The error message "does not appear to be a git repository" indicates that Git can't find the necessary repository metadata (like the `.git` directory) in the specified path, which may happen if you're not in a Git repository or if the repository is incorrectly initialized or corrupted.
git status
Understanding the Error Message
When you come across the message that states your directory “does not appear to be a Git repository,” it means that Git cannot find the necessary files and directories that denote a valid Git repository. Specifically, Git is looking for the `.git` folder, which contains metadata and version controls information.
Why This Error Occurs
This error can happen in multiple scenarios. Understanding the underlying causes is essential for effectively troubleshooting the issue.
The primary distinction to grasp here is the difference between a working directory and a Git repository. A working directory is merely where your projects reside, while a Git repository has a hidden `.git` folder that contains all the data, history, and information Git needs to track changes.

Common Causes of the Error
Incorrect Directory
If you are trying to run a Git command within a directory that isn’t part of a Git repository, you will encounter this error.
For instance, when you execute:
git status
in a directory that isn’t initialized with Git, you will see the message that “does not appear to be a Git repository.” To confirm your current directory, use the command:
pwd # On Unix/Linux/Mac
or
cd # On Windows
Missing `.git` Directory
Every Git repository has a hidden directory called `.git`. This directory contains all the necessary files for version control.
If this directory is missing, Git will naturally respond with an error. If you’ve created a directory without initializing it as a Git repository, you can do so with the following command:
git init
Once you initialize, you can start adding files with:
git add .
Corrupt Git Repository
Corruption of a Git repository can happen due to various reasons, such as improper shutdowns, hardware failures, or software bugs. Signs of a corrupted repository often include missing commits or weird error messages when executing commands.
To diagnose a corrupted repository, you can run the Git integrity check command:
git fsck
This command inspects the integrity of your Git database and can help determine if issues exist.
Cloning Issues
Improperly cloning a repository can lead to this error as well. If a clone operation is incomplete or if you provide an incorrect URL, the expected files and directories won’t be established.
To clone a repository correctly, use the command:
git clone <repository-url>
Make sure to check the URL syntax and also verify that you have the necessary access permissions—either SSH or HTTPS keys.

How to Resolve the Error
Verifying Your Current Directory
First and foremost, confirm that you are in the right directory. Use the command:
pwd
Checking your Git status with:
git status
can also help verify whether you are within a Git repository.
Setting Up a New Git Repository
If you've confirmed that your directory isn't a Git repository, you can easily set one up. First, navigate to your project folder and run the command below to initialize the repository:
git init
Next, you can add your existing files to be tracked by Git:
git add .
Then, don't forget to commit your changes with:
git commit -m "Initial commit"
Recovering from Repository Corruption
In the event that you suspect the corruption of your repository, the first step is to identify the issues through:
git fsck
If the corruption is minor, you may recover your repository using the reflog command:
git reflog
This command shows a history of all recent actions and can help you revert to a previous state before the corruption occurred.
Cloning a Fresh Copy
If necessary, you can always clone a fresh copy of the repository. Double-check the repository URL you use:
git clone <repository-url>
Verify you have correct permissions to access the repository and that the URL is accurately formatted.

