In Git, the line endings of text files can be managed with the `core.autocrlf` setting, where `lf` (line feed) will be replaced by `crlf` (carriage return + line feed) for compatibility with Windows systems when files are checked out.
git config --global core.autocrlf true
Understanding Line Endings
What are Line Endings?
Line endings are essential components in text files that signify where one line ends, and another begins. The two most common types of line endings are LF (Line Feed) and CRLF (Carriage Return + Line Feed).
- LF is represented by the character `\n` and is primarily used in Unix-based systems such as Linux and macOS.
- CRLF is denoted by the characters `\r\n` and is the default line ending for Windows systems.
Understanding the differences between LF and CRLF is crucial, especially when collaborating across different operating systems. Visualizing these can help clarify how they manifest in text editors and code files, leading to a smoother development experience.
Why Does it Matter?
The role of line endings goes beyond mere aesthetics in your code. They have significant implications for code compatibility and can result in frustrating bugs when they are inconsistent:
- Cross-Platform Compatibility: If a project is developed on multiple platforms, the line ending format could vary, leading to confusion and errors in the source code.
- Merge Conflicts: Inconsistent line endings can result in unnecessary merge conflicts that complicate the development process.
For instance, consider a scenario where a developer based on Linux (using LF) collaborates with another developer on Windows (using CRLF). If they both edit the same file without regard to their line-ending settings, they may encounter unnecessary conflicts when trying to merge their changes.
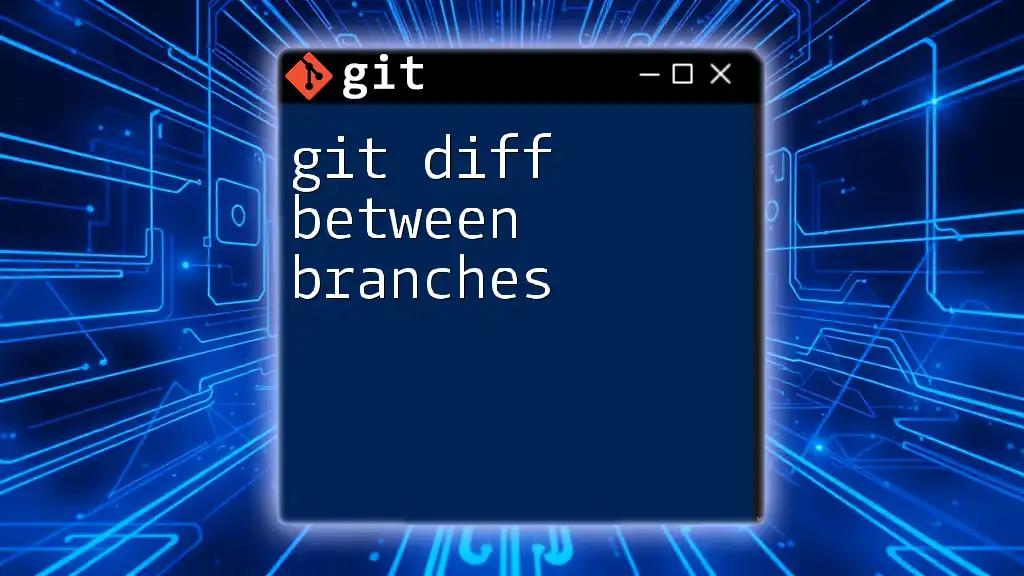
Git's Handling of Line Endings
Default Behavior of Git
By default, Git recognizes line endings and uses the system's default line ending style. However, this may lead to complications when contributors work in different environments. This is where Git's `.gitattributes` file becomes relevant; it allows developers to specify how Git should handle line endings for specific files.
For example:
# In .gitattributes
*.txt text
*.sh text eol=lf
*.bat text eol=crlf
In this snippet, the `.gitattributes` file specifies that text files should always end with LF, while Windows batch files should use CRLF. Such configurations help maintain consistency across a project's codebase.
Configuring Git to Manage Line Endings
You can control how Git handles line endings using the `core.autocrlf` configuration option. This setting can be adjusted based on your development environment:
- Setting `core.autocrlf` to `true`: This setting will convert LF to CRLF when checking files out of the repository on Windows systems.
