To include only one directory in a Git archive, you can use the following command, which enables you to create a compressed tarball of a specific directory within your repository:
git archive --format=tar.gz --output=directory.tar.gz HEAD:path/to/your/directory
Understanding Git's Directory Structure
What is a Git Repository?
A Git repository is a structure utilized by Git to manage the collections of files related to a specific project. It stores all of your project files along with their version history, allowing you to track changes, revert to previous states, and collaborate with others. A well-organized repository helps maintain clarity about your project’s evolution over time.
The Role of Directories in Git
Directories are essential in Git as they structure how files are organized and tracked. Git operates by detecting changes in the files and these changes are grouped per directory. This segmentation allows for easier management, especially when working on larger projects that may consist of multiple components.
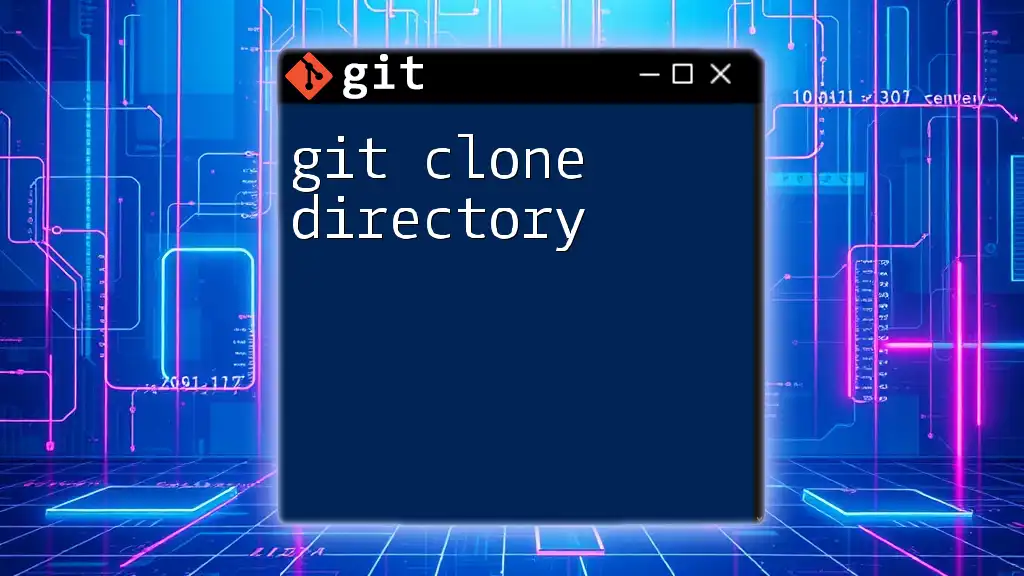
The Need to Include Only One Directory in Commits
Why Commit Selectively?
Committing selectively is crucial in maintaining a clean and understandable commit history. By focusing on one directory at a time, you can isolate changes, making it easier to review what was altered and why. This practice not only keeps your commit logs tidy but also aids collaboration with your team, as others can focus on specific features or fixes without diving through unrelated changes.
Common Scenarios
Situations where including only one directory makes sense include:
- Feature Development: You may have a directory dedicated to developing a feature. By isolating commits to just this directory, it’s easier to track progress separate from other areas of your project.
- Bug Fixes: Applying a bug fix that pertains to a specific module or directory allows for cleaner history management, ensuring that your bug resolution is well-defined and doesn’t interfere with unrelated code changes.
