To remove untracked files in Git, you can use the command `git clean` with the `-f` option, which forcefully deletes all untracked files in your working directory.
git clean -f
Understanding Untracked Files
What Are Untracked Files?
In Git, untracked files are those files in your working directory that Git is currently not tracking. Unlike tracked files, which are part of Git's index and committed to the repository history, untracked files are new or modified files that have not yet been added to your staging area. Essentially, these files are not monitored by Git until they are explicitly added using the `git add` command.
Untracked files may include temporary files, log files, or any other files that have not been added to the Git repository yet. Recognizing the difference between tracked and untracked files is crucial; untracked files can lead to clutter or confusion, particularly as your project develops.
Why You Might Have Untracked Files
There are several common scenarios in which untracked files occur:
- New Files: Whenever you create a new file in your project, it starts as untracked until you add it to the staging area.
- Generated Files: Files created by build processes or other automation tools often show up as untracked, making it essential to manage them.
- Ignored Files: If you have a `.gitignore` file that specifies files or directories for Git to ignore, those items will remain untracked.
Leaving untracked files in your working directory can lead to potential confusion, distractions, and even accidental commits of files you don't wish to track. Therefore, managing those files effectively is a fundamental part of working with Git.
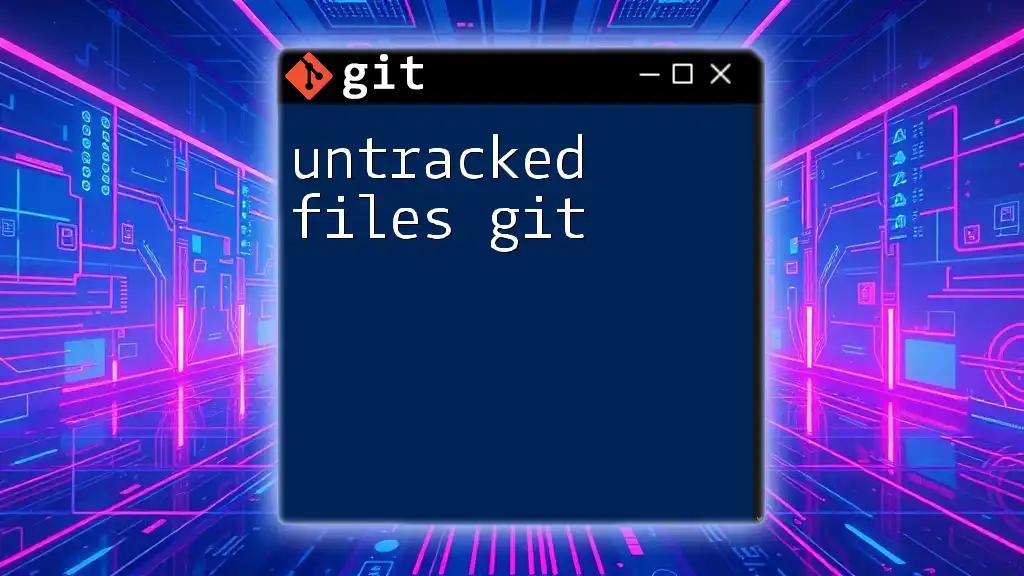
Viewing Untracked Files
Using the `git status` Command
A simple way to see untracked files is by running the `git status` command. This command provides a summary of your project's state, indicating which files are tracked and untracked.
Example command:
git status
When you execute this, the output will include a list of untracked files under the section titled "Untracked files." This is a vital first step in managing your environment, as it gives you visibility into what is currently untracked.
Listing Untracked Files Specifically
If you want a more focused view of your untracked files, you can use the `git ls-files` command. This command allows you to list untracked files without the noise of tracked files.
Example command:
git ls-files --others --exclude-standard
The options used here tell Git to show only files that are untracked while excluding files ignored by `.gitignore`. This can help you pinpoint files that may require your attention.
