In this project, we will demonstrate a Continuous Integration and Continuous Deployment (CI/CD) pipeline utilizing Git for version control, Jenkins for automation, Ansible for configuration management, and Kubernetes for container orchestration.
# Example Jenkins pipeline script to build and deploy a Docker container
pipeline {
agent any
stages {
stage('Build') {
steps {
script {
git 'https://github.com/your-repo.git'
sh 'docker build -t your-image-name .'
}
}
}
stage('Deploy') {
steps {
script {
sh 'ansible-playbook -i inventory/hosts deploy.yml'
}
}
}
}
}
Understanding the Tools
Git
Git is a distributed version control system that allows multiple developers to work on a project simultaneously without conflicting changes. It’s known for its efficiency, flexibility, and strength in handling various development workflows.
The benefits of using Git for version control include:
- Branching and Merging: Git allows you to create branches easily, enabling isolated environments to work on features or fixes without affecting the main codebase. Once it's tested, you can merge it back in.
- Commit History: Every change is recorded, providing a clear history of the changes made over time. This is essential for auditing and understanding when and why changes were made.
- Collaboration: Multiple developers can work on the same repository, facilitating teamwork while minimizing integration problems.
For newcomers, here are some basic Git commands to get started:
git init
git clone <repository-url>
git add <file>
git commit -m "Your commit message"
git push origin <branch-name>
Jenkins
Jenkins is an open-source automation server that facilitates continuous integration and continuous delivery (CI/CD) in software development. It integrates with various tools to automate building, testing, and deploying applications.
Key features of Jenkins include:
- Plugins: Jenkins offers a plethora of plugins that allow integration with a wide range of tools and services, enhancing its capabilities.
- Declarative Pipeline: You can define your CI/CD pipeline as code using a `Jenkinsfile`, which outlines how software is built, tested, and deployed.
- Distributed Builds: Jenkins can run jobs across multiple machines, allowing complex builds to run concurrently.
To integrate Jenkins with Git, you need to configure a new Jenkins job that pulls the code from your Git repository whenever changes are detected.
Ansible
Ansible is an open-source automation tool that simplifies the processes of configuration management, application deployment, and task automation. Its agentless architecture makes it easy to use across any environment.
The advantages of using Ansible include:
- Simplicity: Ansible uses simple YAML configuration files (playbooks) that are easy to read and write, streamlining development and operations.
- Idempotency: Ansible ensures that repeated tasks yield the same result without unwanted side effects, making systems more reliable.
- Community Support: Ansible has a strong open-source community that provides a wealth of modules, playbooks, and support.
Basic Ansible commands to be familiar with include creating playbooks and executing them:
ansible-playbook deploy.yml
Kubernetes
Kubernetes is an open-source platform designed for orchestrating containerized applications across a cluster of machines. It maintains the desired state for applications, enabling automatic deployments, scaling, and management of containers.
The benefits of using Kubernetes in a CI/CD pipeline include:
- Scalability: Kubernetes can scale up or down based on traffic demand, accommodating different workloads efficiently.
- Self-healing: It automatically replaces failed containers, ensuring your applications run smoothly.
- Operational Efficiency: Container orchestration helps to manage deployment complexities, improving resource utilization.
Key components of Kubernetes include Pods, Services, and Deployments, which together manage containerized applications effectively.
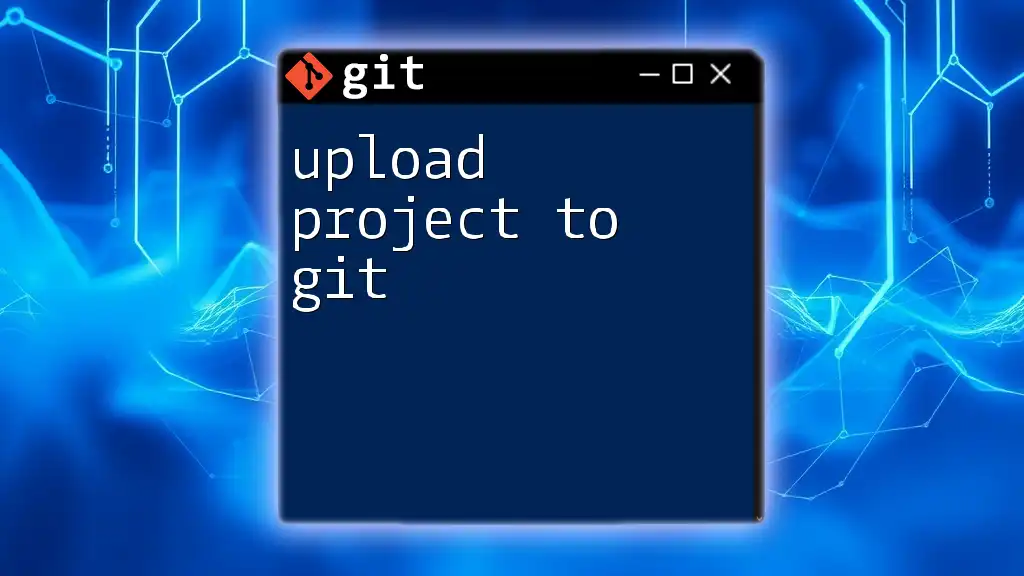
Setting Up Your Development Environment
Installing Git
To begin with Git, you need to install it on your local system. The installation process varies by operating system:
-
Windows: Download the installer from the official Git website and follow the installation steps.
