"Git exit code 128 indicates that there was an error related to the repository, such as attempting to perform an operation in a directory that isn’t a Git repository."
Here's a code snippet demonstrating how you might encounter this error:
git status
This command will return exit code 128 if executed in a non-Git directory.
Understanding Git Exit Codes
What Are Exit Codes?
Exit codes are numerical values returned by command line applications upon completing a task. They signal whether the execution was successful (often a `0`) or if an error occurred (non-zero values). Understanding these codes is crucial for diagnosing and troubleshooting issues, particularly in programming tools like Git.
The Importance of Exit Code 128
Among the various exit codes in Git, exit code 128 signifies that a more severe failure has occurred, often tied to configuration or environmental issues. Recognizing exit code 128 is vital as it enables developers to pinpoint the problem quickly, leading to more effective resolutions.
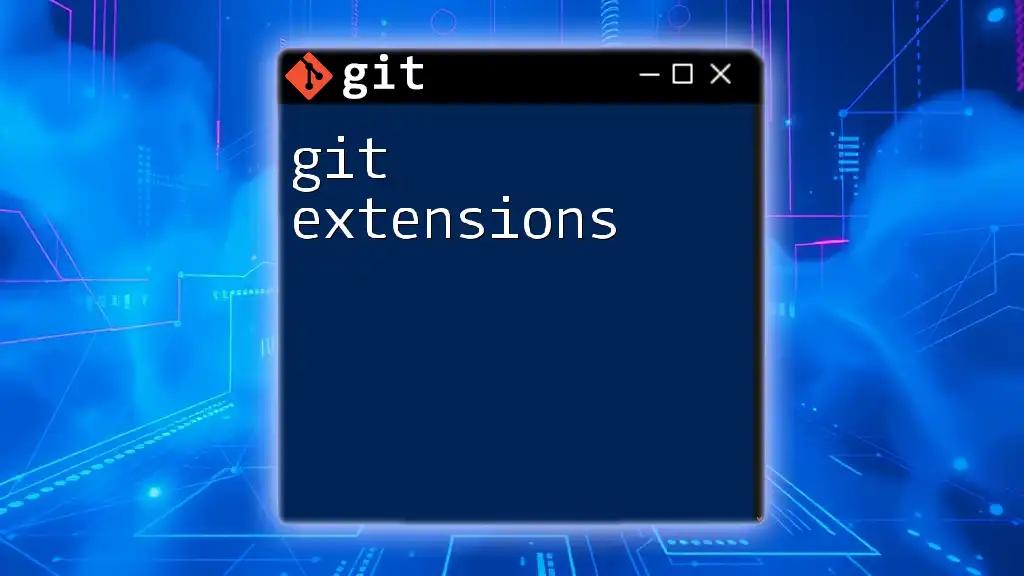
Common Causes of Exit Code 128
Repository Not Found
One common scenario that leads to exit code 128 is attempting to operate on a repository that Git cannot find. This typically happens during clone operations. For example:
git clone https://github.com/nonexistent/repo.git
When this command is executed, Git will attempt to clone a repository that does not exist, resulting in an exit code 128.
Solution: Always double-check the repository URL. A misspelling or incorrect case can lead to this issue.
Permission Denied
Another frequent cause of exit code 128 is permission denial. This occurs when you do not have the required access rights to perform a Git operation. For instance:
git push origin main
If your SSH keys are not properly configured or your HTTP credentials are outdated, Git will throw an exit code of 128.
Solution: Ensure that your SSH keys are accurately set up. You can regenerate your keys if necessary. Verify also that your Git account has the appropriate permissions for the repository in question.
Insufficient Disk Space
An often overlooked trigger for exit code 128 is running out of disk space while executing Git commands. Git requires ample space to perform various tasks, such as creating commits or pushing changes. For example:
git add .
git commit -m "Adding files"
git push
If you execute these commands and your disk is full, Git will fail and return an exit code of 128.
Solution: Regularly check your available disk space and delete unneeded files to avoid this issue.
Invalid Reference
Exit code 128 can also arise when trying to access a branch or tag that does not exist in your repository. For example:
git checkout nonexistent-branch
The attempt to switch to a non-existent branch will cause Git to throw this error.
Solution: Verify the existence and spelling of the branch or tag you wish to access by running commands like `git branch -a` to list all branches.