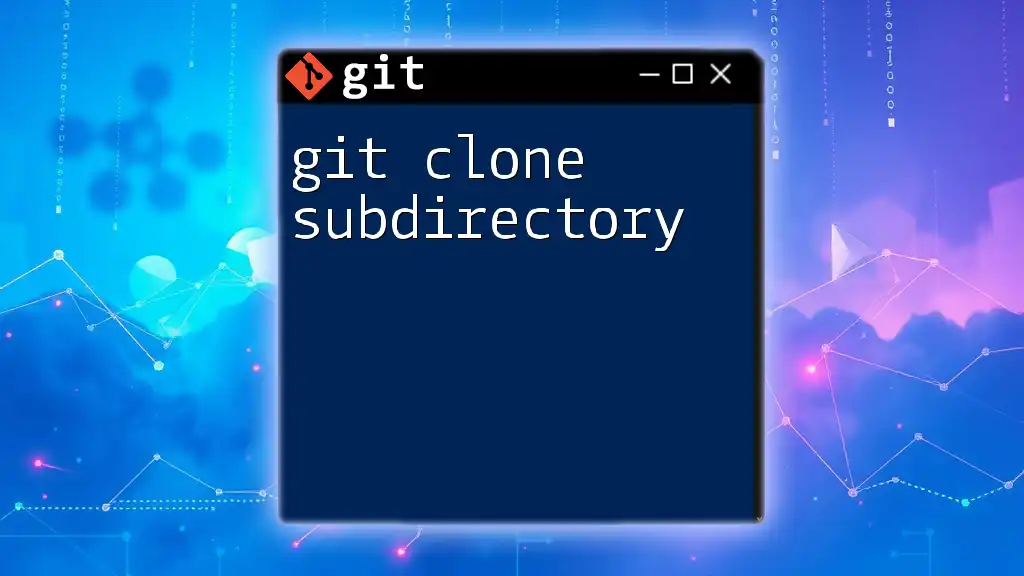
Using Git to Include Only One Directory
The Basics of the `git add` Command
The `git add` command puts changes from your working directory into the staging area. This is the first step before committing those changes. Understanding how to stage files and directories is vital for ensuring that only relevant changes are included in your commit, allowing for cleaner version history.
Staging a Single Directory
To stage an entire directory for committing, use the following syntax:
git add <directory_name>
For example, if you want to stage all the changes within a `src` directory, you would execute:
git add src/
Executing this command stages all changes made in the `src/` directory, while other files/non-staged directories remain untouched.
Excluding Other Directories and Files
To keep your commits clean, you can employ a `.gitignore` file to specify which directories or files Git should ignore during staging. This is particularly useful for excluding build files or temporary configuration files that are not essential to your project's source control.
Committing Your Changes
Once you’ve staged your desired directory, it’s time to commit your changes. You can do this with the following command:
git commit -m "Add new features in the src directory"
For example, when updating UI components in your `src` folder, a suitable commit message could be:
git commit -m "Update UI components in src folder"
This signals to others what changes were made and in which section of the project.
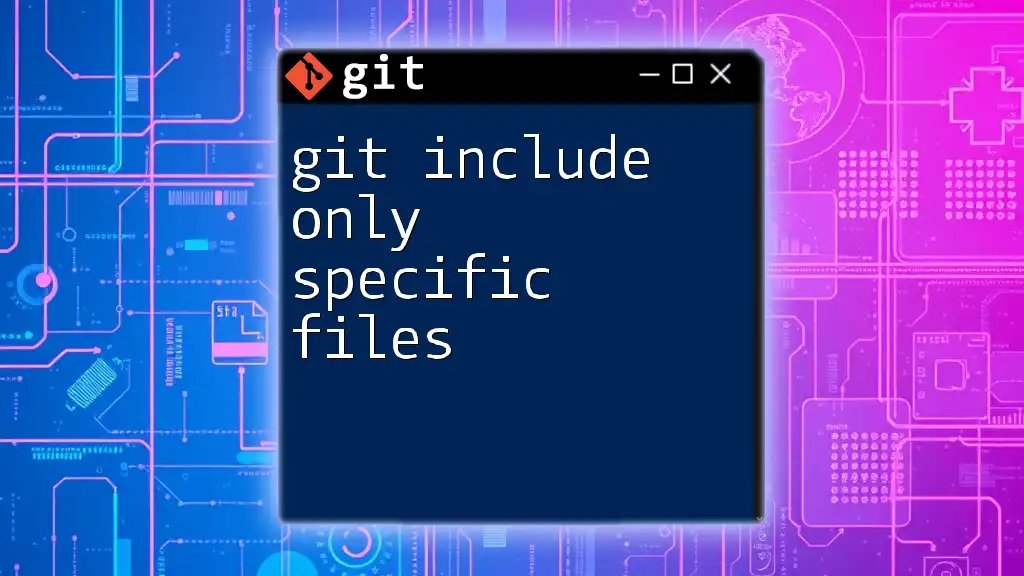
Advanced Techniques: Including Specific Files within a Directory
Staging Selected Files in a Directory
If you prefer to stage specific files instead of the entire directory, you can do so using the command:
git add <directory_name>/<file_name>
For instance, if you only want to stage one file within the `src` directory, you would enter:
git add src/index.js
This method is particularly useful when you have multiple changes in a directory but only want to commit a few of them.
