The "git fatal unable to access" error typically occurs when Git cannot access the specified repository URL, often due to incorrect URLs, network issues, or authentication problems.
git clone https://github.com/username/repository.git
Understanding the Error
What Does "Fatal: Unable to Access" Mean?
The message "fatal: unable to access" typically appears when Git encounters difficulties in connecting to a remote repository. This error can prevent you from pushing, pulling, or cloning repositories, which can be frustrating, especially when you’re in the middle of a project.
Common Causes of the Error
Several factors can lead to this error:
- Network connectivity issues: If you are not connected to the internet or if your connection is unstable, Git cannot reach the remote repository.
- Incorrect repository URLs: Typos in the remote repository URL can lead to access failures.
- Authentication problems: Incorrect credentials or SSH keys can prevent access to the repository.
- SSL certificate validation problems: Issues with SSL certificates can hinder HTTPS connections.
- File permission issues: Local file system permissions can block access to necessary files.

Diagnosing the Problem
Checking Your Internet Connection
Before diving deeper, confirm that your internet connection is working correctly. A stable connection is essential for any Git operation involving remote repositories. You can quickly check your connection using the following command:
ping github.com
If you see replies, you're connected. If you receive timeout errors, ensure your network is functioning.
Verifying the Repository URL
Incorrect URLs are a frequent source of error. To inspect your remote repository settings, run:
git remote -v
This command lists all remote repositories linked to your local directory. Ensure that the URLs are correct. If necessary, update them using:
git remote set-url origin https://github.com/username/repo.git
Authentication and Permissions
Authentication can significantly impact your ability to access a repository. Most Git operations require credentials, especially when using HTTPS. If you've opted for SSH, ensure your SSH keys are configured correctly. You can verify this by running:
ssh -T git@github.com
If you see a message confirming that you've successfully authenticated, your keys are set up correctly. If authentication fails, you may need to generate a new SSH key or update your credentials.
Deal With SSL Issues
SSL certificate verification issues can arise, particularly when using HTTPS. If you're encountering errors related to SSL certificates, one quick but temporary workaround is to disable SSL verification:
git config --global http.sslVerify false
Note: This is generally not recommended in production due to security concerns.
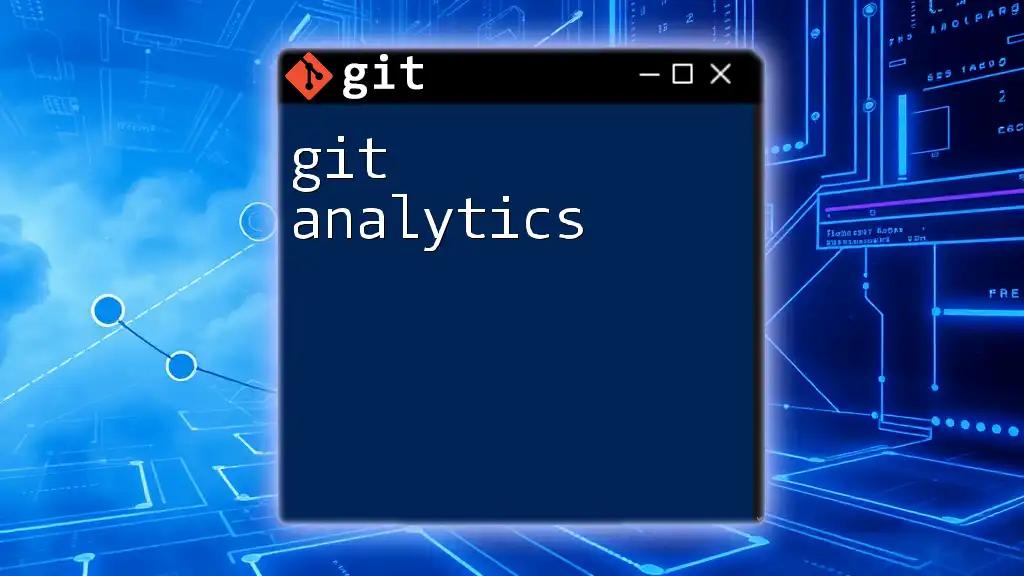
Troubleshooting Steps
Step 1: Update Git to the Latest Version
