The `git ls-tree` command allows you to view the contents of a specific commit or tree object in a concise format, showing the file types, modes, and object IDs.
git ls-tree -l <commit_hash>
Understanding `git ls-tree`
What is `git ls-tree`?
`git ls-tree` is a command used in Git that retrieves and displays the contents of a tree object, allowing users to explore the structure of a repository. Essentially, it provides a snapshot of the repository's file structure at a specific point in time, known as a commit. This command is particularly useful for developers looking to inspect how the files and directories are organized without necessarily checking them out.
Importance of `git ls-tree`
Understanding `git ls-tree` is vital for navigating complex repositories effectively. This command serves several purposes, including:
- Exploring Commit Histories: Developers can see which files were present in a specific commit, making it easier to understand changes over time.
- Debugging: By quickly inspecting file structures, developers can resolve issues related to file locations.
- Auditing: Teams can verify the integrity of their repository by checking that the appropriate files exist in various branches or commits.
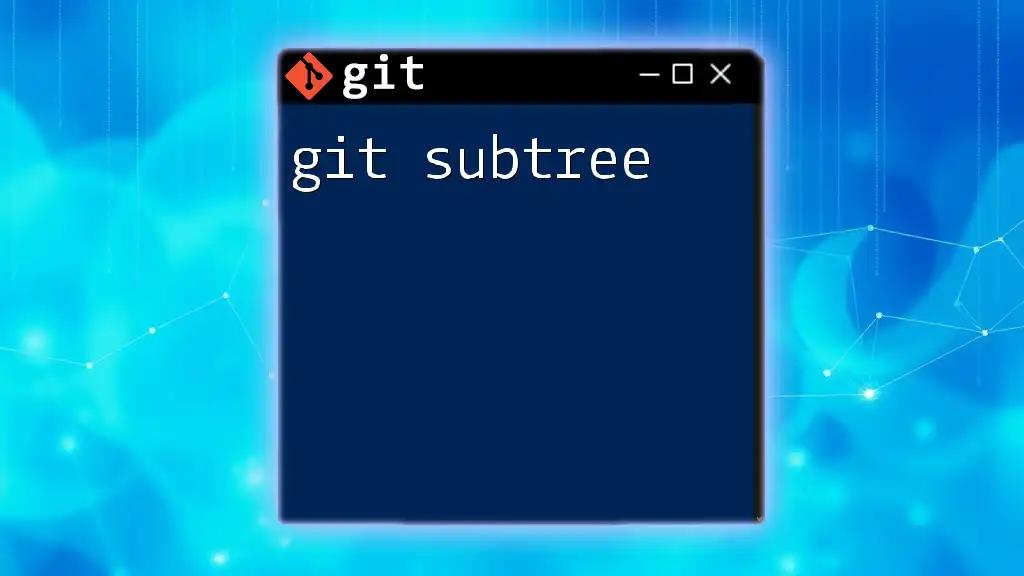
Basic Syntax of `git ls-tree`
Command Structure
The basic syntax of the `git ls-tree` command is as follows:
git ls-tree [options] <tree-ish> [path]
Here’s a breakdown of the syntax:
- `[options]`: Various flags that modify the behavior of the command.
- `<tree-ish>`: Refers to a specific commit, branch, or tag. Common examples include `HEAD` for the latest commit or any specific commit hash.
- `[path]`: An optional argument that allows you to specify a subdirectory within the tree that you want to inspect.
Common Options
`-l` option
The `-l` option lists detailed information about each file, such as file size. This can help users understand the nature of the files they are working with. An example of using this option looks like this:
git ls-tree -l HEAD
When you run the above command, it will return a tree structure of the latest commit along with the sizes of each file.
`-r` option
The `-r` option allows users to list the contents of the tree recursively, meaning it will display all files and folders within subdirectories. This is especially useful when exploring large or nested directory structures. An example command is:
git ls-tree -r HEAD
This command retrieves and displays all files in the latest commit, regardless of how deeply nested they are.
`-t` option
The `-t` option can be used to ensure that only directories (trees) are listed, ignoring files. For instance:
git ls-tree -t HEAD
This will show all subdirectories at the commit pointed to by `HEAD`, providing a clearer view of the structure without the clutter of individual files.