- Setting `core.autocrlf` to `false`: This prevents any conversion, which is useful for projects where line endings need to remain consistent.
- Setting `core.autocrlf` to `input`: This will convert CRLF to LF when checking files into the repository, but it won't modify line endings on checkout.
To set the global configuration to automatically convert line endings, you can run:
git config --global core.autocrlf true
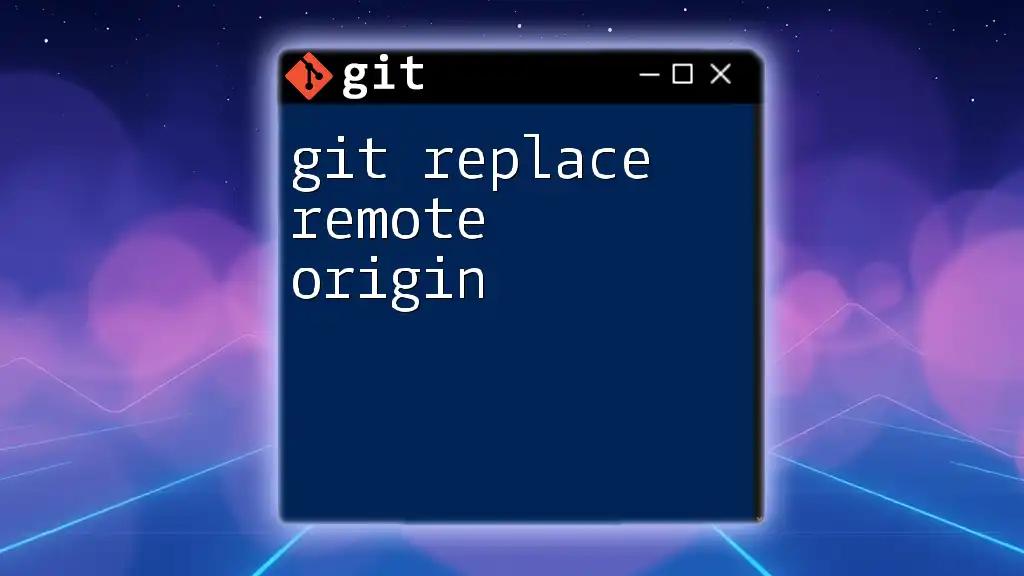
Transition from LF to CRLF
Why the Change?
The transition from LF to CRLF is driven by the need for consistency in collaborative environments. As more teams operate on diverse operating systems, ensuring a uniform line ending style can simplify code reviews and reduce merge conflicts. This change allows developers to avoid the common pitfall of accidental line-ending changes disrupting shared projects.
How the Change Affects Existing Repositories
When transitioning existing projects, there are several considerations to keep in mind. The most prominent issue is the potential for existing files with inconsistent line endings, which could result in confusion and errors.
Before making changes, it is advisable to:
-
Backup Your Repository: Always create a backup of your repository before making any significant changes, particularly if you have multiple contributors.
-
Update the .gitattributes File: Ensure you have a `.gitattributes` file set up that defines how you want Git to handle different file types. An example might look like this:
* text=auto
-
Normalize Line Endings Across All Files: After updating the `.gitattributes` file, run the following command to apply changes to the existing files:
git add --renormalize .
This command helps re-check and apply the line-ending rules specified in your `.gitattributes` file.
