The `git check-ignore` command is used to determine whether specific files are ignored by your `.gitignore` file, helping you manage version control effectively.
Here's a code snippet demonstrating its use:
git check-ignore -v path/to/your/file.txt
What is `git check-ignore`?
Definition
`git check-ignore` is a command in Git that allows developers to check whether specific files are ignored according to the rules defined in the `.gitignore` file. This command is essential for debugging and managing ignored files, ensuring that the correct files are included in version control and that no unintended files are added to the repository.
Common Use Cases
-
Identifying ignored files during development: Sometimes, developers may find that certain files do not appear in their Git status. Using this command helps them verify if those files are intentionally ignored due to .gitignore settings.
-
Verifying that files are correctly ignored: If a developer is unsure whether a file should be ignored, `git check-ignore` can be a quick way to review the current ignore rules and file status.
-
Resolving conflicts with unintended file tracking in Git: If files are tracked despite being intended as ignored, `git check-ignore` can help diagnose the issue.

How to Use `git check-ignore`
Basic Syntax
The basic structure of the command is straightforward:
git check-ignore [options] <file_path>
This means you simply need to replace `<file_path>` with the path of the file you wish to check.
Examples
Example 1: Checking a Single File
If you want to check if a specific file, say `.env`, is ignored, you can run the command:
git check-ignore .env
This command will reveal if `.env` is ignored according to your `.gitignore` settings and will return the corresponding rule, if applicable.
Example 2: Checking Multiple Files
To check several files at once, list them after the command:
git check-ignore file1.txt file2.txt
By using this approach, you can verify multiple files in a single command, which is efficient for managing your project.
Using Wildcards with `git check-ignore`
Checking Ignored Files with Patterns
You can leverage wildcard patterns to find ignored files efficiently. For example:
git check-ignore *.log
This command checks all files matching the `.log` pattern, making it useful for quickly identifying log files that should be ignored.
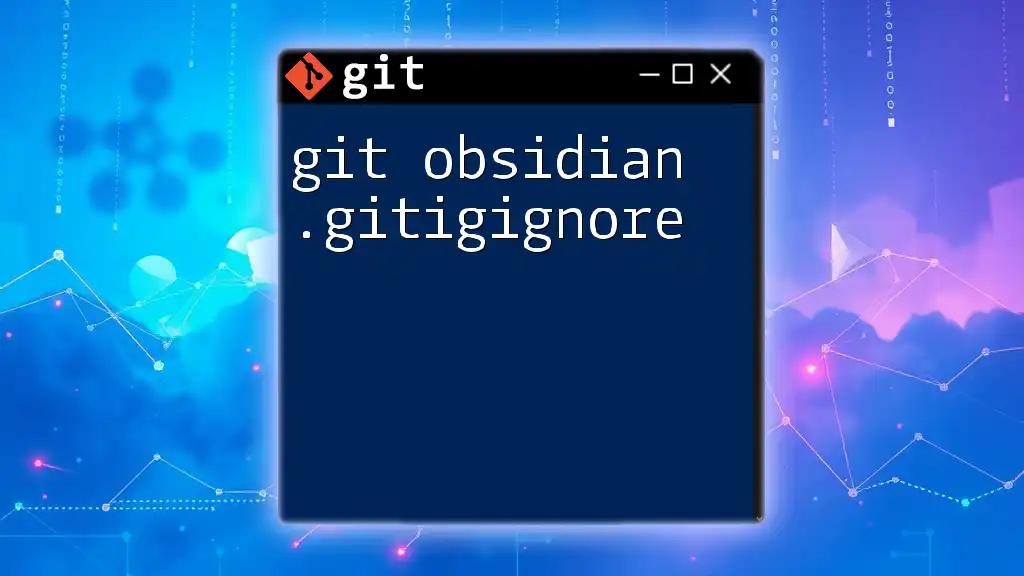
Analyzing Output
Understanding the Results
When you run `git check-ignore`, the output indicates whether a file is ignored and can sometimes include the specific rule that causes the file to be ignored. For example, if the output shows `.env`, it indicates that this file is indeed ignored. If you see no output, that means the file is not ignored based on the current configuration.
Error Messages
