Git branching allows developers to create, manage, and switch between multiple lines of development in a repository, enabling efficient collaboration and experimentation without affecting the main codebase.
Here's a basic example of creating a new branch and switching to it:
git checkout -b new-feature-branch
Understanding Git Branching
What is Branching?
Branching in Git is a powerful feature that allows developers to diverge from the main line of development, create independent paths, and experiment with their code. When you create a branch, you are effectively creating a copy of your code at that moment in time, which you can freely modify without affecting your main codebase. This is essential for collaborative development, enabling multiple developers to work on different features or fixes simultaneously without stepping on each other’s toes.
Default Branches in Git
When you first initialize a Git repository, you typically have a default branch, often called `main` or `master`. This branch serves as the primary line of development where stable code resides. Understanding the role of the default branch is crucial, as it often represents the production-ready state of your project. In many workflows, developers will create separate branches for new features, bugfixes, or experiments, later merging those changes back into the default branch when they are complete.
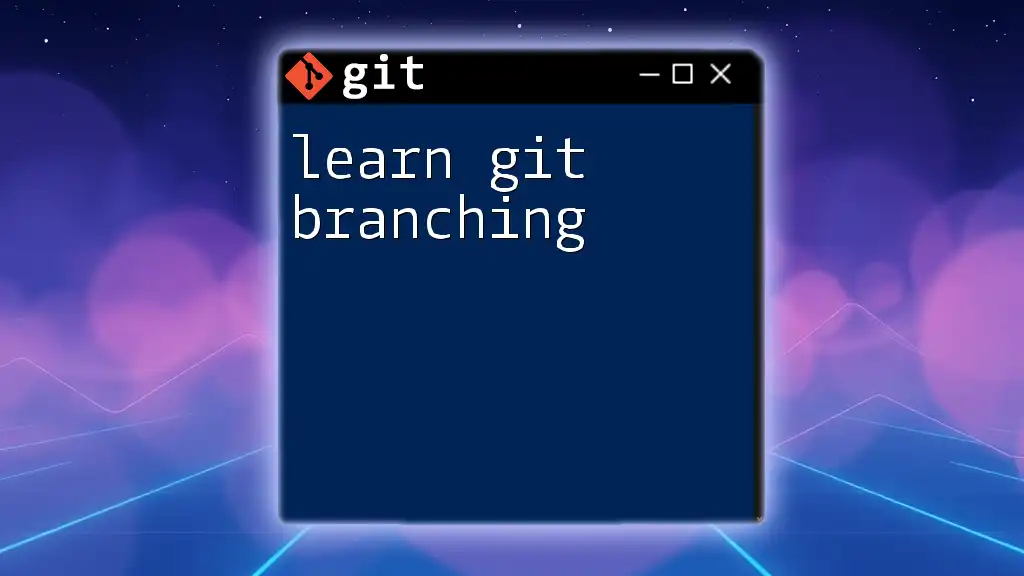
Creating and Managing Branches
Creating a New Branch
Creating a branch in Git is straightforward. You can do this by using the following command:
git branch <branch-name>
For example, if you're adding a new login feature to your application, you might run:
git branch feature/login
This command creates a new branch named `feature/login`. It’s an essential step that enables you to isolate your work.
Switching Between Branches
Switching between branches allows you to move to a different line of development. You can use either of the following commands to switch:
git checkout <branch-name>
or the more recent command that simplifies this task:
git switch <branch-name>
For example, if you want to work on the login feature you just created, you would run:
git switch feature/login
This command changes your working directory to reflect the state of the `feature/login` branch, enabling you to make changes without affecting other branches.
Listing Branches
To see a list of all your current branches, run:
git branch
This command will display all local branches, indicating which one you currently have checked out. If you want to see remote branches as well, you can use:
git branch -a
Understanding the difference between local and remote branches is crucial, as it helps you manage your workflow effectively and keeps your project organized.
