When you perform a `git pull` and want to incorporate changes from the remote branch without creating a merge commit, you can use fast-forward merging, which means your local branch pointer is moved forward to match the remote branch.
Here's how you can do it:
git pull --ff
Understanding Git Pull
What is a Git Pull?
A git pull is a command used to update your local repository with changes from a remote repository. Essentially, it combines two essential operations: fetching changes from the remote repository and merging those changes into the local branch. This makes it particularly useful in collaborative environments where multiple contributors continually push their changes.
It's crucial to differentiate between `git pull` and `git fetch`. While `git fetch` downloads the changes without merging them, `git pull` does both—downloading and integrating changes automatically.
Components of Git Pull
The operation of `git pull` involves three main components:
- Remote Repositories: These are versions of your project stored on a server. Generally, this could be GitHub, Bitbucket, or any other git hosting service.
- Local Branches: Your working environment—this is where you code and make local changes before they are pushed back to the remote.
- Merging Changes: Once the changes are fetched, they are either automatically merged, or you have the option to resolve any conflicts that may arise during the merge process.
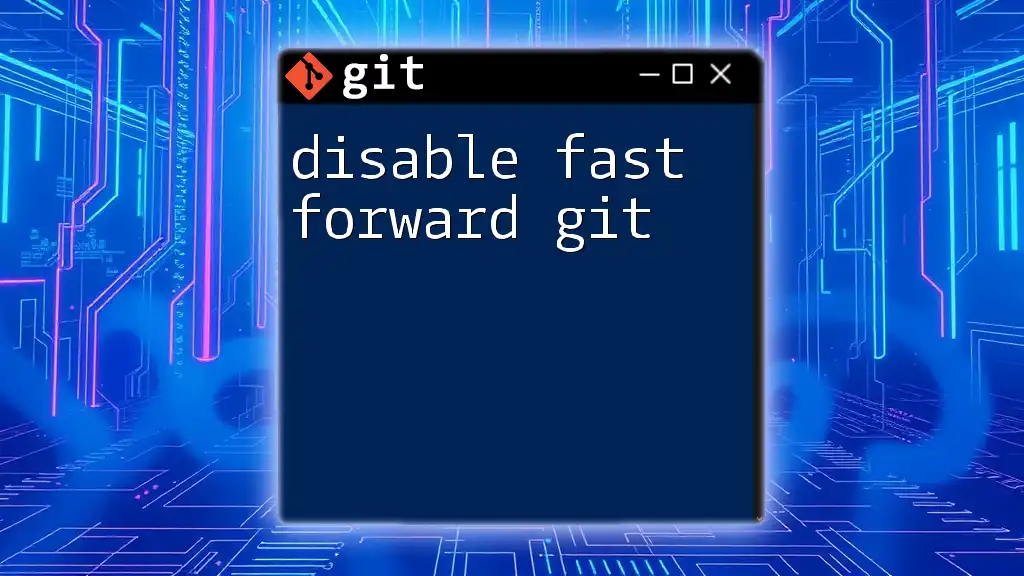
The Fast-Forward Merge Explained
Definition of a Fast-Forward Merge
A fast-forward merge occurs when the current branch's head is directly behind the commit history of the branch being merged. In this case, Git only moves the pointed reference (the head) forward to the latest commit. This leads to a linear history without creating additional merge commits.
Imagine you have the following commit history:
A---B---C (main)
\
D---E (feature-branch)
If you're on the main branch and you perform a `git pull` on the feature branch after D and E were added, a fast-forward is possible since the main branch hasn't moved ahead.
Scenarios Leading to a Fast-Forward Merge
When Fast-Forward is Available
Here's a simple example where a fast-forward can occur:
git checkout main
git pull origin feature-branch
In this situation, as long as no new commits were made on the `main` branch after commit `C`, the merge can proceed without creating a new commit.
Visualizing the Branch Comparison
It can be helpful to visualize branch comparisons before and after a pull operation. Imagine the branching diagram above. After a successful fast-forward merge, your history would look like this:
A---B---C---D---E (main)
Should You Fast Forward?
Advantages of Fast-Forward Merges
Choosing to perform a fast-forward merge comes with several advantages:
- Clean Commit History: Fast-forward merges keep the history straightforward, allowing for simpler navigation and debugging.
- Simple Reversion: If a change needs to be reversed, it’s easier with a linear history."
- Easier to Understand Project Evolution: New collaborators can quickly ascertain what changes have been made without sifting through unrelated commit merges.