-
MacOS: Use Homebrew to install Git:
brew install git
-
Linux: Use the package manager for your distribution:
sudo apt-get install git
After installation, set up your Git configuration with your name and email:
git config --global user.name "Your Name"
git config --global user.email "you@example.com"
Setting Up Jenkins
Jenkins can be installed on various platforms, including Windows, MacOS, and Linux. Here's a simplified installation guide:
- Download and Install Jenkins: Go to the official Jenkins website and download the installer. Follow the instructions provided for your specific operating system.
- Initial Setup: Once installed, open Jenkins in your web browser. You will be prompted to enter the initial admin password, which can be found in the installation directory.
- Plugin Configuration: After the initial setup, install necessary plugins for Git, Ansible, and Kubernetes integration.
To connect Jenkins with your Git repository, create a new job and select "Freestyle project." In the job configuration, specify the Git repository URL and authentication details.
Installing Ansible
Installing Ansible is straightforward. You can install it as follows:
-
Using pip:
pip install ansible
-
On Ubuntu:
sudo apt-get update sudo apt-get install ansible
After installation, create an inventory file that contains the hosts you will manage with Ansible.
Setting Up Kubernetes
To set up Kubernetes, you can use Minikube for local development or a managed Kubernetes service from a cloud provider.
For Minikube:
-
Install Minikube by following the instructions on the official Minikube website.
-
Start Minikube:
minikube start
-
Install kubectl to interact with your Kubernetes cluster:
curl -LO "https://storage.googleapis.com/kubernetes-release/release/$(curl -s https://storage.googleapis.com/kubernetes-release/release/stable.txt)/bin/linux/amd64/kubectl" chmod +x ./kubectl sudo mv ./kubectl /usr/local/bin/kubectl
-
Check the cluster status:
kubectl cluster-info
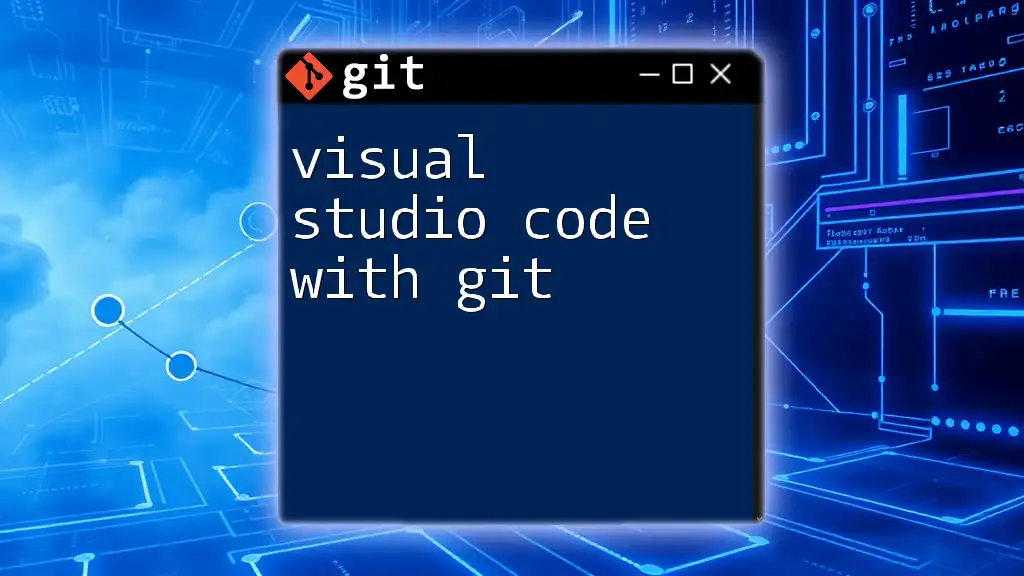
Creating a Simple CI/CD Pipeline
Setting Up the Git Repository
Begin by setting up a sample project repository. Use the following commands to create a Git repository locally:
mkdir sample-devops-project
cd sample-devops-project
git init
Add some simple application code, and commit it:
echo "# Sample DevOps Project" >> README.md
git add README.md
git commit -m "Initial commit"
Configuring Jenkins for CI/CD
To create a Jenkins job:
- Create a New Job: Go to Jenkins dashboard and click on “New Item.” Enter a name for your project and select “Freestyle project.”
