In Git, you can use a `for` loop in conjunction with `git branch` to iterate over and perform actions on each branch in your repository.
for branch in $(git branch | sed 's/^..//'); do echo $branch; done
Understanding Git Branching
What is a Git Branch?
A Git branch is essentially a lightweight movable pointer to a commit in your repository. It allows developers to diverge from the main line of development and continue to work independently without affecting the main codebase until it is ready to be merged back. In collaborative projects, this is crucial as multiple developers can work on different features simultaneously.
Benefits of Using Branches
Utilizing branches in Git provides numerous advantages:
- Parallel Development: Multiple features, bug fixes, or experiments can occur simultaneously. Developers can work on their branches independently, enhancing productivity.
- Enhanced Organization and Management: Keeping work isolated in branches reduces the risk of introducing bugs into the main code.
- Easier Collaboration: Teams can use branches for various features or tasks, and merging their successful implementations back into the main branch becomes straightforward.
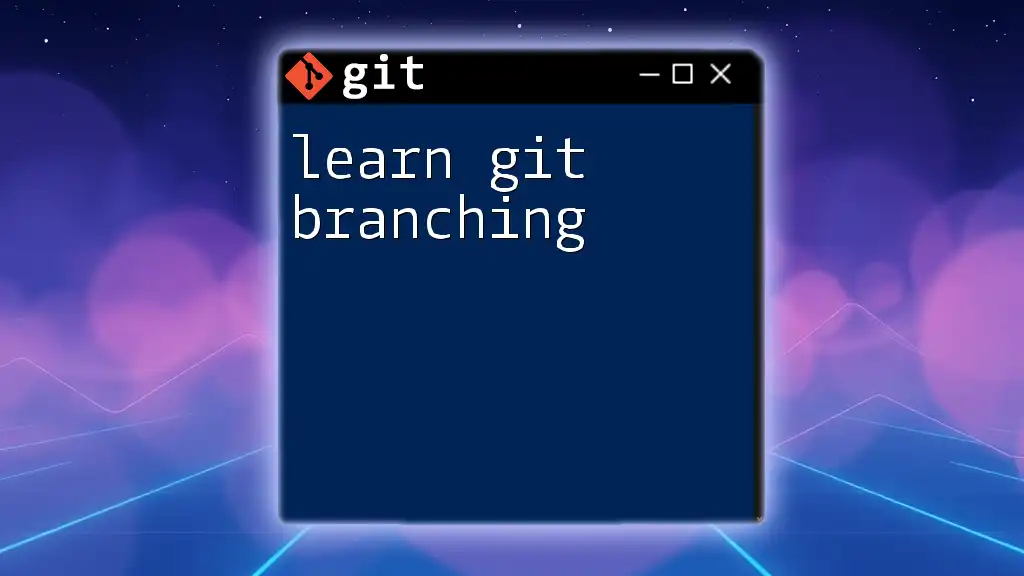
The Structure of Branches in Git
How Git Manages Branches
Git manages branches through a unique model that refers to commits in a data structure akin to a tree. The HEAD is a special pointer that denotes the current branch you are on. Each branch points to the last commit made in that branch, and switching branches updates HEAD to point to the new branch's last commit.
Common Branching Terminology
Understanding Git branching terminology is vital:
- Master/Main Branch: This is the default branch created when setting up a repository; developers typically stabilize and deploy from this branch.
- Feature Branches: These are branches created for developing a specific feature or fixing a bug. They are isolated from other ongoing work until they are merged back into the master branch.
- Merge, Checkout, and Rebase: These terms refer to combining branches, switching between branches, and restructuring commits respectively, and are fundamental to manipulating branches effectively.
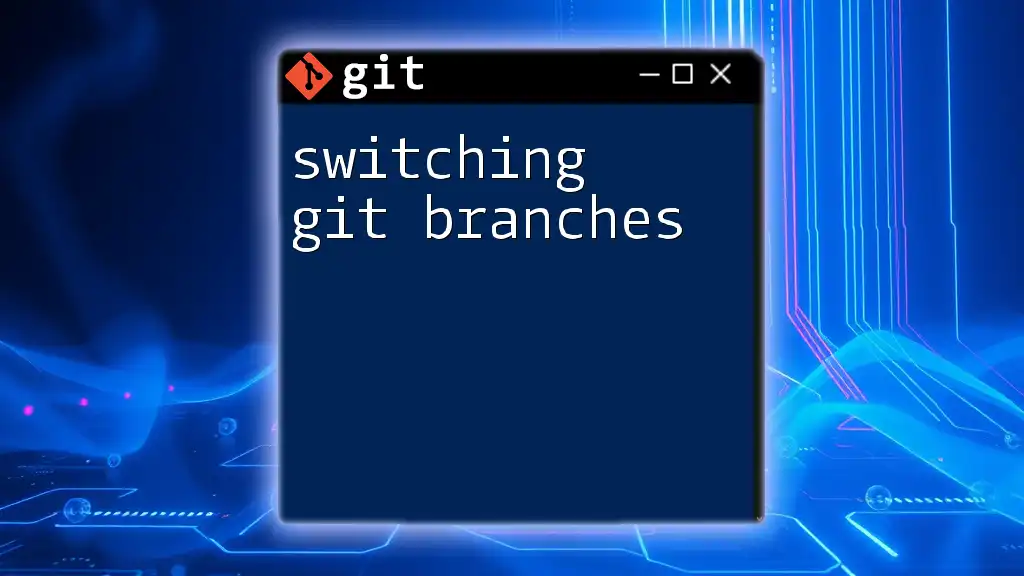
Basic Git Branch Commands
Creating a New Branch
To create a new branch, you use the command:
git branch <branch-name>
For example, to create a feature branch for a login form, you would execute:
git branch feature/login-form
This command simply creates the branch; however, it does not switch to it immediately.
