In the debate of "git or jit," Git refers to the version control system used for tracking changes in source code, while JIT (Just-In-Time compilation) is a method of executing computer code that improves performance by compiling code at runtime.
Here's a basic Git command to create a new repository:
git init my-repo
Understanding Git
What is Git?
Git is a distributed version control system that allows multiple developers to work on a project simultaneously without stepping on each other's toes. Created by Linus Torvalds in 2005, it has since transformed how software development is conducted, becoming an essential tool in the programmer’s toolkit. Unlike centralized version control systems, where a single server stores all the code, Git enables each user to maintain a complete local copy of the repository.
Key Features of Git
Distributed Version Control
In Git, every user has a full-fledged repository on their local machine, including the project’s complete history. This means even without internet access, developers can commit changes, create branches, and record any alterations. When connectivity is restored, they can effortlessly synchronize with the main repository. This decentralization enhances collaboration and mitigates the risk of data loss.
Branching and Merging
One of Git’s standout features is its robust branching model. Branching allows developers to create independent lines of development. For example, a developer can create a new feature branch without affecting the main project. Key commands to know are:
git branch feature/my-new-feature
git checkout feature/my-new-feature
After development, merging these branches back into the main codebase is straightforward with:
git merge feature/my-new-feature
This fluid process encourages experimentation and nurtures innovation while maintaining project integrity.
Git Commands Overview
Getting Started with Git
Before diving into Git, it’s crucial to set it up properly:
-
Installation: Follow [official installation guides](https://git-scm.com/book/en/v2/Getting-Started-Installing-Git).
-
Basic Setup Commands:
- Initialize a new repository:
git init my-project
- Configure user information:
git config --global user.name "Your Name" git config --global user.email "you@example.com"
These foundational commands prepare Git to manage your projects effectively.
Commonly Used Git Commands
Working with Repositories
Git simplifies repository management, starting from initializing a repository to cloning existing ones:
git clone https://github.com/username/repo.git
Staging and Committing Changes
Understanding the staging area is crucial to maintaining clean commits. Use the following commands to handle your work:
- To stage changes:
git add .
- To commit changes with a descriptive message:
git commit -m "Add new feature"
- Check the status of your repository at any time with:
git status
This flow enhances accountability and makes collaboration more efficient.
Pushing and Pulling Changes
Git also allows for seamless synchronization between local and remote repositories, empowering developers to stay updated:
- To sync changes with the remote repository:
git push origin main
- To pull the latest changes from the remote:
git pull origin main
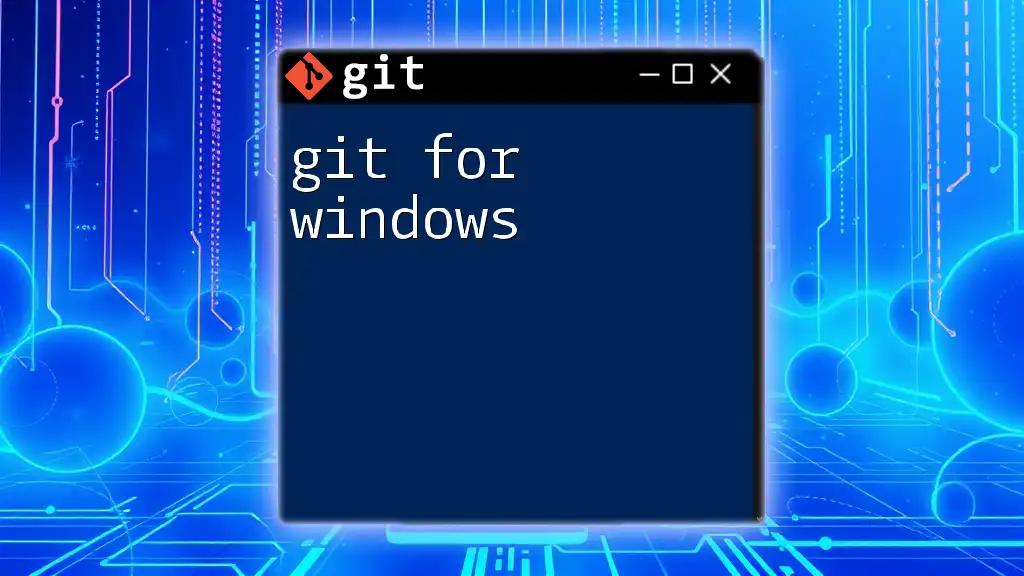
Understanding JIT
What is JIT?