- Configure Source Code Management: Under “Source Code Management,” select “Git,” and provide your repository URL.
- Build Triggers: Enable “Poll SCM” to automatically check for changes in your Git repository.
Here's a simple pipeline in Jenkins defined through a `Jenkinsfile`:
pipeline {
agent any
stages {
stage('Build') {
steps {
sh 'echo Build Step'
}
}
stage('Deploy') {
steps {
sh 'echo Deploy Step'
}
}
}
}
Using Ansible for Deployment
Create a simple Ansible playbook called `deploy.yml`. Here’s an example playbook:
---
- name: Deploy Application
hosts: all
tasks:
- name: Ensure Application is installed
apt:
name: myapp
state: present
To execute the playbook, use the following command:
ansible-playbook -i inventory deploy.yml
Deploying with Kubernetes
The next step is creating your deployment YAML file. Here’s an example of how you might define a simple application deployment:
apiVersion: apps/v1
kind: Deployment
metadata:
name: sample-app
spec:
replicas: 2
selector:
matchLabels:
app: sample-app
template:
metadata:
labels:
app: sample-app
spec:
containers:
- name: sample-app
image: your-docker-image
ports:
- containerPort: 8080
To apply this deployment in your Kubernetes environment, use:
kubectl apply -f deployment.yaml
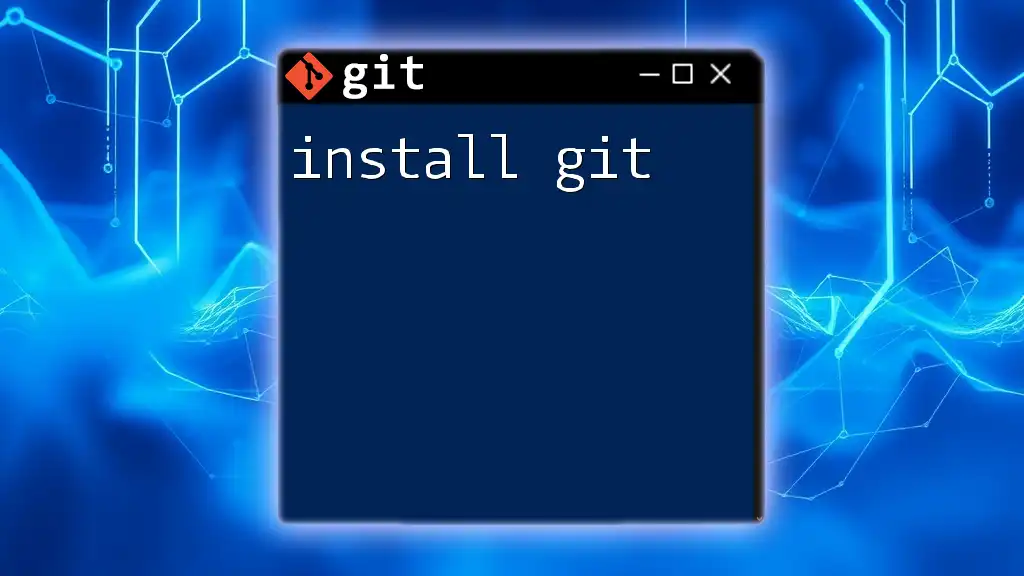
Practical Example: Implementing a CI/CD Pipeline
Project Overview
Let’s create a simple web application using Flask and deploy it through the CI/CD pipeline built with Git, Jenkins, Ansible, and Kubernetes.
