A git fork creates a personal copy of someone else's repository on GitHub for independent development, while a branch allows you to diverge from the main codebase within your own repository, enabling collaboration on features or fixes.
# To create a new branch in your local repository and switch to it
git checkout -b new-feature
# To fork a repository on GitHub, simply visit the repo page and click the "Fork" button.
Understanding Git Terminology
What is Git?
Git is a powerful distributed version control system that allows multiple developers to work on a project simultaneously without interfering with each other's changes. Its robust features facilitate collaboration, making it the go-to tool for software development. By tracking changes in code over time, Git enables developers to maintain history and manage different versions of their projects efficiently.
Key Terminology Related to Forks and Branches
Before diving into details about the differences between a git fork and a branch, it's essential to grasp some core Git terminology.
- Repository: A storage space for your project, which includes all files, history, and branches.
- Commit: A snapshot of changes made to the project files, allowing you to track modifications over time.
- Merge: Integrating changes from one branch or fork into another.
- Clone: Creating a local copy of a remote Git repository for your development needs.
Understanding these terms lays the groundwork for discussing how forks and branches operate within the Git ecosystem.

Forks and Branches: An Overview
What is a Git Branch?
A Git branch is essentially a separate line of development in your repository. It allows you to work on features, bug fixes, or experiments isolated from the main codebase, typically called the `main` or `master` branch. This isolation encourages experimentation without the risk of destabilizing your main project.
Use cases for branches include:
- Feature development: When building a new feature, you can create a branch specifically for that feature. This keeps the main codebase clean until the feature is fully developed and tested.
- Bug fixes: Similarly, when addressing bugs, a dedicated branch allows for validation and correction without affecting the overall stability.
- Experimenting with new ideas: Branches provide a safe space to try new implementation approaches or ideas without any constraints.
Creating a branch is as simple as running the following command:
git checkout -b new-feature
This command creates and switches to a new branch named `new-feature`.
What is a Git Fork?
A fork is a complete copy of a repository that lives under your GitHub account. Forking a repository allows you to freely experiment with changes without affecting the original project. Once you've made changes in your fork, you can propose integrating those changes back into the original repository via a Pull Request.
Use cases for forks include:
- Working on open-source projects: Forks are commonly used to contribute to projects you do not own. You can experiment and make improvements or bug fixes in your version before suggesting them back to the original project.
- Maintaining personal copies of repositories: If you want to customize a repository or maintain your version for personal use, forking is an excellent option.
Overall, forking enables collaborative efforts, particularly in open-source communities.
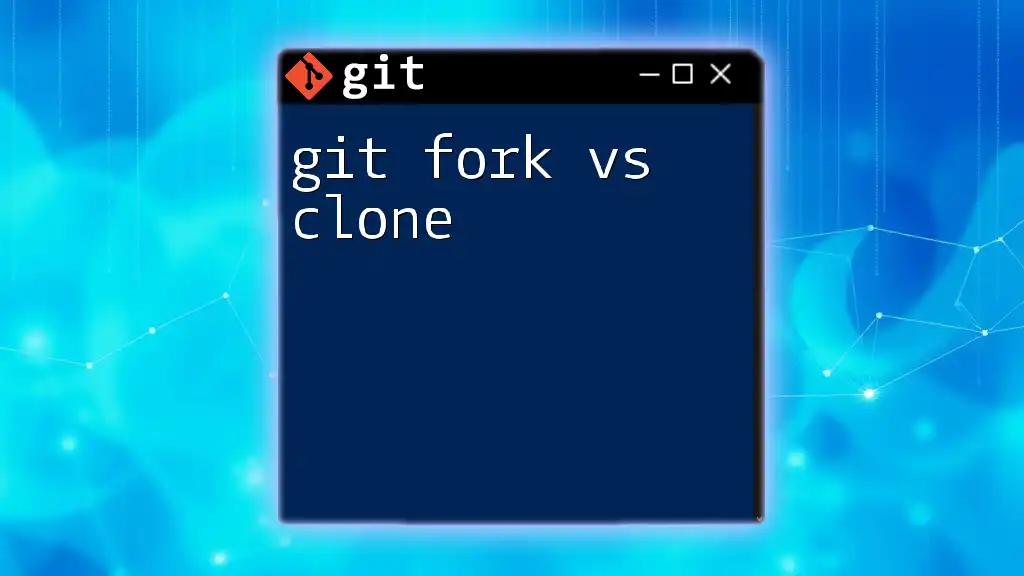
Differences Between Forks and Branches
Conceptual Differences
At a high level, forks and branches serve different purposes within the Git framework:
- A branch exists within a single repository. It's mostly utilized for development within the same project, allowing developers to collaborate closely by keeping all changes in one shared space.