Using Interactive Staging
Git offers an interactive staging feature with the `git add -p` command, allowing you to selectively choose changes to stage. This command breaks down changes into smaller 'hunks' and prompts you to decide whether to stage each change. Here’s how you can initiate it:
git add -p
During this process, Git will present you with each hunk and options to stage it or not. This helps ensure that only the necessary changes are included in your commit, fostering accountability and precision in your version control.
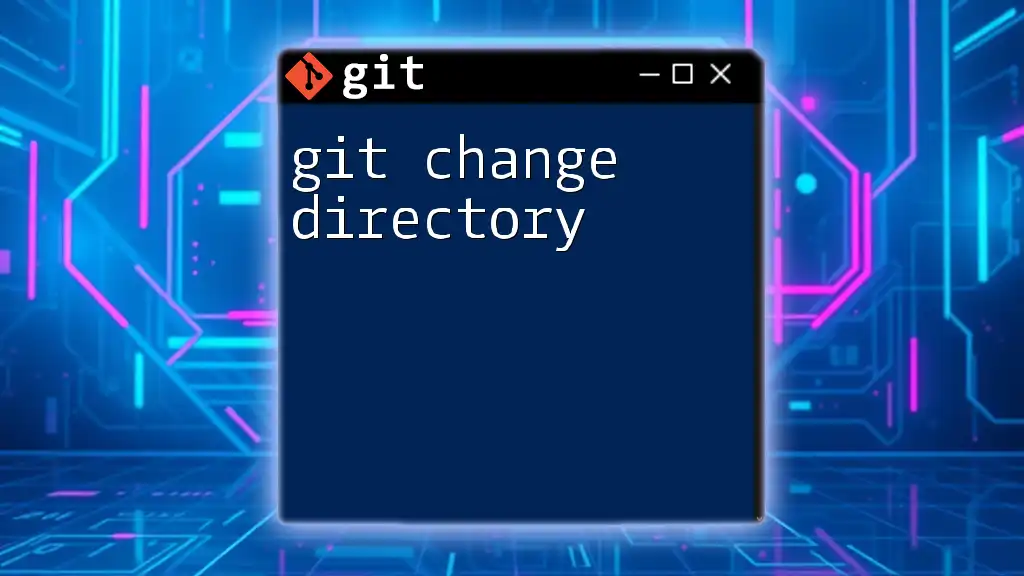
Best Practices for Committing Changes in a Single Directory
Commit Messages
Utilizing clear, descriptive commit messages greatly enhances collaboration and project tracking. When focusing on a single directory, your messages should detail:
- What was changed? This provides context for reviewers.
- Why it matters? Explaining the importance of changes helps other collaborators understand the necessity of the update.
Structuring Your Repository
To maximize your use of Git and facilitate future maintainability, spend time thoughtfully structuring your repository. Consider grouping related functionalities into dedicated directories. This not only enhances clarity but allows others (and your future self) to navigate the project more easily.
Reviewing Changes Before Committing
Before making a commit, reviewing your changes is a prudent step. Use the `git diff` command to examine differences between your current changes and the last commit:
git diff <directory_name>
This practice can prevent mistakes, ensuring that you only include the intended changes in your committed directory.
