowing you to add or edit scheduled tasks according to your needs.
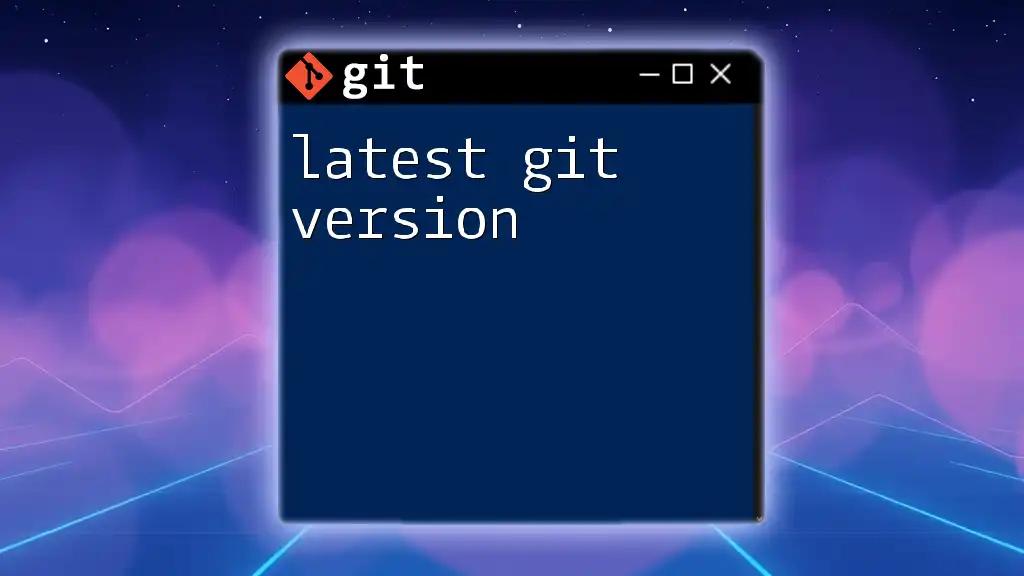
Automating `git pull` with Cron Jobs
Writing the Script
To automate the process of pulling the latest changes from your Git repository, you will need to create a simple shell script. This script will contain the commands necessary to perform the `git pull` operation. Here's an example:
#!/bin/bash
cd /path/to/your/repo
git pull origin main >> /path/to/logfile.log 2>&1
In this script:
- The `cd` command changes the directory to your local Git repository.
- The `git pull` command fetches and merges changes from the remote repository. Ensure you replace `main` with the branch you want to pull.
- The output and any error messages are appended to a log file for later review.
Setting Up the Cron Job
Example Crontab Entry
Once your script is ready, you can set up a cron job to run it at specified intervals or at a specific time. An example crontab entry might look like this:
* * * * * /path/to/your/script.sh
This entry would execute your script every minute. Understanding each part of this entry is essential:
- The first five asterisks correspond to minute, hour, day of the month, month, and day of the week, respectively.
- Modify these values as needed to suit your schedule.
Testing the Cron Job
After adding your cron job, it’s wise to test your shell script separately to ensure it runs without issues. Execute it manually:
bash /path/to/your/script.sh
To check if your cron job is set properly and is executing as expected, you can look at the cron logs. The log files can typically be found at `/var/log/syslog` or `/var/log/cron.log`, depending on your system configuration.
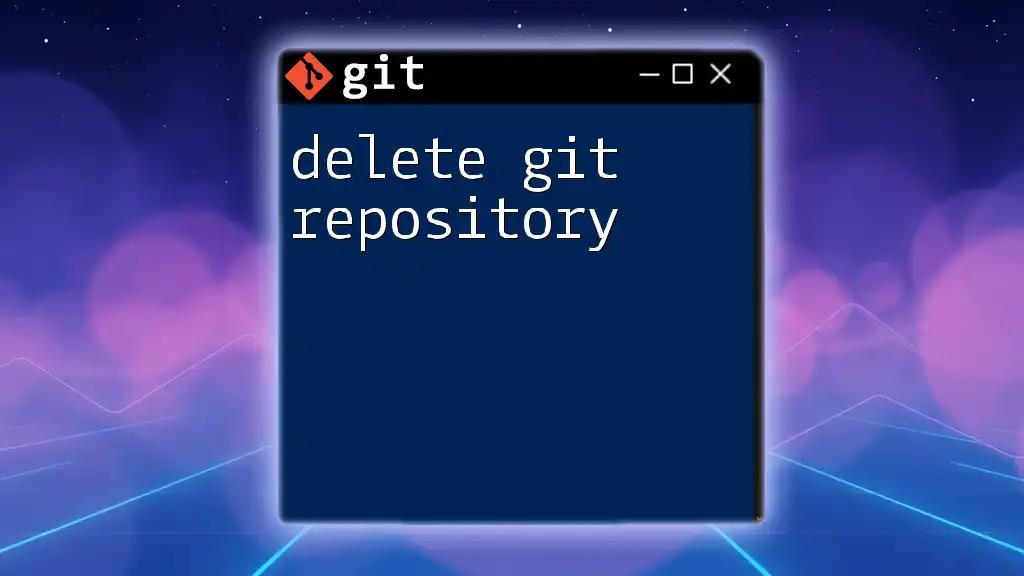
Common Issues and Troubleshooting
Permissions Problems
A common pitfall when executing scripts via cron is permissions errors. The cron environment runs under the user who created the job. You must ensure that this user has the appropriate permissions to access the Git repository and execute Git commands. To modify permissions, you can use:
chmod +x /path/to/your/script.sh
Authentication for Private Repos
If your repository is private, you will need to handle authentication properly. There are two main approaches:
- SSH keys: This method is generally more secure. Generate a key pair using:
ssh-keygen -t rsa -b 4096 -C "your_email@example.com"
Then, add the public key to your Git service provider (e.g., GitHub, GitLab).