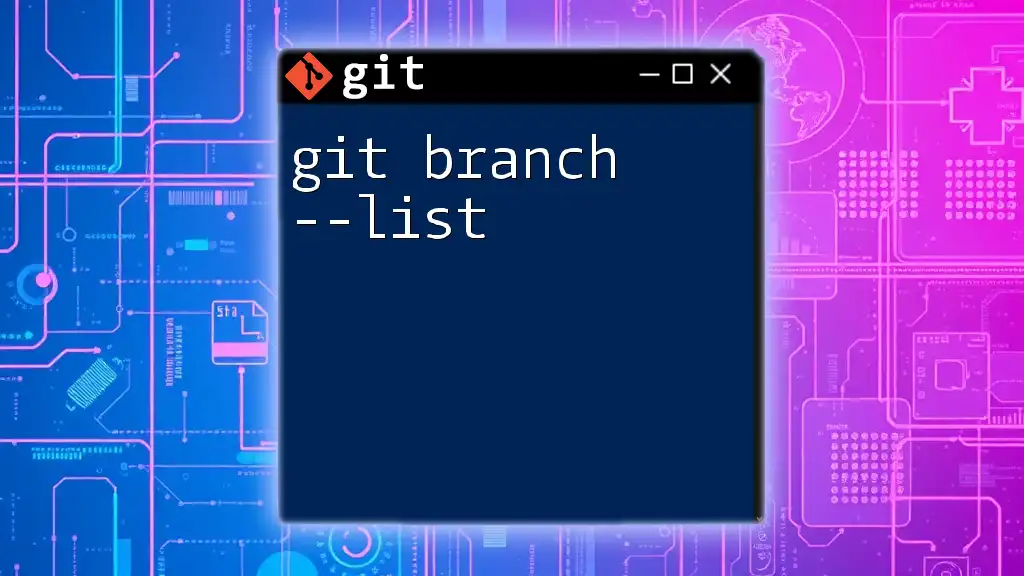
Working with Branches
Making Changes in a Branch
Once you are in a specific branch, you can start making changes. The process typically involves:
- Checking out the branch you want to work on.
- Making changes to your code or documents.
- Staging those changes for commit.
- Committing the changes with a descriptive message.
For example, if you have updated your login functionality, your commands would look like this:
git checkout feature/login
# Make changes to files
git add .
git commit -m "Implement login feature"
This workflow keeps your main branch clean and allows you to develop features independently.
Merging Branches
What is Merging?
Merging is the process of combining changes from one branch into another. When you’re finished with your work on a feature branch, you can merge these changes into the main branch, ensuring that your new feature is included in the primary codebase.
Fast-forward and Three-way Merge
There are two main types of merges you might encounter: fast-forward merges and three-way merges.
In a fast-forward merge, the `main` branch has not diverged, and Git simply moves the pointer of the `main` branch forward to the tip of the feature branch. You would execute a merge like this:
git checkout main
git merge feature/login
On the other hand, a three-way merge happens when the branches have diverged and there are conflicting changes. To ensure all merge aspects are represented, Git uses the common ancestor of the two branches to facilitate the merge. You can execute this kind of merge using:
git merge --no-ff feature/login
Visualizing these merge types can make it easier to understand how Git manages changes over time.
Resolving Merge Conflicts
Merge conflicts occur when two branches have competing changes that Git cannot automatically resolve. This is a common scenario when multiple collaborators are working on the same parts of the code.
When a merge conflict arises, Git will mark the areas of conflict in the affected files with conflict markers:
<<<<<<< HEAD
Current changes
=======
Incoming changes
>>>>>>> branch-name
To resolve these conflicts:
- Open the conflicted file in your text editor.
- Check for the `<<<<<<<`, `=======`, and `>>>>>>>` markers, and edit the file to select the changes you want to keep.
- Once resolved, stage the file for commit:
git add <filename>
- Finally, complete the merge with:
git commit -m "Resolved merge conflicts"
Understanding how to handle merge conflicts is vital for maintaining version control in collaborative environments.