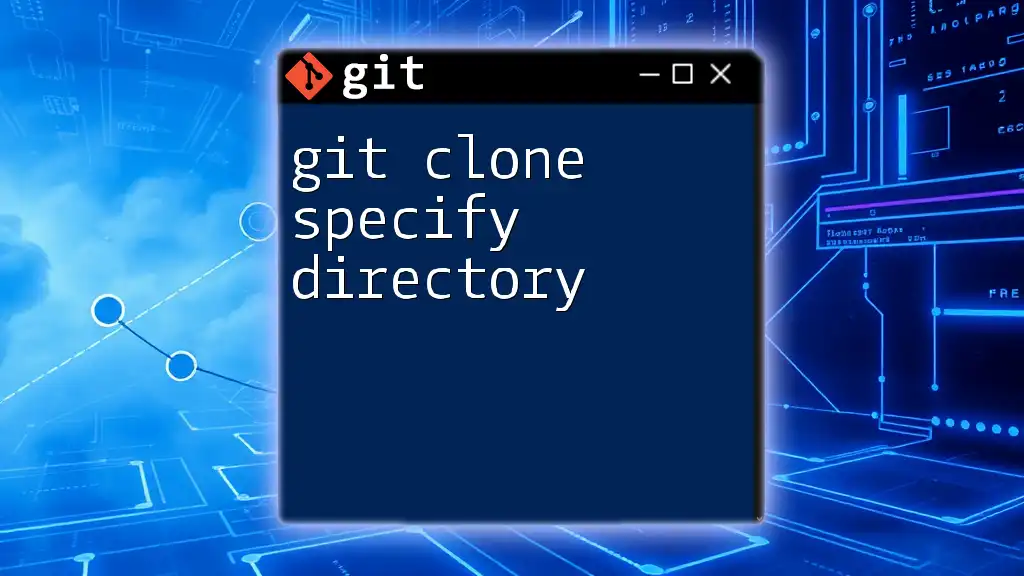
Conclusion
Summary of Key Points
Including only one directory in your commits is a powerful method for keeping your project’s history clean and manageable. Leveraging Git's capabilities effectively enables you to track progress and changes with clarity.
Final Thoughts
Encouragement to practice these selective commit strategies consistently will yield significant benefits in project management and collaboration. Strive to explore further Git functionalities to enhance your skills and workflow efficiency over time.
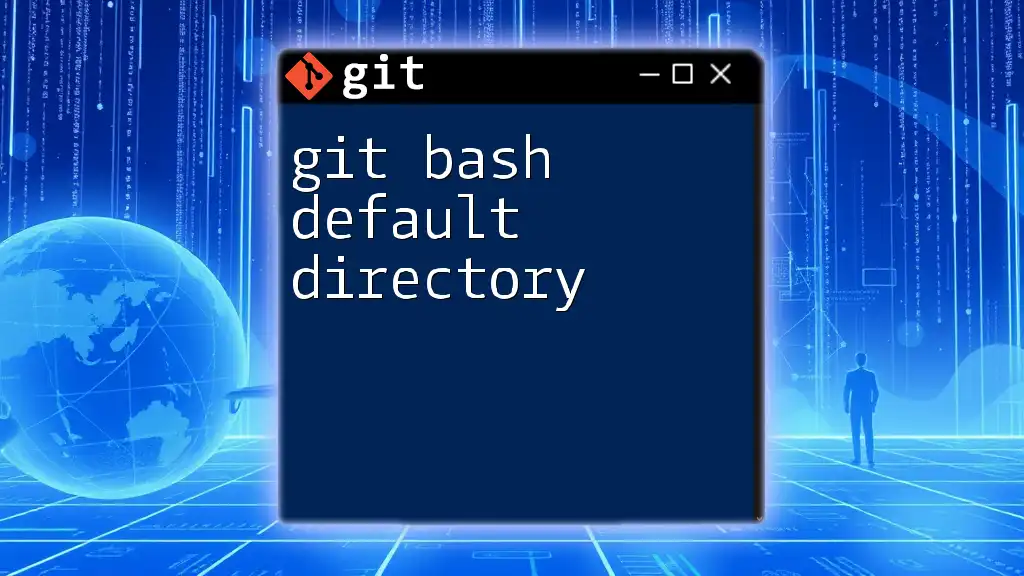
Additional Resources
For those looking to deepen their understanding, many excellent resources and tutorials on Git are available online, enabling you to become proficient in this essential tool. Consider diving into the official Git documentation to explore all the powerful features Git has to offer.