- A fork, on the other hand, is entirely independent and creates a separate copy of a repository under a different user account. It enables collaboration without granting direct access to the original repository, fostering an ecosystem of contributions.
Technical Differences
When it comes to how you manage and work with these constructs:
- Forks are stored on platforms like GitHub and require you to manage them as separate projects. Each fork can have pull requests made to integrate changes back into the parent repository.
- Branches are manipulated via your local repository, making merging and updating processes more seamless as developers can push changes back to the original repository directly.
Merge strategies
- Merging branches often involves straightforward Git commands since they originate from the same repository.
git merge new-feature
- Conversely, incorporating changes from a fork often requires a Pull Request process, leading to a review before the merge can happen.
Usage Scenarios
To determine when to use a branch versus a fork, consider the specific context of your development needs:
-
Use a branch when:
- You are developing features or fixing bugs within the same project you own.
- You aim for rapid changes followed by a review before merging them back into the `main` branch.
-
Use a fork when:
- You want to contribute to another developer’s open-source project.
- You wish for a personal version of a repository that you can manage independently.
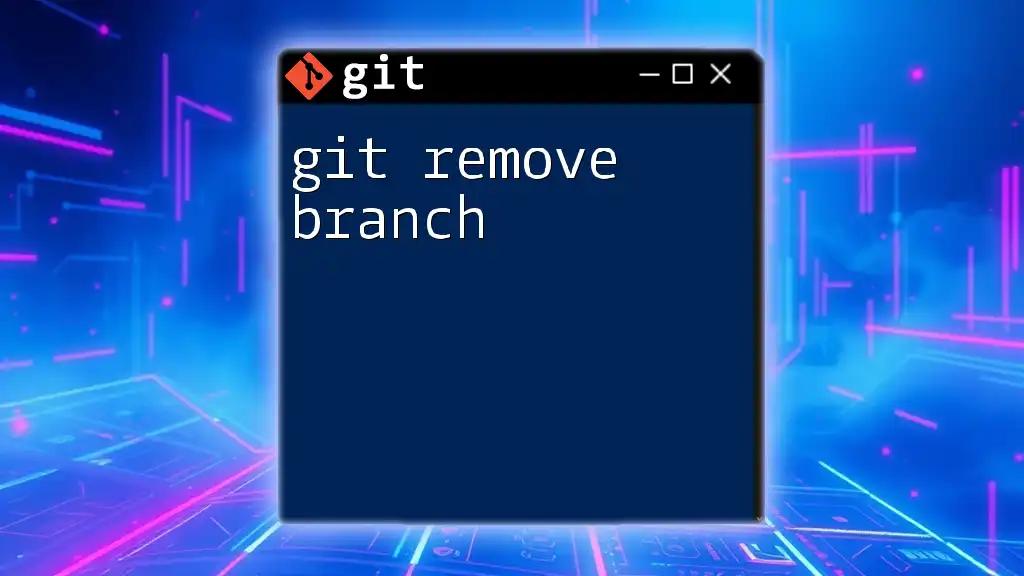
Best Practices for Using Forks and Branches
Managing Branches
To ensure a smooth workflow with branches, consider these best practices:
- Naming conventions: Choose descriptive and concise names for branches, such as `feature/update-user-profile` or `bugfix/fix-login-error`.