- HTTPS: If you opt for HTTPS, you may need to store your username and password in your Git configuration or use a personal access token.
Log File Monitoring
To facilitate easy monitoring of the operations performed by your cron job, logging the output is crucial. You can continuously monitor your log file using:
tail -f /path/to/logfile.log
This command will allow you to see real-time updates and catch any issues as they occur.
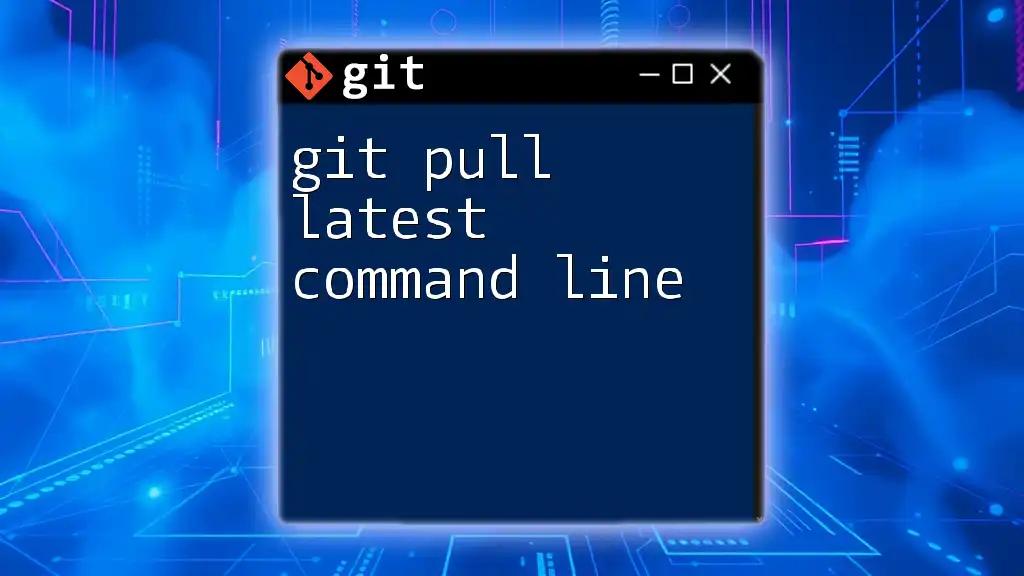
Best Practices
Security Practices
When managing scripts that access your repository, security is paramount. Avoid hard-coding sensitive credentials directly into your scripts. Instead, consider using environment variables or configuration files with restricted access.
Maintaining Your Cron Job
Regularly review your cron jobs to adapt to changes in your development cycle or project structure. Make sure to update the crontab entries as necessary to ensure they are always current, efficient, and relevant to your ongoing projects.
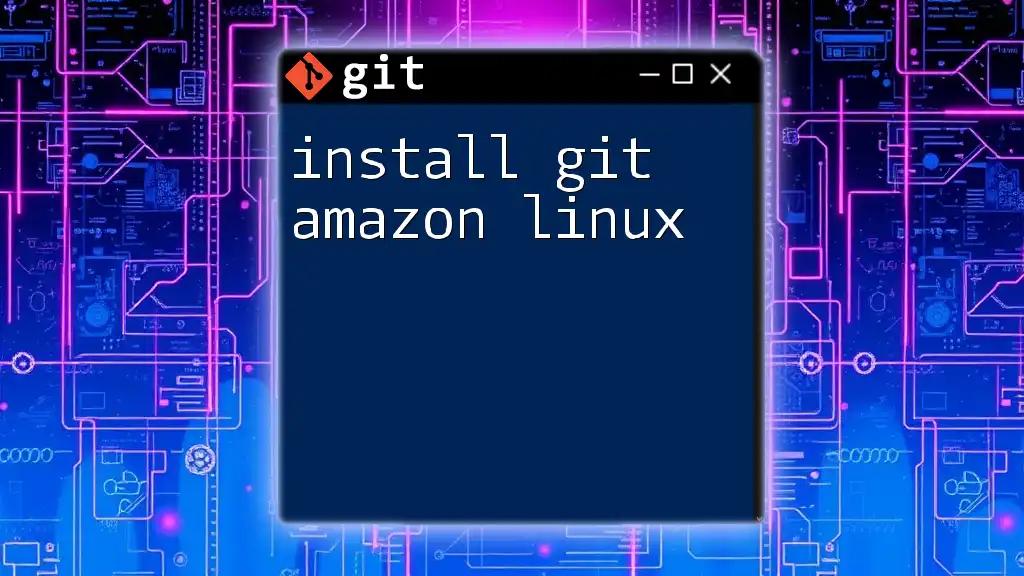
Conclusion
Automating the process to pull the latest Git repo using a cron job in Linux is a useful tactic for keeping your codebase updated effortlessly. By following the steps outlined in this guide, you can set up and maintain a cron job that regularly fetches the latest changes from your Git repository. As you explore automation further, consider how you can incorporate other Git commands and tools to optimize your workflows, enhancing both productivity and collaboration.