For instance, before and after the fast-forward, your commit history is far cleaner:
# Before
A---B---C (main)
\
D---E (feature-branch)
# After fast-forward
A---B---C---D---E (main)
Disadvantages of Fast-Forward Merges
However, there are disadvantages as well, notably:
- Losing Contextual Boundaries: Fast-forward merges remove the distinct separation that is often valuable when tracking features or fixing bugs.
- Potential Confusion in Collaboration: In collaborative scenarios, relying solely on fast-forward can lead to ambiguity about when a feature was introduced. For example, if multiple features are merged back, it becomes less clear how they interrelate.
Consider this misleading scenario:
# After numerous fast-forward merges without context
A---B---C---D---E---F (main)
It can become challenging to track what feature committed `F` represents.
When to Choose Fast-Forward
Fast-forward merges are particularly beneficial in the following situations:
- Working Alone on a Feature Branch: When you’re isolated and there’s no risk of conflicting changes, a fast-forward merge is a straightforward choice.
- Keeping a Linear History: If your team prefers to maintain a clean project trajectory without interlacing merges, going for fast-forward is ideal.
To enforce fast-forward only, you can use:
git config --global pull.ff only
When Not to Choose Fast-Forward
Conversely, a non-fast-forward strategy may be more suitable in scenarios involving:
- Complex Team Collaborations: When multiple contributors are working on different branches, maintaining the contextual boundaries provided by regular merges is essential.
- Multi-Branch Workflows: In these cases, it's often useful to see when features were integrated back into the main codebase.
To prevent fast-forward merges, you can run:
git config --global pull.ff false
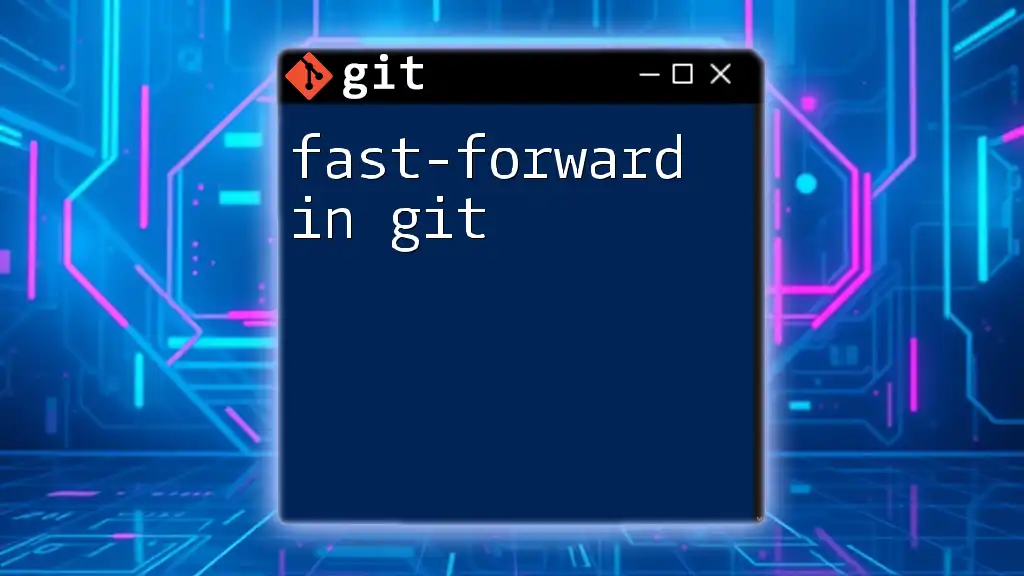
Best Practices for Using Git Pull with Fast-Forward
Configuring Your Git Environment
Ensure that your Git environment is set up according to your team's preferences regarding fast-forward merges. Here are a few commands for this:
- To enforce fast-forward only:
git config --global pull.ff only
- To ensure non-fast-forward merges:
git config --global pull.ff false
Communication with Your Team
The importance of clear communication cannot be overstated. Discuss with your team members the merging strategies that work best for your collaborative environment. Establish consistent guidelines so that everyone is on the same page and aware of when to use fast-forward merges versus standard merges.
Case Studies of Fast-Forward vs Non-Fast-Forward
Real-world scenarios can often illustrate the importance of these merging strategies. For instance, a programming team that frequently opted for fast-forward merges may have initially enjoyed a clean commit history but later found it challenging to track the feature development leading to confusion during regression testing.
In contrast, teams that leveraged a hybrid approach—occasionally using non-fast-forward merges—often cited enhanced clarity and a more nuanced understanding of code evolution. By evaluating these experiences, your team can potentially select an approach tailored to your development structure and workflows.