Switching Between Branches
Once a branch is created, you can switch to it using:
git checkout <branch-name>
Using the previous example, to switch to your new feature branch, you would run:
git checkout feature/login-form
This command updates the HEAD pointer to the specified branch, allowing you to work on it.
Viewing Existing Branches
To see all your branches and determine which one you are currently on, use:
git branch
This command lists all branches in your repository, with an asterisk (*) next to the currently checked-out branch.
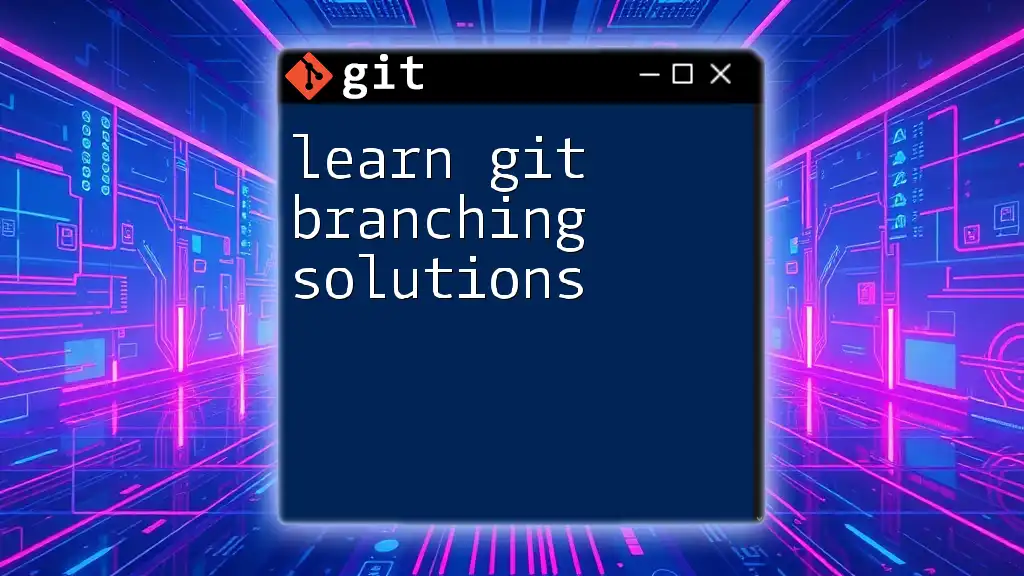
Using "For Each" in Branching
The Concept of Iteration in Branching
In Git, iterating over branches allows you to apply commands selectively or en masse. If you have multiple feature branches, you can automate tasks like merging or checking out all branches using a loop. This not only saves time but also ensures consistency across your operations.
Benefits of "For Each" in Git Branches
Utilizing a "for each" approach in Git helps in various scenarios, such as:
- Streamlining operations across multiple branches, particularly during merges.
- Simplifying management of feature branches by allowing batch operations.