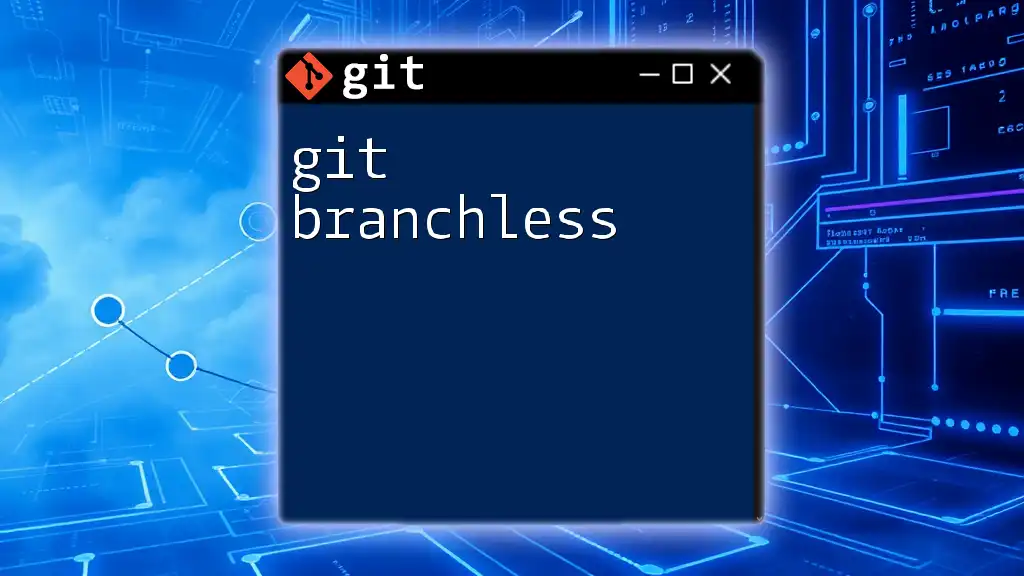
Best Practices for Git Branching
Branch Naming Conventions
Establishing a clear branch naming convention can significantly enhance your workflow. Good branch names describe the purpose of the branch clearly. For instance:
- Use `feature/` prefix for feature development, e.g., `feature/add-search`.
- Use `bugfix/` for bug fixes, e.g., `bugfix/fix-login-error`.
- Use `hotfix/` for urgent fixes in production, e.g., `hotfix/critical-bug`.
Avoid vague or overly generic names like `temp` or `test`, as these can lead to confusion. Clear names save time and improve collaboration.
Regular Merges and Cleanup
Regularly merging branches can prevent larger conflicts later. If you isolate changes in a branch and then wait extensively before merging, you may encounter significant discrepancies. It’s advisable to merge your feature branches back to the main line as soon as possible.
You can delete branches after they are no longer needed to maintain a clean project. Use:
git branch -d <branch-name> # Delete local branch
git push origin --delete <branch-name> # Delete remote branch
This keeps your repository uncluttered, making it easier to manage ongoing development.
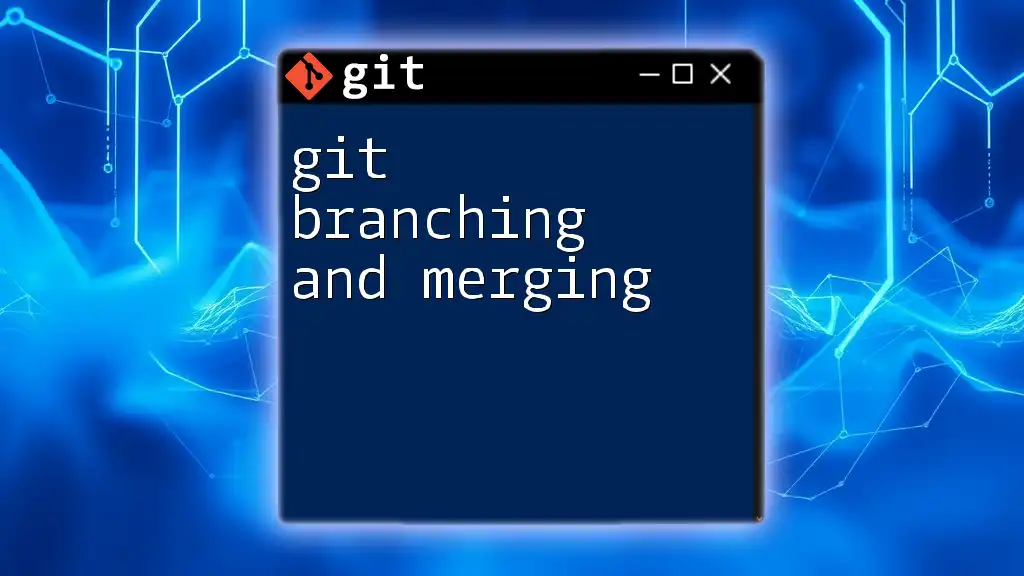
Advanced Branching Solutions
Using Feature Branches
Feature branches allow developers to create isolated environments for feature development. Each feature can evolve independently without the risk of unstable code contaminating the main branch. Once the feature is finished, it can be reviewed and tested before merging back into the main branch.