Step-by-Step Implementation
- Write Your Application Code: Develop your Flask application locally and commit it to the Git repository.
- Set Up Jenkins: Configure Jenkins to pull the latest code from Git and run builds every time changes are pushed.
- Deploy Using Ansible: Write Ansible playbooks to configure the server where the application will run.
- Kubernetes Deployment: Finally, create Kubernetes Pods and manage the scaling of your application using the earlier defined deployment YAML.
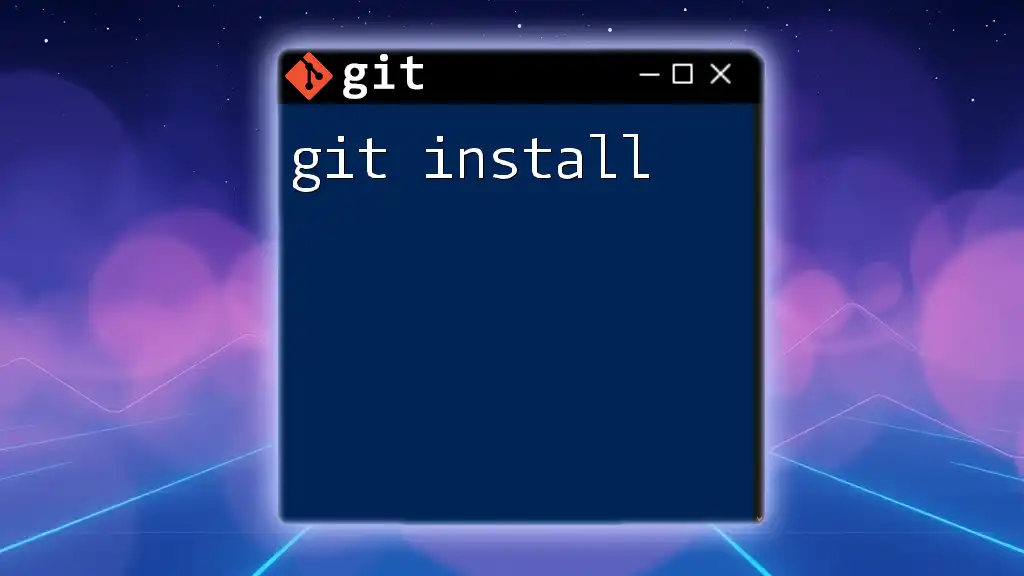
Troubleshooting Common Issues
Git Issues
Common Git Errors: You may face issues such as merge conflicts while collaborating with team members. To resolve them:
- Identify Conflicts: Git will indicate files with conflicts. Open these files in a text editor to manually merge changes.
- Use Commands:
git add <resolved-file>
git commit -m "Resolved merge conflicts"
Jenkins Troubleshooting
Common Build Issues: If your Jenkins build fails:
- Check Console Output: Look at the build console for detailed error messages.
- Check Permissions: Ensure Jenkins has the necessary permissions to access your Git repository.
Ansible and Kubernetes Errors
Handling Deployment Failures: If an Ansible playbook fails:
- Review Logs: Examine the output logs to understand what went wrong.
- Debug: Use the `-vvv` flag when running your playbook for verbose output, which helps in troubleshooting.