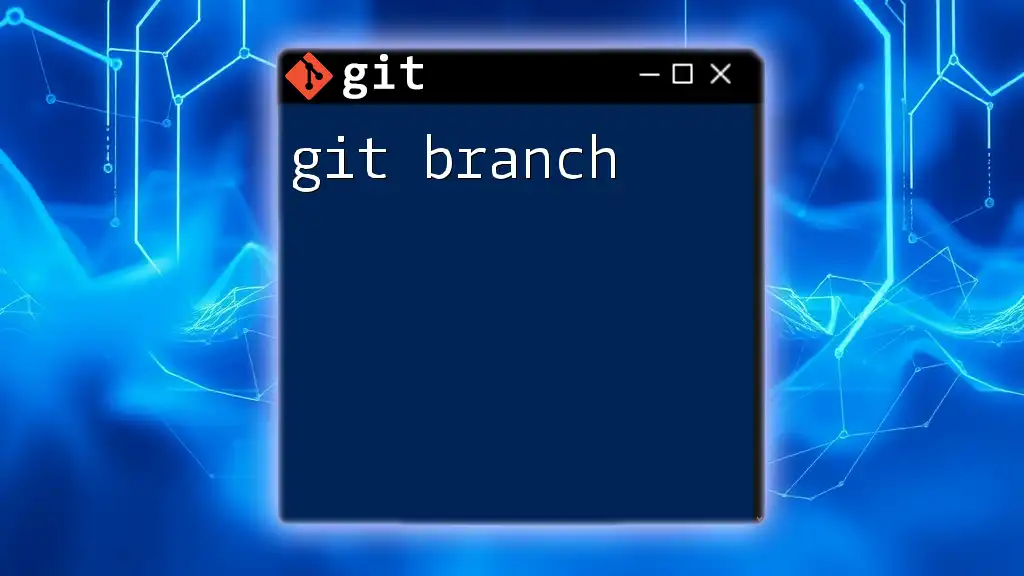
Implementing "For Each" Logic in Git
Basic "For Each" Loop Example
Using shell scripting, you can iterate over your branches effectively with the following command:
for branch in $(git branch | sed 's/..//'); do
echo "Branch: $branch"
done
This script uses `git branch` to list all branches, pipes it through `sed` to remove leading characters, and then echoes each branch name.
Practical Applications
Checking Out All Branches
You might find it necessary to check out and work on each branch consecutively. Here's how you can do it:
for branch in $(git branch | sed 's/..//'); do
git checkout $branch
# Perform actions here
done
This loop will switch to each branch listed. You can add further processing commands where specified.
Merging Changes Across Branches
For teams that frequently need to merge updates from the main branch into all feature branches, the following script can be very handy:
for branch in $(git branch | sed 's/..//'); do
if [ $branch != "main" ]; then
git checkout $branch
git merge main
fi
done
This will check out each branch except for the main branch and merge updates from main into it, ensuring all branches remain up to date.
Using Git Aliases for "For Each" Operations
To enhance efficiency, consider creating an alias for "for each" operations. This can simplify your workflow significantly. Use the following command to create an alias:
git config --global alias.foreach '!f() { for branch in $(git branch | sed 's/..//'); do echo $branch; done; }; f'
Once this is set up, you can call `git foreach` to run your loop, vastly improving your command efficiency.
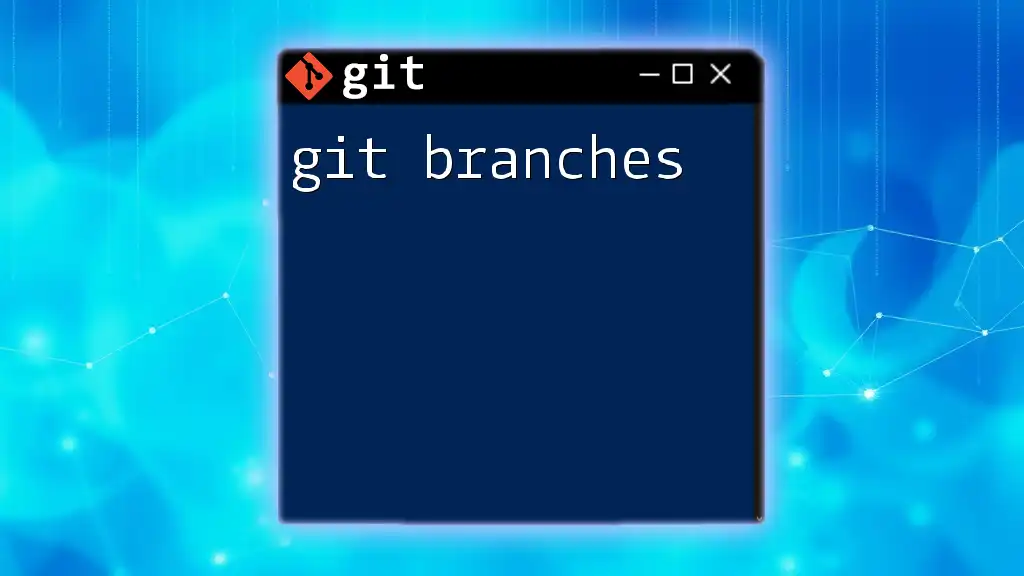
Tips for Efficient Branch Management
Best Practices for Branch Naming
Consistent and descriptive branch naming is key in collaborative environments. Aim for clear identifiers that quickly convey the purpose of the branch. For example, use prefixes like `feature/`, `bugfix/`, or `hotfix/` to categorize branches effectively.
