Git tags are permanent references to specific commits that serve as version markers, while branches are mutable pointers that allow for ongoing development and experimentation on separate lines of work.
Here's a code snippet comparing the two:
# Create a new branch
git checkout -b new-feature-branch
# Create a tag for the current commit
git tag v1.0
Understanding Git Concepts
What are Git Branches?
A branch in Git serves as a pointer to a specific commit in the project’s history. Think of it as a diverging path where developers can work on features, fixes, or experiments without disrupting the main codebase. By creating branches, you can isolate changes until they are ready to be merged back into the main line of development (often referred to as the "main" or "master" branch).
Purpose of Branching
Branches enable parallel development workflows, allowing multiple teams to work on various features simultaneously without affecting each other. For instance, one team can be developing a new feature while another team is fixing bugs or refactoring existing code.
What are Git Tags?
A tag in Git is a marker for a specific commit in the repository's history, often used to denote important milestones such as releases or versions. Tags are notably different from branches, as they do not change or move; they are fixed instances of commits that highlight relevant points in the project's timeline.
Purpose of Tagging
The primary use of tags is to create a clear and accessible history of important events in your project. For example, if you release version 1.0 of your software, you can tag that commit to easily reference it later. Tags allow teams and developers to keep track of significant updates and identify specific versions of their code quickly.
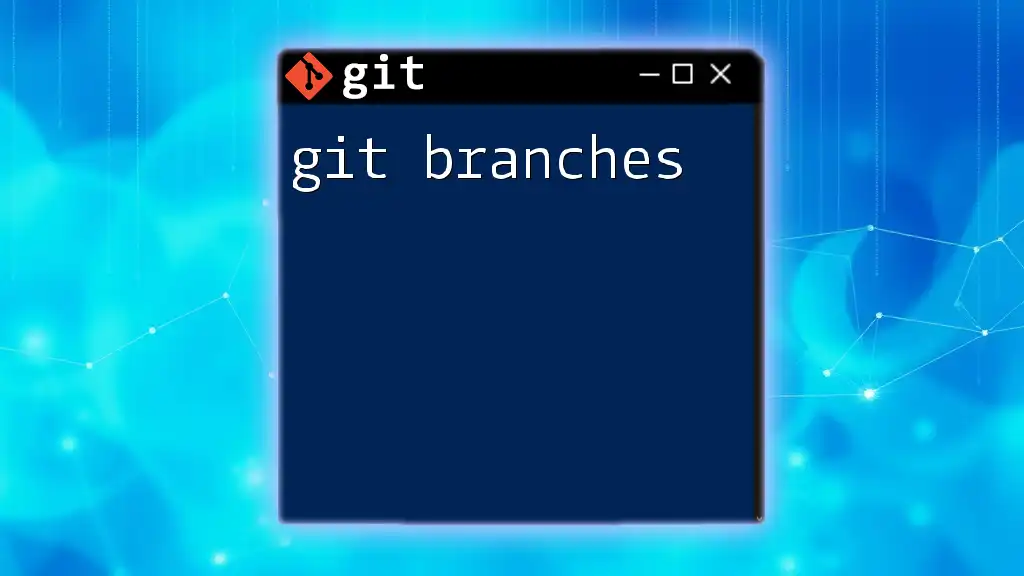
Differences Between Git Tags and Branches
Core Differences
When comparing git tags vs branches, the most significant distinction lies in their mutability:
- Mutability
- Branches are mutable, meaning they can change as development progresses. You can continue to commit new changes to a branch, making it a dynamic reference point.
- Tags, on the other hand, are immutable. Once you create a tag, it points to the same commit indefinitely. This immutability makes tags ideal for marking release points, as they will not inadvertently shift due to further changes in the codebase.
Use Cases
Understanding when to use branches versus tags is critical to leveraging the full potential of Git:
-
When to Use Branches
Branches are ideal for:- Feature development: Creating a new feature without risking the stability of the main codebase.
- Bug fixing: Isolating a defect fix until it is adequately tested.
- Experimentation: Trying out new ideas without affecting the ongoing development process.
-
When to Use Tags
Tags are best suited for:- Marking releases: Identifying significant versions of your software (e.g., v1.0, v2.0).
- Archiving: Keeping a record of past versions for future reference.
- Milestone documentation: Clearly marking checkpoint events in your project timeline.
Visualization
To better understand the relationship and structure of branches and tags, visualizing them can be incredibly helpful. Imagine a tree-like structure where each branch represents a line of development, and tags serve as markers along those branches, signifying crucial points like version releases.