Just-In-Time (JIT) compilation refers to a technique used to improve the runtime performance of programs. This process translates bytecode into native machine code during execution, optimizing the code as it runs. While fundamentally different from Git, which is primarily a version control tool, JIT plays a critical role in programming languages like Java and C# where performance can significantly impact applications' responsiveness.
How JIT Works
The JIT process operates in several critical phases:
- Compilation: Source code is first translated into an intermediate representation (like bytecode).
- Execution: When executed, the JIT compiler identifies hotspots—frequently executed paths in the code—and converts them into optimized machine code.
- Optimization: Subsequent executions of this machine code are faster because JIT can apply various optimizations that wouldn't have been possible during the initial compile phase.
This mechanism ensures that applications run efficiently without sacrificing the flexibility offered by interpreted languages.
JIT in Different Programming Languages
Java's JIT
Java Virtual Machine (JVM) employs JIT to enhance performance, making Java applications faster. A simple example demonstrating Java’s JIT behavior might look like:
public class Example {
public static void main(String[] args) {
for (int i = 0; i < 1000000; i++) {
// Hotspot code
process(i);
}
}
public static void process(int i) {
// Complex processing logic
}
}
In this case, `process(i)` could get optimized by the JIT compiler when it detects it's executed repeatedly.
.NET Framework's JIT
Similarly, Microsoft’s Common Language Runtime (CLR) uses JIT compilation. Here’s a C# example:
using System;
class Example {
static void Main() {
for (int i = 0; i < 1000000; i++) {
Process(i);
}
}
static void Process(int i) {
// Performance-critical logic here
}
}
This code benefits from JIT, boosting performance through various optimizations that the CLR performs at runtime.
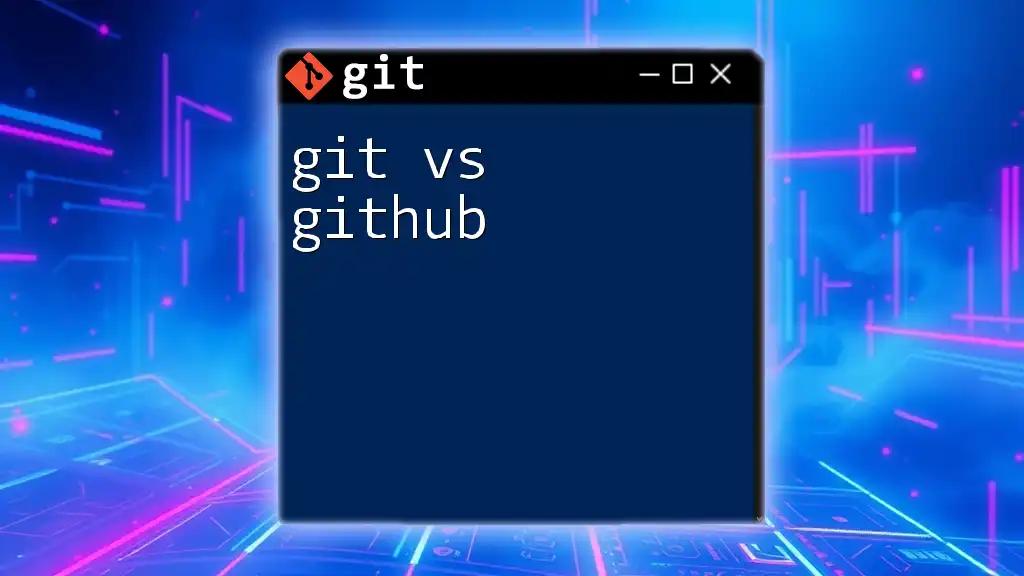
Git vs JIT
Key Differences Between Git and JIT
While Git and JIT serve distinct purposes, it’s essential to understand their core differences:
-
Purpose: Git is focused on version control, handling the history, branches, and changes in code. JIT, on the other hand, is about enhancing performance during the execution of programs.
-
Functionality: Git provides tools to track changes and collaborate, while JIT optimizes the execution of code in real-time.
-
Use Cases: Use Git for collaboration and tracking project changes; leverage JIT to enhance performance in applications that require quick execution.
Common Misconceptions
Many misunderstandings arise around Git and JIT. For instance, some might confuse Git as a means of program execution or view JIT merely as a version control feature. Clarifying these points ensures programmers understand how to employ each tool effectively.