Regular Maintenance of Branches
Keeping your repository clean involves regularly deleting branches that are no longer needed. You can delete local branches using:
git branch -d <branch-name>
And if you want to prune deleted remote branches, you can execute:
git fetch --prune
This practice helps maintain organization and prevents confusion among team members.
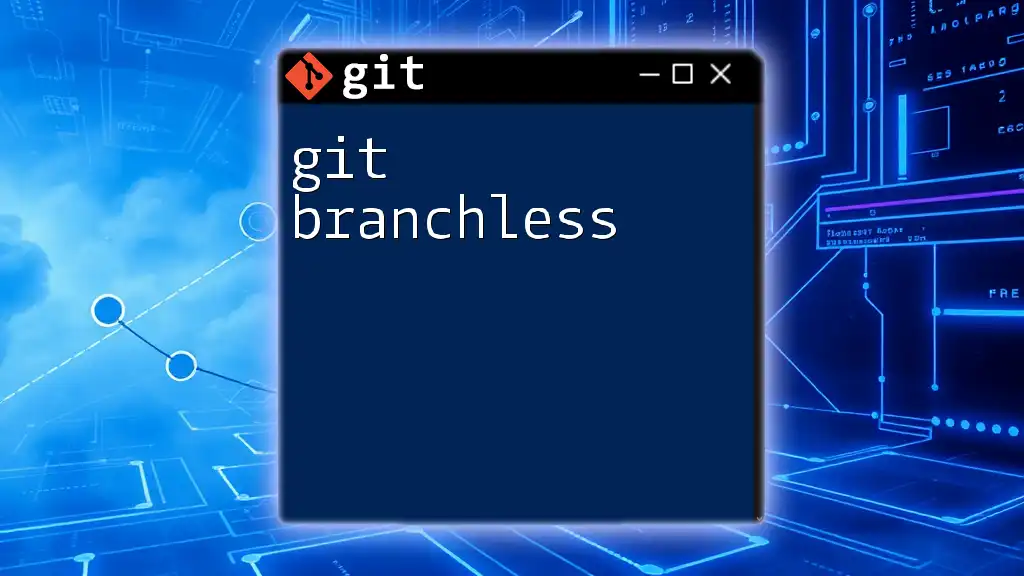
Conclusion
Mastering the concept of for each on git branch allows developers to manage their feature branches effectively, enhancing collaboration and productivity in projects. The techniques discussed in this guide enable not only individual productivity but also foster a better collaborative environment by ensuring features and fixes are neatly organized. By practicing and implementing these commands, you will increase your Git proficiency, leading to more efficient project workflows.
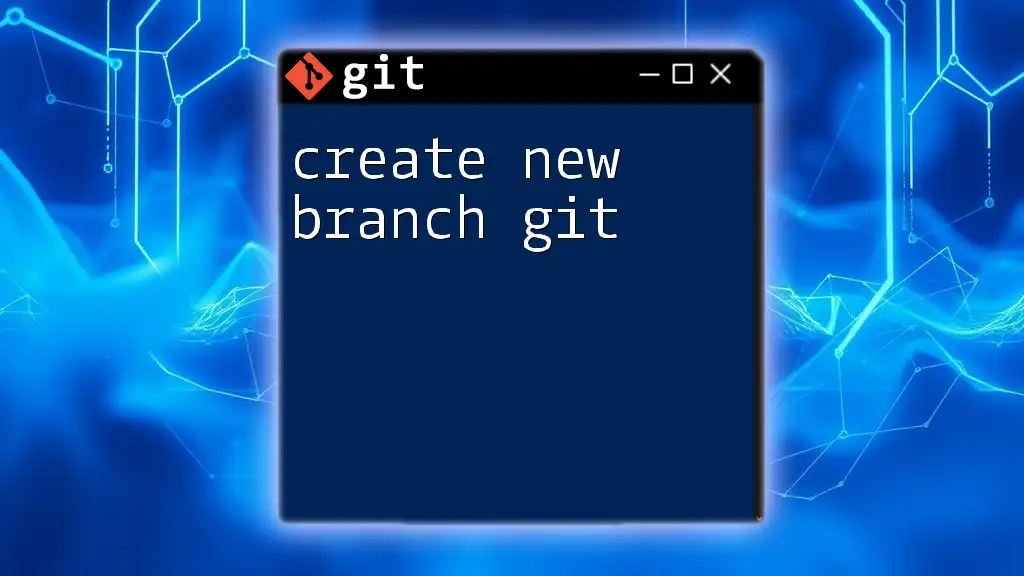
Call to Action
To delve deeper into Git and streamline your version control processes, consider signing up for our Git command tutorials. Access additional resources to enhance your understanding and become an expert in using Git effectively.