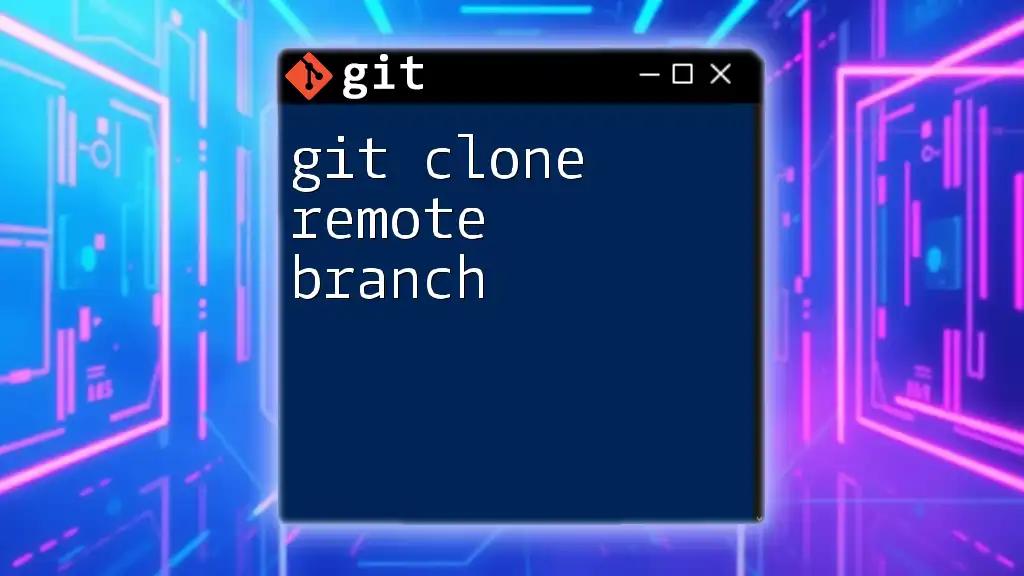
Common Scenarios and Solutions
Dealing with Merge Conflicts
Merge conflicts can occur if two developers edit the same file with different line ending settings. To resolve these conflicts, you can perform the following steps:
- Check for Differing Line Endings: Use tools like `git diff` to identify the files with conflicting line endings.
- Manually Adjust Line Endings: Open the conflicting files in an editor that allows you to choose the line-ending style, or use command-line tools to convert the endings manually.
- Recommit Changes: After resolving conflicts, stage the changes and recommit them.
Collaborating with Cross-Platform Teams
When working in cross-platform environments, consistent line endings can be maintained through best practices that include:
-
Consistent Configuration: Ensure that every team member has set their `core.autocrlf` appropriately for their operating system.
-
Use of .gitattributes: Proper configuration of the `.gitattributes` file can minimize discrepancies; this ensures everyone follows the same rules for line endings.
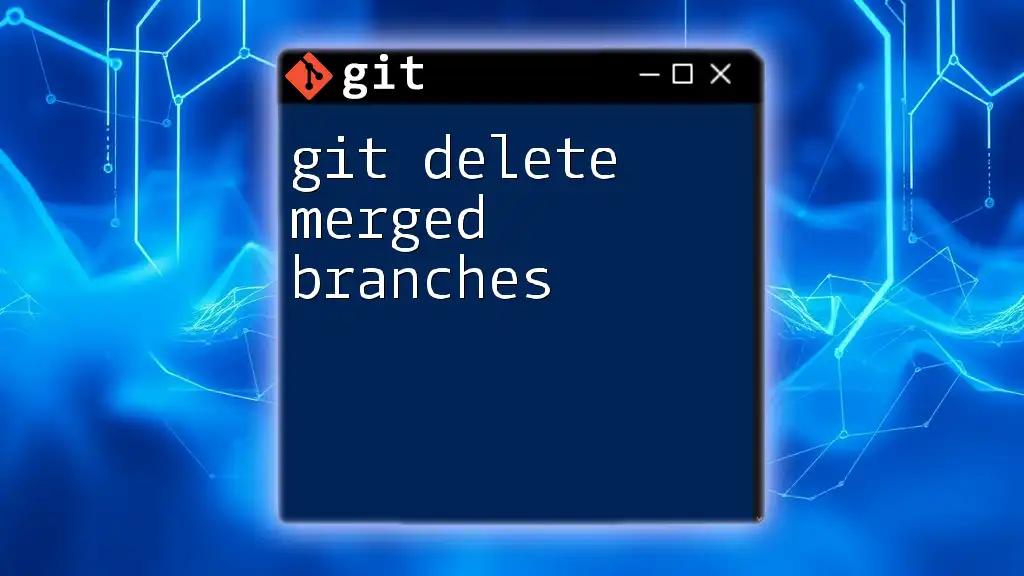
Conclusion
In summary, understanding that Git LF will be replaced by CRLF is vital for ensuring smooth collaboration across diverse environments. By recognizing the significance of line endings, configuring Git appropriately, and maintaining best practices, teams can prevent common pitfalls. As you continue your Git journey, consider adapting to this change for more seamless development efforts. Exploring additional resources or workshops can further enhance your team's efficiency and effectiveness in using Git.