When using `git check-ignore`, you may encounter common error messages such as "fatal: pathspec 'filename' did not match any files." This typically means the specified file does not exist in the working directory or has been incorrectly typed, highlighting the need for accurate file paths when using this command.
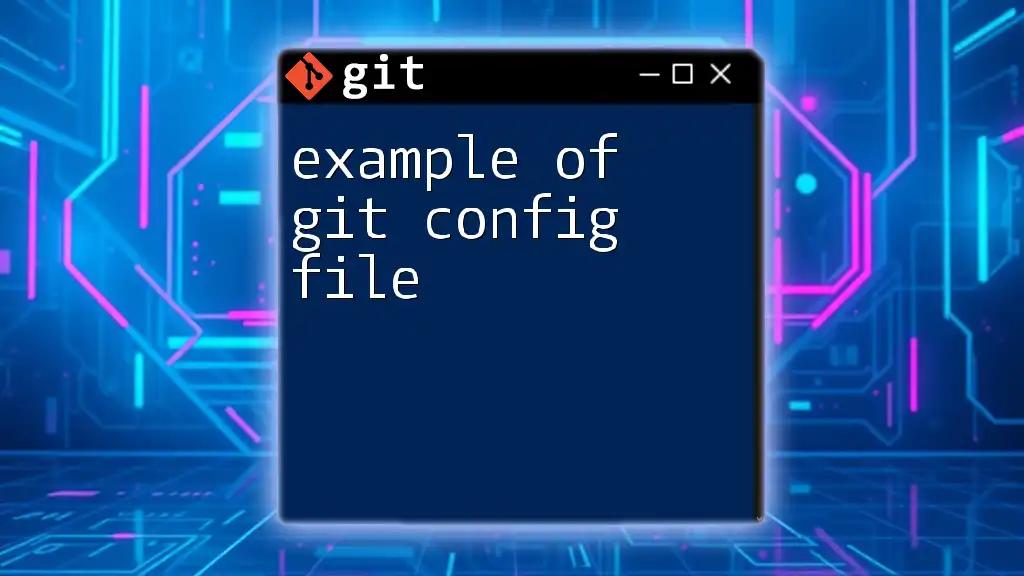
Options and Flags for `git check-ignore`
Overview of Available Options
The command also has several helpful flags to customize its behavior:
-
`-v` (verbose mode): This option provides detailed output showing exactly which ignore rule is causing a specific file to be ignored.
-
`-n` (don’t actually check for ignore): With this flag, Git will output what would be ignored without actually checking. This can be useful for troubleshooting ignore configurations without affecting the repository state.
Practical Examples
Verbose Output
To use the verbose option, you would run:
git check-ignore -v .env
This command outputs not only whether `.env` is ignored but also which line in the `.gitignore` file is responsible for that decision, allowing for a deeper understanding of the ignore rules in play.
Excluding Certain Files
You can also exclude specific patterns from your check using:
git check-ignore -n file_to_check --exclude=*.log
In this case, the command will show you whether `file_to_check` would be ignored while excluding any `.log` files from consideration.
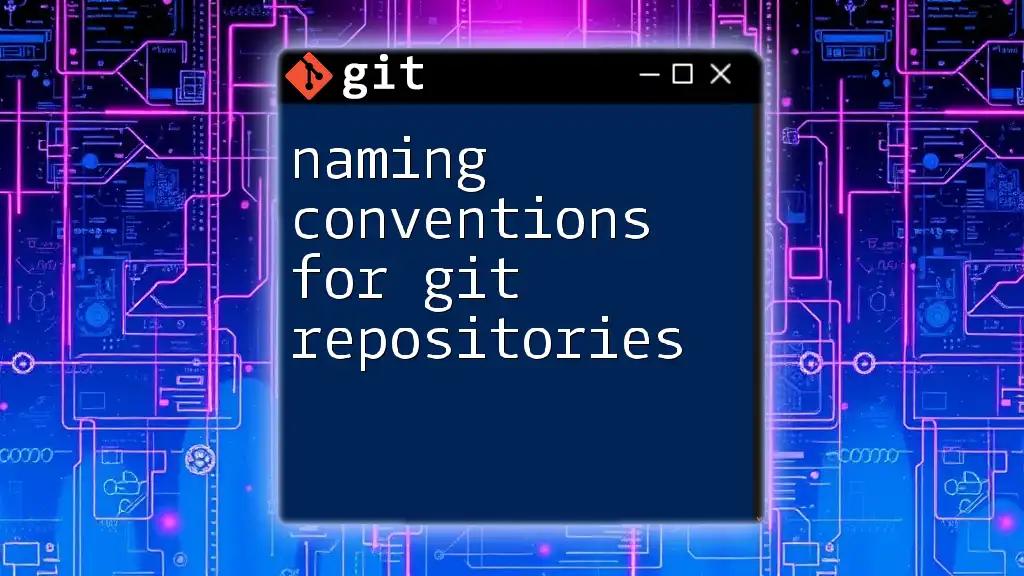
Real-World Application Scenarios
Scenario 1: Working in Teams
When multiple developers collaborate on a project, it's crucial to maintain consistent ignoring rules across the team to avoid issues. Using `git check-ignore`, teams can verify that common files such as log files or configuration files, which should not be included in the repository, are correctly ignored according to the shared `.gitignore` file.
Scenario 2: Open Source Contributions
Contributing to open source projects often comes with the challenge of dealing with ignored files. As contributors submit their changes, developers can utilize `git check-ignore` to verify how external files interact with their local ignore settings. This is important in maintaining a clean and manageable repository.

Conclusion
In summary, the `git check-ignore` command is an invaluable tool for developers working with Git. Its ability to quickly identify and diagnose ignored files ensures clarity and consistency in version control processes. By integrating this command into your workflow, you can minimize potential issues and enhance your Git experience. Remember that effective use of `.gitignore` paired with `git check-ignore` can lead to a more organized and efficient development environment.

Additional Resources
For further learning, you can check the [official Git documentation](https://git-scm.com/doc) to dive deeper into ignore rules and discover best practices for managing your `.gitignore` file. Additionally, keep an eye out for our upcoming classes designed to empower you with efficient Git command mastery!