- Keep branches up to date: Regularly merge changes from the main branch into your feature branches to avoid large merge conflicts later.
To update a branch with the latest changes from the main branch, you can run:
git fetch origin
git merge origin/main
Managing Forks
When working with forks, it is crucial to maintain synchronization with the original repository. Here are some best practices:
- Sync your fork: Regularly pull in changes from the original repository to keep your fork updated.
- Add the original repository as an upstream remote:
git remote add upstream https://github.com/original-owner/repo.git
- Then to fetch and merge:
git fetch upstream
git merge upstream/main
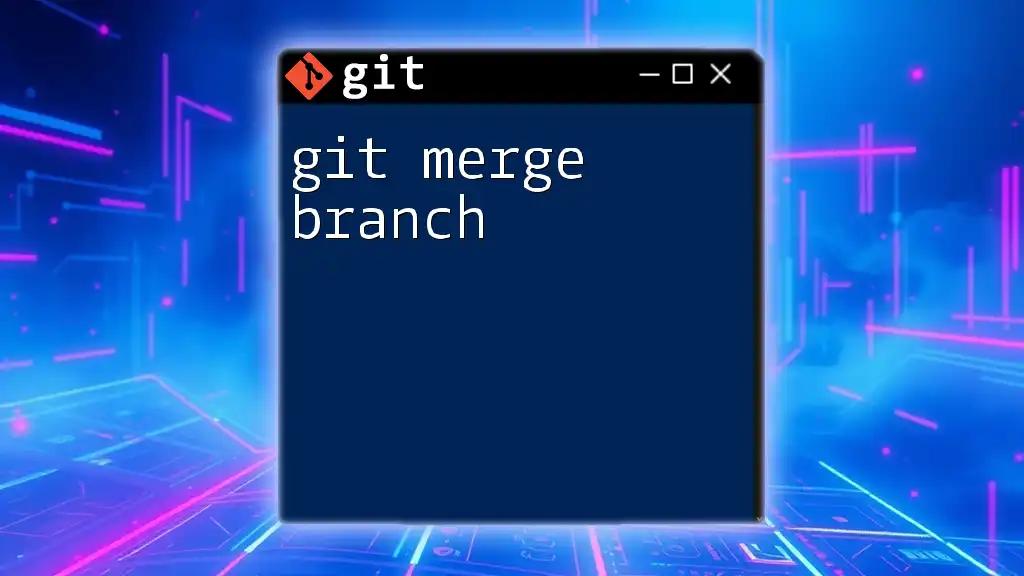
Common Workflows
Branching Workflow
When a team collaborates on a project, following a structured branching workflow is essential. Here's how such a workflow might look:
- Create a new branch for the feature you are developing.
git checkout -b feature-1
- Make code changes and then commit them.
git commit -m "Add new feature"
- Switch back to the main branch and merge the feature branch.
git checkout main git merge feature-1
This method keeps the main branch clean and allows for thorough testing of new features.
Forking Workflow
Contributing to an open-source project using a fork involves several steps:
- Fork the repository from GitHub. This creates a copy in your account.
- Clone your fork to have the repository locally.
git clone https://github.com/your-user/repo.git
- Make your changes, commit them, and push the updates to your fork.
git push origin your-feature-branch
- Navigate to the original repository to submit a Pull Request with your proposed changes.