Git Flow Workflow
Git Flow is a branching model that defines a standard for managing branches in a collaborative environment. It includes different types of branches:
- Feature Branches for developing new functionality.
- Develop Branch where all the feature branches merge.
- Release Branches for preparing new production versions.
- Hotfix Branches for urgent fixes in production.
This structured approach enables teams to have a clear process and reduces chaos in large-scale projects.
Release Branches
Release branches are specifically used to prepare for a new release. They are created from the develop branch and allow for final adjustments, bug fixes, and testing before a deployment:
git checkout develop
git checkout -b release/v1.0
# Make final adjustments, commits, and test the release
After completion, you would merge release branches into the main branch to publish the new version, ensuring all changes are recorded.
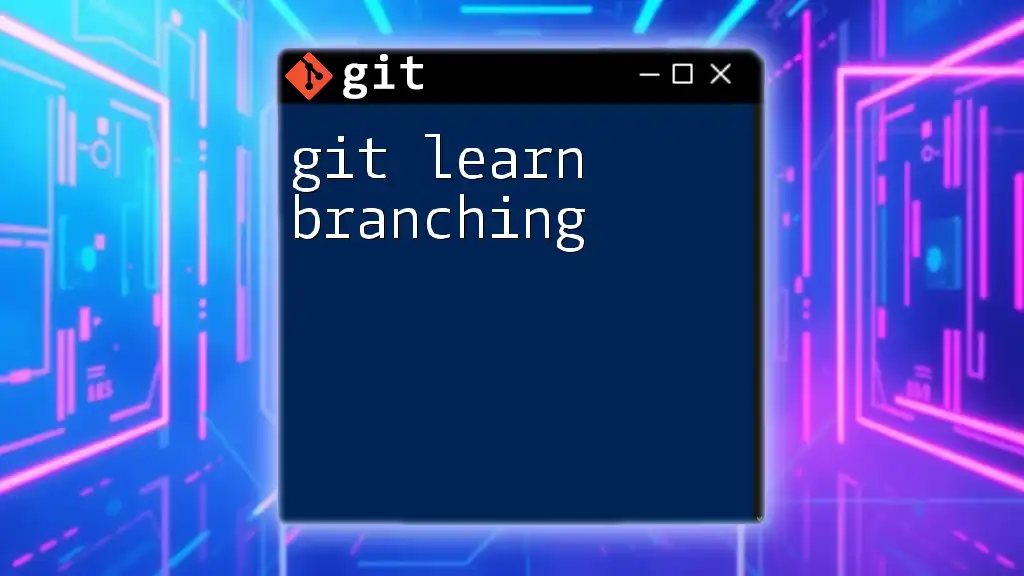
Conclusion
Learning Git branching solutions is an essential aspect of becoming proficient in version control. Mastering branching allows developers to work collaboratively, maintain a clean and organized repository, and manage code changes more effectively. Practice these commands and strategies often, as familiarity with these workflows will greatly enhance your development efficiency.