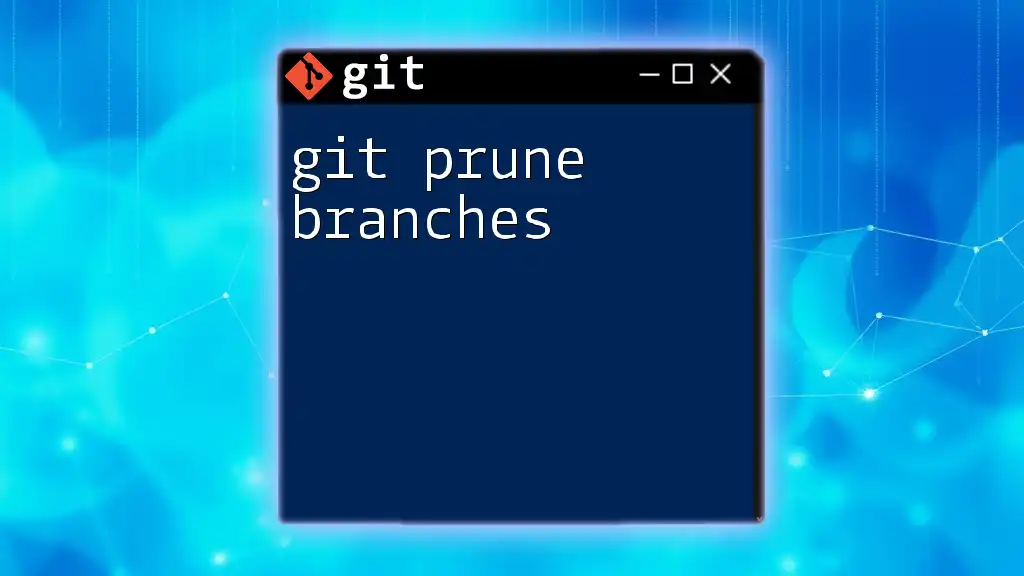
Practical Usage of Git Tags
Creating and Managing Tags
Creating a tag is straightforward. For example, to create an annotated tag labeled `v1.0`, you can use the following command:
git tag -a v1.0 -m "Version 1.0 release"
In this command, `-a` stands for 'annotated', allowing you to add a message that describes the tag. The `-m` option specifies the message.
Listing Tags
You can easily view all existing tags in your repository with the command:
git tag
This will display a list of all tags you've created, serving as a handy reference for your project's notable versions.
Deleting a Tag
If you need to remove a tag for any reason, you can do so with the command:
git tag -d v1.0
Be aware that deleting a tag does not affect the actual commits; it only removes the label you’ve applied to a specific commit.
Pushing and Pulling Tags
After creating tags locally, you often want to share them with others by pushing to a remote repository. To push a specific tag, use:
git push origin v1.0
If you have multiple tags and wish to push all of them, you can do so with:
git push --tags
To fetch tags from a remote repository, enabling you to see updates from your team:
git fetch --tags
Annotated vs Lightweight Tags
Understanding the difference between tag types is essential:
-
Lightweight Tags are essentially bookmarks to a commit without any additional information apart from the reference to that commit. They are quicker to create but lack the detailed metadata that might be useful later on.
-
Annotated Tags are the recommended choice because they include additional information, such as the author's name, email, and date of the creation. This makes it easier to identify when and why a specific tag was created, adding valuable context.