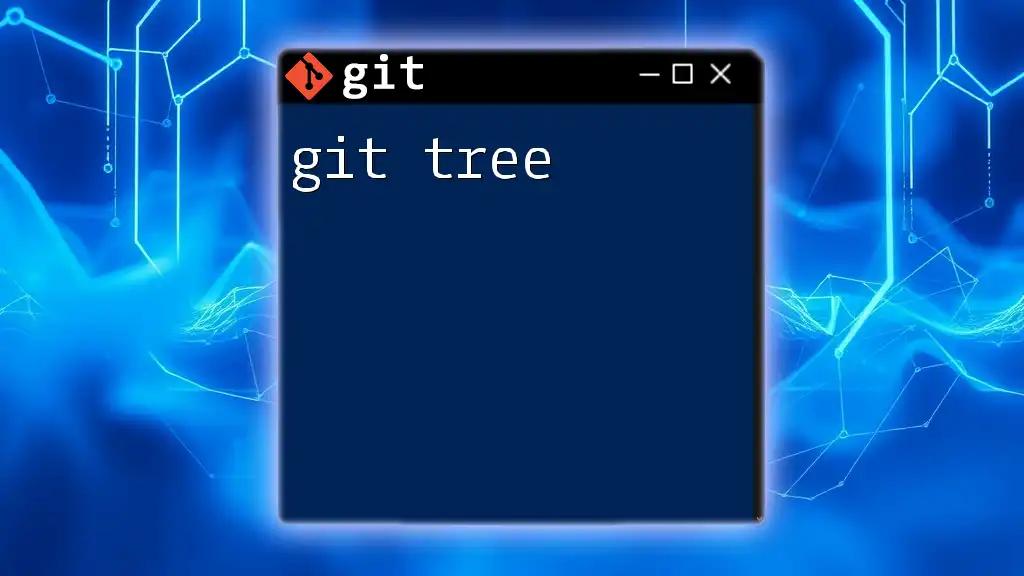
Practical Examples of `git ls-tree`
Viewing the Current Commit Tree
To view the tree structure of the current commit, simply use:
git ls-tree HEAD
Running this command will return a list of all the files in the latest commit, along with their mode, type, hash, and file names. Each entry reflects key information, such as whether the object is a file or a directory.
Exploring a Specific Commit
If you want to see the state of the repository at a specific commit, use the commit hash:
git ls-tree <commit-hash>
This command is particularly valuable for pinpointing the structure during crucial points in your project’s history. Replace `<commit-hash>` with the actual hash to see the contents of that commit.
Listing Objects in a Subdirectory
To examine a specific subdirectory, use:
git ls-tree HEAD:path/to/subdirectory
This command will show you only the files and directories located within the specified subdirectory at the current commit. It allows for targeted exploration within large projects.
Viewing All Branches
To check trees across branches, employ the following command:
git ls-tree -r --full-tree <branch-name>
This command provides a comprehensive view of all files in the specified branch, facilitating an understanding of its entire file structure. Ensure to replace `<branch-name>` with the name of the branch you wish to inspect.