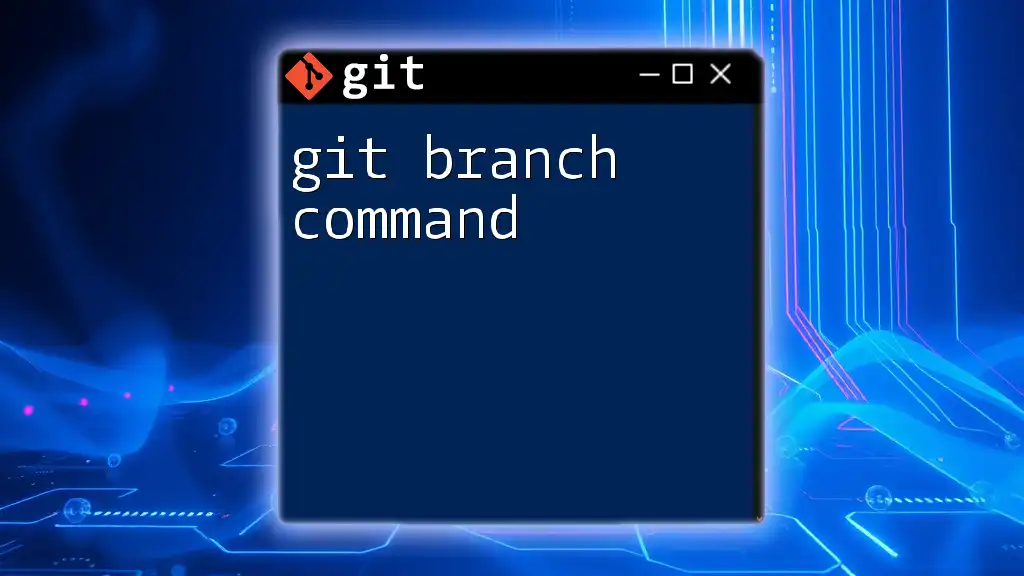
Additional Resources
To deepen your understanding of Git branching, refer to the official Git documentation, online tutorials, and branching best practices articles. Engaging with these resources will solidify your skills and prepare you for more advanced topics.
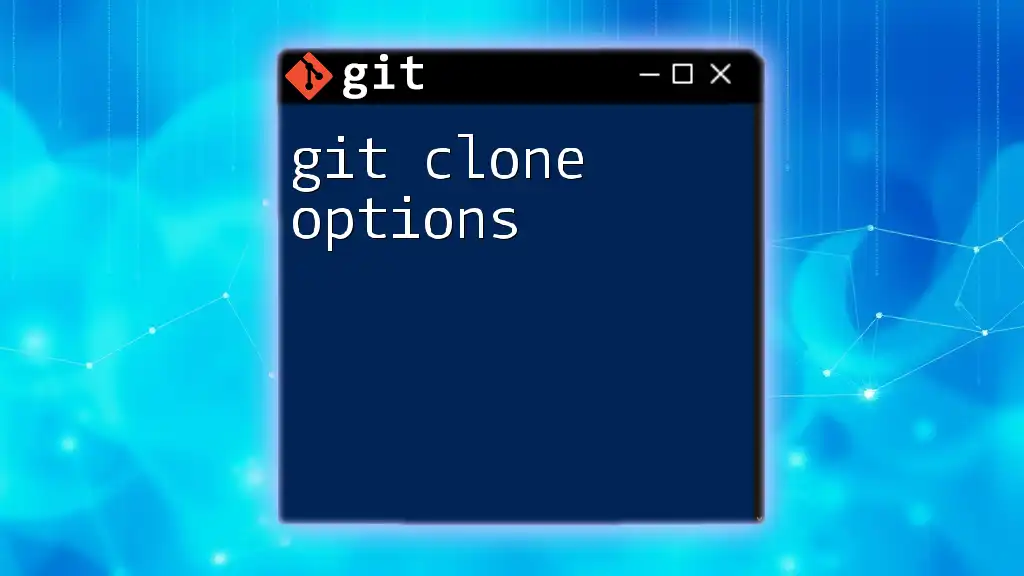
FAQs
Common Questions about Git Branching
-
What should I do if I accidentally deleted a branch? You can often recover the branch using the reflog. Run `git reflog` to find the commit reference, then create a new branch from that commit.
-
How can I undo a merge? You can undo a merge by using `git reset --hard HEAD` before any commits are made. If you have already committed, use `git revert` to create a new commit that undoes the changes.
By grasping these concepts and the scenarios presented, you can navigate Git branching more confidently and contribute effectively to team projects.