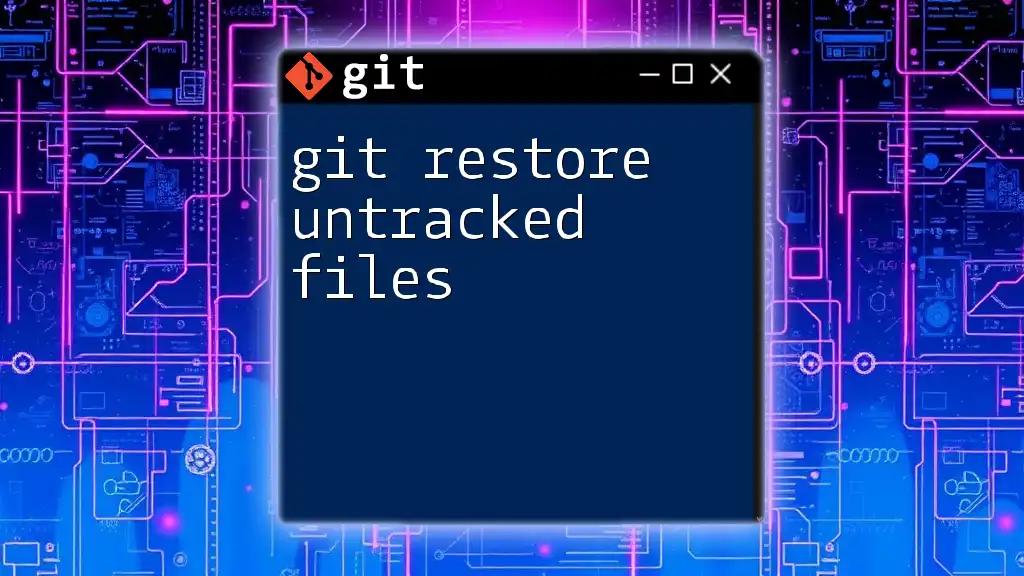
Removing Untracked Files
Soft Removal with `git clean`
To remove untracked files in Git safely, the command `git clean` is used. It offers various options to tailor its behavior for different scenarios, allowing you to clean your workspace effectively.
Warning: Before using `git clean`, proceed with caution. This command permanently deletes files, so make sure you really want to remove them!
Removing Untracked Files and Directories
Removing Only Untracked Files
If you decide to remove just the untracked files without touching directories, you can execute:
git clean -f
Here, `-f` stands for "force," as Git protects against accidental deletions. This command will remove untracked files from your working directory.
Removing Untracked Directories
Should you need to clean up both untracked files and directories, use:
git clean -fd
The `-d` option tells Git to also remove untracked directories in addition to untracked files.
Dry Run Before Removal
Before executing the deletion commands, especially in larger projects, it's often wise to perform a dry run. This allows you to preview what would be deleted without actually making any changes. Use the following command to simulate a clean-up:
git clean -n
Running this will list the files and directories that would be removed if you ran `git clean -f` or `git clean -fd`, providing an extra layer of safety before executing the actual removal.
Removing Ignored Files
Sometimes, you may wish to remove files specifically listed in your `.gitignore` without affecting other untracked files. To do this, you can run:
git clean -f -X
The `-X` option indicates that only ignored files should be removed.
