In this post, we'll explore the most popular Git shortcuts that streamline your workflow and boost your productivity.
# Stage all changes
git add .
# Commit changes with a message
git commit -m "Your commit message"
# Check the status of your repository
git status
# View the commit history
git log --oneline
# Create a new branch and switch to it
git checkout -b new-branch-name
# Merge a branch into the current branch
git merge branch-name
# Push changes to the remote repository
git push origin branch-name
# Pull changes from the remote repository
git pull origin branch-name
Understanding Git Basics
What is Git?
Git is a widely used version control system that allows developers to track changes in their codebases over time. It is essential for collaboration among teams, enabling multiple individuals to work on a project simultaneously while maintaining a clear history of modifications. Understanding core concepts like repositories, commits, and branches is vital for leveraging Git effectively.
Why Use Shortcuts?
In a fast-paced development environment, efficiency is critical. Shortcuts can significantly reduce the time spent on routine Git operations, allowing you to focus on coding rather than typing lengthy commands. Integrating these shortcuts into your daily workflow will help you become a more productive developer.
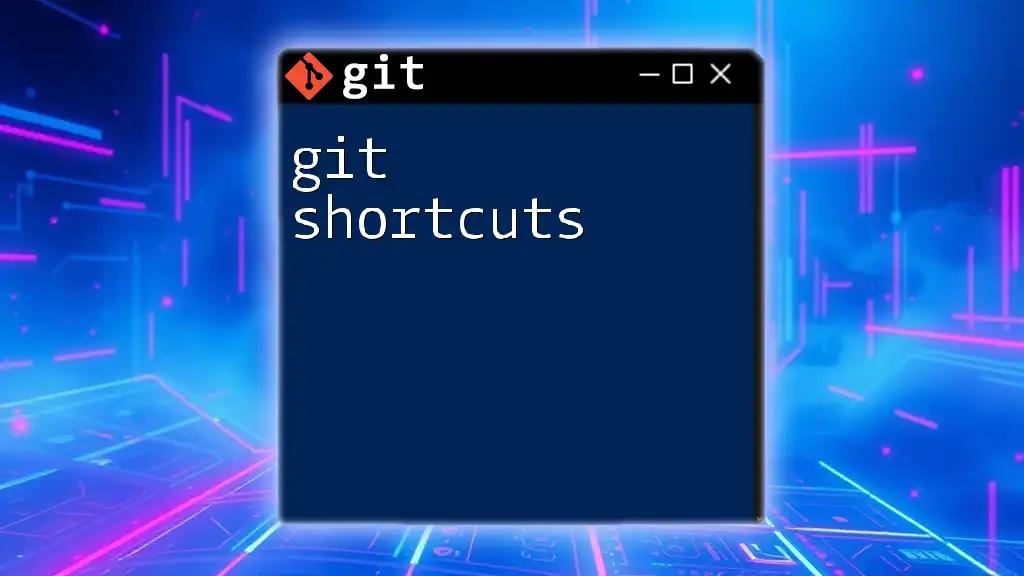
Essential Git Shortcuts
Setting Up Your Environment
Configuring User Information
Before diving into using Git, it is important to configure your user information to ensure that your contributions are correctly recorded. Use the following commands to set your name and email:
git config --global user.name "Your Name"
git config --global user.email "youremail@example.com"
Setting your user information is crucial for maintaining a clear record of contributions in collaborative projects.
Navigating Repositories
Changing Directories Quickly
Navigating to your Git repository can be accomplished quickly using the `cd` command. For example:
cd path/to/your/repo
This command allows you to swiftly enter your project directory, saving you time when collaborating on multiple projects.
Working with Branches
Creating a New Branch
Creating new branches is a fundamental aspect of working in Git. By using this command, you can instantly create and switch to a new branch:
git checkout -b new-branch-name
This shortcut combines two actions into one, making it easy to start new features without disrupting the main branch.
Switching Between Branches
To switch back to another branch, use the following command:
git checkout branch-name
This allows you to effortlessly navigate between branches as you work on various features or bug fixes.
Committing Changes
Staging Changes
When you're ready to save your modifications, you'll need to stage them first. Instead of staging files individually, you can stage all changes with:
git add .
This command simplifies the process of preparing your changes for a commit, especially in larger projects with numerous modified files.
Committing Changes
Once your changes have been staged, you can commit them with a concise message that describes your modifications:
git commit -m "Your commit message"
Writing thoughtful commit messages is essential for maintaining clarity in your project's history.
Reviewing History
Viewing Commit History
To view a simplified history of your commits, use the following command:
git log --oneline
This command presents a condensed view of your project’s history, making it easier to analyze past changes.
Diffing Changes
To see the differences between your working directory and the staged area, use:
git diff
This command is invaluable for reviewing what changes are pending before committing.