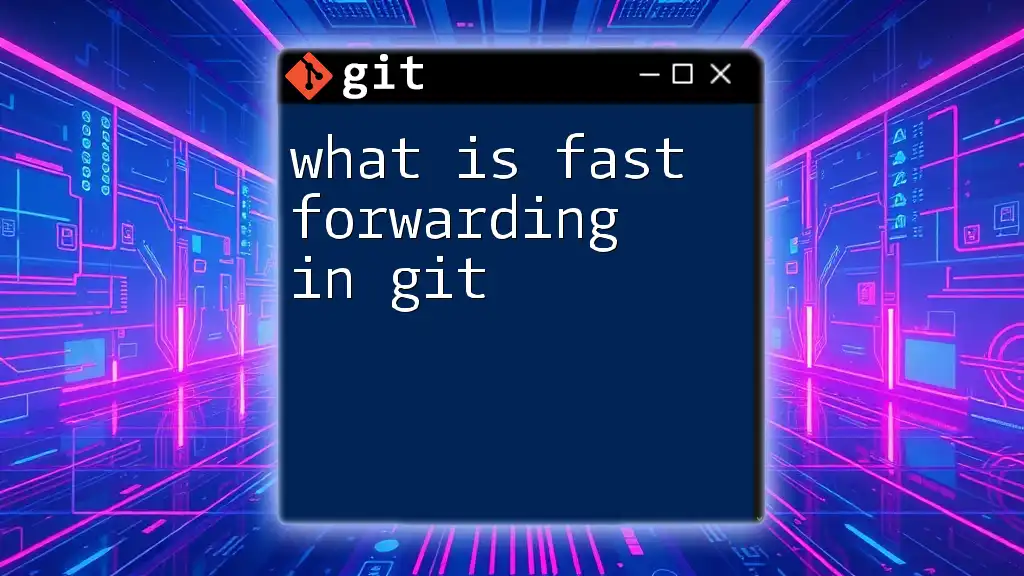
Conclusion
In sum, whether or not you should fast forward for git pull depends significantly on your project's needs and your team's collaboration style. A fast-forward merge offers a clean history and simplicity but can obscure important context in collaborative settings.
Taking the time to assess your project needs, team dynamics, and best practices can lead to more efficient version control management in your Git projects. Always remember, the choice lies in understanding the merits of fast-forward merges and being adaptable to the needs of your development environment.
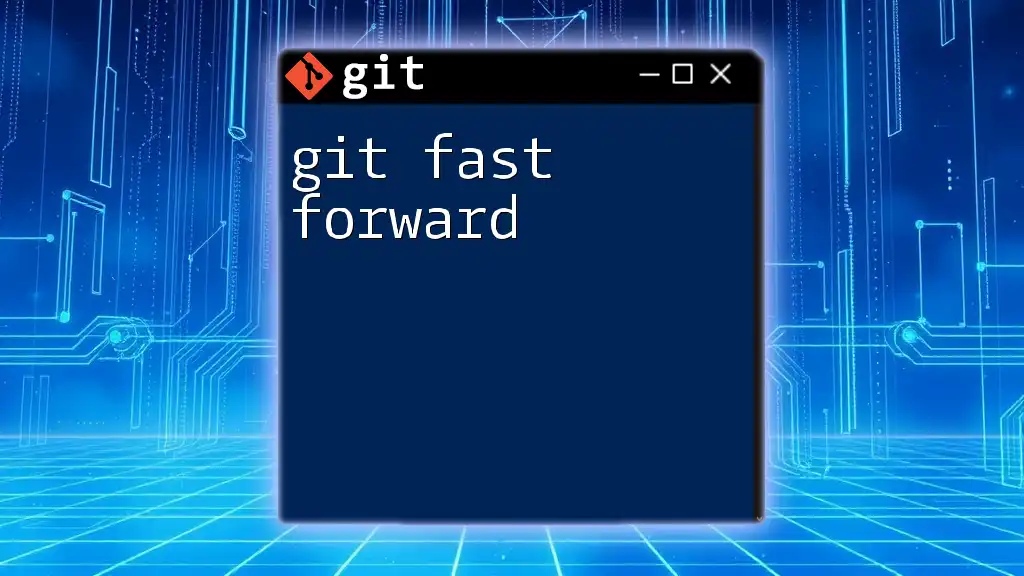
Additional Resources
To deepen your understanding of Git and merging strategies, consult the following resources:
- The official Git documentation for comprehensive coverage of commands and functionalities.
- Books and online courses focused on mastering Git fundamentals and advanced strategies.
- Join community forums and platforms to engage in discussions and gain insights from peers.