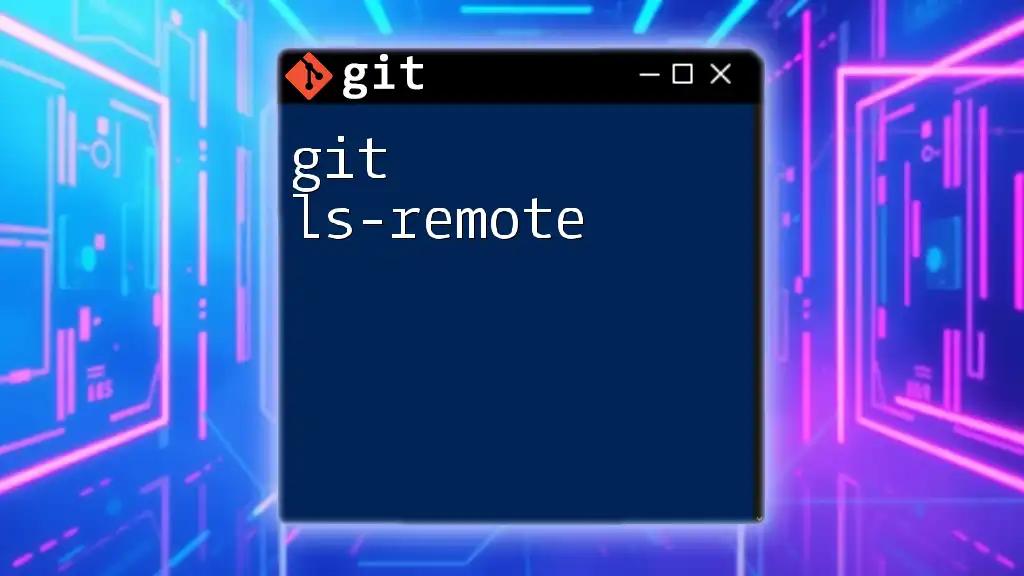
Advanced Usage of `git ls-tree`
Using with Other Git Commands
Combining with `git checkout`
You can combine the results of `git ls-tree` with `git checkout` to fetch specific files from a commit without checking out the entire commit. For example:
git checkout <commit-hash> -- <path>
This command checks out a specific file or directory from an earlier commit, allowing easy access to past versions of files.
Integrating with Scripting
For users looking to automate their workflows, `git ls-tree` can be integrated into scripts. You can use it to programmatically check for the existence of files or to generate reports on the repository structure. An example of a simple script might look like this:
#!/bin/bash
git ls-tree -r HEAD | awk '{print $4}'
This script lists all the file paths in the latest commit, which can be useful for logging or auditing purposes.
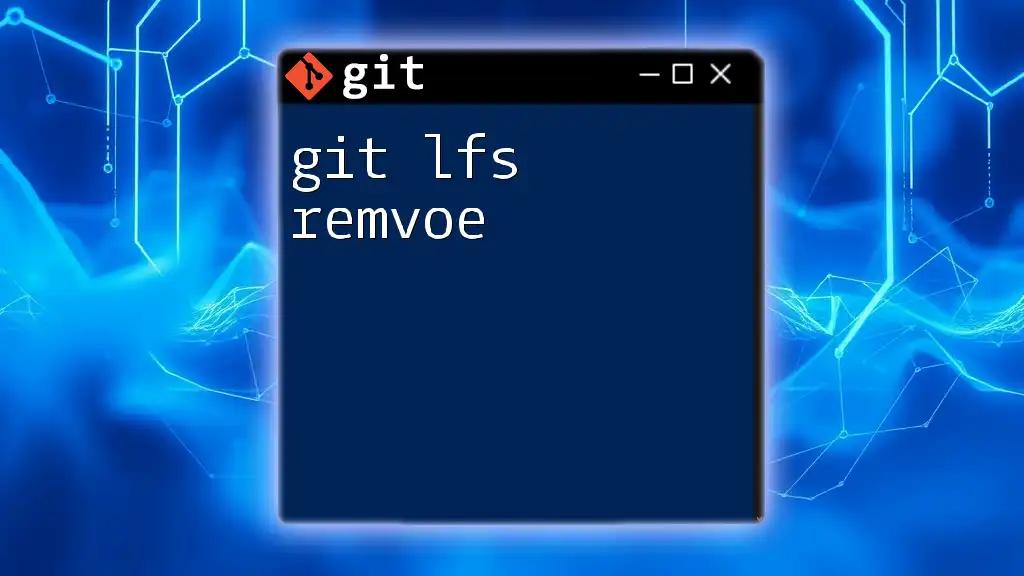
Conclusion
In summary, mastering the `git ls-tree` command is essential for anyone looking to navigate and understand their Git repositories more effectively. It not only helps you explore the structure of your projects but also enhances your ability to handle changes and track history. Regular practice with this command will boost your efficiency as a developer and improve your Git skills significantly.
For further mastery of Git, refer to the official Git documentation, explore recommended books, or take online courses that delve deeper into version control concepts.