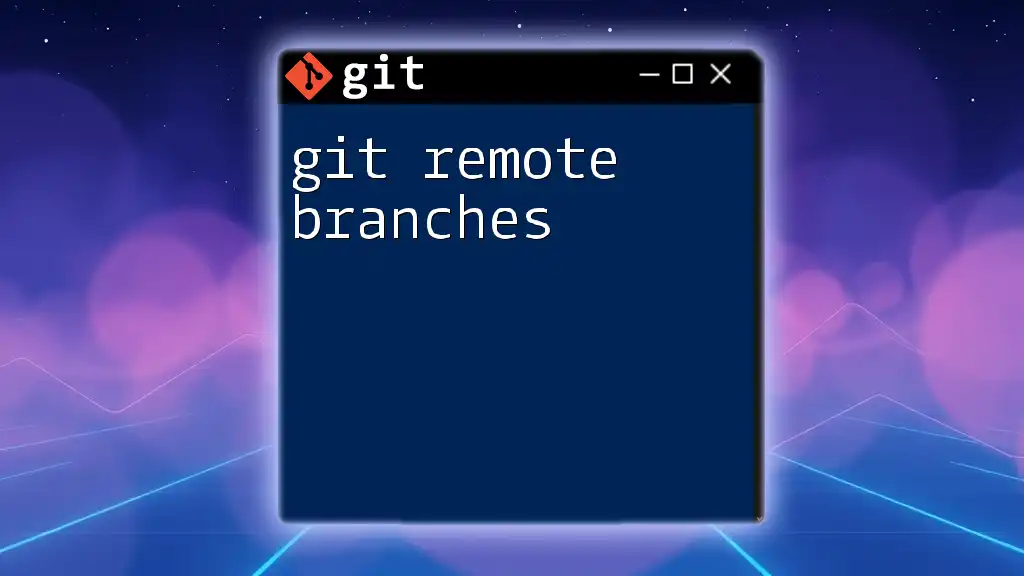
Practical Usage of Git Branches
Creating and Managing Branches
Creating a branch in Git is a simple but essential task. To create a new branch named `feature-branch`, you would use:
git branch feature-branch
Once the branch is created, you can switch to it with:
git checkout feature-branch
If you need to delete a branch after you’re done with it, use:
git branch -d feature-branch
Merging Branches
Once the work on a feature branch is complete and thoroughly tested, you might want to merge it back into the main branch. Use the following command to merge:
git merge feature-branch
This integrates the changes from `feature-branch` into your current branch, typically the main or master branch.
Branching Strategies
Employing a clear branching strategy can greatly enhance your workflow. Here are two popular strategies:
-
Git Flow involves defining a strict process where branches are created for specific tasks—such as feature development, releases, or hotfixes—and provides a structured approach to development.
-
GitHub Flow is a more simplified approach, emphasizing the idea of creating short-lived branches to handle features and fixes, which are then merged into the main branch frequently. This strategy promotes continuous integration and ensures that the main branch remains always deployable.
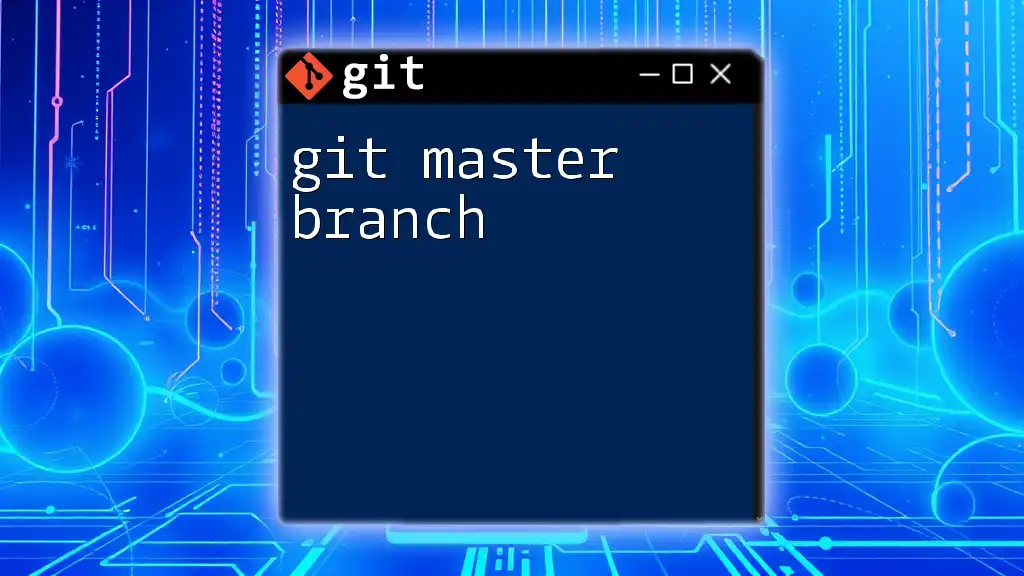
Conclusion
In summary, understanding git tags vs branches is crucial for effective version control in software development. Branches allow for structured workflows and parallel development, while tags provide an invaluable record of your project’s history, marking key milestones and versions.
As you grow more familiar with Git, experimenting with both branches and tags will enhance your ability to manage complex projects and maintain a clear historical record of your work.