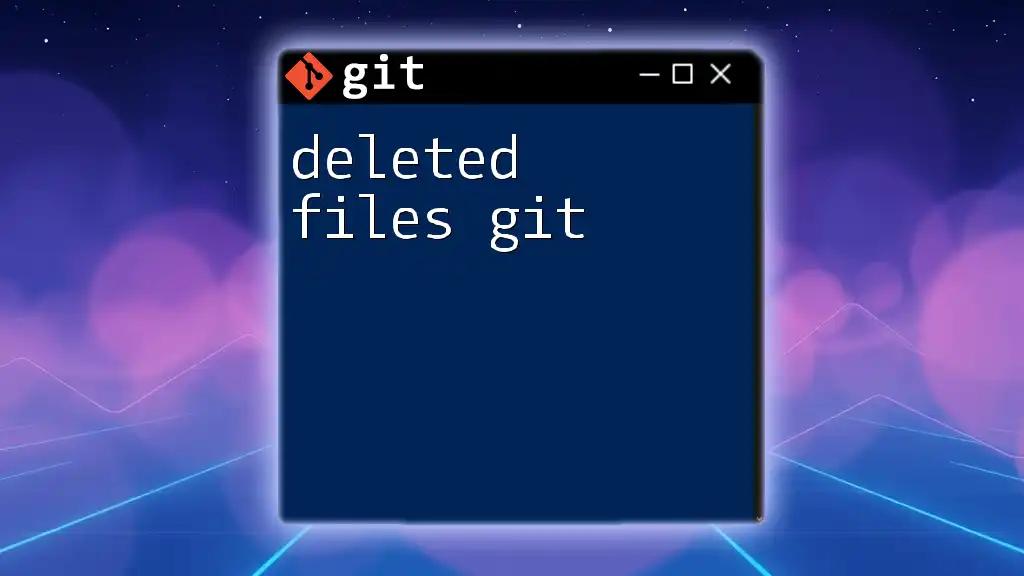
Best Practices
Regularly Checking for Untracked Files
It is prudent to routinely check for untracked files in your working directory. By incorporating a habit of running `git status` or `git ls-files` frequently, you can maintain a tidy repository and avoid clutter over time.
Using `.gitignore` Effectively
Maintaining a .gitignore file is crucial for managing untracked files efficiently. This file tells Git which files or directories to ignore, streamlining your workflow. A proper `.gitignore` can prevent unintentional submissions of unnecessary files such as build artifacts or local configuration files.
An example of a standard `.gitignore` file might look like this:
*.log
*.tmp
node_modules/
By ensuring that files listed in `.gitignore` remain untracked, you can keep your repository neat and prevent unwanted files from being committed.
Automation Tips
For those looking to automate the management of untracked files, consider the use of Git hooks. Set these hooks to check for untracked files before a commit, prompting a warning or cleaning up as necessary. Implementing automated checks such as a pre-commit hook can simplify your workflow and improve productivity.
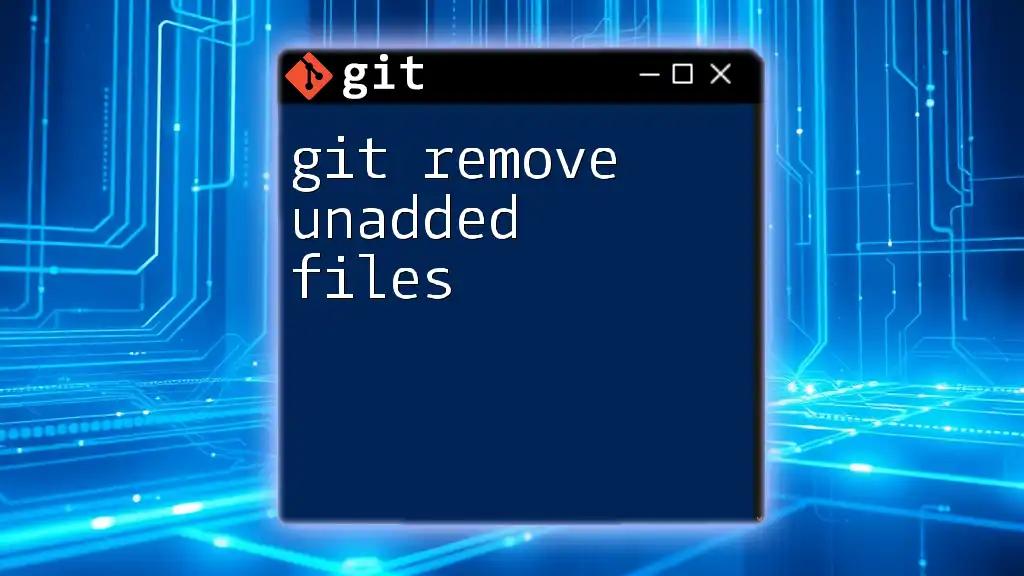
Conclusion
In summary, understanding how to remove untracked files in Git is a vital skill for any developer. By maintaining awareness of untracked files, employing commands like `git clean`, and establishing best practices, you can effectively manage your repository and maintain a clean working environment. Regular engagement with these practices will enhance your productivity and help ensure that your project remains organized.