Preventing Future Issues
Best Practices for Managing Git Repositories
To avoid encountering the "does not appear to be a Git repository" error in the future, consider implementing a few best practices. Regularly checking the integrity of your repository using `git fsck` can preemptively catch issues.
Additionally, always write meaningful commit messages. Good messages help you understand changes in your repository history and easier collaboration.
Keeping Repositories Up to Date
It's a good habit to pull changes frequently using:
git pull
This helps keep your local repository in sync with its remote counterpart and mitigates the risk of merge conflicts, which can lead to confusion about the repository's integrity.
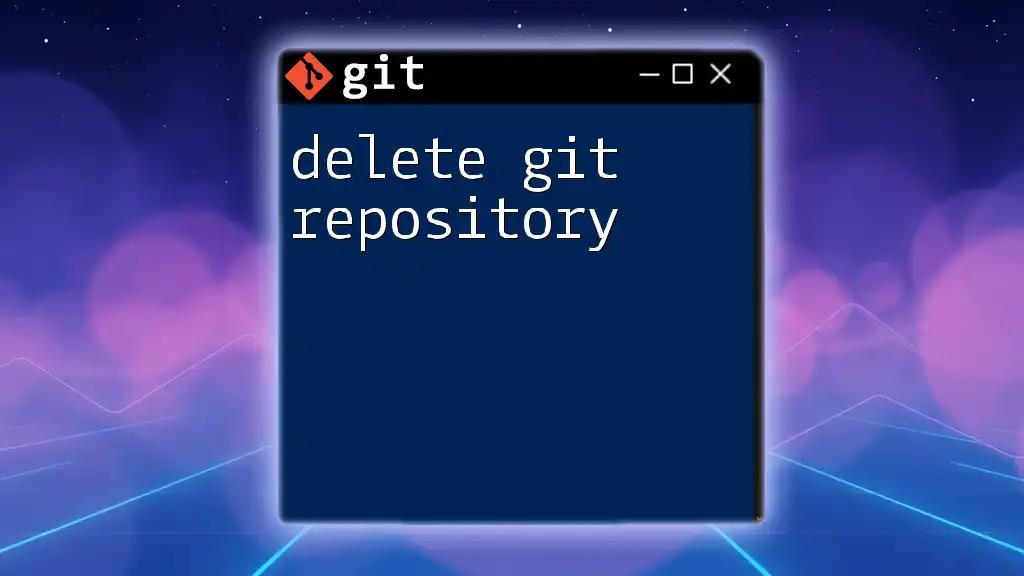
Conclusion
In summary, encountering the error that your directory “does not appear to be a Git repository” can be frustrating but is often resolvable with a few simple checks and commands. Remember to verify your current directory, initialize new repositories correctly, and keep an eye on repository integrity to minimize these issues.
With regular practice of Git commands and a proactive approach to repository management, you will find it easier to avoid this error in the future. Don't hesitate to experiment with different Git functionalities; the more you practice, the more confident you'll become in using this powerful version control tool.
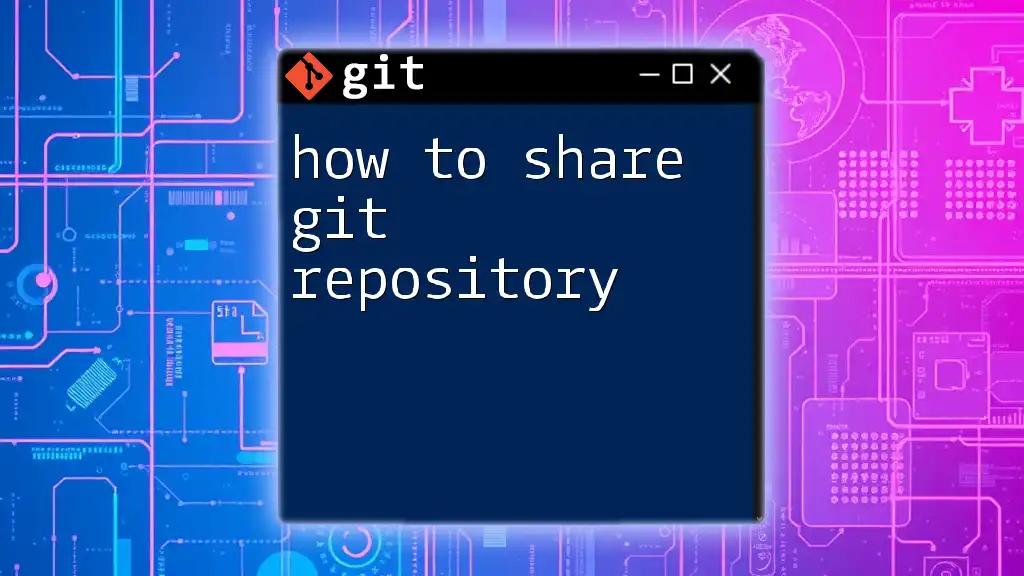
Appendix
Useful Commands Reference Table
- Check current directory: `pwd`
- Verify Git status: `git status`
- Initialize a repository: `git init`
- Add files: `git add .`
- Commit changes: `git commit -m "message"`
- Check integrity: `git fsck`
- View reflog: `git reflog`
- Clone a repository: `git clone <repository-url>`
Further Reading & Resources
- Comprehensive guides on Git usage
- Video tutorials explaining Git commands and workflows
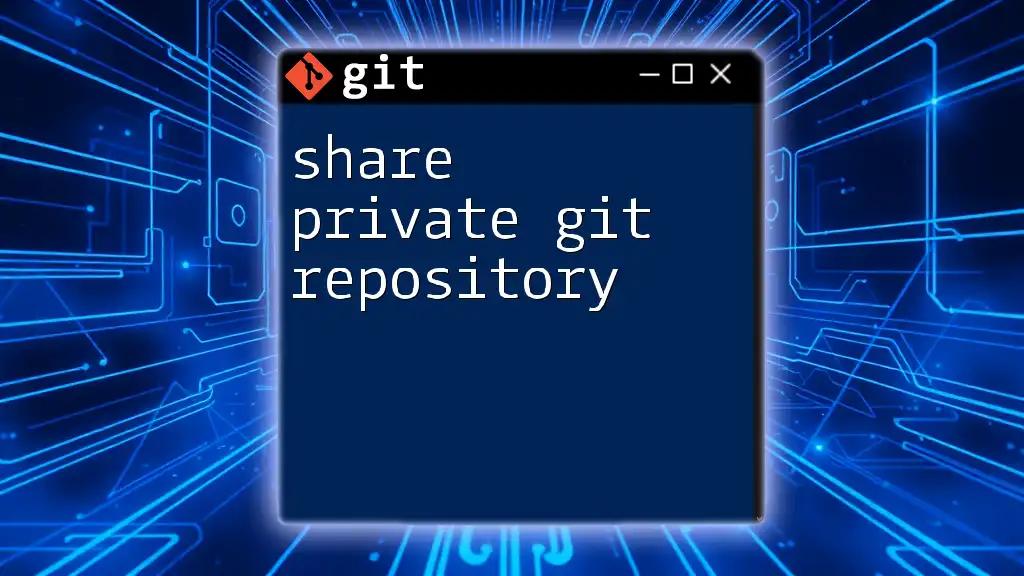
FAQ
What if the issue persists after trying all solutions? Consider investigating specific aspects of your environment, such as file permissions or exploring if your local Git installation needs updating or reinstallation.
Can I undo changes made to the `.git` directory? Recovery can often be achieved via the reflog or by restoring the repository from a backup, if available.
Are there tools available for Git repository management?
Yes, there are various GUI tools and IDE plugins that provide a user-friendly interface for managing Git repositories, making it easier to visually interact with version control features.