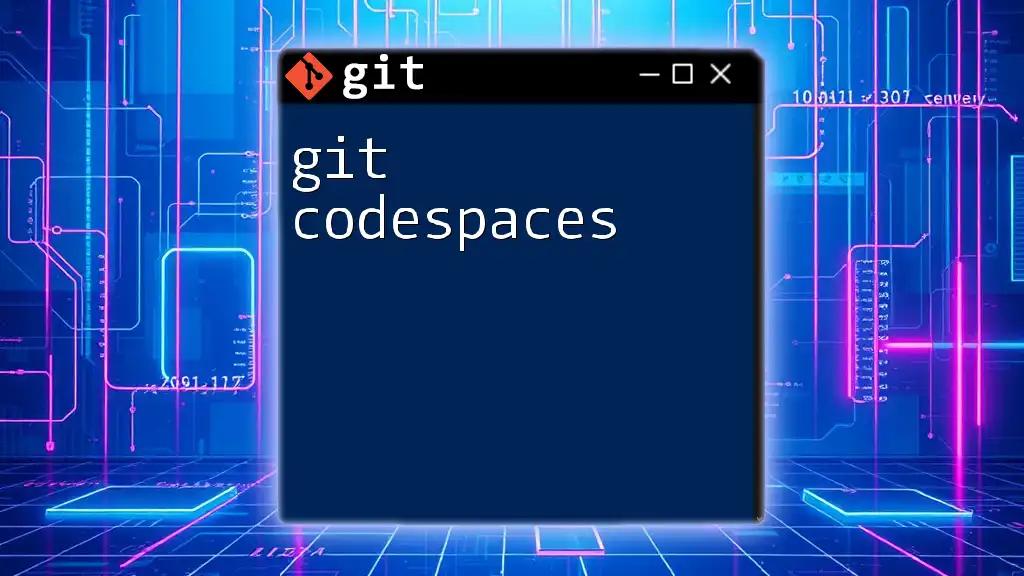
Diagnosing Exit Code 128 Errors
Checking the Output Messages
When you encounter exit code 128, the command line typically provides context via error messages. Carefully read these output messages; they often contain the key to resolving your issue. Understanding what Git is trying to communicate can help you pinpoint the exact cause of the failure.
Utilizing Git Commands for Troubleshooting
Several Git commands can assist in diagnosing problems that lead to exit code 128. For example:
- To validate remote URLs, run:
git remote -v
- To inspect the status of your repository before executing any operations:
git status
These commands can provide insights and help confirm that issues stem from the repository itself.
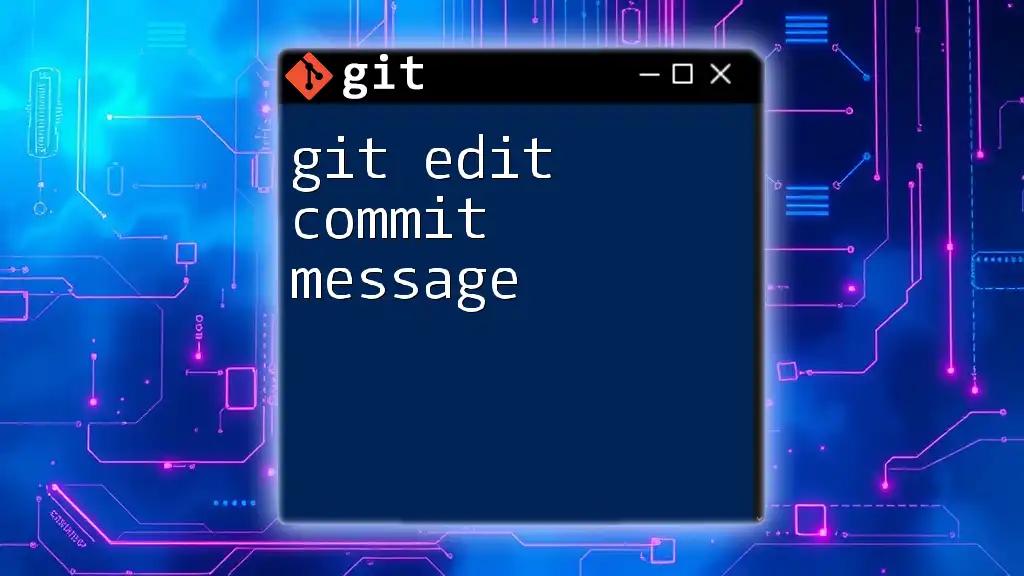
Fixing Exit Code 128 Issues
Correcting Repository Location Issues
If you find that the repository location is incorrect, fixing it is usually straightforward. You can update the URL of a remote repository using:
git remote set-url origin https://github.com/user/repo.git
This command will correct the remote URL, allowing you to proceed without errors.
Resolving Permission Issues
When you encounter permission denied errors, updating or generating new SSH keys might be necessary. To generate an SSH key, use:
ssh-keygen -t rsa -b 4096 -C "your_email@example.com"
Follow the prompts to create a new key, then add this key to your Git hosting service (e.g., GitHub or GitLab) to resolve access issues.