For Kubernetes, check pod statuses:
kubectl get pods
kubectl describe pod <pod-name>
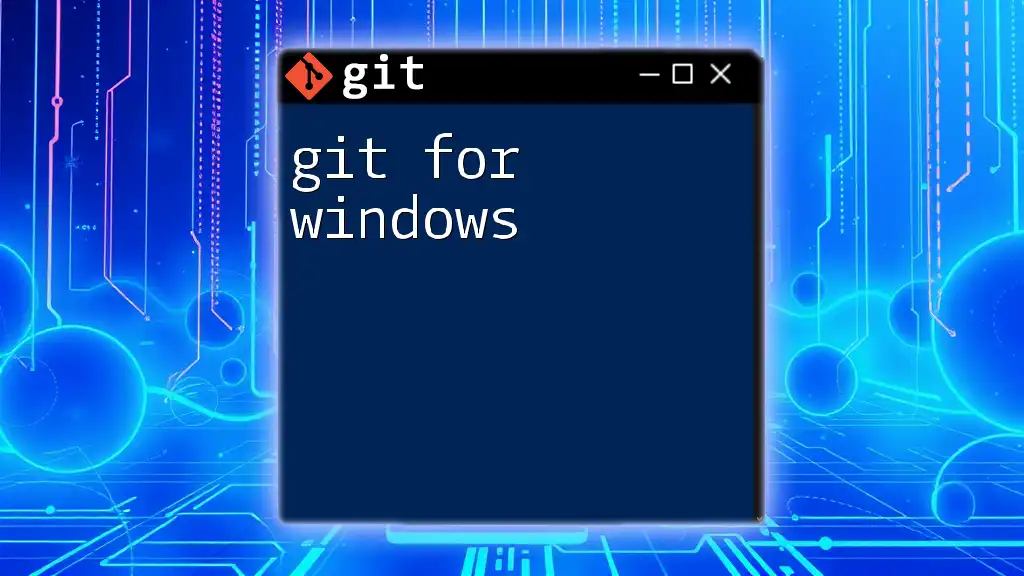
Best Practices for CI/CD in DevOps
Version Control Practices
Commit Messages: Adopting a standardized format for commit messages improves collaboration. Consider using the following structure:
- Type: (feat, fix, docs, style, refactor, test)
- Scope: A brief scope (module name)
- Description: A concise description of what changed.
Use branches effectively. Implement a branching strategy like Git Flow or trunk-based development for better management.
Jenkins Optimization Techniques
- Pipeline as Code: Utilize `Jenkinsfile` to define and version control your CI/CD pipeline.
- Security: Protect your Jenkins instance and credentials by implementing user authentication and authorization.
Ansible Roles and Playbook Organization
Structure your playbooks for better maintainability:
- Use Roles: Group related tasks and variables into roles for reusability.
- Version Control: Keep your playbooks and roles versioned in a Git repository for better collaboration.
Managing Kubernetes Resources Efficiently
- Resource Management: Configure resource limits and requests in your deployments to optimize resource utilization.
- Environment Separation: Use different namespaces for staging and production environments to avoid potential conflicts.
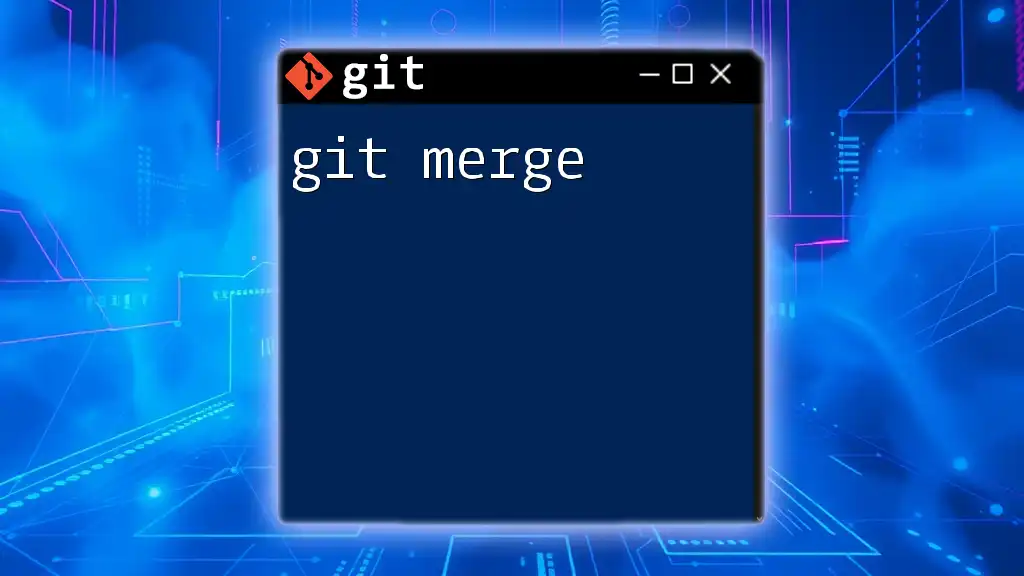
Conclusion
Throughout this guide on your DevOps Project 1 - CI/CD with Git, Jenkins, Ansible, and Kubernetes, you've learned how to leverage these powerful tools to automate and streamline your software development lifecycle. By practicing these implementations, you will enhance your understanding of CI/CD processes in real-world scenarios. As you proceed, continue exploring community resources and documentation to stay updated and expand your skill set in these technologies.