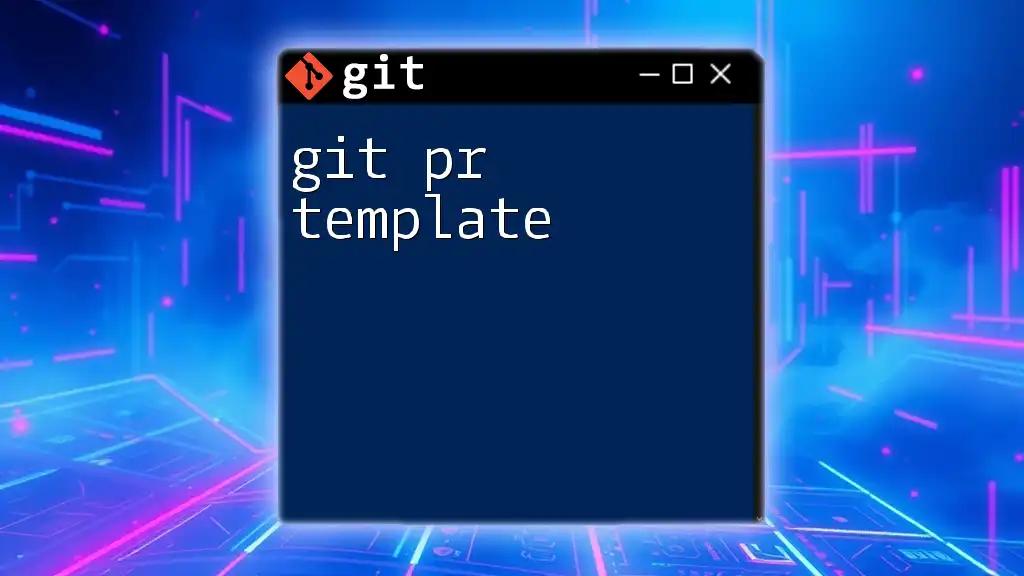
Conclusion
In summary, understanding both Git and JIT equips developers with the right tools to enhance their workflow and optimize application performance. As technology evolves, both systems continue to play critical roles in modern software development, reinforcing the necessity of mastery in both areas. Whether you’re managing versions with Git or optimizing code execution with JIT, exploring these technologies will unlock new levels of efficiency and innovation in your projects.
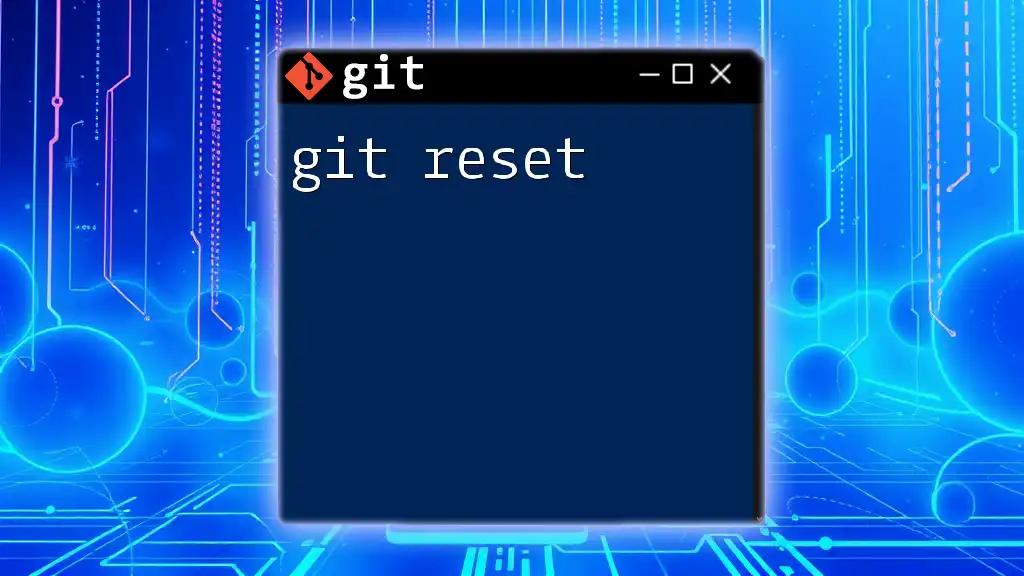
Resources
The journey to mastering Git and understanding JIT is supported by an abundance of resources:
- Helpful Links: Dive into the [official Git documentation](https://git-scm.com/doc).
- Recommended Tools: Explore Git GUI tools and JIT-enabled IDEs to streamline your workflow.
Embrace this knowledge as you continue your coding adventures!