To change the default behavior of `git pull` to always use rebase instead of merge, you can set the configuration variable `pull.rebase` to `true` using the following command:
git config --global pull.rebase true
Understanding `git pull`
What is `git pull`?
The `git pull` command is a crucial tool in Git, responsible for synchronizing your local repository with a remote one. This command effectively combines two fundamental operations: fetching and merging. When you execute `git pull`, it first fetches the changes from the specified remote repository and then attempts to merge those changes into the current local branch.
Default Behavior of `git pull`
By default, `git pull` operates under the assumption that you want to integrate the changes from the remote tracking branch into your current branch through a merge. This can lead to complications, especially when working on a shared codebase with multiple collaborators. Understanding how to tailor the behavior of this command can greatly enhance your workflow and reduce potential conflicts.
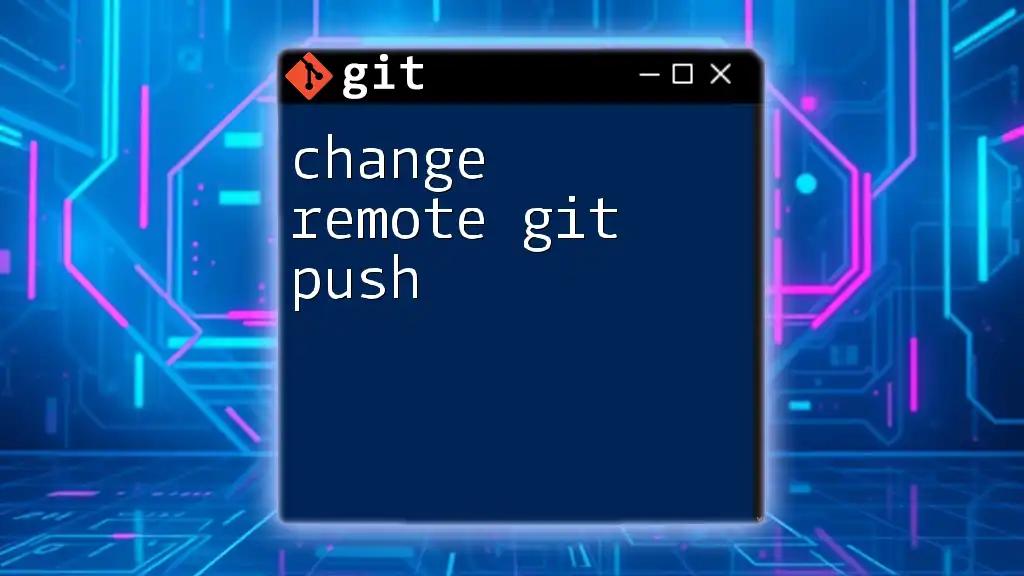
Changing the Default Behavior of `git pull`
Setting Pull Behavior to `rebase`
What is Rebasing?
Rebasing is a powerful feature in Git that allows developers to integrate changes from one branch into another in a linear fashion, making the history much cleaner. By using rebase, commits from the upstream branch are applied onto your local branch without creating a merge commit, which can lead to a more streamlined project history.
How to Change the Default Behavior to Rebase
To change the default behavior of `git pull` to use rebase instead of merge, use the following command:
git config --global pull.rebase true
By configuring this globally, every time you execute `git pull`, it will automatically perform a rebase. This setting can significantly reduce the clutter in your commit history.
Example Workflow
Imagine you are working on a feature branch and several commits were made to the master branch in the meantime. If you run `git pull` without rebase, Git will create a new merge commit that ties together your changes and the ones from the master branch. However, with `git pull --rebase`, your local commits will be reapplied on top of the latest master commits, leading to a cleaner history.
Configuring Pull to Only Fetch
What Does Fetching Alone Mean?
The `git fetch` command downloads changes from the remote repository into your local repository but does not integrate those changes into your current working branch. This allows you to see changes without merging them immediately, giving you time to review and plan your next move.
Changing the Behavior to Fetch
You can configure `git pull` to allow only fast-forward merges without merging any changes directly using:
git config --global pull.ff only
This configuration means that `git pull` will only complete successfully if the local branch can be fast-forwarded to the updated remote branch. If it cannot be fast-forwarded, Git will not perform the merge, prompting you to handle it manually.
Example Workflow
When working on a complex project, there may be a time when you want to inspect incoming changes before merging them into your branch. This fetch-only behavior allows you to pull the latest changes from the remote repository while retaining full control over when and how to integrate those changes into your local working branch.
Ignoring Rebase and Merge Conflicts
Using the `--no-rebase` Flag
In some cases, reverting to the default merge behavior can be beneficial, especially if you anticipate conflicts that are easier to resolve in a traditional merge context. The `--no-rebase` option forces Git to use merging, overriding any global configurations you have set.
Command Example
To ignore a global rebase setting temporarily, you can execute:
git pull --no-rebase
This command will allow for a regular merge even if you've set a global configuration that defaults to rebase.
Managing Configuration on a Repository Level
Local vs Global Configuration
While global configurations are helpful, sometimes you might want to tailor the behavior for just one specific repository. This approach allows you to customize your workflow based on the needs of a particular project.
Example Command
To set the pull behavior for a single repository, use:
git config pull.rebase false
This command makes it unnecessary to use the `--no-rebase` flag in that specific repository, providing indispensable flexibility in your workflow.
Setting Up Different Behaviors for Different Branches
Branch-Specific Configurations
It's possible to assign unique pull behavior to different branches, allowing for tailored workflows suited to specific branches' purposes and contexts.
Code Snippet
For example, to configure rebase for a feature branch:
git config branch.feature-branch.rebase true
By setting branch-specific configurations, you can adopt diverse strategies that enhance your collaboration efforts and maintain a clean project history.