Managing Local Repository Issues
If your local repository has become corrupted or misconfigured, it may also lead to exit code 128. In such cases, you can perform repository maintenance via:
git fsck
This command checks the integrity of your repository, identifying and potentially repairing issues. If the repository is severely damaged, consider cloning a fresh copy of the repository as a last resort.
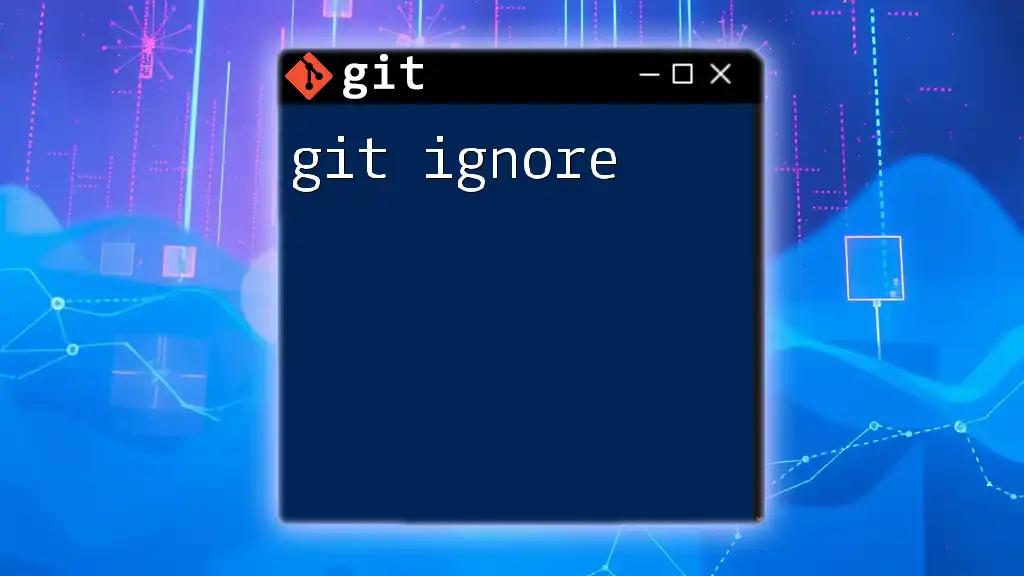
Best Practices to Avoid Exit Code 128
Regular Maintenance of Repositories
To minimize the chances of encountering exit code 128, it’s advisable to conduct regular maintenance on your repositories. This includes:
- Pruning remote-tracking branches that no longer exist:
git remote prune origin
Maintaining a clean repository structure allows you to avoid confusion and errors down the line.
Understanding and Using Branching Best Practices
Employing structured branching strategies, such as Git Flow, can significantly reduce chaos within repositories. This structured approach helps ensure that branches are managed correctly, making it less likely to run into issues associated with invalid references.
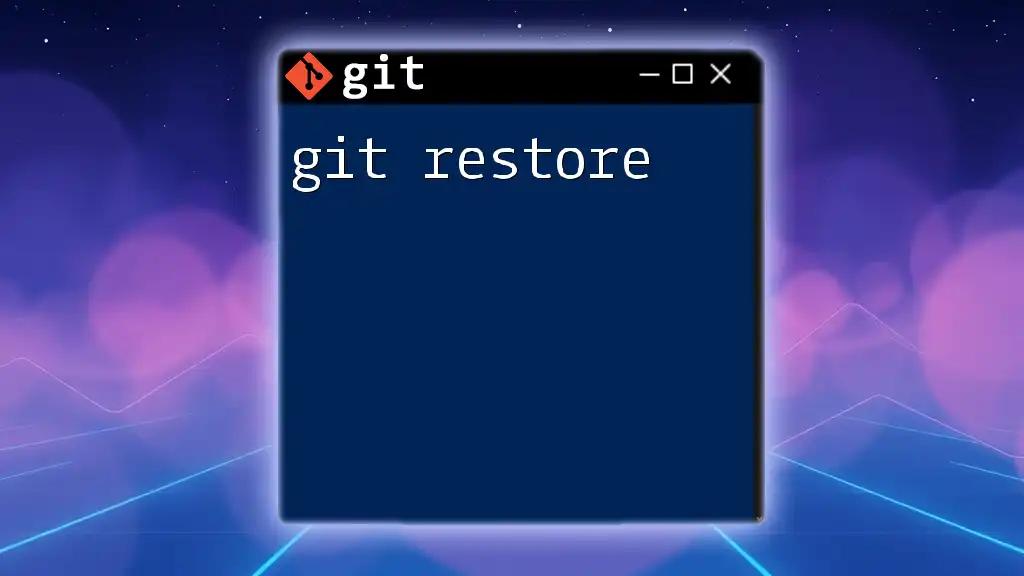
Conclusion
Understanding exit code 128 is crucial for any Git user. It serves as a signal indicating deeper issues within your repository or configuration. By mastering the causes and remedies associated with this exit code, you can troubleshoot effectively and streamline your development workflow. Engaging with Git's official documentation and continued practice will further elevate your command over Git, enabling you to prevent and resolve issues swiftly.