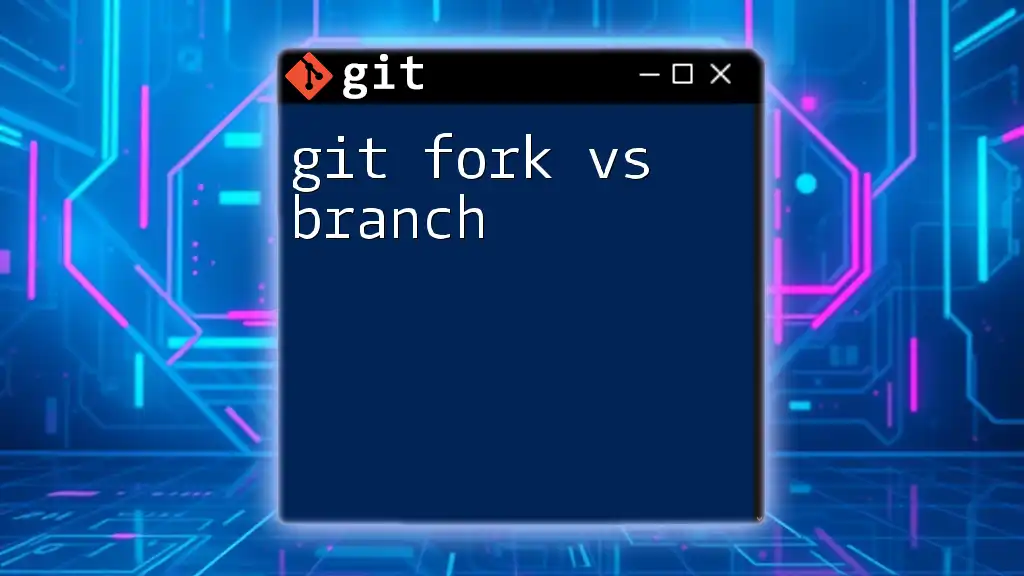
Frequently Asked Questions (FAQs)
Can I Tag a Branch?
Yes, you can create a tag that points to any commit, including those that are part of a branch. This flexibility allows you to mark specific points of interest regardless of their associated branch.
What Happens If I Delete a Branch with Tags?
Deleting a branch does not affect the tags, as tags are independent markers. However, keep in mind that if the tag is not pushed to the remote, it will only exist locally and can potentially be lost if your local branch is deleted.
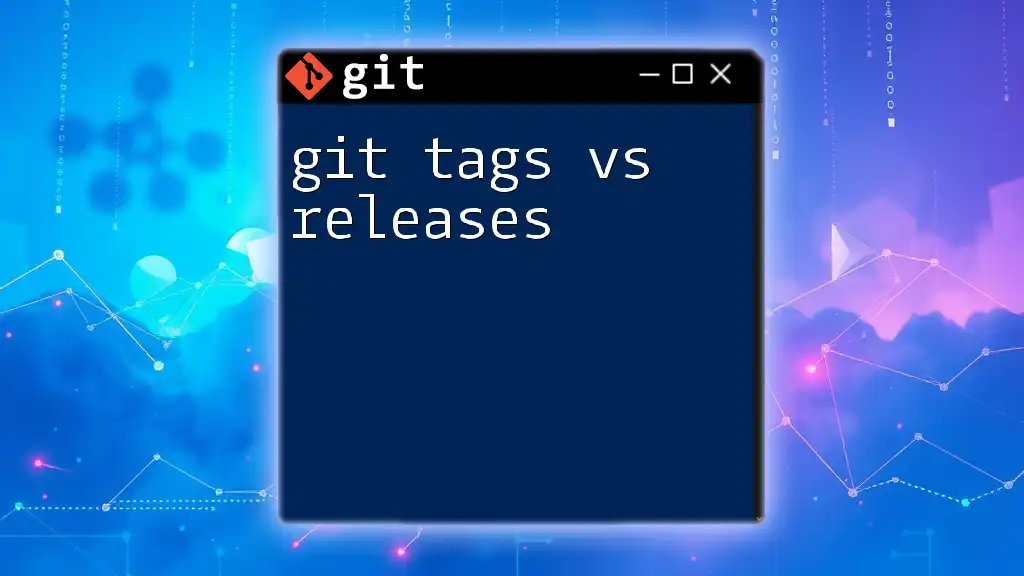
Additional Resources
For further learning, consider exploring the official Git documentation or other resources such as tutorials and guides focused on Git workflows and best practices. These materials can provide deeper insights and advanced techniques to enhance your Git skills.