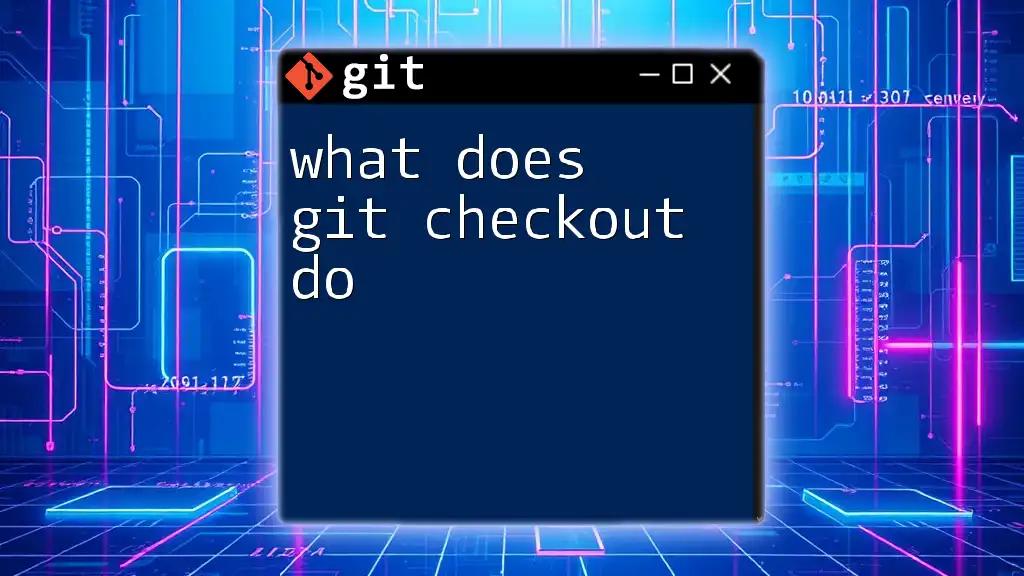
Advanced Shortcuts
Combined Commands
Fetch and Merge in One Step
To synchronize your local repository with changes from the remote, you can use:
git pull
This command fetches and merges remote changes into your current branch efficiently.
Undoing Changes
Checkout a File
If you need to discard changes made to a specific file, you can restore it to its last committed state with:
git checkout -- filename
This command allows you to undo modifications on a granular level.
Revert a Commit
To undo changes introduced by a specific commit, you can create a new commit that reverses those changes:
git revert commit-id
This approach ensures that your project's history maintains its integrity, rather than altering past commits.
Managing Remotes
Adding a Remote
If you're collaborating with others, you might need to link your local repository to a remote one. Use the following command to add a new remote:
git remote add origin https://github.com/yourusername/repo.git
This command establishes a connection between your local work and the specified remote repository.
Pushing Changes
Once your changes are ready to be shared, you can upload them to the remote with:
git push origin branch-name
This command enables you to publish your local updates and collaborate effectively.
Helpful Aliases
Creating Shortcuts for Long Commands
To make your Git workflow even more efficient, consider setting up aliases for frequently used commands. For example:
git config --global alias.co checkout
With this alias, you can switch branches using:
git co branch-name
Similar aliases can be set for other commands, such as:
git config --global alias.ci commit
This lets you commit changes using `git ci`, streamlining your interactions with Git.
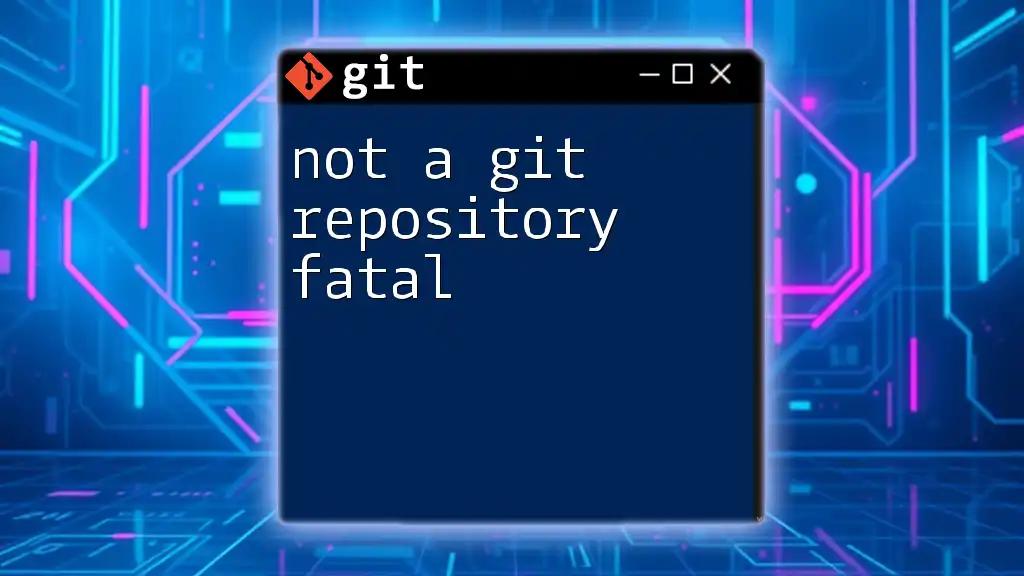
Conclusion
Mastering the most popular Git shortcuts can drastically enhance your efficiency as a developer. By incorporating these shortcuts into your everyday tasks, you will save time, reduce repetitive actions, and allow for a smoother collaborative process. Take the leap to practice these commands regularly, and soon they'll become second nature in your workflow. Remember, the key to mastering Git is continuous practice and exploration. Whether you're working on a personal project or collaborating with a team, these shortcuts will empower you to navigate your codebase with confidence and ease.