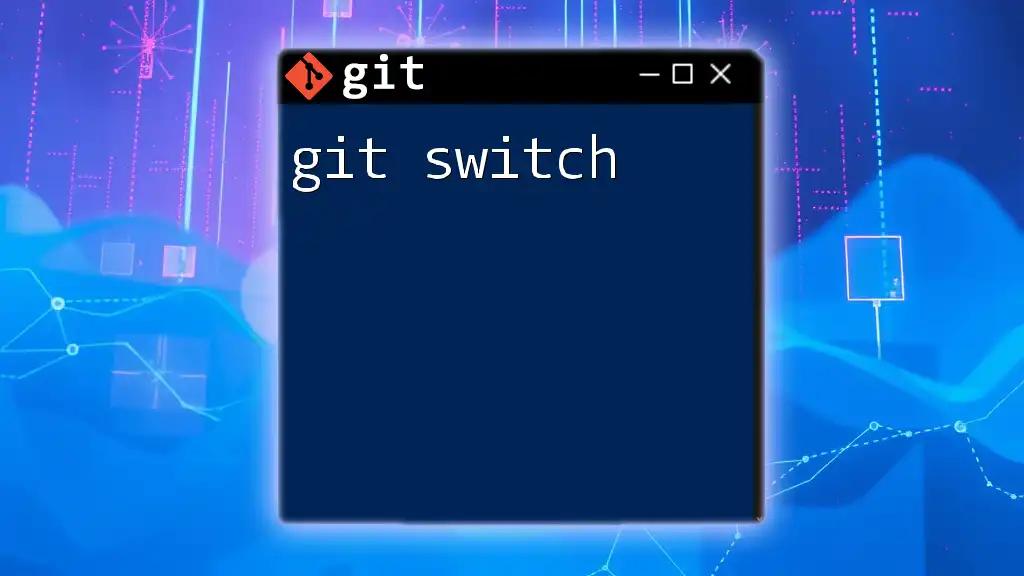
Additional Resources
For more detailed information on handling exit codes and Git in general, refer to the [official Git documentation](https://git-scm.com/doc). Additionally, consider exploring online courses and tutorials focused on mastering Git commands and troubleshooting practices.
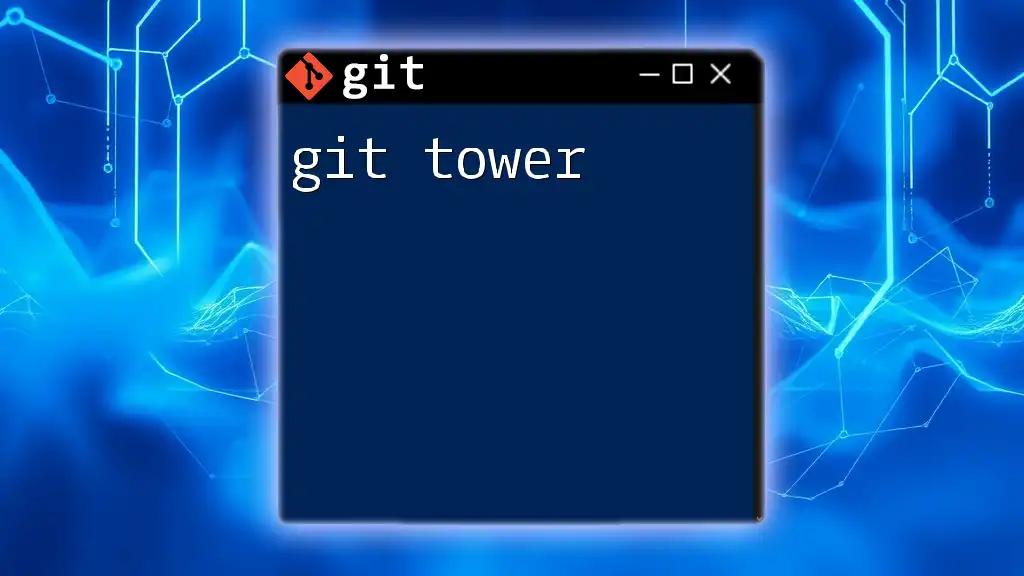
Glossary of Terms
In any technical discussion, using clear terminology enhances understanding:
- Exit Code: A number returned by a command indicating its success or failure.
- Repository: A storage location for your projects and their version histories.
- Commit: A recorded change in the repository that documents the state of files at a certain point.
- Branch: A separate line of development within a repository, allowing for isolated changes.