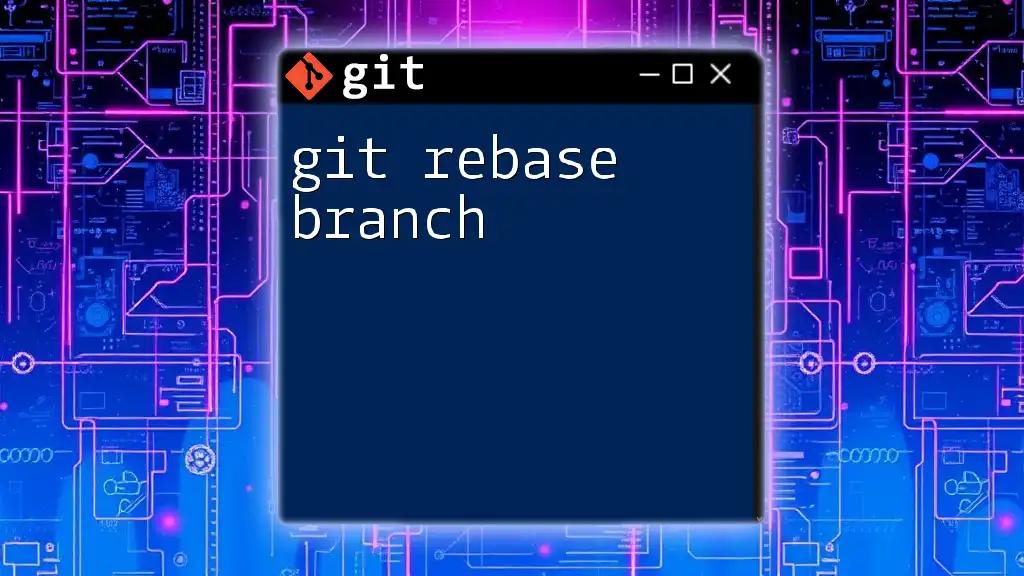
Conclusion
Understanding the distinction between a git fork and a branch is essential for effective collaboration in software development. Each serves specific purposes based on project requirements and collaborative contexts. By applying the best practices and workflows discussed, you can enhance your usage of Git in both personal and team projects. Experimenting with both forks and branches will deepen your understanding, making you a more proficient Git user.

Additional Resources
Documentation and Tutorials
For further reading, explore the official Git documentation, which provides detailed guidance on version control concepts. Books on Git and online courses can also bolster your skills.
Community and Support
Engage with communities such as Stack Overflow, GitHub forums, or even local meetups dedicated to Git. These platforms are filled with developers keen to share knowledge and assist with queries.
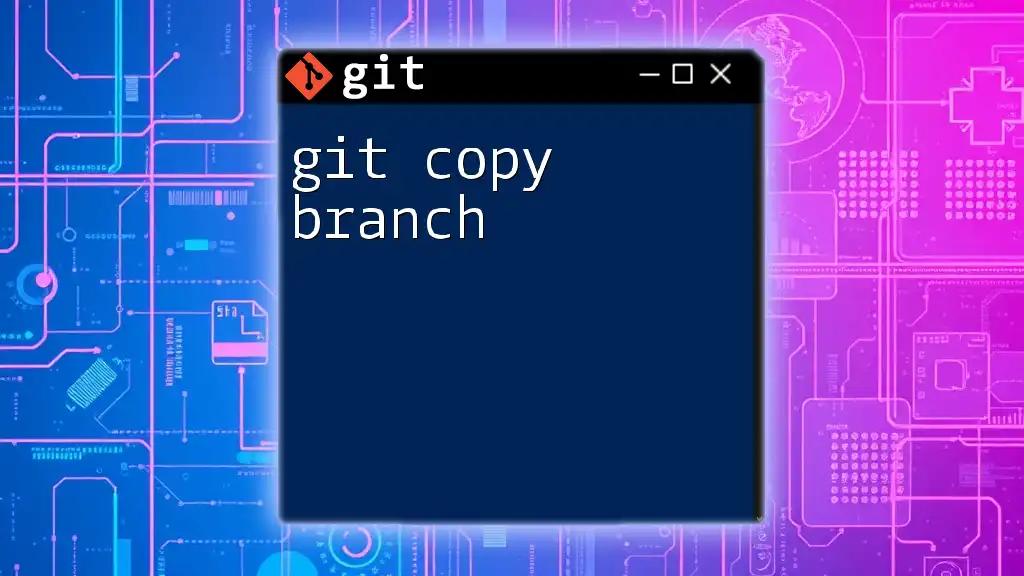
Call to Action
Stay tuned for more bite-sized learning material on Git commands and workflows. Don't forget to follow us on social media to keep your Git skills sharp and receive the latest updates!