Understanding Merge Strategies
The `--strategy` Option
Git allows various merge strategies to define how merging takes place when conflicts arise. Different strategies can be useful depending on project requirements and team workflows.
How to Implement in Pull Commands
For instance, to use a specific merge strategy during a pull operation, run:
git pull -s ours
This tells Git to prefer your changes in the event of a conflict, rather than merging all changes naturally. This flexibility provides comprehensive control over how changes are integrated into a repository.

Docker and CI/CD Considerations
Impact of Pull Behavior on Docker Builds
In CI/CD pipelines, the behavior of `git pull` can significantly affect the quality and speed of your Docker builds. If you are pulling and merging changes effectively without conflicts throughout development cycles, you create a smoother path for deployments and a predictable build environment.

Best Practices for Customizing Git Pull Behavior
When to Use Rebase vs. Merge
Choosing between rebase and merge largely depends on your project's collaboration style and team preferences. Rebasing is often preferred for its clean linear history, whereas merging captures the mixed history, which might be necessary for preserving context.
Common Pitfalls to Avoid
New users of Git often mismanage pull behaviors leading to unexpected merge commits and conflicts. It's critical to develop a clear understanding of how your pull configurations affect your workflow, as this can ensure a smoother development process.
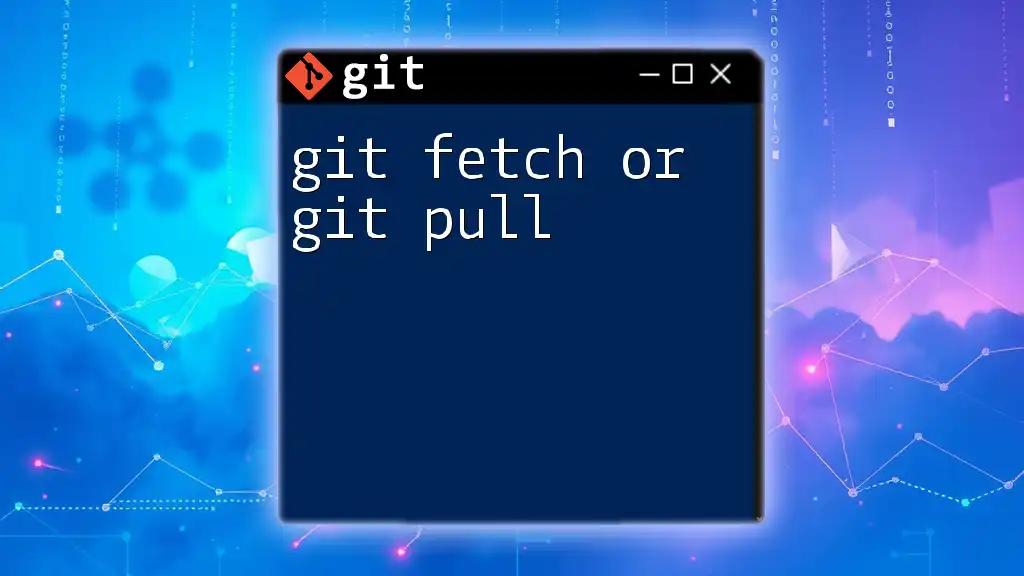
Conclusion
Customizing your Git environment by learning how to change the default behavior of `git pull` is fundamental in achieving an efficient workflow and maintaining a clean project history. The ability to leverage various strategies and configurations enables developers to adapt to different projects dynamically. As you familiarize yourself with these commands and configurations, you'll enhance not only your productivity but also the quality of your code collaborations.
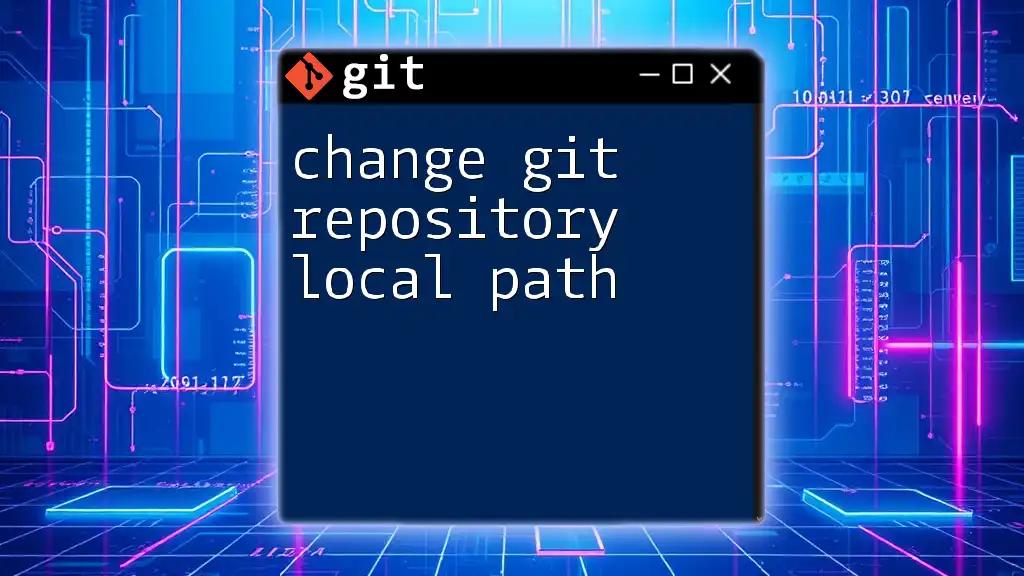
Resources and Further Reading
For further learning materials, you may refer to the [official Git documentation](https://git-scm.com/doc) and [Git's Pro Book](https://git-scm.com/book/en/v2), which provide extensive detail on advanced commands and best practices in version control.