Keeping Git updated is paramount for performance and access capabilities. An older version may lead to compatibility issues with newer repository features. Depending on your operating system, you can find instructions to update Git on the official Git website.
Step 2: Reconfigure Your Remotes
Should the remote URLs be incorrect, reconfiguring them can resolve your access blocks. Use the earlier mentioned command to change the URLs to the correct ones.
Step 3: Verify Your Credentials
Inconsistent credentials can prevent access. For HTTPS users, it's vital to input the correct username and password. To streamline your authentication process, you can cache your credentials with:
git config --global credential.helper cache
This allows Git to store your login information for a brief period, minimizing the need to re-enter them.
Step 4: Testing SSH Connectivity
For those using SSH, testing connectivity is critical. Running the command:
ssh -T git@github.com
will inform you if your SSH connection is properly set up.
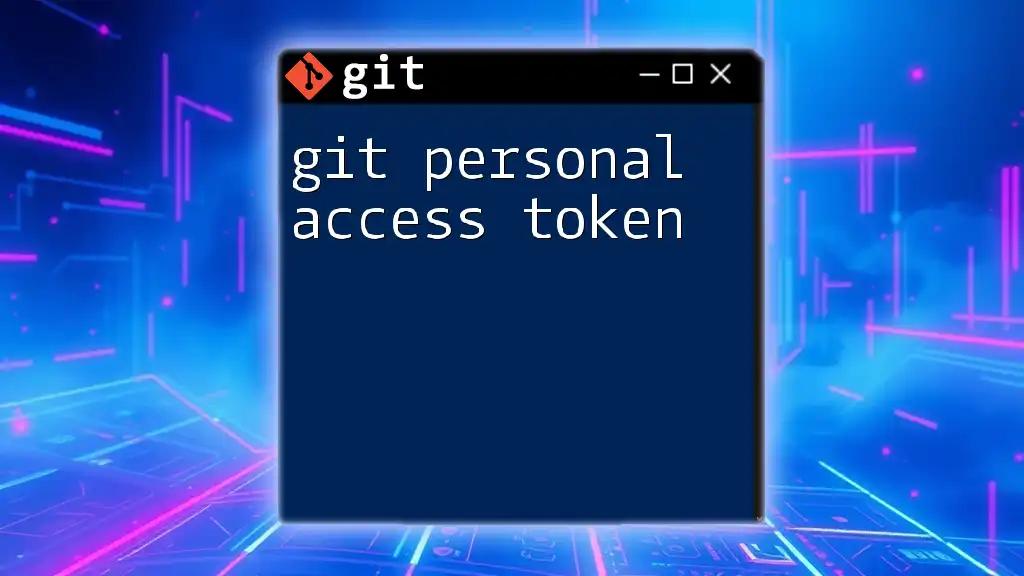
Advanced Solutions
Configure Git to Use HTTP Instead of HTTPS
In certain situations, switching from HTTPS to HTTP may help. This usually alleviates SSL-related issues. You can configure this setting with the command:
git config --global url."http://".insteadOf "https://"
This approach may compromise security, so use caution when sharing data.
DNS and Proxy Issues
If you're behind a corporate firewall or utilizing a proxy server, Git may struggle to connect to external repositories. To set Git to work through a proxy, use:
git config --global http.proxy http://proxyserver:port
This configuration will enable Git to tunnel through your proxy, ensuring connectivity to remote repositories.
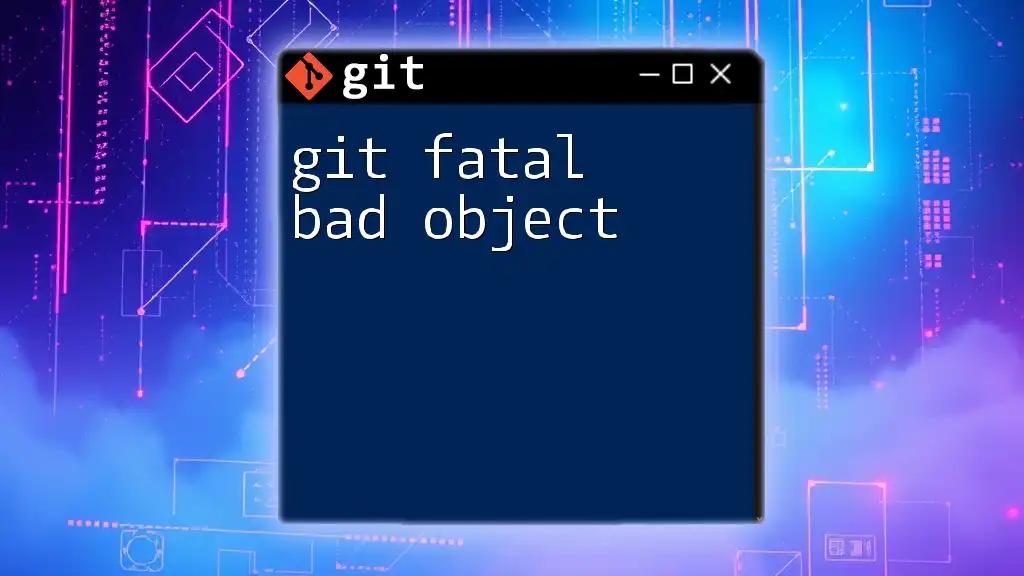
When to Seek Help
Checking GitHub Status
Occasionally, the issue may not originate from your end. Before digging too deep, verify whether Git services are experiencing downtime by checking the [GitHub status page](https://www.githubstatus.com).
Utilizing Git Community Resources
When all else fails, reaching out to the Git community can be invaluable. Platforms such as Stack Overflow or the GitHub Community can provide insight and solutions from developers who may have faced similar issues.

Conclusion
Dealing with the "git fatal: unable to access" error can initially seem daunting, but understanding the root causes and taking systematic troubleshooting steps can greatly simplify the process. Remember that networking basics like checking your internet connection can save time, and always ensure your Git installation is up to date. Should problems persist, don’t hesitate to seek help from the community or check for service disruptions. With these tools and knowledge in hand, you'll find managing Git commands much more accessible.

Additional Resources
For further claims on Git functionality, you can explore the official [Git documentation](https://git-scm.com/doc), ensuring you're up-to-date with the latest best practices and command line usage. Happy coding!